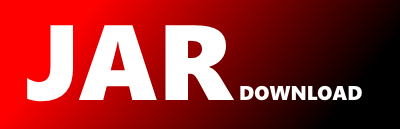
com.github.datalking.annotation.meta.AnnotationAttributes Maven / Gradle / Ivy
package com.github.datalking.annotation.meta;
import com.github.datalking.util.Assert;
import java.lang.annotation.Annotation;
import java.lang.reflect.Array;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* @author yaoo on 4/13/18
*/
public class AnnotationAttributes extends LinkedHashMap {
private static final String UNKNOWN = "unknown";
private final Class extends Annotation> annotationType;
// 用于断言验证
private final String displayName;
public AnnotationAttributes() {
this.annotationType = null;
this.displayName = UNKNOWN;
}
public AnnotationAttributes(Class extends Annotation> annotationType) {
Assert.notNull(annotationType, "'annotationType' must not be null");
this.annotationType = annotationType;
this.displayName = annotationType.getName();
}
public AnnotationAttributes(Map map) {
super(map);
this.annotationType = null;
this.displayName = UNKNOWN;
}
public Class extends Annotation> annotationType() {
return this.annotationType;
}
public String getString(String attributeName) {
return getRequiredAttribute(attributeName, String.class);
}
public String[] getStringArray(String attributeName) {
return getRequiredAttribute(attributeName, String[].class);
}
public boolean getBoolean(String attributeName) {
return getRequiredAttribute(attributeName, Boolean.class);
}
public N getNumber(String attributeName) {
return (N) getRequiredAttribute(attributeName, Number.class);
}
public > E getEnum(String attributeName) {
return (E) getRequiredAttribute(attributeName, Enum.class);
}
public Class extends T> getClass(String attributeName) {
return getRequiredAttribute(attributeName, Class.class);
}
public Class>[] getClassArray(String attributeName) {
return getRequiredAttribute(attributeName, Class[].class);
}
public AnnotationAttributes getAnnotation(String attributeName) {
return getRequiredAttribute(attributeName, AnnotationAttributes.class);
}
public A getAnnotation(String attributeName, Class annotationType) {
return getRequiredAttribute(attributeName, annotationType);
}
public AnnotationAttributes[] getAnnotationArray(String attributeName) {
return getRequiredAttribute(attributeName, AnnotationAttributes[].class);
}
public A[] getAnnotationArray(String attributeName, Class annotationType) {
Object array = Array.newInstance(annotationType, 0);
return (A[]) getRequiredAttribute(attributeName, array.getClass());
}
public Class extends Annotation> getAnnotationType() {
return annotationType;
}
/**
* 从输入的map中创建AnnotationAttributes
*
* @param map 输入键值对
* @return AnnotationAttributes
*/
public static AnnotationAttributes fromMap(Map map) {
if (map == null) {
return null;
}
if (map instanceof AnnotationAttributes) {
return (AnnotationAttributes) map;
}
return new AnnotationAttributes(map);
}
private T getAttribute(String attributeName, Class expectedType) {
Object value = get(attributeName);
return (T) value;
}
private T getRequiredAttribute(String attributeName, Class expectedType) {
Assert.notNull(attributeName, "'attributeName' must not be null or empty");
Object value = get(attributeName);
if (!expectedType.isInstance(value) && expectedType.isArray() && expectedType.getComponentType().isInstance(value)) {
Object array = Array.newInstance(expectedType.getComponentType(), 1);
Array.set(array, 0, value);
value = array;
}
return (T) value;
}
private static Class extends Annotation> getAnnotationType(String annotationType, ClassLoader classLoader) {
if (classLoader != null) {
try {
return (Class extends Annotation>) classLoader.loadClass(annotationType);
} catch (ClassNotFoundException ex) {
// Annotation Class not resolvable
}
}
return null;
}
@Override
public String toString() {
Iterator> entries = entrySet().iterator();
StringBuilder sb = new StringBuilder("{");
while (entries.hasNext()) {
Map.Entry entry = entries.next();
sb.append(entry.getKey());
sb.append('=');
sb.append(entry.getValue().toString());
sb.append(entries.hasNext() ? ", " : "");
}
sb.append("}");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy