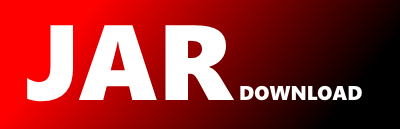
com.github.datalking.web.config.WebMvcConfigurationSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of play-mvc Show documentation
Show all versions of play-mvc Show documentation
simple mvc framework based on java servlet.
The newest version!
package com.github.datalking.web.config;
import com.github.datalking.annotation.Bean;
import com.github.datalking.common.MessageCodesResolver;
import com.github.datalking.common.convert.DefaultConversionService;
import com.github.datalking.common.convert.GenericConversionService;
import com.github.datalking.context.ApplicationContext;
import com.github.datalking.context.ApplicationContextAware;
import com.github.datalking.util.AntPathMatcher;
import com.github.datalking.util.ClassUtils;
import com.github.datalking.util.PathMatcher;
import com.github.datalking.util.web.UrlPathHelper;
import com.github.datalking.web.bind.ConfigurableWebBindingInitializer;
import com.github.datalking.web.http.MediaType;
import com.github.datalking.web.http.accept.ContentNegotiationManager;
import com.github.datalking.web.http.converter.HttpMessageConverter;
import com.github.datalking.web.http.converter.MappingJackson2HttpMessageConverter;
import com.github.datalking.web.http.converter.StringHttpMessageConverter;
import com.github.datalking.web.mvc.HttpRequestHandlerAdapter;
import com.github.datalking.web.mvc.SimpleControllerHandlerAdapter;
import com.github.datalking.web.mvc.method.ExceptionHandlerExceptionResolver;
import com.github.datalking.web.mvc.method.RequestMappingHandlerAdapter;
import com.github.datalking.web.mvc.method.RequestMappingHandlerMapping;
import com.github.datalking.web.mvc.method.ResponseStatusExceptionResolver;
import com.github.datalking.web.servlet.HandlerExceptionResolver;
import com.github.datalking.web.servlet.HandlerMapping;
import com.github.datalking.web.servlet.handler.AbstractHandlerMapping;
import com.github.datalking.web.servlet.handler.HandlerExceptionResolverComposite;
import com.github.datalking.web.support.DefaultHandlerExceptionResolver;
import com.github.datalking.web.support.HandlerMethodArgumentResolver;
import com.github.datalking.web.support.HandlerMethodReturnValueHandler;
import com.github.datalking.web.support.PathMatchConfigurer;
import com.github.datalking.web.context.ServletContextAware;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 在java代码中配置mvc的主要实现类
*
* @author yaoo on 4/25/18
*/
public class WebMvcConfigurationSupport implements ApplicationContextAware, ServletContextAware {
private static ClassLoader thisClassLoader = WebMvcConfigurationSupport.class.getClassLoader();
private static final boolean jaxb2Present = ClassUtils.isPresent("javax.xml.bind.Binder", thisClassLoader);
private static final boolean jackson2Present = ClassUtils.isPresent("com.fasterxml.jackson.databind.ObjectMapper", thisClassLoader)
&& ClassUtils.isPresent("com.fasterxml.jackson.core.JsonGenerator", thisClassLoader);
private ApplicationContext applicationContext;
private ServletContext servletContext;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy