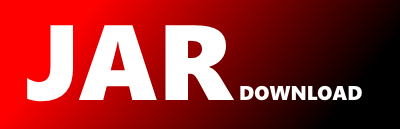
com.github.dakusui.floorplan.component.Operator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of floorplan Show documentation
Show all versions of floorplan Show documentation
A library to model a heterogeneous and distributed software
system for testing
package com.github.dakusui.floorplan.component;
import com.github.dakusui.actionunit.core.Action;
import com.github.dakusui.actionunit.core.Context;
import com.github.dakusui.floorplan.exception.Exceptions;
import java.util.function.Function;
public interface Operator extends Function, Component.ActionFactory> {
/**
* This enumerates categories of actions that can be performed on a component.
*/
enum Type {
/**
* An operation that belongs to this category installs a component from which
* it is created.
*/
INSTALL,
/**
* An operation that belongs to this category starts a component from which
* it is created.
*/
START,
/**
* An operation that belongs to this category stops a component from which
* it is created.
*/
STOP,
/**
* An operation that belongs to this category kills a component from which
* it is created.
*/
NUKE,
/**
* An operation that belongs to this category uninstalls a component from which
* it is created.
*/
UNINSTALL
}
Type type();
interface Factory extends Function, Operator> {
static Factory of(Type type, Function, Function> func) {
return new Factory() {
@Override
public Operator apply(ComponentSpec aComponentSpec) {
return new Operator() {
@Override
public Type type() {
return type;
}
@Override
public Component.ActionFactory apply(Component component) {
return context -> context.named(
String.format("%s %s", type, component),
func.apply(component).apply(context)
);
}
@Override
public String toString() {
return String.format("operator(%s %s)", type, aComponentSpec);
}
};
}
@Override
public Type type() {
return type;
}
};
}
static Factory nop(Type type) {
return of(
type,
component -> Context::nop
);
}
static Factory unsupported(Type type) {
return of(
type,
component -> context -> {
throw Exceptions.throwUnsupportedOperation(String.format("This operation is not supported by '%s'", component));
}
);
}
Type type();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy