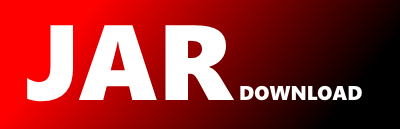
com.github.datalking.common.convert.converter.GenericConverter Maven / Gradle / Ivy
package com.github.datalking.common.convert.converter;
import com.github.datalking.common.convert.descriptor.TypeDescriptor;
import com.github.datalking.util.Assert;
import java.util.Set;
/**
* @author yaoo on 5/10/18
*/
public interface GenericConverter {
Set getConvertibleTypes();
Object convert(Object source, TypeDescriptor sourceType, TypeDescriptor targetType);
final class ConvertiblePair {
private final Class> sourceType;
private final Class> targetType;
public ConvertiblePair(Class> sourceType, Class> targetType) {
Assert.notNull(sourceType, "Source type must not be null");
Assert.notNull(targetType, "Target type must not be null");
this.sourceType = sourceType;
this.targetType = targetType;
}
public Class> getSourceType() {
return this.sourceType;
}
public Class> getTargetType() {
return this.targetType;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || obj.getClass() != ConvertiblePair.class) {
return false;
}
ConvertiblePair other = (ConvertiblePair) obj;
return this.sourceType.equals(other.sourceType) && this.targetType.equals(other.targetType);
}
@Override
public int hashCode() {
return this.sourceType.hashCode() * 31 + this.targetType.hashCode();
}
@Override
public String toString() {
return this.sourceType.getName() + " -> " + this.targetType.getName();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy