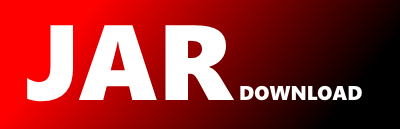
com.github.datalking.common.env.CompositePropertySource Maven / Gradle / Ivy
package com.github.datalking.common.env;
import com.github.datalking.util.StringUtils;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
/**
* 可保存多个PropertySource
*
* @author yaoo on 5/29/18
*/
public class CompositePropertySource extends EnumerablePropertySource
© 2015 - 2025 Weber Informatics LLC | Privacy Policy