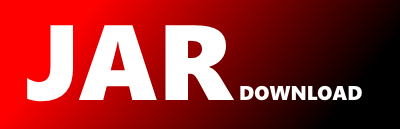
com.github.danielflower.mavenplugins.gitlog.renderers.SimpleHtmlRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-gitlog-plugin Show documentation
Show all versions of maven-gitlog-plugin Show documentation
Generates a changelog based on commits to a git repository in text and HTML format showing the changes
that are included in each version. A possible use of this is to include these changelogs when packaging your
maven project so that you have an accurate list of commits that the current package includes.
package com.github.danielflower.mavenplugins.gitlog.renderers;
import org.apache.commons.lang3.StringEscapeUtils;
import org.apache.maven.plugin.logging.Log;
import org.eclipse.jgit.revwalk.RevCommit;
import org.eclipse.jgit.revwalk.RevTag;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import static com.github.danielflower.mavenplugins.gitlog.renderers.Formatter.NEW_LINE;
public class SimpleHtmlRenderer extends FileRenderer {
private String title;
private String template;
protected StringBuilder tableHtml = new StringBuilder();
protected final MessageConverter messageConverter;
private final boolean tableOnly;
private final boolean fullGitMessage;
public SimpleHtmlRenderer(Log log, File targetFolder, String filename, boolean fullGitMessage, MessageConverter messageConverter, boolean tableOnly) throws IOException {
super(log, targetFolder, filename);
this.messageConverter = messageConverter;
this.tableOnly = tableOnly;
this.fullGitMessage = fullGitMessage;
if (!tableOnly) {
this.template = loadResourceToString("/html/SimpleHtmlTemplate.html");
}
}
protected static String htmlEncode(String input) {
input = StringEscapeUtils.escapeHtml4(input);
return input.replaceAll("\n", "
");
}
@Override
public void renderHeader(String reportTitle) throws IOException {
this.title = reportTitle;
tableHtml.append("\t")
.append(NEW_LINE)
.append("\t\t")
.append(NEW_LINE);
}
@Override
public void renderTag(RevTag tag) throws IOException {
tableHtml.append("\t\t")
.append(SimpleHtmlRenderer.htmlEncode(tag.getTagName()))
.append(" ")
.append(NEW_LINE);
}
@Override
public void renderCommit(RevCommit commit) throws IOException {
String date = Formatter.formatDateTime(commit.getCommitTime());
String message = null;
if (fullGitMessage){
message = messageConverter.formatCommitMessage(SimpleHtmlRenderer.htmlEncode(commit.getFullMessage()));
} else {
message = messageConverter.formatCommitMessage(SimpleHtmlRenderer.htmlEncode(commit.getShortMessage()));
}
String author = SimpleHtmlRenderer.htmlEncode(commit.getCommitterIdent().getName());
String committer = SimpleHtmlRenderer.htmlEncode(commit.getCommitterIdent().getName());
String authorHtml = "" + commit.getAuthorIdent().getName() + "";
if (!areSame(author, committer)) {
authorHtml += "and ";
}
tableHtml.append("\t\t")
.append("").append(date).append(" ")
.append("").append(message).append(" ")
.append("").append(authorHtml).append(" ")
.append(" ").append(NEW_LINE);
}
@Override
public void renderFooter() throws IOException {
tableHtml.append("\t\t")
.append(NEW_LINE)
.append("\t
")
.append(NEW_LINE);
if (tableOnly) {
writer.append(tableHtml.toString());
} else {
String html = template
.replace("{title}", htmlEncode(title))
.replace("{table}", tableHtml.toString());
writer.append(html);
}
}
private boolean areSame(String author, String committer) {
return ("" + author).toLowerCase().equals("" + committer.toLowerCase());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy