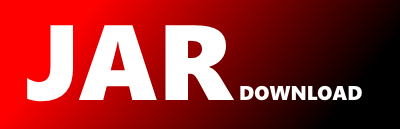
com.github.datalking.io.PropertiesLoaderSupport Maven / Gradle / Ivy
package com.github.datalking.io;
import com.github.datalking.common.DefaultPropertiesPersister;
import com.github.datalking.common.PropertiesPersister;
import com.github.datalking.util.CollectionUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.Properties;
/**
* @author yaoo on 5/28/18
*/
public abstract class PropertiesLoaderSupport {
protected final Logger logger = LoggerFactory.getLogger(getClass());
protected Properties[] localProperties;
protected boolean localOverride = false;
private Resource[] locations;
private boolean ignoreResourceNotFound = false;
private String fileEncoding;
private PropertiesPersister propertiesPersister = new DefaultPropertiesPersister();
public void setProperties(Properties properties) {
this.localProperties = new Properties[]{properties};
}
/**
* Set local properties, e.g. via the "props" tag in XML bean definitions,
* allowing for merging multiple properties sets into one.
*/
public void setPropertiesArray(Properties... propertiesArray) {
this.localProperties = propertiesArray;
}
/**
* Set a location of a properties file to be loaded.
* Can point to a classic properties file or to an XML file
* that follows JDK 1.5's properties XML format.
*/
public void setLocation(Resource location) {
this.locations = new Resource[]{location};
}
/**
* Set locations of properties files to be loaded.
*
Can point to classic properties files or to XML files
* that follow JDK 1.5's properties XML format.
*
Note: Properties defined in later files will override
* properties defined earlier files, in case of overlapping keys.
* Hence, make sure that the most specific files are the last
* ones in the given list of locations.
*/
public void setLocations(Resource... locations) {
this.locations = locations;
}
/**
* Set whether local properties override properties from files.
*
Default is "false": Properties from files override local defaults.
* Can be switched to "true" to let local properties override defaults
* from files.
*/
public void setLocalOverride(boolean localOverride) {
this.localOverride = localOverride;
}
/**
* Set if failure to find the property resource should be ignored.
*
"true" is appropriate if the properties file is completely optional.
* Default is "false".
*/
public void setIgnoreResourceNotFound(boolean ignoreResourceNotFound) {
this.ignoreResourceNotFound = ignoreResourceNotFound;
}
/**
* Set the encoding to use for parsing properties files.
*
Default is none, using the {@code java.util.Properties}
* default encoding.
*
Only applies to classic properties files, not to XML files.
*/
public void setFileEncoding(String encoding) {
this.fileEncoding = encoding;
}
/**
* Set the PropertiesPersister to use for parsing properties files.
* The default is DefaultPropertiesPersister.
*/
public void setPropertiesPersister(PropertiesPersister propertiesPersister) {
this.propertiesPersister =
(propertiesPersister != null ? propertiesPersister : new DefaultPropertiesPersister());
}
/**
* Return a merged Properties instance containing both the
* loaded properties and properties set on this FactoryBean.
*/
protected Properties mergeProperties() throws IOException {
Properties result = new Properties();
if (this.localOverride) {
// Load properties from file upfront, to let local properties override.
loadProperties(result);
}
if (this.localProperties != null) {
for (Properties localProp : this.localProperties) {
CollectionUtils.mergePropertiesIntoMap(localProp, result);
}
}
if (!this.localOverride) {
// Load properties from file afterwards, to let those properties override.
loadProperties(result);
}
return result;
}
/**
* Load properties into the given instance.
*
* @param props the Properties instance to load into
* @throws IOException in case of I/O errors
*/
protected void loadProperties(Properties props) throws IOException {
if (this.locations != null) {
for (Resource location : this.locations) {
if (logger.isInfoEnabled()) {
logger.info("Loading properties file from " + location);
}
PropertiesLoaderUtils.fillProperties(
props,
new EncodedResource(location, this.fileEncoding),
this.propertiesPersister);
}
}
}
}