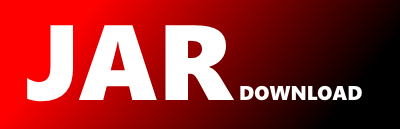
com.github.dakusui.jcunit8.factorspace.fsm.Player Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcunit Show documentation
Show all versions of jcunit Show documentation
Automated combinatorial testing framework on top of JUnit
The newest version!
package com.github.dakusui.jcunit8.factorspace.fsm;
import com.github.dakusui.jcunit.fsm.Action;
import com.github.dakusui.jcunit.fsm.Args;
import com.github.dakusui.jcunit.fsm.Expectation;
import com.github.dakusui.jcunit.fsm.State;
import java.util.function.Predicate;
import static org.junit.Assert.assertThat;
import static org.junit.Assert.assertTrue;
public interface Player {
void visit(Stimulus stimulus);
void visit(Edge edge);
void visit(Sequence sequence);
void visit(Scenario scenario);
abstract class Base implements Player {
protected final SUT sut;
public Base(SUT sut) {
this.sut = sut;
}
@Override
public void visit(Stimulus stimulus) {
throw new UnsupportedOperationException();
}
@Override
public void visit(Sequence sequence) {
for (Edge each : sequence) {
each.accept(this);
}
}
@Override
public void visit(Scenario scenario) {
scenario.setUp().accept(this);
scenario.main().accept(this);
}
public void play(Scenario scenario) {
if (!scenario.setUp().isEmpty())
scenario.setUp().get(0).from.check(sut);
visit(scenario);
}
}
class Simple extends Base {
public Simple(SUT sut) {
super(sut);
}
@Override
public void visit(Edge edge) {
Expectation expectation = edge.from.expectation(edge.action, edge.args);
try {
assertThat(
edge.action.perform(sut, edge.args),
expectation.getType().returnedValueMatcher(getOutputChecker(sut, edge.from, edge.action, edge.args))
);
checkState(expectation);
} catch (AssertionError e) {
throw e;
} catch (Throwable throwable) {
assertThat(
throwable,
expectation.getType().thrownExceptionMatcher(getExceptionChecker(sut, edge.from, edge.action, edge.args))
);
checkState(expectation);
}
}
private void checkState(Expectation expectation) {
assertTrue(
String.format(
"SUT '%s' is not in state '%s'",
sut,
expectation.state
),
expectation.state.check(sut)
);
}
protected Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy