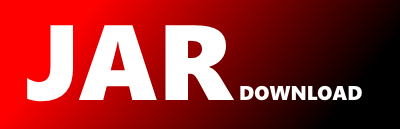
com.github.dakusui.jcunit8.core.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcunit Show documentation
Show all versions of jcunit Show documentation
Automated combinatorial testing framework on top of JUnit
The newest version!
package com.github.dakusui.jcunit8.core;
import com.github.dakusui.jcunit.core.tuples.Tuple;
import com.github.dakusui.jcunit8.exceptions.FrameworkException;
import org.junit.runners.Parameterized;
import org.junit.runners.model.FrameworkMethod;
import org.junit.runners.model.TestClass;
import java.io.PrintStream;
import java.lang.annotation.Annotation;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
import java.util.function.Predicate;
import static com.github.dakusui.jcunit.core.reflect.ReflectionUtils.getMethod;
import static com.github.dakusui.jcunit8.exceptions.FrameworkException.unexpectedByDesign;
import static java.util.Collections.singletonList;
public enum Utils {
;
public static PrintStream out = System.out;
public static Function printer() {
return printer(Object::toString);
}
public static Function printer(Function formatter) {
return t -> {
System.out.println(formatter.apply(t));
return t;
};
}
@SuppressWarnings("unchecked")
public static T print(T data) {
return (T) printer().apply(data);
}
public static List unique(List in) {
return new ArrayList<>(new LinkedHashSet<>(in));
}
public static int sizeOfIntersection(Set a, Set b) {
Set lhs;
Set rhs;
if (a.size() > b.size()) {
lhs = b;
rhs = a;
} else {
lhs = a;
rhs = b;
}
int ret = 0;
for (T each : lhs) {
if (rhs.contains(each))
ret++;
}
return ret;
}
public static Tuple project(List keys, Tuple from) {
Tuple.Builder builder = new Tuple.Builder();
keys.forEach((String key) -> builder.put(key, from.get(key)));
return builder.build();
}
public static TestClass createTestClassMock(final TestClass testClass) {
return new TestClass(testClass.getJavaClass()) {
@Override
public List getAnnotatedMethods(final Class extends Annotation> annClass) {
if (Parameterized.Parameters.class.equals(annClass)) {
return singletonList(createDummyFrameworkMethod());
}
return super.getAnnotatedMethods(annClass);
}
private FrameworkMethod createDummyFrameworkMethod() {
return new FrameworkMethod(getDummyMethod()) {
public boolean isStatic() {
return true;
}
@Override
public Object invokeExplosively(Object target, Object... params) {
return new Object[] {};
}
@SuppressWarnings("unchecked")
@Override
public T getAnnotation(Class annotationType) {
FrameworkException.checkCondition(Parameterized.Parameters.class.equals(annotationType));
return (T) new Parameterized.Parameters() {
@Override
public Class extends Annotation> annotationType() {
return Parameterized.Parameters.class;
}
@Override
public String name() {
return "{index}";
}
};
}
};
}
private Method getDummyMethod() {
return getToStringMethod(Object.class);
}
};
}
private static Method getToStringMethod(Class> klass) {
return getMethod(klass, "toString");
}
public static String className(Class klass) {
return className(klass, "");
}
private static String className(Class klass, String work) {
String canonicalName = klass.getCanonicalName();
if (canonicalName != null)
return canonicalName;
return className(klass.getEnclosingClass(), work + "$");
}
/**
* Creates and returns an instance of a class represented by {@code TestClass}.
*
* @param testClass Must be validated beforehand.
* @return created instance.
*/
public static Object createInstanceOf(TestClass testClass) {
try {
return testClass.getOnlyConstructor().newInstance();
} catch (InstantiationException | IllegalAccessException | InvocationTargetException e) {
throw unexpectedByDesign(e);
}
}
public static , E> Predicate conjunct(Iterable predicates) {
Predicate ret = tuple -> true;
for (Predicate each : predicates) {
ret = ret.and(each);
}
return ret;
}
public static Function memoize(Function function) {
Map memo = new ConcurrentHashMap<>();
return t -> memo.computeIfAbsent(t, function);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy