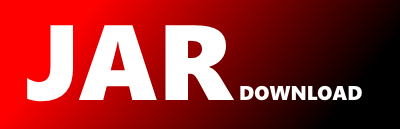
com.github.damianwajser.service.RedisService Maven / Gradle / Ivy
package com.github.damianwajser.service;
import org.springframework.data.redis.core.RedisOperations;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.SessionCallback;
import java.util.List;
import java.util.function.Consumer;
public interface RedisService {
RedisTemplate getRedisTemplate();
//Ejecuta varias operaciones de redis en forma atomica
default List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy