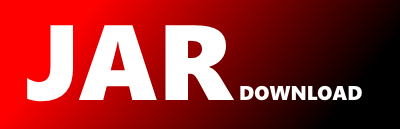
com.github.ojil.algorithm.RgbSelectGray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ojil-core Show documentation
Show all versions of ojil-core Show documentation
Open Java Imaging Library.
/*
* RgbSelectGray.java
*
* Created on August 27, 2006, 11:33 AM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*
* Copyright 2007 by Jon A. Webb
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the Lesser GNU General Public License
* along with this program. If not, see .
*
*/
package com.github.ojil.algorithm;
import com.github.ojil.core.Error;
import com.github.ojil.core.Gray8Image;
import com.github.ojil.core.Image;
import com.github.ojil.core.PipelineStage;
import com.github.ojil.core.RgbImage;
import com.github.ojil.core.RgbVal;
/**
*
* Transforms a RgbImage into a Gray8Image by selecting one of the three
* bands. The pixel value chosen is adjusted from the range 0→255 to the
* range -128→127.
*
*
* Usage:
*
*
* RgbImage imageRgb = ...;
* RgbSelectGray rgb = new RgbSelectGray(RgbSelectGray.RED);
* rgb.push(imageRgb);
*
*
* @author webb
*/
public class RgbSelectGray extends PipelineStage {
/* In the absence of enums in version 1.3 we use three empty singleton
* classes to represent the color choice.
*/
/**
* Used to represent the three colors red, green, or blue.
*/
public static class ColorClass {
private String name;
private static final ColorClass RED = new ColorClass("RED");
private static final ColorClass GREEN = new ColorClass("GREEN");
private static final ColorClass BLUE = new ColorClass("BLUE");
private ColorClass(String name) {
this.name = name;
}
/**
* Represents the color red.
* @return A ColorClass object that represents the color red.
*/
public static ColorClass Red() {
return RED;
}
/**
* Represents the color green.
* @return A ColorClass object that represents the color green.
*/
public static ColorClass Green() {
return GREEN;
}
/**
* Represents the color blue.
* @return A ColorClass object that represents the color blue.
*/
public static ColorClass Blue() {
return BLUE;
}
/**
* Returns a string representation of the RgbSelectGray operation.
* @return a String representing the RgbSelectGray operation.
*/
public String toString() {
return name;
}
};
/**
* The class represents the color red. It is used like an enumerated value when
* calling the RgbSelectGray constructor.
*/
public static final ColorClass RED = ColorClass.Red();
/**
* The class represents the color green. It is used like an enumerated value when
* calling the RgbSelectGray constructor.
*/
public static final ColorClass GREEN = ColorClass.Green();
/**
* The class represents the color blue. It is used like an enumerated value when
* calling the RgbSelectGray constructor.
*/
public static final ColorClass BLUE = ColorClass.Blue();
/**
* Aliases for red, green, and blue used when we're thinking of the RGB image
* as an HSV image.
*/
public static final ColorClass HUE = ColorClass.Red();
public static final ColorClass SATURATION = ColorClass.Green();
public static final ColorClass VALUE = ColorClass.Blue();
private ColorClass colorChosen;
/**
* Creates a new instance of RgbSelectGray.
* @param color the color selected from the color image to create the gray image.
* @throws com.github.ojil.core.Error if color is not RED, GREEN, or BLUE.
*/
public RgbSelectGray(ColorClass color) throws com.github.ojil.core.Error {
setColor(color);
}
/**
* Returns the current color selected.
*
* @return the current color, as a ColorClass object.
*/
public ColorClass getColor() {
return this.colorChosen;
}
/** Convert a color image to gray by selecting one of the color
* bands: red, green, or blue. The band selected is chosen in the
* class constructor. The gray pixel value is adjusted so its range
* is from -128→127 instead of the 0-255 value in the ARGB word.
*
* @param image the input image
* @throws com.github.ojil.core.Error if the input image is not a color
* image.
*/
public void push(Image image) throws com.github.ojil.core.Error {
if (!(image instanceof RgbImage)) {
throw new Error(
Error.PACKAGE.ALGORITHM,
ErrorCodes.IMAGE_NOT_RGBIMAGE,
image.toString(),
null,
null);
}
RgbImage rgb = (RgbImage) image;
Integer[] rgbData = rgb.getData();
Gray8Image gray = new Gray8Image(image.getWidth(), image.getHeight());
Byte[] grayData = gray.getData();
if (colorChosen.equals(RED)) {
for (int i=0; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy