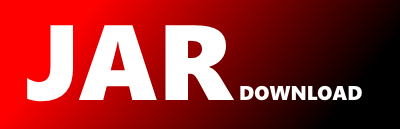
com.github.datalking.web.mvc.condition.RequestConditionHolder Maven / Gradle / Ivy
package com.github.datalking.web.mvc.condition;
import javax.servlet.http.HttpServletRequest;
import java.util.Collection;
import java.util.Collections;
/**
*/
public class RequestConditionHolder extends AbstractRequestCondition {
private final RequestCondition
© 2015 - 2025 Weber Informatics LLC | Privacy Policy