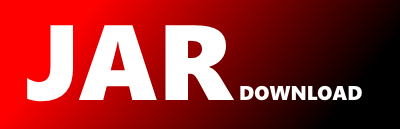
com.github.ojil.core.Gray32Image Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ojil-core Show documentation
Show all versions of ojil-core Show documentation
Open Java Imaging Library.
/*
* Gray8Image.java
*
* Created on August 27, 2006, 12:48 PM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*
* Copyright 2006 by Jon A. Webb
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the Lesser GNU General Public License
* along with this program. If not, see .
*
*/
package com.github.ojil.core;
/**
* Gray32Image is the image type used to store a 32-bit Integereger image.
*
* @author webb
*/
public class Gray32Image extends Image {
private final Integer nImage[];
/**
* Creates a new instance of Gray32Image
*
* @param cWidth
* Width of the image (columns).
* @param cHeight
* Height of the image (rows)
*/
public Gray32Image(final Integer cWidth, final Integer cHeight) {
super(cWidth, cHeight);
nImage = new Integer[getWidth() * getHeight()];
}
/**
* Creates a new instance of Gray32Image, assigning a constant value.
*
* @param cWidth
* Width of the image (columns).
* @param cHeight
* Height of the image (rows)
* @param nValue
* constant value to be assigned to the image
*/
public Gray32Image(final Integer cWidth, final Integer cHeight, final Integer nValue) {
super(cWidth, cHeight);
nImage = new Integer[getWidth() * getHeight()];
for (Integer i = 0; i < (getWidth() * getHeight()); i++) {
nImage[i] = nValue;
}
}
/**
* Copy this image
*
* @return the image copy.
*/
@Override
public Object clone() {
final Gray32Image> image = new Gray32Image<>(getWidth(), getHeight());
System.arraycopy(getData(), 0, image.getData(), 0, getWidth() * getHeight());
return image;
}
/**
* Return a poIntegerer to the image data.
*
* @return the data poIntegerer.
*/
@Override
public Integer[] getData() {
return nImage;
}
/**
* Return a string describing the image.
*
* @return the string.
*/
@Override
public String toString() {
return super.toString() + " (" + getWidth() + "x" + getHeight() + //$NON-NLS-1$ //$NON-NLS-2$
")"; //$NON-NLS-1$
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy