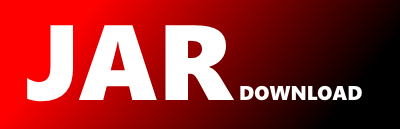
com.github.df.restypass.http.converter.JsonResponseConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resty-pass Show documentation
Show all versions of resty-pass Show documentation
High-Performance Restful Client Library
The newest version!
package com.github.df.restypass.http.converter;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.type.TypeFactory;
import com.github.df.restypass.util.JsonTools;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.lang.reflect.Type;
/**
* Json类型响应解析类
* Created by darrenfu on 17-7-19.
*/
public class JsonResponseConverter implements ResponseConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy