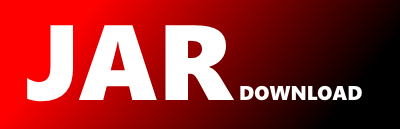
br.com.daruma.jna.UTIL Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of darumaframeworkjna Show documentation
Show all versions of darumaframeworkjna Show documentation
Wrapper to communication libraries DarumaFramework.dll (Windows) and libDarumaFramework.so (Linux) Java applications
The newest version!
package br.com.daruma.jna;
import com.sun.jna.Pointer;
public class UTIL {
private static DarumaFrameworkNative dfw = DarumaFrameworkNative.INSTANCE;
private static DarumaUtilitario darUtil = new DarumaUtilitario();
public static int comEnviarDados(String pszBytes, int iLenBytes) {
int iRetorno = dfw.comEnviarDados_Daruma(pszBytes, iLenBytes);
return iRetorno;
}
public static int comReceberDados(char[] pszBufferEntrada) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.comReceberDados_Daruma(pValor1);
if(iRetorno == 1 || iRetorno == -12 || iRetorno == -50 || iRetorno == -51 || iRetorno == -52){
darUtil.swapPointer(pszBufferEntrada, pValor1);
}
return iRetorno;
}
public static int eAbrirSerial(String pszPorta, String pszVelocidade) {
int iRetorno = dfw.eAbrirSerial_Daruma(pszPorta, pszVelocidade);
return iRetorno;
}
public static int eAguardarRecepcao() {
int iRetorno = dfw.eAguardarRecepcao_Daruma();
return iRetorno;
}
public static int eAuditar(String cAuditoria, int iFlag) {
int iRetorno = dfw.eAuditar_Daruma(cAuditoria, iFlag);
return iRetorno;
}
public static int eCancelaComunicacao() {
int iRetorno = dfw.eCancelaComunicacao_Daruma();
return iRetorno;
}
public static int eDefinirModoRegistro(int iTipo) {
int iRetorno = dfw.eDefinirModoRegistro_Daruma(iTipo);
return iRetorno;
}
public static int eDefinirProduto(String pszProduto) {
int iRetorno = dfw.eDefinirProduto_Daruma(pszProduto);
return iRetorno;
}
public static int eEncurtarUrl(String pszUrl, char[] pszShortUrlRet) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.eEncurtarUrl_Daruma(pszUrl, pValor1);
if(iRetorno == 1){
darUtil.swapPointer(pszShortUrlRet, pValor1);
}
return iRetorno;
}
public static int eFecharSerial() {
int iRetorno = dfw.eFecharSerial_Daruma();
return iRetorno;
}
public static int eRegistry_Carregar() {
int iRetorno = dfw.eRegistry_Carregar_Daruma();
return iRetorno;
}
public static int eRegistry_Gravar() {
int iRetorno = dfw.eRegistry_Gravar_Daruma();
return iRetorno;
}
public static int eRetornarPathDLL(char[] pszPathDLL) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.eRetornarPathDLL_Daruma(pValor1);
if(iRetorno == 1){
darUtil.swapPointer(pszPathDLL, pValor1);
}
return iRetorno;
}
public static int eVerificarVersaoDLL(char[] pszRet) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.eVerificarVersaoDLL_Daruma(pValor1);
if(iRetorno == 1 || iRetorno == -12 || iRetorno == -50 || iRetorno == -51 || iRetorno == -52){
darUtil.swapPointer(pszRet, pValor1);
}
return iRetorno;
}
public static int eXML_AlterarValor(String szTag, String szValor) {
int iRetorno = dfw.eXML_AlterarValor_Daruma(szTag, szValor);
return iRetorno;
}
public static int eXML_CarregarArquivo() {
int iRetorno = dfw.eXML_CarregarArquivo_Daruma();
return iRetorno;
}
public static int eXML_CriarArquivo() {
int iRetorno = dfw.eXML_CriarArquivo_Daruma();
return iRetorno;
}
public static int eXML_RetornarValor(String szTag, char[] szValorXML) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.eXML_RetornarValor_Daruma(szTag, pValor1);
if(iRetorno == 1 || iRetorno == -12 || iRetorno == -50 || iRetorno == -51 || iRetorno == -52){
darUtil.swapPointer(szValorXML, pValor1);
}
return iRetorno;
}
public static int rReceberDados(char[] pszBufferEntrada) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.rReceberDados_Daruma(pValor1);
if(iRetorno == 1 || iRetorno == -12 || iRetorno == -50 || iRetorno == -51 || iRetorno == -52){
darUtil.swapPointer(pszBufferEntrada, pValor1);
}
return iRetorno;
}
public static int regAlteraValorChave(String pszProduto, String pszChave, String pszValor) {
int iRetorno = dfw.regAlteraValorChave_DarumaFramework(pszProduto, pszChave, pszValor);
return iRetorno;
}
public static int regAlterarValor(String pszPathChave, String pszValor) {
int iRetorno = dfw.regAlterarValor_Daruma(pszPathChave, pszValor);
return iRetorno;
}
public static int regGeral_GERAL(String pszChave, String pszValor) {
int iRetorno = dfw.regGeral_GERAL_Daruma(pszChave, pszValor);
return iRetorno;
}
public static int regGeral_START(String pszChave, String pszValor) {
int iRetorno = dfw.regGeral_START_Daruma(pszChave, pszValor);
return iRetorno;
}
public static int regLogin(String pszPDV) {
int iRetorno = dfw.regLogin_Daruma(pszPDV);
return iRetorno;
}
public static int regRetornaValorChave(String pszProduto, String pszChave, char[] pszValor) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.regRetornaValorChave_DarumaFramework(pszProduto, pszChave, pValor1);
if(iRetorno == 1){
darUtil.swapPointer(pszValor, pValor1);
}
return iRetorno;
}
public static int tCarregarChaveRSA_Arquivo(String szCaminhoArquivo) {
int iRetorno = dfw.tCarregarChaveRSA_Arquivo_Daruma(szCaminhoArquivo);
return iRetorno;
}
public static int tCarregarChaveRSA(String n, String e, String d, String p, String q, String dp, String dq, String qp) {
int iRetorno = dfw.tCarregarChaveRSA_Daruma(n, e, d, p, q, dp, dq, qp);
return iRetorno;
}
public static int tEnviarDados(String pszBytes, int iTamBytes) {
int iRetorno = dfw.tEnviarDados_Daruma(pszBytes, iTamBytes);
return iRetorno;
}
public static int eVersoesBibliotecas(char[] pszVersoes) {
Pointer pValor1 = darUtil.getMemory();
int iRetorno = dfw.eVersoesBibliotecas_Daruma(pValor1);
if (iRetorno == 1)
darUtil.swapPointer(pszVersoes, pValor1);
return iRetorno;
}
// ===========================================================================
// =========================== FUNCOES LEGADAS =============================
// ===========================================================================
//@java.lang.Deprecated
public static int eVerificarVersaoDLL_Daruma(char[] pszValorRet){
return eVerificarVersaoDLL(pszValorRet);
}
//@java.lang.Deprecated
public static int iSelecionarImpressao_DS2000(String szDestino) {
return dfw.iSelecionarImpressao_DS2000_DarumaFramework(szDestino);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy