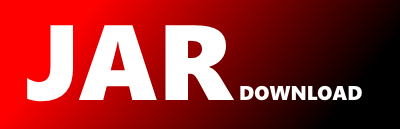
com.github.ojil.algorithm.Gray8Crop Maven / Gradle / Ivy
Show all versions of ojil-core Show documentation
/*
* Gray8Crop.java
*
* Created on August 27, 2006, 2:38 PM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*
* Copyright 2007 by Jon A. Webb
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the Lesser GNU General Public License
* along with this program. If not, see .
*
*/
package com.github.ojil.algorithm;
import com.github.ojil.core.Error;
import com.github.ojil.core.Gray8Image;
import com.github.ojil.core.Image;
import com.github.ojil.core.PipelineStage;
import com.github.ojil.core.Rect;
/**
* Pipeline stage crops a Gray8Image to a given rectangular cropping window.
*
* @author webb
*/
public class Gray8Crop extends PipelineStage {
int cHeight; /* height of cropping window */
int cWidth; /* width of cropping window */
int cX; /* left edge of cropping window */
int cY; /* top of cropping window */
/** Creates a new instance of Gray8Crop. The cropping window
* is specified here.
*
* @param x left edge of cropping window
* @param y top edge of cropping window
* @param width width of cropping window
* @param height height of cropping window
* @throws com.github.ojil.core.Error if the top left corner of the
* window is negative, or the window area is non-positive.
*/
public Gray8Crop(
int x,
int y,
int width,
int height) throws com.github.ojil.core.Error {
setWindow(x, y, width, height);
}
/** Creates a new instance of Gray8Crop. The cropping window
* is specified here.
*
* @param r Rect to crop to.
* @throws com.github.ojil.core.Error if the top left corner of the
* window is negative, or the window area is non-positive.
*/
public Gray8Crop(Rect r) throws com.github.ojil.core.Error {
setWindow(r.getLeft(), r.getTop(), r.getWidth(), r.getHeight());
}
/** Crops the input gray image to the cropping window that was
* specified in the constructor.
*
* @param image the input image.
* @throws com.github.ojil.core.Error if the cropping window
* extends outside the input image, or the input image
* is not a Gray8Image.
*/
public void push(Image image) throws com.github.ojil.core.Error {
if (!(image instanceof Gray8Image)) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.IMAGE_NOT_GRAY8IMAGE,
image.toString(),
null,
null);
}
Gray8Image imageInput = (Gray8Image) image;
if (this.cX + this.cWidth > image.getWidth() ||
this.cY + this.cHeight > image.getHeight()) {
throw new Error(
Error.PACKAGE.CORE,
com.github.ojil.core.ErrorCodes.BOUNDS_OUTSIDE_IMAGE,
image.toString(),
this.toString(),
null);
}
Gray8Image imageResult = new Gray8Image(this.cWidth,this.cHeight);
Byte[] src = imageInput.getData();
Byte[] dst = imageResult.getData();
for (int i=0; i