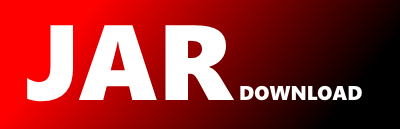
com.github.ojil.algorithm.Gray8RectStretch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ojil-core Show documentation
Show all versions of ojil-core Show documentation
Open Java Imaging Library.
/*
* Gray8RectStretch.java
*
* Created on September 9, 2006, 8:08 AM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*
* Copyright 2007 by Jon A. Webb
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the Lesser GNU General Public License
* along with this program. If not, see .
*
*/
package com.github.ojil.algorithm;
import com.github.ojil.core.Error;
import com.github.ojil.core.Gray8Image;
import com.github.ojil.core.Image;
import com.github.ojil.core.PipelineStage;
/** Pipeline stage stretches an image to a larger rectangular size with
* bilinear interpolation. For more information on this and other image
* warps, see George Wolberg's excellent book, "Digital Image Warping",
* Wiley-IEEE Computer Society Press, 1990.
* @author webb
*/
public class Gray8RectStretch extends PipelineStage {
private int cHeight;
private int cWidth;
/** Creates a new instance of Gray8RectStretch.
*
* @param cWidth new image width
* @param cHeight new image height
* @throws com.github.ojil.core.Error if either is less than or equal to zero.
*/
public Gray8RectStretch(int cWidth, int cHeight)
throws com.github.ojil.core.Error {
setWidth(cWidth);
setHeight(cHeight);
}
/** Gets current target height
*
* @return current height
*/
public int getHeight() {
return this.cHeight;
}
/** Gets current target width
*
* @return current width
*/
public int getWidth() {
return this.cWidth;
}
/** Bilinear interpolation to stretch image to (cWidth, cHeight).
* Does this in two passes, for more efficient computation.
*
* @param image the input image
* @throws com.github.ojil.core.Error if input image is not gray 8 bits,
* or the input image size is larger than the target size. This class
* does not do subsampling, only interpolation.
*/
public void push(Image image) throws com.github.ojil.core.Error {
if (!(image instanceof Gray8Image)) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.IMAGE_NOT_GRAY8IMAGE,
image.toString(),
null,
null);
}
if (image.getWidth() > this.cWidth || image.getHeight() > this.cHeight) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.STRETCH_OUTPUT_SMALLER_THAN_INPUT,
image.toString(),
new Integer(this.cWidth).toString(),
new Integer(this.cHeight).toString());
}
Gray8Image input = (Gray8Image) image;
/** we do the stretch in to passes, one horizontal and the other
* vertical. This leads to less computation than doing it in one
* pass, because the calculation of the interpolation weights
* has to happen only once.
*/
/* horizontal stretch */
Gray8Image horiz = stretchHoriz(input);
Gray8Image result = stretchVert(horiz);
super.setOutput(result);
}
/** Changes target height
*
* @param cHeight the new target height.
* @throws com.github.ojil.core.Error if height is not positive
*/
public void setHeight(int cHeight) throws com.github.ojil.core.Error {
if (cHeight <= 0) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.OUTPUT_IMAGE_SIZE_NEGATIVE,
new Integer(cHeight).toString(),
null,
null);
}
this.cHeight = cHeight;
}
/** Changes target width
*
* @param cWidth the new target width.
* @throws com.github.ojil.core.Error if height is not positive
*/
public void setWidth(int cWidth) throws com.github.ojil.core.Error {
if (cWidth <= 0) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.OUTPUT_IMAGE_SIZE_NEGATIVE,
new Integer(cWidth).toString(),
null,
null);
}
this.cWidth = cWidth;
}
/** Horizontal stretch. Stretches the input from
* (input.getWidth(),input.getHeight()) to (this.cWidth,input.getHeight())
*
* @param input the input image
* @return the stretched image
*/
private Gray8Image stretchHoriz(Gray8Image input) {
/* horizontal stretch */
Gray8Image horiz = new Gray8Image(this.cWidth, input.getHeight());
Byte[] inData = input.getData();
Byte[] outData = horiz.getData();
for (int j=0; j> 8);
}
} else {
/* adjust cX so we will use the last column of the input
* image for interpolating beyond its right edge, without
* accessing a value outside the array bounds. In other
* words, for fractional input positions beyond input.getWidth()-1
* we use input.getWidth()-1 as the column.
*/
cX = input.getWidth() - 2;
for (int i=0; i> 8);
}
} else {
/* adjust cX so we will use the last row of the input
* image for interpolating beyond its bottom edge, without
* accessing a value outside the array bounds. In other
* words, for fractional input positions beyond input.getHeight()-1
* we use input.getHeight()-1 as the row.
*/
cY = input.getHeight() - 2;
for (int j=0; j
© 2015 - 2024 Weber Informatics LLC | Privacy Policy