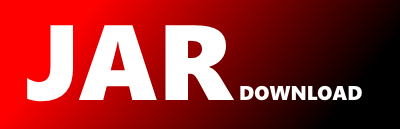
org.springframework.data.mongodb.datatables.DataTablesRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-data-mongodb-datatables Show documentation
Show all versions of spring-data-mongodb-datatables Show documentation
Spring Data MongoDB extension to work with the great jQuery plug-in DataTables (http://datatables.net/)
The newest version!
package org.springframework.data.mongodb.datatables;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.data.repository.NoRepositoryBean;
import java.io.Serializable;
import java.util.function.Function;
@NoRepositoryBean
public interface DataTablesRepository extends MongoRepository {
/**
* Returns the filtered list for the given {@link DataTablesInput}.
*
* @param input the {@link DataTablesInput} mapped from the Ajax request
* @return a {@link DataTablesOutput}
*/
DataTablesOutput findAll(DataTablesInput input);
/**
* Returns the filtered list for the given {@link DataTablesInput}.
*
* @param input the {@link DataTablesInput} mapped from the Ajax request
* @param additionalCriteria an additional {@link Criteria} to apply to the query (with
* an "AND" clause)
* @return a {@link DataTablesOutput}
*/
DataTablesOutput findAll(DataTablesInput input, Criteria additionalCriteria);
/**
* Returns the filtered list for the given {@link DataTablesInput}.
*
* @param input the {@link DataTablesInput} mapped from the Ajax request
* @param additionalCriteria an additional {@link Criteria} to apply to the query (with an "AND" clause)
* @param preFilteringCriteria a pre-filtering {@link Criteria} to apply to the query (with an "AND" clause)
* @return a {@link DataTablesOutput}
*/
DataTablesOutput findAll(DataTablesInput input, Criteria additionalCriteria, Criteria preFilteringCriteria);
/**
* Returns the filtered list for the given {@link DataTablesInput}.
*
* @param input the {@link DataTablesInput} mapped from the Ajax request
* @param converter the {@link Function} to apply to the results of the query
* @return a {@link DataTablesOutput}
*/
DataTablesOutput findAll(DataTablesInput input, Function converter);
/**
* Returns the filtered list for the given {@link DataTablesInput}.
*
* @param input the {@link DataTablesInput} mapped from the Ajax request
* @param additionalCriteria an additional {@link Criteria} to apply to the query (with an "AND" clause)
* @param preFilteringCriteria a pre-filtering {@link Criteria} to apply to the query (with an "AND" clause)
* @param converter the {@link Function} to apply to the results of the query
* @return a {@link DataTablesOutput}
*/
DataTablesOutput findAll(DataTablesInput input, Criteria additionalCriteria,
Criteria preFilteringCriteria, Function converter);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy