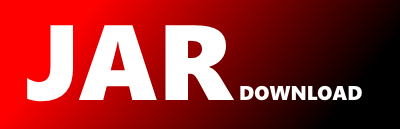
com.github.dakusui.enumerator.Combinator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of enumerator Show documentation
Show all versions of enumerator Show documentation
A mathematical enumeration library for Java
package com.github.dakusui.enumerator;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class Combinator extends Enumerator {
static class CDivResult {
long mod;
int quotient;
}
private static List arrayList(List items) {
if (items instanceof ArrayList) {
return items;
}
return new ArrayList(items);
}
private static void cdiv(CDivResult result, long index, int n, int k) {
int q = 0;
for (int nn = n-1; nn >= k-1; nn--) {
long nnCk_1 = nCk(nn, k-1);
if (index < nnCk_1) {
result.mod = index;
result.quotient = q;
break;
}
index -= nnCk_1;
q++;
}
}
private static int[] index2locator(long index, int n, int k) {
int[] ret = new int[k];
CDivResult result = new CDivResult();
for (int i = 0; i < k; i ++) {
cdiv(result, index, n, k-i);
ret[i] = result.quotient;
index = result.mod;
n -= (result.quotient + 1);
}
return ret;
}
public Combinator(List items, int k) {
super(arrayList(items), k);
}
@Override
protected List get_Protected(long index) {
List ret = new LinkedList();
List tmp = new ArrayList(this.items);
int[] locator = index2locator(index, items.size(), k);
for (int i : locator) {
T last = null;
for (int j = 0; j <= i; j++) {
last = tmp.remove(0);
}
ret.add(last);
}
return ret;
}
@Override
public long size() {
return nCk(this.items.size(), this.k);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy