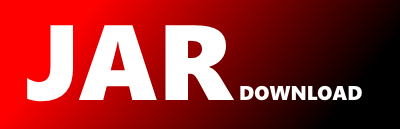
com.github.dakusui.jcunit8.testsuite.TupleSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcunit Show documentation
Show all versions of jcunit Show documentation
Automated combinatorial testing framework on top of JUnit
The newest version!
package com.github.dakusui.jcunit8.testsuite;
import com.github.dakusui.jcunit.core.tuples.Tuple;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.Set;
import java.util.stream.Stream;
public interface TupleSet extends Set {
TupleSet cartesianProduct(TupleSet tupleSet);
Stream streamCartesianProduct(TupleSet tupleSet);
class Impl extends LinkedHashSet implements TupleSet {
public Impl(Collection tuples) {
this.addAll(tuples);
}
@Override
public TupleSet cartesianProduct(TupleSet rhs) {
TupleSet.Builder builder = new TupleSet.Builder();
for (Tuple eachFromLhs : this) {
for (Tuple eachFromRhs : rhs) {
builder.add(new Tuple.Builder().putAll(eachFromLhs).putAll(eachFromRhs).build());
}
}
return builder.build();
}
@Override
public Stream streamCartesianProduct(TupleSet tupleSet) {
Stream lhs = this.stream();
return lhs.flatMap(
eachFromLhs -> tupleSet.stream().map(
eachFromRhs -> Tuple.builder().putAll(
eachFromLhs
).putAll(
eachFromRhs
).build()
)
);
}
}
class Builder {
private final Set work;
public Builder() {
this.work = new LinkedHashSet<>();
}
public Builder addAll(Collection tuples) {
this.work.addAll(tuples);
return this;
}
public Builder add(Tuple tuple) {
this.work.add(tuple);
return this;
}
public TupleSet build() {
return new Impl(this.work);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy