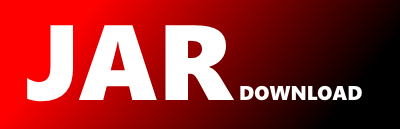
com.github.davidmoten.aws.lw.client.ResponseInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-lightweight-client-java Show documentation
Show all versions of aws-lightweight-client-java Show documentation
Lightweight client for all AWS services (but still with useful builders and XML parser)
The newest version!
package com.github.davidmoten.aws.lw.client;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
public final class ResponseInputStream extends InputStream {
private final Closeable closeable; // nullable
private final int statusCode;
private final Map> headers;
private final InputStream content;
public ResponseInputStream(HttpURLConnection connection, int statusCode,
Map> headers, InputStream content) {
this(() -> connection.disconnect(), statusCode, headers, content);
}
public ResponseInputStream(Closeable closeable, int statusCode,
Map> headers, InputStream content) {
this.closeable = closeable;
this.statusCode = statusCode;
this.headers = headers;
this.content = content;
}
@Override
public int read(byte[] b, int off, int len) throws IOException {
return content.read(b, off, len);
}
@Override
public int read() throws IOException {
return content.read();
}
@Override
public void close() throws IOException {
try {
content.close();
} finally {
closeable.close();
}
}
public int statusCode() {
return statusCode;
}
public Map> headers() {
return headers;
}
public Optional header(String name) {
for (String key : headers.keySet()) {
if (name.equalsIgnoreCase(key)) {
return Optional.of(headers.get(key).stream().collect(Collectors.joining(",")));
}
}
return Optional.empty();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy