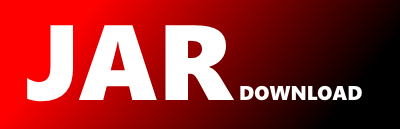
com.github.danielflower.mavenplugins.gitlog.GenerateMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-gitlog-plugin Show documentation
Show all versions of maven-gitlog-plugin Show documentation
Generates a changelog based on commits to a git repository in text and HTML format showing the changes
that are included in each version. A possible use of this is to include these changelogs when packaging your
maven project so that you have an accurate list of commits that the current package includes.
package com.github.danielflower.mavenplugins.gitlog;
import com.github.danielflower.mavenplugins.gitlog.renderers.*;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* Goal which generates a changelog based on commits made to the current git repo.
*/
@Mojo(
name = "generate",
defaultPhase = LifecyclePhase.PREPARE_PACKAGE,
aggregator = true // the plugin should only run once against the aggregator pom
)
public class GenerateMojo extends AbstractMojo {
/**
* The directory to put the reports in. Defaults to the project build directory (normally target).
*/
@Parameter(property = "project.build.directory")
private File outputDirectory;
/**
* The title of the reports. Defaults to: ${project.name} v${project.version} changelog
*/
@Parameter(defaultValue = "${project.name} v${project.version} changelog")
private String reportTitle;
/**
* If true, then a plain text changelog will be generated.
*/
@Parameter(defaultValue = "true")
private boolean generatePlainTextChangeLog;
/**
* The filename of the plain text changelog, if generated.
*/
@Parameter(defaultValue = "changelog.txt")
private String plainTextChangeLogFilename;
/**
* If true, then a markdown changelog will be generated.
*/
@Parameter(defaultValue = "false")
private boolean generateMarkdownChangeLog;
/**
* The filename of the markdown changelog, if generated.
*/
@Parameter(defaultValue = "changelog.md")
private String markdownChangeLogFilename;
/**
* If true, then a simple HTML changelog will be generated.
*/
@Parameter(defaultValue = "true")
private boolean generateSimpleHTMLChangeLog;
/**
* The filename of the simple HTML changelog, if generated.
*/
@Parameter(defaultValue = "changelog.html")
private String simpleHTMLChangeLogFilename;
/**
* If true, then an HTML changelog which contains only a table element will be generated.
* This incomplete HTML page is suitable for inclusion in other webpages, for example you
* may want to embed it in a wiki page.
*/
@Parameter(defaultValue = "false")
private boolean generateHTMLTableOnlyChangeLog;
/**
* The filename of the HTML table changelog, if generated.
*/
@Parameter(defaultValue = "changelogtable.html")
private String htmlTableOnlyChangeLogFilename;
/**
* If true, then a JSON changelog will be generated.
*/
@Parameter(defaultValue = "true")
private boolean generateJSONChangeLog;
/**
* The filename of the JSON changelog, if generated.
*/
@Parameter(defaultValue = "changelog.json")
private String jsonChangeLogFilename;
/**
* If true, the changelog will be printed to the Maven build log during packaging.
*/
@Parameter(defaultValue = "false")
private boolean verbose;
/**
* Used to create links to your issue tracking system for HTML reports. If unspecified, it will try to use the value
* specified in the issueManagement section of your project's POM. The following values are supported:
* a value containing the string "github" for the GitHub Issue tracking software;
* a value containing the string "jira" for Jira tracking software.
* Any other value will result in no links being made.
*/
@Parameter(property = "project.issueManagement.system")
private String issueManagementSystem;
/**
* Used to create links to your issue tracking system for HTML reports. If unspecified, it will try to use the value
* specified in the issueManagement section of your project's POM.
*/
@Parameter(property="project.issueManagement.url")
private String issueManagementUrl;
/**
* Used to set date format in log messages.
*/
@Parameter(defaultValue = "yyyy-MM-dd HH:mm:ss Z")
private String dateFormat;
/**
* If true, the changelog will include the full git message rather that the short git message
*/
@Parameter(defaultValue="false")
private boolean fullGitMessage;
/**
* Include in the changelog the commits after this parameter value.
*/
@Parameter(defaultValue="1970-01-01 00:00:00.0 AM")
private Date includeCommitsAfter;
public void execute() throws MojoExecutionException, MojoFailureException {
getLog().info("Generating changelog in " + outputDirectory.getAbsolutePath()
+ " with title " + reportTitle);
File f = outputDirectory;
if (!f.exists()) {
f.mkdirs();
}
List renderers;
try {
renderers = createRenderers();
} catch (IOException e) {
getLog().warn("Error while setting up gitlog renderers. No changelog will be generated.", e);
return;
}
Generator generator = new Generator(renderers, Defaults.COMMIT_FILTERS, getLog());
try {
generator.openRepository();
} catch (IOException e) {
getLog().warn("Error opening git repository. Is this Maven project hosted in a git repository? " +
"No changelog will be generated.", e);
return;
} catch (NoGitRepositoryException e) {
getLog().warn("This maven project does not appear to be in a git repository, " +
"therefore no git changelog will be generated.");
return;
}
if (!"".equals(dateFormat)) {
Formatter.setFormat(dateFormat, getLog());
}
try {
generator.generate(reportTitle, includeCommitsAfter);
} catch (IOException e) {
getLog().warn("Error while generating changelog. Some changelogs may be incomplete or corrupt.", e);
}
}
private List createRenderers() throws IOException {
ArrayList renderers = new ArrayList();
if (generatePlainTextChangeLog) {
renderers.add(new PlainTextRenderer(getLog(), outputDirectory, plainTextChangeLogFilename, fullGitMessage));
}
if (generateSimpleHTMLChangeLog || generateHTMLTableOnlyChangeLog || generateMarkdownChangeLog) {
MessageConverter messageConverter = getCommitMessageConverter();
if (generateSimpleHTMLChangeLog) {
renderers.add(new SimpleHtmlRenderer(getLog(), outputDirectory, simpleHTMLChangeLogFilename, fullGitMessage, messageConverter, false));
}
if (generateHTMLTableOnlyChangeLog) {
renderers.add(new SimpleHtmlRenderer(getLog(), outputDirectory, htmlTableOnlyChangeLogFilename, fullGitMessage, messageConverter, true));
}
if (generateMarkdownChangeLog) {
renderers.add(new MarkdownRenderer(getLog(), outputDirectory, markdownChangeLogFilename, fullGitMessage, messageConverter));
}
}
if (generateJSONChangeLog) {
renderers.add(new JsonRenderer(getLog(), outputDirectory, jsonChangeLogFilename, fullGitMessage));
}
if (verbose) {
renderers.add(new MavenLoggerRenderer(getLog()));
}
return renderers;
}
private MessageConverter getCommitMessageConverter() {
getLog().debug("Trying to load issue tracking info: " + issueManagementSystem + " / " + issueManagementUrl);
MessageConverter converter = null;
try {
if (issueManagementUrl != null && issueManagementUrl.contains("://")) {
String system = ("" + issueManagementSystem).toLowerCase();
if (system.contains("jira")) {
converter = new JiraIssueLinkConverter(getLog(), issueManagementUrl);
} else if (system.contains("github")) {
converter = new GitHubIssueLinkConverter(getLog(), issueManagementUrl);
}
}
} catch (Exception ex) {
getLog().warn("Could not load issue management system information; no HTML links will be generated.", ex);
}
if (converter == null) {
converter = new NullMessageConverter();
}
getLog().debug("Using tracker " + converter.getClass().getSimpleName());
return converter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy