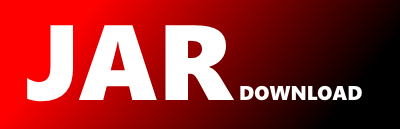
com.github.datalking.web.mvc.condition.ParamsRequestCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of play-mvc Show documentation
Show all versions of play-mvc Show documentation
simple mvc framework based on java servlet.
The newest version!
package com.github.datalking.web.mvc.condition;
import com.github.datalking.util.web.WebUtils;
import javax.servlet.http.HttpServletRequest;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Set;
/**
* @author yaoo on 4/28/18
*/
public class ParamsRequestCondition extends AbstractRequestCondition {
private final Set expressions;
public ParamsRequestCondition(String... params) {
this(parseExpressions(params));
}
private ParamsRequestCondition(Collection conditions) {
this.expressions = Collections.unmodifiableSet(new LinkedHashSet<>(conditions));
}
private static Collection parseExpressions(String... params) {
Set expressions = new LinkedHashSet<>();
if (params != null) {
for (String param : params) {
expressions.add(new ParamExpression(param));
}
}
return expressions;
}
public Set> getExpressions() {
return new LinkedHashSet<>(this.expressions);
}
@Override
protected Collection getContent() {
return this.expressions;
}
@Override
protected String getToStringInfix() {
return " && ";
}
public ParamsRequestCondition combine(ParamsRequestCondition other) {
Set set = new LinkedHashSet<>(this.expressions);
set.addAll(other.expressions);
return new ParamsRequestCondition(set);
}
public ParamsRequestCondition getMatchingCondition(HttpServletRequest request) {
for (ParamExpression expression : expressions) {
if (!expression.match(request)) {
return null;
}
}
return this;
}
public int compareTo(ParamsRequestCondition other, HttpServletRequest request) {
return (other.expressions.size() - this.expressions.size());
}
static class ParamExpression extends AbstractNameValueExpression {
ParamExpression(String expression) {
super(expression);
}
@Override
protected String parseValue(String valueExpression) {
return valueExpression;
}
@Override
protected boolean matchName(HttpServletRequest request) {
return WebUtils.hasSubmitParameter(request, name);
}
@Override
protected boolean matchValue(HttpServletRequest request) {
return value.equals(request.getParameter(name));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy