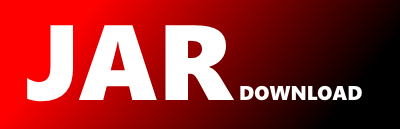
com.github.dakusui.scriptiveunit.model.statement.Statement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scriptiveunit Show documentation
Show all versions of scriptiveunit Show documentation
Action based junit test runner library
package com.github.dakusui.scriptiveunit.model.statement;
import com.github.dakusui.scriptiveunit.exceptions.SyntaxException;
import com.github.dakusui.scriptiveunit.exceptions.TypeMismatch;
import com.github.dakusui.scriptiveunit.model.Stage;
import com.github.dakusui.scriptiveunit.model.TestSuiteDescriptor;
import com.github.dakusui.scriptiveunit.model.func.Func;
import com.github.dakusui.scriptiveunit.model.func.FuncHandler;
import com.github.dakusui.scriptiveunit.model.func.FuncInvoker;
import com.google.common.collect.Lists;
import java.util.List;
import java.util.Objects;
import static com.github.dakusui.scriptiveunit.exceptions.TypeMismatch.headOfCallMustBeString;
import static java.util.Objects.requireNonNull;
public interface Statement {
Func execute(FuncInvoker invoker);
interface Atom extends Statement {
}
interface Nested extends Statement {
Form getForm();
Arguments getArguments();
}
class Factory {
private final Form.Factory formFactory;
private final Arguments.Factory argumentsFactory;
private final Func.Factory funcFactory;
private final FuncHandler funcHandler;
public Factory(TestSuiteDescriptor testSuiteDescriptor) {
this.funcHandler = new FuncHandler();
this.funcFactory = new Func.Factory(funcHandler);
this.argumentsFactory = new Arguments.Factory(this);
this.formFactory = new Form.Factory(testSuiteDescriptor, funcFactory, this);
}
public Statement create(Object object) throws TypeMismatch {
if (isAtom(object))
return (Atom) invoker -> (Func
© 2015 - 2025 Weber Informatics LLC | Privacy Policy