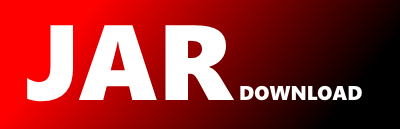
org.example.service.querydsl.QueryDslContactService Maven / Gradle / Ivy
The newest version!
package org.example.service.querydsl;
import org.example.jpadomain.Company;
import org.example.jpadomain.Contact;
import org.example.jpadomain.QContact;
import org.example.service.springdata.CompanyRepository;
import org.example.service.springdata.ContactRepository;
import org.springframework.data.domain.PageRequest;
import javax.ejb.Stateless;
import javax.inject.Inject;
import java.util.List;
/**
* Spring Data + QueryDSL based implementation. QueryDSL can also be used alone,
* but its sweet spot is as a compliment to the "repository helpers" like Spring
* Data and DeltaSpike Data. Here we just implement couple of queries with it
* and rely simple stuff to Spring Data. We also use built in QueryDSL
* integration in Spring Data so we only need to construct the predicate part.
* The Q prefixed classess, on which the QueryDSL magic is based on, are
* autogenerated based on entity classes, see pom.xml for a setup.
*/
@Stateless
public class QueryDslContactService {
@Inject
ContactRepository repository;
@Inject
CompanyRepository companyRepository;
public List findPageByCompanyAndName(Company company, String filter,
int firstrow, int maxrows) {
QContact c = QContact.contact;
return repository.findAll(c.company.eq(company).and(c.name.
containsIgnoreCase(filter)),
new PageRequest(firstrow / maxrows, maxrows)
).getContent();
}
public Long countByCompanyAndName(Company company, String filter) {
final QContact c = QContact.contact;
return repository.count(c.company.eq(company).and(
c.name.containsIgnoreCase(filter)));
}
public void save(Contact entry) {
repository.save(entry);
}
public void delete(Contact value) {
repository.delete(value);
}
public List findCompanies() {
return companyRepository.findAll();
}
public Contact refreshEntry(Contact entry) {
return repository.findOne(entry.getId());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy