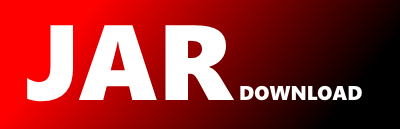
com.github.dakusui.jcunit8.exceptions.TestDefinitionException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcunit Show documentation
Show all versions of jcunit Show documentation
Automated combinatorial testing framework on top of JUnit
package com.github.dakusui.jcunit8.exceptions;
import com.github.dakusui.jcunit.core.tuples.Tuple;
import com.github.dakusui.jcunit8.factorspace.Constraint;
import java.util.List;
import java.util.function.Predicate;
import java.util.function.Supplier;
import static java.lang.String.format;
/**
* Indicates user's artifact (Typically test classes, annotated with
* {@literal @}{@code Runwith(JCUnit.class)}), is invalid.
*/
public class TestDefinitionException extends BaseException {
private TestDefinitionException(String message) {
super(message);
}
private TestDefinitionException(String message, Throwable e) {
super(message, e);
}
public static TestDefinitionException wrap(Throwable t) {
throw new TestDefinitionException(format("Test definition is not valid: %s", t.getMessage()), t);
}
public static TestDefinitionException impossibleConstraint(List constraints) {
throw new TestDefinitionException(format("Constraints '%s' did not become true.", constraints));
}
public static TestDefinitionException fsmDoesNotHaveRouteToSpecifiedState(Object destinationState, String fsmName, Object fsmModel) {
throw new TestDefinitionException(format("No route to '%s' was found on FSM:'%s'(%s)", destinationState, fsmName, fsmModel));
}
public static TestDefinitionException failedToCover(String factorName, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy