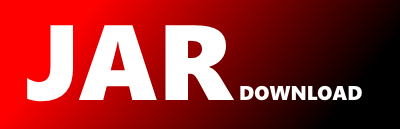
com.github.ojil.algorithm.Gray8VertVar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ojil-core Show documentation
Show all versions of ojil-core Show documentation
Open Java Imaging Library.
/*
* Gray8VertVar.java
*
* Created on February 3, 2008, 4:32, PM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*
* Copyright 2008 by Jon A. Webb
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the Lesser GNU General Public License
* along with this program. If not, see .
*
*/
package com.github.ojil.algorithm;
import com.github.ojil.core.Error;
import com.github.ojil.core.Gray16Image;
import com.github.ojil.core.Gray8Image;
import com.github.ojil.core.Image;
import com.github.ojil.core.PipelineStage;
/**
* Computes the variance of pixels vertically distributed around
* the current pixel.
* @author webb
*/
public class Gray8VertVar extends PipelineStage {
/**
* The window size -- pixels within nWindow of the current
* pixel are included in the window.
*/
int nWindow;
/**
* The output image.
*/
Gray16Image g16 = null;
/**
* Creates a new instance of Gray8VertVar
* @param nWindow height of window to calculate variance over.
*/
public Gray8VertVar(int nWindow) {
this.nWindow = nWindow;
}
/** Compute the vertical variance of pixels within nWindow
* of the current pixel.
* @param image the input Gray8Image
* @throws com.github.ojil.core.Error if image is not a Gray8Image
*/
public void push(Image image) throws com.github.ojil.core.Error {
if (!(image instanceof Gray8Image)) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.IMAGE_NOT_GRAY8IMAGE,
image.toString(),
null,
null);
}
if (this.g16 == null ||
this.g16.getWidth() != image.getWidth() ||
this.g16.getHeight() != image.getHeight()) {
this.g16 = new Gray16Image(
image.getWidth(),
image.getHeight());
}
Gray8Image input = (Gray8Image) image;
Byte[] bIn = input.getData();
int cHeight = input.getHeight();
int cWidth = input.getWidth();
Short[] sOut = g16.getData();
for (int i=0; i= this.nWindow) {
nSum -= bIn[(j - this.nWindow)*cWidth + i];
nSumSq -= bIn[(j - this.nWindow)*cWidth + i] *
bIn[(j - this.nWindow)*cWidth + i];
nCount --;
}
short nVar = (short)
Math.min(Short.MAX_VALUE,
(nSumSq - nSum * nSum / nCount) / (nCount - 1));
sOut[j*cWidth + i] = nVar;
}
}
super.setOutput(g16);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy