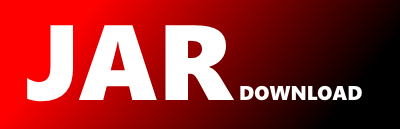
microsoft.vs.analytics.v1.model.entity.Iteration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-analytics Show documentation
Show all versions of odata-client-microsoft-analytics Show documentation
Java client as template for Microsoft Analytics organisation endpoints
package microsoft.vs.analytics.v1.model.entity;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.vs.analytics.v1.model.entity.collection.request.TeamCollectionRequest;
import microsoft.vs.analytics.v1.model.entity.request.ProjectRequest;
@JsonPropertyOrder({
"@odata.type",
"ProjectSK",
"IterationSK",
"IterationId",
"IterationName",
"Number",
"IterationPath",
"StartDate",
"EndDate",
"IterationLevel1",
"IterationLevel2",
"IterationLevel3",
"IterationLevel4",
"IterationLevel5",
"IterationLevel6",
"IterationLevel7",
"IterationLevel8",
"IterationLevel9",
"IterationLevel10",
"IterationLevel11",
"IterationLevel12",
"IterationLevel13",
"IterationLevel14",
"Depth",
"IsEnded"})
@JsonInclude(Include.NON_NULL)
public class Iteration implements ODataEntityType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JacksonInject
@JsonIgnore
protected ChangedFields changedFields;
@Override
public String odataTypeName() {
return "Microsoft.VisualStudio.Services.Analytics.Model.Iteration";
}
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("ProjectSK")
protected String projectSK;
@JsonProperty("IterationSK")
protected String iterationSK;
@JsonProperty("IterationId")
protected String iterationId;
@JsonProperty("IterationName")
protected String iterationName;
@JsonProperty("Number")
protected Integer number;
@JsonProperty("IterationPath")
protected String iterationPath;
@JsonProperty("StartDate")
protected OffsetDateTime startDate;
@JsonProperty("EndDate")
protected OffsetDateTime endDate;
@JsonProperty("IterationLevel1")
protected String iterationLevel1;
@JsonProperty("IterationLevel2")
protected String iterationLevel2;
@JsonProperty("IterationLevel3")
protected String iterationLevel3;
@JsonProperty("IterationLevel4")
protected String iterationLevel4;
@JsonProperty("IterationLevel5")
protected String iterationLevel5;
@JsonProperty("IterationLevel6")
protected String iterationLevel6;
@JsonProperty("IterationLevel7")
protected String iterationLevel7;
@JsonProperty("IterationLevel8")
protected String iterationLevel8;
@JsonProperty("IterationLevel9")
protected String iterationLevel9;
@JsonProperty("IterationLevel10")
protected String iterationLevel10;
@JsonProperty("IterationLevel11")
protected String iterationLevel11;
@JsonProperty("IterationLevel12")
protected String iterationLevel12;
@JsonProperty("IterationLevel13")
protected String iterationLevel13;
@JsonProperty("IterationLevel14")
protected String iterationLevel14;
@JsonProperty("Depth")
protected Integer depth;
@JsonProperty("IsEnded")
protected Boolean isEnded;
protected Iteration() {
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String projectSK;
private String iterationSK;
private String iterationId;
private String iterationName;
private Integer number;
private String iterationPath;
private OffsetDateTime startDate;
private OffsetDateTime endDate;
private String iterationLevel1;
private String iterationLevel2;
private String iterationLevel3;
private String iterationLevel4;
private String iterationLevel5;
private String iterationLevel6;
private String iterationLevel7;
private String iterationLevel8;
private String iterationLevel9;
private String iterationLevel10;
private String iterationLevel11;
private String iterationLevel12;
private String iterationLevel13;
private String iterationLevel14;
private Integer depth;
private Boolean isEnded;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder projectSK(String projectSK) {
this.projectSK = projectSK;
this.changedFields = changedFields.add("ProjectSK");
return this;
}
public Builder iterationSK(String iterationSK) {
this.iterationSK = iterationSK;
this.changedFields = changedFields.add("IterationSK");
return this;
}
public Builder iterationId(String iterationId) {
this.iterationId = iterationId;
this.changedFields = changedFields.add("IterationId");
return this;
}
public Builder iterationName(String iterationName) {
this.iterationName = iterationName;
this.changedFields = changedFields.add("IterationName");
return this;
}
public Builder number(Integer number) {
this.number = number;
this.changedFields = changedFields.add("Number");
return this;
}
public Builder iterationPath(String iterationPath) {
this.iterationPath = iterationPath;
this.changedFields = changedFields.add("IterationPath");
return this;
}
public Builder startDate(OffsetDateTime startDate) {
this.startDate = startDate;
this.changedFields = changedFields.add("StartDate");
return this;
}
public Builder endDate(OffsetDateTime endDate) {
this.endDate = endDate;
this.changedFields = changedFields.add("EndDate");
return this;
}
public Builder iterationLevel1(String iterationLevel1) {
this.iterationLevel1 = iterationLevel1;
this.changedFields = changedFields.add("IterationLevel1");
return this;
}
public Builder iterationLevel2(String iterationLevel2) {
this.iterationLevel2 = iterationLevel2;
this.changedFields = changedFields.add("IterationLevel2");
return this;
}
public Builder iterationLevel3(String iterationLevel3) {
this.iterationLevel3 = iterationLevel3;
this.changedFields = changedFields.add("IterationLevel3");
return this;
}
public Builder iterationLevel4(String iterationLevel4) {
this.iterationLevel4 = iterationLevel4;
this.changedFields = changedFields.add("IterationLevel4");
return this;
}
public Builder iterationLevel5(String iterationLevel5) {
this.iterationLevel5 = iterationLevel5;
this.changedFields = changedFields.add("IterationLevel5");
return this;
}
public Builder iterationLevel6(String iterationLevel6) {
this.iterationLevel6 = iterationLevel6;
this.changedFields = changedFields.add("IterationLevel6");
return this;
}
public Builder iterationLevel7(String iterationLevel7) {
this.iterationLevel7 = iterationLevel7;
this.changedFields = changedFields.add("IterationLevel7");
return this;
}
public Builder iterationLevel8(String iterationLevel8) {
this.iterationLevel8 = iterationLevel8;
this.changedFields = changedFields.add("IterationLevel8");
return this;
}
public Builder iterationLevel9(String iterationLevel9) {
this.iterationLevel9 = iterationLevel9;
this.changedFields = changedFields.add("IterationLevel9");
return this;
}
public Builder iterationLevel10(String iterationLevel10) {
this.iterationLevel10 = iterationLevel10;
this.changedFields = changedFields.add("IterationLevel10");
return this;
}
public Builder iterationLevel11(String iterationLevel11) {
this.iterationLevel11 = iterationLevel11;
this.changedFields = changedFields.add("IterationLevel11");
return this;
}
public Builder iterationLevel12(String iterationLevel12) {
this.iterationLevel12 = iterationLevel12;
this.changedFields = changedFields.add("IterationLevel12");
return this;
}
public Builder iterationLevel13(String iterationLevel13) {
this.iterationLevel13 = iterationLevel13;
this.changedFields = changedFields.add("IterationLevel13");
return this;
}
public Builder iterationLevel14(String iterationLevel14) {
this.iterationLevel14 = iterationLevel14;
this.changedFields = changedFields.add("IterationLevel14");
return this;
}
public Builder depth(Integer depth) {
this.depth = depth;
this.changedFields = changedFields.add("Depth");
return this;
}
public Builder isEnded(Boolean isEnded) {
this.isEnded = isEnded;
this.changedFields = changedFields.add("IsEnded");
return this;
}
public Iteration build() {
Iteration _x = new Iteration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.VisualStudio.Services.Analytics.Model.Iteration";
_x.projectSK = projectSK;
_x.iterationSK = iterationSK;
_x.iterationId = iterationId;
_x.iterationName = iterationName;
_x.number = number;
_x.iterationPath = iterationPath;
_x.startDate = startDate;
_x.endDate = endDate;
_x.iterationLevel1 = iterationLevel1;
_x.iterationLevel2 = iterationLevel2;
_x.iterationLevel3 = iterationLevel3;
_x.iterationLevel4 = iterationLevel4;
_x.iterationLevel5 = iterationLevel5;
_x.iterationLevel6 = iterationLevel6;
_x.iterationLevel7 = iterationLevel7;
_x.iterationLevel8 = iterationLevel8;
_x.iterationLevel9 = iterationLevel9;
_x.iterationLevel10 = iterationLevel10;
_x.iterationLevel11 = iterationLevel11;
_x.iterationLevel12 = iterationLevel12;
_x.iterationLevel13 = iterationLevel13;
_x.iterationLevel14 = iterationLevel14;
_x.depth = depth;
_x.isEnded = isEnded;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && iterationSK != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(iterationSK.toString()));
}
}
@Property(name="ProjectSK")
@JsonIgnore
public Optional getProjectSK() {
return Optional.ofNullable(projectSK);
}
public Iteration withProjectSK(String projectSK) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("ProjectSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.projectSK = projectSK;
return _x;
}
@Property(name="IterationSK")
@JsonIgnore
public Optional getIterationSK() {
return Optional.ofNullable(iterationSK);
}
public Iteration withIterationSK(String iterationSK) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationSK = iterationSK;
return _x;
}
@Property(name="IterationId")
@JsonIgnore
public Optional getIterationId() {
return Optional.ofNullable(iterationId);
}
public Iteration withIterationId(String iterationId) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationId = iterationId;
return _x;
}
@Property(name="IterationName")
@JsonIgnore
public Optional getIterationName() {
return Optional.ofNullable(iterationName);
}
public Iteration withIterationName(String iterationName) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationName");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationName = iterationName;
return _x;
}
@Property(name="Number")
@JsonIgnore
public Optional getNumber() {
return Optional.ofNullable(number);
}
public Iteration withNumber(Integer number) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("Number");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.number = number;
return _x;
}
@Property(name="IterationPath")
@JsonIgnore
public Optional getIterationPath() {
return Optional.ofNullable(iterationPath);
}
public Iteration withIterationPath(String iterationPath) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationPath");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationPath = iterationPath;
return _x;
}
@Property(name="StartDate")
@JsonIgnore
public Optional getStartDate() {
return Optional.ofNullable(startDate);
}
public Iteration withStartDate(OffsetDateTime startDate) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("StartDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.startDate = startDate;
return _x;
}
@Property(name="EndDate")
@JsonIgnore
public Optional getEndDate() {
return Optional.ofNullable(endDate);
}
public Iteration withEndDate(OffsetDateTime endDate) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("EndDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.endDate = endDate;
return _x;
}
@Property(name="IterationLevel1")
@JsonIgnore
public Optional getIterationLevel1() {
return Optional.ofNullable(iterationLevel1);
}
public Iteration withIterationLevel1(String iterationLevel1) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel1");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel1 = iterationLevel1;
return _x;
}
@Property(name="IterationLevel2")
@JsonIgnore
public Optional getIterationLevel2() {
return Optional.ofNullable(iterationLevel2);
}
public Iteration withIterationLevel2(String iterationLevel2) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel2");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel2 = iterationLevel2;
return _x;
}
@Property(name="IterationLevel3")
@JsonIgnore
public Optional getIterationLevel3() {
return Optional.ofNullable(iterationLevel3);
}
public Iteration withIterationLevel3(String iterationLevel3) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel3");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel3 = iterationLevel3;
return _x;
}
@Property(name="IterationLevel4")
@JsonIgnore
public Optional getIterationLevel4() {
return Optional.ofNullable(iterationLevel4);
}
public Iteration withIterationLevel4(String iterationLevel4) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel4");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel4 = iterationLevel4;
return _x;
}
@Property(name="IterationLevel5")
@JsonIgnore
public Optional getIterationLevel5() {
return Optional.ofNullable(iterationLevel5);
}
public Iteration withIterationLevel5(String iterationLevel5) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel5");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel5 = iterationLevel5;
return _x;
}
@Property(name="IterationLevel6")
@JsonIgnore
public Optional getIterationLevel6() {
return Optional.ofNullable(iterationLevel6);
}
public Iteration withIterationLevel6(String iterationLevel6) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel6");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel6 = iterationLevel6;
return _x;
}
@Property(name="IterationLevel7")
@JsonIgnore
public Optional getIterationLevel7() {
return Optional.ofNullable(iterationLevel7);
}
public Iteration withIterationLevel7(String iterationLevel7) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel7");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel7 = iterationLevel7;
return _x;
}
@Property(name="IterationLevel8")
@JsonIgnore
public Optional getIterationLevel8() {
return Optional.ofNullable(iterationLevel8);
}
public Iteration withIterationLevel8(String iterationLevel8) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel8");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel8 = iterationLevel8;
return _x;
}
@Property(name="IterationLevel9")
@JsonIgnore
public Optional getIterationLevel9() {
return Optional.ofNullable(iterationLevel9);
}
public Iteration withIterationLevel9(String iterationLevel9) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel9");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel9 = iterationLevel9;
return _x;
}
@Property(name="IterationLevel10")
@JsonIgnore
public Optional getIterationLevel10() {
return Optional.ofNullable(iterationLevel10);
}
public Iteration withIterationLevel10(String iterationLevel10) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel10");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel10 = iterationLevel10;
return _x;
}
@Property(name="IterationLevel11")
@JsonIgnore
public Optional getIterationLevel11() {
return Optional.ofNullable(iterationLevel11);
}
public Iteration withIterationLevel11(String iterationLevel11) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel11");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel11 = iterationLevel11;
return _x;
}
@Property(name="IterationLevel12")
@JsonIgnore
public Optional getIterationLevel12() {
return Optional.ofNullable(iterationLevel12);
}
public Iteration withIterationLevel12(String iterationLevel12) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel12");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel12 = iterationLevel12;
return _x;
}
@Property(name="IterationLevel13")
@JsonIgnore
public Optional getIterationLevel13() {
return Optional.ofNullable(iterationLevel13);
}
public Iteration withIterationLevel13(String iterationLevel13) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel13");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel13 = iterationLevel13;
return _x;
}
@Property(name="IterationLevel14")
@JsonIgnore
public Optional getIterationLevel14() {
return Optional.ofNullable(iterationLevel14);
}
public Iteration withIterationLevel14(String iterationLevel14) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IterationLevel14");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.iterationLevel14 = iterationLevel14;
return _x;
}
@Property(name="Depth")
@JsonIgnore
public Optional getDepth() {
return Optional.ofNullable(depth);
}
public Iteration withDepth(Integer depth) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("Depth");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.depth = depth;
return _x;
}
@Property(name="IsEnded")
@JsonIgnore
public Optional getIsEnded() {
return Optional.ofNullable(isEnded);
}
public Iteration withIsEnded(Boolean isEnded) {
Iteration _x = _copy();
_x.changedFields = changedFields.add("IsEnded");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.Iteration");
_x.isEnded = isEnded;
return _x;
}
public Iteration withUnmappedField(String name, String value) {
Iteration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="Project")
@JsonIgnore
public ProjectRequest getProject() {
return new ProjectRequest(contextPath.addSegment("Project"), RequestHelper.getValue(unmappedFields, "Project"));
}
@NavigationProperty(name="Teams")
@JsonIgnore
public TeamCollectionRequest getTeams() {
return new TeamCollectionRequest(
contextPath.addSegment("Teams"), RequestHelper.getValue(unmappedFields, "Teams"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Iteration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Iteration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Iteration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Iteration _x = _copy();
_x.changedFields = null;
return _x;
}
private Iteration _copy() {
Iteration _x = new Iteration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.projectSK = projectSK;
_x.iterationSK = iterationSK;
_x.iterationId = iterationId;
_x.iterationName = iterationName;
_x.number = number;
_x.iterationPath = iterationPath;
_x.startDate = startDate;
_x.endDate = endDate;
_x.iterationLevel1 = iterationLevel1;
_x.iterationLevel2 = iterationLevel2;
_x.iterationLevel3 = iterationLevel3;
_x.iterationLevel4 = iterationLevel4;
_x.iterationLevel5 = iterationLevel5;
_x.iterationLevel6 = iterationLevel6;
_x.iterationLevel7 = iterationLevel7;
_x.iterationLevel8 = iterationLevel8;
_x.iterationLevel9 = iterationLevel9;
_x.iterationLevel10 = iterationLevel10;
_x.iterationLevel11 = iterationLevel11;
_x.iterationLevel12 = iterationLevel12;
_x.iterationLevel13 = iterationLevel13;
_x.iterationLevel14 = iterationLevel14;
_x.depth = depth;
_x.isEnded = isEnded;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Iteration[");
b.append("ProjectSK=");
b.append(this.projectSK);
b.append(", ");
b.append("IterationSK=");
b.append(this.iterationSK);
b.append(", ");
b.append("IterationId=");
b.append(this.iterationId);
b.append(", ");
b.append("IterationName=");
b.append(this.iterationName);
b.append(", ");
b.append("Number=");
b.append(this.number);
b.append(", ");
b.append("IterationPath=");
b.append(this.iterationPath);
b.append(", ");
b.append("StartDate=");
b.append(this.startDate);
b.append(", ");
b.append("EndDate=");
b.append(this.endDate);
b.append(", ");
b.append("IterationLevel1=");
b.append(this.iterationLevel1);
b.append(", ");
b.append("IterationLevel2=");
b.append(this.iterationLevel2);
b.append(", ");
b.append("IterationLevel3=");
b.append(this.iterationLevel3);
b.append(", ");
b.append("IterationLevel4=");
b.append(this.iterationLevel4);
b.append(", ");
b.append("IterationLevel5=");
b.append(this.iterationLevel5);
b.append(", ");
b.append("IterationLevel6=");
b.append(this.iterationLevel6);
b.append(", ");
b.append("IterationLevel7=");
b.append(this.iterationLevel7);
b.append(", ");
b.append("IterationLevel8=");
b.append(this.iterationLevel8);
b.append(", ");
b.append("IterationLevel9=");
b.append(this.iterationLevel9);
b.append(", ");
b.append("IterationLevel10=");
b.append(this.iterationLevel10);
b.append(", ");
b.append("IterationLevel11=");
b.append(this.iterationLevel11);
b.append(", ");
b.append("IterationLevel12=");
b.append(this.iterationLevel12);
b.append(", ");
b.append("IterationLevel13=");
b.append(this.iterationLevel13);
b.append(", ");
b.append("IterationLevel14=");
b.append(this.iterationLevel14);
b.append(", ");
b.append("Depth=");
b.append(this.depth);
b.append(", ");
b.append("IsEnded=");
b.append(this.isEnded);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy