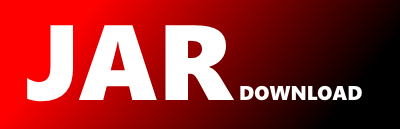
com.github.davidmoten.grumpy.projection.ProjectorBounds Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grumpy-projection Show documentation
Show all versions of grumpy-projection Show documentation
OGC tools including WMS server
package com.github.davidmoten.grumpy.projection;
import java.util.ArrayList;
import java.util.List;
public class ProjectorBounds {
private final double minX;
private final double minY;
private final double maxX;
private final double maxY;
private final String srs;
public String getSrs() {
return srs;
}
public ProjectorBounds(String srs, double minX, double minY, double maxX, double maxY) {
this.minX = minX;
this.minY = minY;
this.maxX = maxX;
this.maxY = maxY;
this.srs = srs;
}
public double getMinX() {
return minX;
}
public double getMinY() {
return minY;
}
public double getMaxX() {
return maxX;
}
public double getMaxY() {
return maxY;
}
public double getSizeX() {
return this.maxX - this.minX;
}
public double getSizeY() {
return maxY - minY;
}
public List splitHorizontally() {
List list = new ArrayList();
list.add(new ProjectorBounds(srs, minX, minY, minX + getSizeX() / 2, maxY));
list.add(new ProjectorBounds(srs, minX + getSizeX() / 2, minY, maxX, maxY));
return list;
}
public List splitVertically() {
List list = new ArrayList();
list.add(new ProjectorBounds(srs, minX, minY, maxX, minY + getSizeY() / 2));
list.add(new ProjectorBounds(srs, minX, minY + getSizeY() / 2, maxX, maxY));
return list;
}
public List quarter() {
List list = new ArrayList();
for (ProjectorBounds b : splitHorizontally())
list.addAll(b.splitVertically());
return list;
}
@Override
public String toString() {
return "ProjectorBounds [srs=" + srs + ", minX=" + minX + ", minY=" + minY + ", maxX="
+ maxX + ", maxY=" + maxY + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy