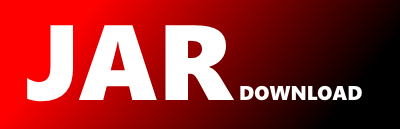
microsoft.vs.analytics.v1.model.entity.WorkItemSnapshot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-analytics Show documentation
Show all versions of odata-client-microsoft-analytics Show documentation
Java client as template for Microsoft Analytics organisation endpoints
package microsoft.vs.analytics.v1.model.entity;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Double;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.vs.analytics.v1.model.entity.collection.request.TagCollectionRequest;
import microsoft.vs.analytics.v1.model.entity.collection.request.TeamCollectionRequest;
import microsoft.vs.analytics.v1.model.entity.request.AreaRequest;
import microsoft.vs.analytics.v1.model.entity.request.CalendarDateRequest;
import microsoft.vs.analytics.v1.model.entity.request.IterationRequest;
import microsoft.vs.analytics.v1.model.entity.request.ProjectRequest;
import microsoft.vs.analytics.v1.model.entity.request.UserRequest;
import microsoft.vs.analytics.v1.model.enums.Period;
@JsonPropertyOrder({
"@odata.type",
"WorkItemId",
"DateSK",
"DateValue",
"IsLastDayOfPeriod",
"RevisedDate",
"RevisedDateSK",
"ProjectSK",
"WorkItemRevisionSK",
"AreaSK",
"IterationSK",
"AssignedToUserSK",
"ChangedByUserSK",
"CreatedByUserSK",
"ActivatedByUserSK",
"ClosedByUserSK",
"ResolvedByUserSK",
"ActivatedDateSK",
"ChangedDateSK",
"ClosedDateSK",
"CreatedDateSK",
"ResolvedDateSK",
"StateChangeDateSK",
"InProgressDateSK",
"CompletedDateSK",
"Revision",
"Watermark",
"Title",
"WorkItemType",
"ChangedDate",
"CreatedDate",
"State",
"Reason",
"FoundIn",
"IntegrationBuild",
"ActivatedDate",
"Activity",
"BacklogPriority",
"BusinessValue",
"ClosedDate",
"Issue",
"Priority",
"Rating",
"ResolvedDate",
"ResolvedReason",
"Risk",
"Severity",
"StackRank",
"TimeCriticality",
"ValueArea",
"CompletedWork",
"DueDate",
"Effort",
"FinishDate",
"OriginalEstimate",
"RemainingWork",
"StartDate",
"StoryPoints",
"TargetDate",
"Blocked",
"ParentWorkItemId",
"TagNames",
"StateCategory",
"InProgressDate",
"CompletedDate",
"LeadTimeDays",
"CycleTimeDays",
"AutomatedTestId",
"AutomatedTestName",
"AutomatedTestStorage",
"AutomatedTestType",
"AutomationStatus",
"StateChangeDate",
"Count",
"CommentCount",
"Microsoft_VSTS_CodeReview_AcceptedBySK",
"Microsoft_VSTS_CodeReview_AcceptedDate",
"Microsoft_VSTS_CodeReview_ClosedStatus",
"Microsoft_VSTS_CodeReview_ClosedStatusCode",
"Microsoft_VSTS_CodeReview_ClosingComment",
"Microsoft_VSTS_CodeReview_Context",
"Microsoft_VSTS_CodeReview_ContextCode",
"Microsoft_VSTS_CodeReview_ContextOwner",
"Microsoft_VSTS_CodeReview_ContextType",
"Microsoft_VSTS_Common_ReviewedBySK",
"Microsoft_VSTS_Common_StateCode",
"Microsoft_VSTS_Feedback_ApplicationType",
"Microsoft_VSTS_TCM_TestSuiteType",
"Microsoft_VSTS_TCM_TestSuiteTypeId"})
@JsonInclude(Include.NON_NULL)
public class WorkItemSnapshot implements ODataEntityType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JacksonInject
@JsonIgnore
protected ChangedFields changedFields;
@Override
public String odataTypeName() {
return "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot";
}
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("WorkItemId")
protected Integer workItemId;
@JsonProperty("DateSK")
protected Integer dateSK;
@JsonProperty("DateValue")
protected OffsetDateTime dateValue;
@JsonProperty("IsLastDayOfPeriod")
protected Period isLastDayOfPeriod;
@JsonProperty("RevisedDate")
protected OffsetDateTime revisedDate;
@JsonProperty("RevisedDateSK")
protected Integer revisedDateSK;
@JsonProperty("ProjectSK")
protected String projectSK;
@JsonProperty("WorkItemRevisionSK")
protected Integer workItemRevisionSK;
@JsonProperty("AreaSK")
protected String areaSK;
@JsonProperty("IterationSK")
protected String iterationSK;
@JsonProperty("AssignedToUserSK")
protected String assignedToUserSK;
@JsonProperty("ChangedByUserSK")
protected String changedByUserSK;
@JsonProperty("CreatedByUserSK")
protected String createdByUserSK;
@JsonProperty("ActivatedByUserSK")
protected String activatedByUserSK;
@JsonProperty("ClosedByUserSK")
protected String closedByUserSK;
@JsonProperty("ResolvedByUserSK")
protected String resolvedByUserSK;
@JsonProperty("ActivatedDateSK")
protected Integer activatedDateSK;
@JsonProperty("ChangedDateSK")
protected Integer changedDateSK;
@JsonProperty("ClosedDateSK")
protected Integer closedDateSK;
@JsonProperty("CreatedDateSK")
protected Integer createdDateSK;
@JsonProperty("ResolvedDateSK")
protected Integer resolvedDateSK;
@JsonProperty("StateChangeDateSK")
protected Integer stateChangeDateSK;
@JsonProperty("InProgressDateSK")
protected Integer inProgressDateSK;
@JsonProperty("CompletedDateSK")
protected Integer completedDateSK;
@JsonProperty("Revision")
protected Integer revision;
@JsonProperty("Watermark")
protected Integer watermark;
@JsonProperty("Title")
protected String title;
@JsonProperty("WorkItemType")
protected String workItemType;
@JsonProperty("ChangedDate")
protected OffsetDateTime changedDate;
@JsonProperty("CreatedDate")
protected OffsetDateTime createdDate;
@JsonProperty("State")
protected String state;
@JsonProperty("Reason")
protected String reason;
@JsonProperty("FoundIn")
protected String foundIn;
@JsonProperty("IntegrationBuild")
protected String integrationBuild;
@JsonProperty("ActivatedDate")
protected OffsetDateTime activatedDate;
@JsonProperty("Activity")
protected String activity;
@JsonProperty("BacklogPriority")
protected Double backlogPriority;
@JsonProperty("BusinessValue")
protected Integer businessValue;
@JsonProperty("ClosedDate")
protected OffsetDateTime closedDate;
@JsonProperty("Issue")
protected String issue;
@JsonProperty("Priority")
protected Integer priority;
@JsonProperty("Rating")
protected String rating;
@JsonProperty("ResolvedDate")
protected OffsetDateTime resolvedDate;
@JsonProperty("ResolvedReason")
protected String resolvedReason;
@JsonProperty("Risk")
protected String risk;
@JsonProperty("Severity")
protected String severity;
@JsonProperty("StackRank")
protected Double stackRank;
@JsonProperty("TimeCriticality")
protected Double timeCriticality;
@JsonProperty("ValueArea")
protected String valueArea;
@JsonProperty("CompletedWork")
protected Double completedWork;
@JsonProperty("DueDate")
protected OffsetDateTime dueDate;
@JsonProperty("Effort")
protected Double effort;
@JsonProperty("FinishDate")
protected OffsetDateTime finishDate;
@JsonProperty("OriginalEstimate")
protected Double originalEstimate;
@JsonProperty("RemainingWork")
protected Double remainingWork;
@JsonProperty("StartDate")
protected OffsetDateTime startDate;
@JsonProperty("StoryPoints")
protected Double storyPoints;
@JsonProperty("TargetDate")
protected OffsetDateTime targetDate;
@JsonProperty("Blocked")
protected String blocked;
@JsonProperty("ParentWorkItemId")
protected Integer parentWorkItemId;
@JsonProperty("TagNames")
protected String tagNames;
@JsonProperty("StateCategory")
protected String stateCategory;
@JsonProperty("InProgressDate")
protected OffsetDateTime inProgressDate;
@JsonProperty("CompletedDate")
protected OffsetDateTime completedDate;
@JsonProperty("LeadTimeDays")
protected Double leadTimeDays;
@JsonProperty("CycleTimeDays")
protected Double cycleTimeDays;
@JsonProperty("AutomatedTestId")
protected String automatedTestId;
@JsonProperty("AutomatedTestName")
protected String automatedTestName;
@JsonProperty("AutomatedTestStorage")
protected String automatedTestStorage;
@JsonProperty("AutomatedTestType")
protected String automatedTestType;
@JsonProperty("AutomationStatus")
protected String automationStatus;
@JsonProperty("StateChangeDate")
protected OffsetDateTime stateChangeDate;
@JsonProperty("Count")
protected Long count;
@JsonProperty("CommentCount")
protected Integer commentCount;
@JsonProperty("Microsoft_VSTS_CodeReview_AcceptedBySK")
protected String microsoft_VSTS_CodeReview_AcceptedBySK;
@JsonProperty("Microsoft_VSTS_CodeReview_AcceptedDate")
protected OffsetDateTime microsoft_VSTS_CodeReview_AcceptedDate;
@JsonProperty("Microsoft_VSTS_CodeReview_ClosedStatus")
protected String microsoft_VSTS_CodeReview_ClosedStatus;
@JsonProperty("Microsoft_VSTS_CodeReview_ClosedStatusCode")
protected Long microsoft_VSTS_CodeReview_ClosedStatusCode;
@JsonProperty("Microsoft_VSTS_CodeReview_ClosingComment")
protected String microsoft_VSTS_CodeReview_ClosingComment;
@JsonProperty("Microsoft_VSTS_CodeReview_Context")
protected String microsoft_VSTS_CodeReview_Context;
@JsonProperty("Microsoft_VSTS_CodeReview_ContextCode")
protected Long microsoft_VSTS_CodeReview_ContextCode;
@JsonProperty("Microsoft_VSTS_CodeReview_ContextOwner")
protected String microsoft_VSTS_CodeReview_ContextOwner;
@JsonProperty("Microsoft_VSTS_CodeReview_ContextType")
protected String microsoft_VSTS_CodeReview_ContextType;
@JsonProperty("Microsoft_VSTS_Common_ReviewedBySK")
protected String microsoft_VSTS_Common_ReviewedBySK;
@JsonProperty("Microsoft_VSTS_Common_StateCode")
protected Long microsoft_VSTS_Common_StateCode;
@JsonProperty("Microsoft_VSTS_Feedback_ApplicationType")
protected String microsoft_VSTS_Feedback_ApplicationType;
@JsonProperty("Microsoft_VSTS_TCM_TestSuiteType")
protected String microsoft_VSTS_TCM_TestSuiteType;
@JsonProperty("Microsoft_VSTS_TCM_TestSuiteTypeId")
protected Long microsoft_VSTS_TCM_TestSuiteTypeId;
protected WorkItemSnapshot() {
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Integer workItemId;
private Integer dateSK;
private OffsetDateTime dateValue;
private Period isLastDayOfPeriod;
private OffsetDateTime revisedDate;
private Integer revisedDateSK;
private String projectSK;
private Integer workItemRevisionSK;
private String areaSK;
private String iterationSK;
private String assignedToUserSK;
private String changedByUserSK;
private String createdByUserSK;
private String activatedByUserSK;
private String closedByUserSK;
private String resolvedByUserSK;
private Integer activatedDateSK;
private Integer changedDateSK;
private Integer closedDateSK;
private Integer createdDateSK;
private Integer resolvedDateSK;
private Integer stateChangeDateSK;
private Integer inProgressDateSK;
private Integer completedDateSK;
private Integer revision;
private Integer watermark;
private String title;
private String workItemType;
private OffsetDateTime changedDate;
private OffsetDateTime createdDate;
private String state;
private String reason;
private String foundIn;
private String integrationBuild;
private OffsetDateTime activatedDate;
private String activity;
private Double backlogPriority;
private Integer businessValue;
private OffsetDateTime closedDate;
private String issue;
private Integer priority;
private String rating;
private OffsetDateTime resolvedDate;
private String resolvedReason;
private String risk;
private String severity;
private Double stackRank;
private Double timeCriticality;
private String valueArea;
private Double completedWork;
private OffsetDateTime dueDate;
private Double effort;
private OffsetDateTime finishDate;
private Double originalEstimate;
private Double remainingWork;
private OffsetDateTime startDate;
private Double storyPoints;
private OffsetDateTime targetDate;
private String blocked;
private Integer parentWorkItemId;
private String tagNames;
private String stateCategory;
private OffsetDateTime inProgressDate;
private OffsetDateTime completedDate;
private Double leadTimeDays;
private Double cycleTimeDays;
private String automatedTestId;
private String automatedTestName;
private String automatedTestStorage;
private String automatedTestType;
private String automationStatus;
private OffsetDateTime stateChangeDate;
private Long count;
private Integer commentCount;
private String microsoft_VSTS_CodeReview_AcceptedBySK;
private OffsetDateTime microsoft_VSTS_CodeReview_AcceptedDate;
private String microsoft_VSTS_CodeReview_ClosedStatus;
private Long microsoft_VSTS_CodeReview_ClosedStatusCode;
private String microsoft_VSTS_CodeReview_ClosingComment;
private String microsoft_VSTS_CodeReview_Context;
private Long microsoft_VSTS_CodeReview_ContextCode;
private String microsoft_VSTS_CodeReview_ContextOwner;
private String microsoft_VSTS_CodeReview_ContextType;
private String microsoft_VSTS_Common_ReviewedBySK;
private Long microsoft_VSTS_Common_StateCode;
private String microsoft_VSTS_Feedback_ApplicationType;
private String microsoft_VSTS_TCM_TestSuiteType;
private Long microsoft_VSTS_TCM_TestSuiteTypeId;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder workItemId(Integer workItemId) {
this.workItemId = workItemId;
this.changedFields = changedFields.add("WorkItemId");
return this;
}
public Builder dateSK(Integer dateSK) {
this.dateSK = dateSK;
this.changedFields = changedFields.add("DateSK");
return this;
}
public Builder dateValue(OffsetDateTime dateValue) {
this.dateValue = dateValue;
this.changedFields = changedFields.add("DateValue");
return this;
}
public Builder isLastDayOfPeriod(Period isLastDayOfPeriod) {
this.isLastDayOfPeriod = isLastDayOfPeriod;
this.changedFields = changedFields.add("IsLastDayOfPeriod");
return this;
}
public Builder revisedDate(OffsetDateTime revisedDate) {
this.revisedDate = revisedDate;
this.changedFields = changedFields.add("RevisedDate");
return this;
}
public Builder revisedDateSK(Integer revisedDateSK) {
this.revisedDateSK = revisedDateSK;
this.changedFields = changedFields.add("RevisedDateSK");
return this;
}
public Builder projectSK(String projectSK) {
this.projectSK = projectSK;
this.changedFields = changedFields.add("ProjectSK");
return this;
}
public Builder workItemRevisionSK(Integer workItemRevisionSK) {
this.workItemRevisionSK = workItemRevisionSK;
this.changedFields = changedFields.add("WorkItemRevisionSK");
return this;
}
public Builder areaSK(String areaSK) {
this.areaSK = areaSK;
this.changedFields = changedFields.add("AreaSK");
return this;
}
public Builder iterationSK(String iterationSK) {
this.iterationSK = iterationSK;
this.changedFields = changedFields.add("IterationSK");
return this;
}
public Builder assignedToUserSK(String assignedToUserSK) {
this.assignedToUserSK = assignedToUserSK;
this.changedFields = changedFields.add("AssignedToUserSK");
return this;
}
public Builder changedByUserSK(String changedByUserSK) {
this.changedByUserSK = changedByUserSK;
this.changedFields = changedFields.add("ChangedByUserSK");
return this;
}
public Builder createdByUserSK(String createdByUserSK) {
this.createdByUserSK = createdByUserSK;
this.changedFields = changedFields.add("CreatedByUserSK");
return this;
}
public Builder activatedByUserSK(String activatedByUserSK) {
this.activatedByUserSK = activatedByUserSK;
this.changedFields = changedFields.add("ActivatedByUserSK");
return this;
}
public Builder closedByUserSK(String closedByUserSK) {
this.closedByUserSK = closedByUserSK;
this.changedFields = changedFields.add("ClosedByUserSK");
return this;
}
public Builder resolvedByUserSK(String resolvedByUserSK) {
this.resolvedByUserSK = resolvedByUserSK;
this.changedFields = changedFields.add("ResolvedByUserSK");
return this;
}
public Builder activatedDateSK(Integer activatedDateSK) {
this.activatedDateSK = activatedDateSK;
this.changedFields = changedFields.add("ActivatedDateSK");
return this;
}
public Builder changedDateSK(Integer changedDateSK) {
this.changedDateSK = changedDateSK;
this.changedFields = changedFields.add("ChangedDateSK");
return this;
}
public Builder closedDateSK(Integer closedDateSK) {
this.closedDateSK = closedDateSK;
this.changedFields = changedFields.add("ClosedDateSK");
return this;
}
public Builder createdDateSK(Integer createdDateSK) {
this.createdDateSK = createdDateSK;
this.changedFields = changedFields.add("CreatedDateSK");
return this;
}
public Builder resolvedDateSK(Integer resolvedDateSK) {
this.resolvedDateSK = resolvedDateSK;
this.changedFields = changedFields.add("ResolvedDateSK");
return this;
}
public Builder stateChangeDateSK(Integer stateChangeDateSK) {
this.stateChangeDateSK = stateChangeDateSK;
this.changedFields = changedFields.add("StateChangeDateSK");
return this;
}
public Builder inProgressDateSK(Integer inProgressDateSK) {
this.inProgressDateSK = inProgressDateSK;
this.changedFields = changedFields.add("InProgressDateSK");
return this;
}
public Builder completedDateSK(Integer completedDateSK) {
this.completedDateSK = completedDateSK;
this.changedFields = changedFields.add("CompletedDateSK");
return this;
}
public Builder revision(Integer revision) {
this.revision = revision;
this.changedFields = changedFields.add("Revision");
return this;
}
public Builder watermark(Integer watermark) {
this.watermark = watermark;
this.changedFields = changedFields.add("Watermark");
return this;
}
public Builder title(String title) {
this.title = title;
this.changedFields = changedFields.add("Title");
return this;
}
public Builder workItemType(String workItemType) {
this.workItemType = workItemType;
this.changedFields = changedFields.add("WorkItemType");
return this;
}
public Builder changedDate(OffsetDateTime changedDate) {
this.changedDate = changedDate;
this.changedFields = changedFields.add("ChangedDate");
return this;
}
public Builder createdDate(OffsetDateTime createdDate) {
this.createdDate = createdDate;
this.changedFields = changedFields.add("CreatedDate");
return this;
}
public Builder state(String state) {
this.state = state;
this.changedFields = changedFields.add("State");
return this;
}
public Builder reason(String reason) {
this.reason = reason;
this.changedFields = changedFields.add("Reason");
return this;
}
public Builder foundIn(String foundIn) {
this.foundIn = foundIn;
this.changedFields = changedFields.add("FoundIn");
return this;
}
public Builder integrationBuild(String integrationBuild) {
this.integrationBuild = integrationBuild;
this.changedFields = changedFields.add("IntegrationBuild");
return this;
}
public Builder activatedDate(OffsetDateTime activatedDate) {
this.activatedDate = activatedDate;
this.changedFields = changedFields.add("ActivatedDate");
return this;
}
public Builder activity(String activity) {
this.activity = activity;
this.changedFields = changedFields.add("Activity");
return this;
}
public Builder backlogPriority(Double backlogPriority) {
this.backlogPriority = backlogPriority;
this.changedFields = changedFields.add("BacklogPriority");
return this;
}
public Builder businessValue(Integer businessValue) {
this.businessValue = businessValue;
this.changedFields = changedFields.add("BusinessValue");
return this;
}
public Builder closedDate(OffsetDateTime closedDate) {
this.closedDate = closedDate;
this.changedFields = changedFields.add("ClosedDate");
return this;
}
public Builder issue(String issue) {
this.issue = issue;
this.changedFields = changedFields.add("Issue");
return this;
}
public Builder priority(Integer priority) {
this.priority = priority;
this.changedFields = changedFields.add("Priority");
return this;
}
public Builder rating(String rating) {
this.rating = rating;
this.changedFields = changedFields.add("Rating");
return this;
}
public Builder resolvedDate(OffsetDateTime resolvedDate) {
this.resolvedDate = resolvedDate;
this.changedFields = changedFields.add("ResolvedDate");
return this;
}
public Builder resolvedReason(String resolvedReason) {
this.resolvedReason = resolvedReason;
this.changedFields = changedFields.add("ResolvedReason");
return this;
}
public Builder risk(String risk) {
this.risk = risk;
this.changedFields = changedFields.add("Risk");
return this;
}
public Builder severity(String severity) {
this.severity = severity;
this.changedFields = changedFields.add("Severity");
return this;
}
public Builder stackRank(Double stackRank) {
this.stackRank = stackRank;
this.changedFields = changedFields.add("StackRank");
return this;
}
public Builder timeCriticality(Double timeCriticality) {
this.timeCriticality = timeCriticality;
this.changedFields = changedFields.add("TimeCriticality");
return this;
}
public Builder valueArea(String valueArea) {
this.valueArea = valueArea;
this.changedFields = changedFields.add("ValueArea");
return this;
}
public Builder completedWork(Double completedWork) {
this.completedWork = completedWork;
this.changedFields = changedFields.add("CompletedWork");
return this;
}
public Builder dueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
this.changedFields = changedFields.add("DueDate");
return this;
}
public Builder effort(Double effort) {
this.effort = effort;
this.changedFields = changedFields.add("Effort");
return this;
}
public Builder finishDate(OffsetDateTime finishDate) {
this.finishDate = finishDate;
this.changedFields = changedFields.add("FinishDate");
return this;
}
public Builder originalEstimate(Double originalEstimate) {
this.originalEstimate = originalEstimate;
this.changedFields = changedFields.add("OriginalEstimate");
return this;
}
public Builder remainingWork(Double remainingWork) {
this.remainingWork = remainingWork;
this.changedFields = changedFields.add("RemainingWork");
return this;
}
public Builder startDate(OffsetDateTime startDate) {
this.startDate = startDate;
this.changedFields = changedFields.add("StartDate");
return this;
}
public Builder storyPoints(Double storyPoints) {
this.storyPoints = storyPoints;
this.changedFields = changedFields.add("StoryPoints");
return this;
}
public Builder targetDate(OffsetDateTime targetDate) {
this.targetDate = targetDate;
this.changedFields = changedFields.add("TargetDate");
return this;
}
public Builder blocked(String blocked) {
this.blocked = blocked;
this.changedFields = changedFields.add("Blocked");
return this;
}
public Builder parentWorkItemId(Integer parentWorkItemId) {
this.parentWorkItemId = parentWorkItemId;
this.changedFields = changedFields.add("ParentWorkItemId");
return this;
}
public Builder tagNames(String tagNames) {
this.tagNames = tagNames;
this.changedFields = changedFields.add("TagNames");
return this;
}
public Builder stateCategory(String stateCategory) {
this.stateCategory = stateCategory;
this.changedFields = changedFields.add("StateCategory");
return this;
}
public Builder inProgressDate(OffsetDateTime inProgressDate) {
this.inProgressDate = inProgressDate;
this.changedFields = changedFields.add("InProgressDate");
return this;
}
public Builder completedDate(OffsetDateTime completedDate) {
this.completedDate = completedDate;
this.changedFields = changedFields.add("CompletedDate");
return this;
}
public Builder leadTimeDays(Double leadTimeDays) {
this.leadTimeDays = leadTimeDays;
this.changedFields = changedFields.add("LeadTimeDays");
return this;
}
public Builder cycleTimeDays(Double cycleTimeDays) {
this.cycleTimeDays = cycleTimeDays;
this.changedFields = changedFields.add("CycleTimeDays");
return this;
}
public Builder automatedTestId(String automatedTestId) {
this.automatedTestId = automatedTestId;
this.changedFields = changedFields.add("AutomatedTestId");
return this;
}
public Builder automatedTestName(String automatedTestName) {
this.automatedTestName = automatedTestName;
this.changedFields = changedFields.add("AutomatedTestName");
return this;
}
public Builder automatedTestStorage(String automatedTestStorage) {
this.automatedTestStorage = automatedTestStorage;
this.changedFields = changedFields.add("AutomatedTestStorage");
return this;
}
public Builder automatedTestType(String automatedTestType) {
this.automatedTestType = automatedTestType;
this.changedFields = changedFields.add("AutomatedTestType");
return this;
}
public Builder automationStatus(String automationStatus) {
this.automationStatus = automationStatus;
this.changedFields = changedFields.add("AutomationStatus");
return this;
}
public Builder stateChangeDate(OffsetDateTime stateChangeDate) {
this.stateChangeDate = stateChangeDate;
this.changedFields = changedFields.add("StateChangeDate");
return this;
}
public Builder count(Long count) {
this.count = count;
this.changedFields = changedFields.add("Count");
return this;
}
public Builder commentCount(Integer commentCount) {
this.commentCount = commentCount;
this.changedFields = changedFields.add("CommentCount");
return this;
}
public Builder microsoft_VSTS_CodeReview_AcceptedBySK(String microsoft_VSTS_CodeReview_AcceptedBySK) {
this.microsoft_VSTS_CodeReview_AcceptedBySK = microsoft_VSTS_CodeReview_AcceptedBySK;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_AcceptedBySK");
return this;
}
public Builder microsoft_VSTS_CodeReview_AcceptedDate(OffsetDateTime microsoft_VSTS_CodeReview_AcceptedDate) {
this.microsoft_VSTS_CodeReview_AcceptedDate = microsoft_VSTS_CodeReview_AcceptedDate;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_AcceptedDate");
return this;
}
public Builder microsoft_VSTS_CodeReview_ClosedStatus(String microsoft_VSTS_CodeReview_ClosedStatus) {
this.microsoft_VSTS_CodeReview_ClosedStatus = microsoft_VSTS_CodeReview_ClosedStatus;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ClosedStatus");
return this;
}
public Builder microsoft_VSTS_CodeReview_ClosedStatusCode(Long microsoft_VSTS_CodeReview_ClosedStatusCode) {
this.microsoft_VSTS_CodeReview_ClosedStatusCode = microsoft_VSTS_CodeReview_ClosedStatusCode;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ClosedStatusCode");
return this;
}
public Builder microsoft_VSTS_CodeReview_ClosingComment(String microsoft_VSTS_CodeReview_ClosingComment) {
this.microsoft_VSTS_CodeReview_ClosingComment = microsoft_VSTS_CodeReview_ClosingComment;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ClosingComment");
return this;
}
public Builder microsoft_VSTS_CodeReview_Context(String microsoft_VSTS_CodeReview_Context) {
this.microsoft_VSTS_CodeReview_Context = microsoft_VSTS_CodeReview_Context;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_Context");
return this;
}
public Builder microsoft_VSTS_CodeReview_ContextCode(Long microsoft_VSTS_CodeReview_ContextCode) {
this.microsoft_VSTS_CodeReview_ContextCode = microsoft_VSTS_CodeReview_ContextCode;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ContextCode");
return this;
}
public Builder microsoft_VSTS_CodeReview_ContextOwner(String microsoft_VSTS_CodeReview_ContextOwner) {
this.microsoft_VSTS_CodeReview_ContextOwner = microsoft_VSTS_CodeReview_ContextOwner;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ContextOwner");
return this;
}
public Builder microsoft_VSTS_CodeReview_ContextType(String microsoft_VSTS_CodeReview_ContextType) {
this.microsoft_VSTS_CodeReview_ContextType = microsoft_VSTS_CodeReview_ContextType;
this.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ContextType");
return this;
}
public Builder microsoft_VSTS_Common_ReviewedBySK(String microsoft_VSTS_Common_ReviewedBySK) {
this.microsoft_VSTS_Common_ReviewedBySK = microsoft_VSTS_Common_ReviewedBySK;
this.changedFields = changedFields.add("Microsoft_VSTS_Common_ReviewedBySK");
return this;
}
public Builder microsoft_VSTS_Common_StateCode(Long microsoft_VSTS_Common_StateCode) {
this.microsoft_VSTS_Common_StateCode = microsoft_VSTS_Common_StateCode;
this.changedFields = changedFields.add("Microsoft_VSTS_Common_StateCode");
return this;
}
public Builder microsoft_VSTS_Feedback_ApplicationType(String microsoft_VSTS_Feedback_ApplicationType) {
this.microsoft_VSTS_Feedback_ApplicationType = microsoft_VSTS_Feedback_ApplicationType;
this.changedFields = changedFields.add("Microsoft_VSTS_Feedback_ApplicationType");
return this;
}
public Builder microsoft_VSTS_TCM_TestSuiteType(String microsoft_VSTS_TCM_TestSuiteType) {
this.microsoft_VSTS_TCM_TestSuiteType = microsoft_VSTS_TCM_TestSuiteType;
this.changedFields = changedFields.add("Microsoft_VSTS_TCM_TestSuiteType");
return this;
}
public Builder microsoft_VSTS_TCM_TestSuiteTypeId(Long microsoft_VSTS_TCM_TestSuiteTypeId) {
this.microsoft_VSTS_TCM_TestSuiteTypeId = microsoft_VSTS_TCM_TestSuiteTypeId;
this.changedFields = changedFields.add("Microsoft_VSTS_TCM_TestSuiteTypeId");
return this;
}
public WorkItemSnapshot build() {
WorkItemSnapshot _x = new WorkItemSnapshot();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot";
_x.workItemId = workItemId;
_x.dateSK = dateSK;
_x.dateValue = dateValue;
_x.isLastDayOfPeriod = isLastDayOfPeriod;
_x.revisedDate = revisedDate;
_x.revisedDateSK = revisedDateSK;
_x.projectSK = projectSK;
_x.workItemRevisionSK = workItemRevisionSK;
_x.areaSK = areaSK;
_x.iterationSK = iterationSK;
_x.assignedToUserSK = assignedToUserSK;
_x.changedByUserSK = changedByUserSK;
_x.createdByUserSK = createdByUserSK;
_x.activatedByUserSK = activatedByUserSK;
_x.closedByUserSK = closedByUserSK;
_x.resolvedByUserSK = resolvedByUserSK;
_x.activatedDateSK = activatedDateSK;
_x.changedDateSK = changedDateSK;
_x.closedDateSK = closedDateSK;
_x.createdDateSK = createdDateSK;
_x.resolvedDateSK = resolvedDateSK;
_x.stateChangeDateSK = stateChangeDateSK;
_x.inProgressDateSK = inProgressDateSK;
_x.completedDateSK = completedDateSK;
_x.revision = revision;
_x.watermark = watermark;
_x.title = title;
_x.workItemType = workItemType;
_x.changedDate = changedDate;
_x.createdDate = createdDate;
_x.state = state;
_x.reason = reason;
_x.foundIn = foundIn;
_x.integrationBuild = integrationBuild;
_x.activatedDate = activatedDate;
_x.activity = activity;
_x.backlogPriority = backlogPriority;
_x.businessValue = businessValue;
_x.closedDate = closedDate;
_x.issue = issue;
_x.priority = priority;
_x.rating = rating;
_x.resolvedDate = resolvedDate;
_x.resolvedReason = resolvedReason;
_x.risk = risk;
_x.severity = severity;
_x.stackRank = stackRank;
_x.timeCriticality = timeCriticality;
_x.valueArea = valueArea;
_x.completedWork = completedWork;
_x.dueDate = dueDate;
_x.effort = effort;
_x.finishDate = finishDate;
_x.originalEstimate = originalEstimate;
_x.remainingWork = remainingWork;
_x.startDate = startDate;
_x.storyPoints = storyPoints;
_x.targetDate = targetDate;
_x.blocked = blocked;
_x.parentWorkItemId = parentWorkItemId;
_x.tagNames = tagNames;
_x.stateCategory = stateCategory;
_x.inProgressDate = inProgressDate;
_x.completedDate = completedDate;
_x.leadTimeDays = leadTimeDays;
_x.cycleTimeDays = cycleTimeDays;
_x.automatedTestId = automatedTestId;
_x.automatedTestName = automatedTestName;
_x.automatedTestStorage = automatedTestStorage;
_x.automatedTestType = automatedTestType;
_x.automationStatus = automationStatus;
_x.stateChangeDate = stateChangeDate;
_x.count = count;
_x.commentCount = commentCount;
_x.microsoft_VSTS_CodeReview_AcceptedBySK = microsoft_VSTS_CodeReview_AcceptedBySK;
_x.microsoft_VSTS_CodeReview_AcceptedDate = microsoft_VSTS_CodeReview_AcceptedDate;
_x.microsoft_VSTS_CodeReview_ClosedStatus = microsoft_VSTS_CodeReview_ClosedStatus;
_x.microsoft_VSTS_CodeReview_ClosedStatusCode = microsoft_VSTS_CodeReview_ClosedStatusCode;
_x.microsoft_VSTS_CodeReview_ClosingComment = microsoft_VSTS_CodeReview_ClosingComment;
_x.microsoft_VSTS_CodeReview_Context = microsoft_VSTS_CodeReview_Context;
_x.microsoft_VSTS_CodeReview_ContextCode = microsoft_VSTS_CodeReview_ContextCode;
_x.microsoft_VSTS_CodeReview_ContextOwner = microsoft_VSTS_CodeReview_ContextOwner;
_x.microsoft_VSTS_CodeReview_ContextType = microsoft_VSTS_CodeReview_ContextType;
_x.microsoft_VSTS_Common_ReviewedBySK = microsoft_VSTS_Common_ReviewedBySK;
_x.microsoft_VSTS_Common_StateCode = microsoft_VSTS_Common_StateCode;
_x.microsoft_VSTS_Feedback_ApplicationType = microsoft_VSTS_Feedback_ApplicationType;
_x.microsoft_VSTS_TCM_TestSuiteType = microsoft_VSTS_TCM_TestSuiteType;
_x.microsoft_VSTS_TCM_TestSuiteTypeId = microsoft_VSTS_TCM_TestSuiteTypeId;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && dateSK != null && workItemId != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue("DateSK", dateSK), new NameValue("WorkItemId", workItemId));
}
}
@Property(name="WorkItemId")
@JsonIgnore
public Optional getWorkItemId() {
return Optional.ofNullable(workItemId);
}
public WorkItemSnapshot withWorkItemId(Integer workItemId) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("WorkItemId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.workItemId = workItemId;
return _x;
}
@Property(name="DateSK")
@JsonIgnore
public Optional getDateSK() {
return Optional.ofNullable(dateSK);
}
public WorkItemSnapshot withDateSK(Integer dateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("DateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.dateSK = dateSK;
return _x;
}
@Property(name="DateValue")
@JsonIgnore
public Optional getDateValue() {
return Optional.ofNullable(dateValue);
}
public WorkItemSnapshot withDateValue(OffsetDateTime dateValue) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("DateValue");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.dateValue = dateValue;
return _x;
}
@Property(name="IsLastDayOfPeriod")
@JsonIgnore
public Optional getIsLastDayOfPeriod() {
return Optional.ofNullable(isLastDayOfPeriod);
}
public WorkItemSnapshot withIsLastDayOfPeriod(Period isLastDayOfPeriod) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("IsLastDayOfPeriod");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.isLastDayOfPeriod = isLastDayOfPeriod;
return _x;
}
@Property(name="RevisedDate")
@JsonIgnore
public Optional getRevisedDate() {
return Optional.ofNullable(revisedDate);
}
public WorkItemSnapshot withRevisedDate(OffsetDateTime revisedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("RevisedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.revisedDate = revisedDate;
return _x;
}
@Property(name="RevisedDateSK")
@JsonIgnore
public Optional getRevisedDateSK() {
return Optional.ofNullable(revisedDateSK);
}
public WorkItemSnapshot withRevisedDateSK(Integer revisedDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("RevisedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.revisedDateSK = revisedDateSK;
return _x;
}
@Property(name="ProjectSK")
@JsonIgnore
public Optional getProjectSK() {
return Optional.ofNullable(projectSK);
}
public WorkItemSnapshot withProjectSK(String projectSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ProjectSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.projectSK = projectSK;
return _x;
}
@Property(name="WorkItemRevisionSK")
@JsonIgnore
public Optional getWorkItemRevisionSK() {
return Optional.ofNullable(workItemRevisionSK);
}
public WorkItemSnapshot withWorkItemRevisionSK(Integer workItemRevisionSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("WorkItemRevisionSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.workItemRevisionSK = workItemRevisionSK;
return _x;
}
@Property(name="AreaSK")
@JsonIgnore
public Optional getAreaSK() {
return Optional.ofNullable(areaSK);
}
public WorkItemSnapshot withAreaSK(String areaSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AreaSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.areaSK = areaSK;
return _x;
}
@Property(name="IterationSK")
@JsonIgnore
public Optional getIterationSK() {
return Optional.ofNullable(iterationSK);
}
public WorkItemSnapshot withIterationSK(String iterationSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("IterationSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.iterationSK = iterationSK;
return _x;
}
@Property(name="AssignedToUserSK")
@JsonIgnore
public Optional getAssignedToUserSK() {
return Optional.ofNullable(assignedToUserSK);
}
public WorkItemSnapshot withAssignedToUserSK(String assignedToUserSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AssignedToUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.assignedToUserSK = assignedToUserSK;
return _x;
}
@Property(name="ChangedByUserSK")
@JsonIgnore
public Optional getChangedByUserSK() {
return Optional.ofNullable(changedByUserSK);
}
public WorkItemSnapshot withChangedByUserSK(String changedByUserSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ChangedByUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.changedByUserSK = changedByUserSK;
return _x;
}
@Property(name="CreatedByUserSK")
@JsonIgnore
public Optional getCreatedByUserSK() {
return Optional.ofNullable(createdByUserSK);
}
public WorkItemSnapshot withCreatedByUserSK(String createdByUserSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CreatedByUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.createdByUserSK = createdByUserSK;
return _x;
}
@Property(name="ActivatedByUserSK")
@JsonIgnore
public Optional getActivatedByUserSK() {
return Optional.ofNullable(activatedByUserSK);
}
public WorkItemSnapshot withActivatedByUserSK(String activatedByUserSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ActivatedByUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.activatedByUserSK = activatedByUserSK;
return _x;
}
@Property(name="ClosedByUserSK")
@JsonIgnore
public Optional getClosedByUserSK() {
return Optional.ofNullable(closedByUserSK);
}
public WorkItemSnapshot withClosedByUserSK(String closedByUserSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ClosedByUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.closedByUserSK = closedByUserSK;
return _x;
}
@Property(name="ResolvedByUserSK")
@JsonIgnore
public Optional getResolvedByUserSK() {
return Optional.ofNullable(resolvedByUserSK);
}
public WorkItemSnapshot withResolvedByUserSK(String resolvedByUserSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ResolvedByUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.resolvedByUserSK = resolvedByUserSK;
return _x;
}
@Property(name="ActivatedDateSK")
@JsonIgnore
public Optional getActivatedDateSK() {
return Optional.ofNullable(activatedDateSK);
}
public WorkItemSnapshot withActivatedDateSK(Integer activatedDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ActivatedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.activatedDateSK = activatedDateSK;
return _x;
}
@Property(name="ChangedDateSK")
@JsonIgnore
public Optional getChangedDateSK() {
return Optional.ofNullable(changedDateSK);
}
public WorkItemSnapshot withChangedDateSK(Integer changedDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ChangedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.changedDateSK = changedDateSK;
return _x;
}
@Property(name="ClosedDateSK")
@JsonIgnore
public Optional getClosedDateSK() {
return Optional.ofNullable(closedDateSK);
}
public WorkItemSnapshot withClosedDateSK(Integer closedDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ClosedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.closedDateSK = closedDateSK;
return _x;
}
@Property(name="CreatedDateSK")
@JsonIgnore
public Optional getCreatedDateSK() {
return Optional.ofNullable(createdDateSK);
}
public WorkItemSnapshot withCreatedDateSK(Integer createdDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CreatedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.createdDateSK = createdDateSK;
return _x;
}
@Property(name="ResolvedDateSK")
@JsonIgnore
public Optional getResolvedDateSK() {
return Optional.ofNullable(resolvedDateSK);
}
public WorkItemSnapshot withResolvedDateSK(Integer resolvedDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ResolvedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.resolvedDateSK = resolvedDateSK;
return _x;
}
@Property(name="StateChangeDateSK")
@JsonIgnore
public Optional getStateChangeDateSK() {
return Optional.ofNullable(stateChangeDateSK);
}
public WorkItemSnapshot withStateChangeDateSK(Integer stateChangeDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("StateChangeDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.stateChangeDateSK = stateChangeDateSK;
return _x;
}
@Property(name="InProgressDateSK")
@JsonIgnore
public Optional getInProgressDateSK() {
return Optional.ofNullable(inProgressDateSK);
}
public WorkItemSnapshot withInProgressDateSK(Integer inProgressDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("InProgressDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.inProgressDateSK = inProgressDateSK;
return _x;
}
@Property(name="CompletedDateSK")
@JsonIgnore
public Optional getCompletedDateSK() {
return Optional.ofNullable(completedDateSK);
}
public WorkItemSnapshot withCompletedDateSK(Integer completedDateSK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CompletedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.completedDateSK = completedDateSK;
return _x;
}
@Property(name="Revision")
@JsonIgnore
public Optional getRevision() {
return Optional.ofNullable(revision);
}
public WorkItemSnapshot withRevision(Integer revision) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Revision");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.revision = revision;
return _x;
}
@Property(name="Watermark")
@JsonIgnore
public Optional getWatermark() {
return Optional.ofNullable(watermark);
}
public WorkItemSnapshot withWatermark(Integer watermark) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Watermark");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.watermark = watermark;
return _x;
}
@Property(name="Title")
@JsonIgnore
public Optional getTitle() {
return Optional.ofNullable(title);
}
public WorkItemSnapshot withTitle(String title) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Title");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.title = title;
return _x;
}
@Property(name="WorkItemType")
@JsonIgnore
public Optional getWorkItemType() {
return Optional.ofNullable(workItemType);
}
public WorkItemSnapshot withWorkItemType(String workItemType) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("WorkItemType");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.workItemType = workItemType;
return _x;
}
@Property(name="ChangedDate")
@JsonIgnore
public Optional getChangedDate() {
return Optional.ofNullable(changedDate);
}
public WorkItemSnapshot withChangedDate(OffsetDateTime changedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ChangedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.changedDate = changedDate;
return _x;
}
@Property(name="CreatedDate")
@JsonIgnore
public Optional getCreatedDate() {
return Optional.ofNullable(createdDate);
}
public WorkItemSnapshot withCreatedDate(OffsetDateTime createdDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CreatedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.createdDate = createdDate;
return _x;
}
@Property(name="State")
@JsonIgnore
public Optional getState() {
return Optional.ofNullable(state);
}
public WorkItemSnapshot withState(String state) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("State");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.state = state;
return _x;
}
@Property(name="Reason")
@JsonIgnore
public Optional getReason() {
return Optional.ofNullable(reason);
}
public WorkItemSnapshot withReason(String reason) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Reason");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.reason = reason;
return _x;
}
@Property(name="FoundIn")
@JsonIgnore
public Optional getFoundIn() {
return Optional.ofNullable(foundIn);
}
public WorkItemSnapshot withFoundIn(String foundIn) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("FoundIn");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.foundIn = foundIn;
return _x;
}
@Property(name="IntegrationBuild")
@JsonIgnore
public Optional getIntegrationBuild() {
return Optional.ofNullable(integrationBuild);
}
public WorkItemSnapshot withIntegrationBuild(String integrationBuild) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("IntegrationBuild");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.integrationBuild = integrationBuild;
return _x;
}
@Property(name="ActivatedDate")
@JsonIgnore
public Optional getActivatedDate() {
return Optional.ofNullable(activatedDate);
}
public WorkItemSnapshot withActivatedDate(OffsetDateTime activatedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ActivatedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.activatedDate = activatedDate;
return _x;
}
@Property(name="Activity")
@JsonIgnore
public Optional getActivity() {
return Optional.ofNullable(activity);
}
public WorkItemSnapshot withActivity(String activity) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Activity");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.activity = activity;
return _x;
}
@Property(name="BacklogPriority")
@JsonIgnore
public Optional getBacklogPriority() {
return Optional.ofNullable(backlogPriority);
}
public WorkItemSnapshot withBacklogPriority(Double backlogPriority) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("BacklogPriority");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.backlogPriority = backlogPriority;
return _x;
}
@Property(name="BusinessValue")
@JsonIgnore
public Optional getBusinessValue() {
return Optional.ofNullable(businessValue);
}
public WorkItemSnapshot withBusinessValue(Integer businessValue) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("BusinessValue");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.businessValue = businessValue;
return _x;
}
@Property(name="ClosedDate")
@JsonIgnore
public Optional getClosedDate() {
return Optional.ofNullable(closedDate);
}
public WorkItemSnapshot withClosedDate(OffsetDateTime closedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ClosedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.closedDate = closedDate;
return _x;
}
@Property(name="Issue")
@JsonIgnore
public Optional getIssue() {
return Optional.ofNullable(issue);
}
public WorkItemSnapshot withIssue(String issue) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Issue");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.issue = issue;
return _x;
}
@Property(name="Priority")
@JsonIgnore
public Optional getPriority() {
return Optional.ofNullable(priority);
}
public WorkItemSnapshot withPriority(Integer priority) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Priority");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.priority = priority;
return _x;
}
@Property(name="Rating")
@JsonIgnore
public Optional getRating() {
return Optional.ofNullable(rating);
}
public WorkItemSnapshot withRating(String rating) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Rating");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.rating = rating;
return _x;
}
@Property(name="ResolvedDate")
@JsonIgnore
public Optional getResolvedDate() {
return Optional.ofNullable(resolvedDate);
}
public WorkItemSnapshot withResolvedDate(OffsetDateTime resolvedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ResolvedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.resolvedDate = resolvedDate;
return _x;
}
@Property(name="ResolvedReason")
@JsonIgnore
public Optional getResolvedReason() {
return Optional.ofNullable(resolvedReason);
}
public WorkItemSnapshot withResolvedReason(String resolvedReason) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ResolvedReason");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.resolvedReason = resolvedReason;
return _x;
}
@Property(name="Risk")
@JsonIgnore
public Optional getRisk() {
return Optional.ofNullable(risk);
}
public WorkItemSnapshot withRisk(String risk) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Risk");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.risk = risk;
return _x;
}
@Property(name="Severity")
@JsonIgnore
public Optional getSeverity() {
return Optional.ofNullable(severity);
}
public WorkItemSnapshot withSeverity(String severity) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Severity");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.severity = severity;
return _x;
}
@Property(name="StackRank")
@JsonIgnore
public Optional getStackRank() {
return Optional.ofNullable(stackRank);
}
public WorkItemSnapshot withStackRank(Double stackRank) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("StackRank");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.stackRank = stackRank;
return _x;
}
@Property(name="TimeCriticality")
@JsonIgnore
public Optional getTimeCriticality() {
return Optional.ofNullable(timeCriticality);
}
public WorkItemSnapshot withTimeCriticality(Double timeCriticality) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("TimeCriticality");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.timeCriticality = timeCriticality;
return _x;
}
@Property(name="ValueArea")
@JsonIgnore
public Optional getValueArea() {
return Optional.ofNullable(valueArea);
}
public WorkItemSnapshot withValueArea(String valueArea) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ValueArea");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.valueArea = valueArea;
return _x;
}
@Property(name="CompletedWork")
@JsonIgnore
public Optional getCompletedWork() {
return Optional.ofNullable(completedWork);
}
public WorkItemSnapshot withCompletedWork(Double completedWork) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CompletedWork");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.completedWork = completedWork;
return _x;
}
@Property(name="DueDate")
@JsonIgnore
public Optional getDueDate() {
return Optional.ofNullable(dueDate);
}
public WorkItemSnapshot withDueDate(OffsetDateTime dueDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("DueDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.dueDate = dueDate;
return _x;
}
@Property(name="Effort")
@JsonIgnore
public Optional getEffort() {
return Optional.ofNullable(effort);
}
public WorkItemSnapshot withEffort(Double effort) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Effort");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.effort = effort;
return _x;
}
@Property(name="FinishDate")
@JsonIgnore
public Optional getFinishDate() {
return Optional.ofNullable(finishDate);
}
public WorkItemSnapshot withFinishDate(OffsetDateTime finishDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("FinishDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.finishDate = finishDate;
return _x;
}
@Property(name="OriginalEstimate")
@JsonIgnore
public Optional getOriginalEstimate() {
return Optional.ofNullable(originalEstimate);
}
public WorkItemSnapshot withOriginalEstimate(Double originalEstimate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("OriginalEstimate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.originalEstimate = originalEstimate;
return _x;
}
@Property(name="RemainingWork")
@JsonIgnore
public Optional getRemainingWork() {
return Optional.ofNullable(remainingWork);
}
public WorkItemSnapshot withRemainingWork(Double remainingWork) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("RemainingWork");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.remainingWork = remainingWork;
return _x;
}
@Property(name="StartDate")
@JsonIgnore
public Optional getStartDate() {
return Optional.ofNullable(startDate);
}
public WorkItemSnapshot withStartDate(OffsetDateTime startDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("StartDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.startDate = startDate;
return _x;
}
@Property(name="StoryPoints")
@JsonIgnore
public Optional getStoryPoints() {
return Optional.ofNullable(storyPoints);
}
public WorkItemSnapshot withStoryPoints(Double storyPoints) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("StoryPoints");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.storyPoints = storyPoints;
return _x;
}
@Property(name="TargetDate")
@JsonIgnore
public Optional getTargetDate() {
return Optional.ofNullable(targetDate);
}
public WorkItemSnapshot withTargetDate(OffsetDateTime targetDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("TargetDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.targetDate = targetDate;
return _x;
}
@Property(name="Blocked")
@JsonIgnore
public Optional getBlocked() {
return Optional.ofNullable(blocked);
}
public WorkItemSnapshot withBlocked(String blocked) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Blocked");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.blocked = blocked;
return _x;
}
@Property(name="ParentWorkItemId")
@JsonIgnore
public Optional getParentWorkItemId() {
return Optional.ofNullable(parentWorkItemId);
}
public WorkItemSnapshot withParentWorkItemId(Integer parentWorkItemId) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("ParentWorkItemId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.parentWorkItemId = parentWorkItemId;
return _x;
}
@Property(name="TagNames")
@JsonIgnore
public Optional getTagNames() {
return Optional.ofNullable(tagNames);
}
public WorkItemSnapshot withTagNames(String tagNames) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("TagNames");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.tagNames = tagNames;
return _x;
}
@Property(name="StateCategory")
@JsonIgnore
public Optional getStateCategory() {
return Optional.ofNullable(stateCategory);
}
public WorkItemSnapshot withStateCategory(String stateCategory) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("StateCategory");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.stateCategory = stateCategory;
return _x;
}
@Property(name="InProgressDate")
@JsonIgnore
public Optional getInProgressDate() {
return Optional.ofNullable(inProgressDate);
}
public WorkItemSnapshot withInProgressDate(OffsetDateTime inProgressDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("InProgressDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.inProgressDate = inProgressDate;
return _x;
}
@Property(name="CompletedDate")
@JsonIgnore
public Optional getCompletedDate() {
return Optional.ofNullable(completedDate);
}
public WorkItemSnapshot withCompletedDate(OffsetDateTime completedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CompletedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.completedDate = completedDate;
return _x;
}
@Property(name="LeadTimeDays")
@JsonIgnore
public Optional getLeadTimeDays() {
return Optional.ofNullable(leadTimeDays);
}
public WorkItemSnapshot withLeadTimeDays(Double leadTimeDays) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("LeadTimeDays");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.leadTimeDays = leadTimeDays;
return _x;
}
@Property(name="CycleTimeDays")
@JsonIgnore
public Optional getCycleTimeDays() {
return Optional.ofNullable(cycleTimeDays);
}
public WorkItemSnapshot withCycleTimeDays(Double cycleTimeDays) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CycleTimeDays");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.cycleTimeDays = cycleTimeDays;
return _x;
}
@Property(name="AutomatedTestId")
@JsonIgnore
public Optional getAutomatedTestId() {
return Optional.ofNullable(automatedTestId);
}
public WorkItemSnapshot withAutomatedTestId(String automatedTestId) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AutomatedTestId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.automatedTestId = automatedTestId;
return _x;
}
@Property(name="AutomatedTestName")
@JsonIgnore
public Optional getAutomatedTestName() {
return Optional.ofNullable(automatedTestName);
}
public WorkItemSnapshot withAutomatedTestName(String automatedTestName) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AutomatedTestName");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.automatedTestName = automatedTestName;
return _x;
}
@Property(name="AutomatedTestStorage")
@JsonIgnore
public Optional getAutomatedTestStorage() {
return Optional.ofNullable(automatedTestStorage);
}
public WorkItemSnapshot withAutomatedTestStorage(String automatedTestStorage) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AutomatedTestStorage");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.automatedTestStorage = automatedTestStorage;
return _x;
}
@Property(name="AutomatedTestType")
@JsonIgnore
public Optional getAutomatedTestType() {
return Optional.ofNullable(automatedTestType);
}
public WorkItemSnapshot withAutomatedTestType(String automatedTestType) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AutomatedTestType");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.automatedTestType = automatedTestType;
return _x;
}
@Property(name="AutomationStatus")
@JsonIgnore
public Optional getAutomationStatus() {
return Optional.ofNullable(automationStatus);
}
public WorkItemSnapshot withAutomationStatus(String automationStatus) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("AutomationStatus");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.automationStatus = automationStatus;
return _x;
}
@Property(name="StateChangeDate")
@JsonIgnore
public Optional getStateChangeDate() {
return Optional.ofNullable(stateChangeDate);
}
public WorkItemSnapshot withStateChangeDate(OffsetDateTime stateChangeDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("StateChangeDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.stateChangeDate = stateChangeDate;
return _x;
}
@Property(name="Count")
@JsonIgnore
public Optional getCount() {
return Optional.ofNullable(count);
}
public WorkItemSnapshot withCount(Long count) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Count");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.count = count;
return _x;
}
@Property(name="CommentCount")
@JsonIgnore
public Optional getCommentCount() {
return Optional.ofNullable(commentCount);
}
public WorkItemSnapshot withCommentCount(Integer commentCount) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("CommentCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.commentCount = commentCount;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_AcceptedBySK")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_AcceptedBySK() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_AcceptedBySK);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_AcceptedBySK(String microsoft_VSTS_CodeReview_AcceptedBySK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_AcceptedBySK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_AcceptedBySK = microsoft_VSTS_CodeReview_AcceptedBySK;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_AcceptedDate")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_AcceptedDate() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_AcceptedDate);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_AcceptedDate(OffsetDateTime microsoft_VSTS_CodeReview_AcceptedDate) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_AcceptedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_AcceptedDate = microsoft_VSTS_CodeReview_AcceptedDate;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_ClosedStatus")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_ClosedStatus() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_ClosedStatus);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_ClosedStatus(String microsoft_VSTS_CodeReview_ClosedStatus) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ClosedStatus");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_ClosedStatus = microsoft_VSTS_CodeReview_ClosedStatus;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_ClosedStatusCode")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_ClosedStatusCode() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_ClosedStatusCode);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_ClosedStatusCode(Long microsoft_VSTS_CodeReview_ClosedStatusCode) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ClosedStatusCode");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_ClosedStatusCode = microsoft_VSTS_CodeReview_ClosedStatusCode;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_ClosingComment")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_ClosingComment() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_ClosingComment);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_ClosingComment(String microsoft_VSTS_CodeReview_ClosingComment) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ClosingComment");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_ClosingComment = microsoft_VSTS_CodeReview_ClosingComment;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_Context")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_Context() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_Context);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_Context(String microsoft_VSTS_CodeReview_Context) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_Context");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_Context = microsoft_VSTS_CodeReview_Context;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_ContextCode")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_ContextCode() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_ContextCode);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_ContextCode(Long microsoft_VSTS_CodeReview_ContextCode) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ContextCode");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_ContextCode = microsoft_VSTS_CodeReview_ContextCode;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_ContextOwner")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_ContextOwner() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_ContextOwner);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_ContextOwner(String microsoft_VSTS_CodeReview_ContextOwner) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ContextOwner");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_ContextOwner = microsoft_VSTS_CodeReview_ContextOwner;
return _x;
}
@Property(name="Microsoft_VSTS_CodeReview_ContextType")
@JsonIgnore
public Optional getMicrosoft_VSTS_CodeReview_ContextType() {
return Optional.ofNullable(microsoft_VSTS_CodeReview_ContextType);
}
public WorkItemSnapshot withMicrosoft_VSTS_CodeReview_ContextType(String microsoft_VSTS_CodeReview_ContextType) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_CodeReview_ContextType");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_CodeReview_ContextType = microsoft_VSTS_CodeReview_ContextType;
return _x;
}
@Property(name="Microsoft_VSTS_Common_ReviewedBySK")
@JsonIgnore
public Optional getMicrosoft_VSTS_Common_ReviewedBySK() {
return Optional.ofNullable(microsoft_VSTS_Common_ReviewedBySK);
}
public WorkItemSnapshot withMicrosoft_VSTS_Common_ReviewedBySK(String microsoft_VSTS_Common_ReviewedBySK) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_Common_ReviewedBySK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_Common_ReviewedBySK = microsoft_VSTS_Common_ReviewedBySK;
return _x;
}
@Property(name="Microsoft_VSTS_Common_StateCode")
@JsonIgnore
public Optional getMicrosoft_VSTS_Common_StateCode() {
return Optional.ofNullable(microsoft_VSTS_Common_StateCode);
}
public WorkItemSnapshot withMicrosoft_VSTS_Common_StateCode(Long microsoft_VSTS_Common_StateCode) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_Common_StateCode");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_Common_StateCode = microsoft_VSTS_Common_StateCode;
return _x;
}
@Property(name="Microsoft_VSTS_Feedback_ApplicationType")
@JsonIgnore
public Optional getMicrosoft_VSTS_Feedback_ApplicationType() {
return Optional.ofNullable(microsoft_VSTS_Feedback_ApplicationType);
}
public WorkItemSnapshot withMicrosoft_VSTS_Feedback_ApplicationType(String microsoft_VSTS_Feedback_ApplicationType) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_Feedback_ApplicationType");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_Feedback_ApplicationType = microsoft_VSTS_Feedback_ApplicationType;
return _x;
}
@Property(name="Microsoft_VSTS_TCM_TestSuiteType")
@JsonIgnore
public Optional getMicrosoft_VSTS_TCM_TestSuiteType() {
return Optional.ofNullable(microsoft_VSTS_TCM_TestSuiteType);
}
public WorkItemSnapshot withMicrosoft_VSTS_TCM_TestSuiteType(String microsoft_VSTS_TCM_TestSuiteType) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_TCM_TestSuiteType");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_TCM_TestSuiteType = microsoft_VSTS_TCM_TestSuiteType;
return _x;
}
@Property(name="Microsoft_VSTS_TCM_TestSuiteTypeId")
@JsonIgnore
public Optional getMicrosoft_VSTS_TCM_TestSuiteTypeId() {
return Optional.ofNullable(microsoft_VSTS_TCM_TestSuiteTypeId);
}
public WorkItemSnapshot withMicrosoft_VSTS_TCM_TestSuiteTypeId(Long microsoft_VSTS_TCM_TestSuiteTypeId) {
WorkItemSnapshot _x = _copy();
_x.changedFields = changedFields.add("Microsoft_VSTS_TCM_TestSuiteTypeId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.WorkItemSnapshot");
_x.microsoft_VSTS_TCM_TestSuiteTypeId = microsoft_VSTS_TCM_TestSuiteTypeId;
return _x;
}
public WorkItemSnapshot withUnmappedField(String name, String value) {
WorkItemSnapshot _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="Date")
@JsonIgnore
public CalendarDateRequest getDate() {
return new CalendarDateRequest(contextPath.addSegment("Date"), RequestHelper.getValue(unmappedFields, "Date"));
}
@NavigationProperty(name="RevisedOn")
@JsonIgnore
public CalendarDateRequest getRevisedOn() {
return new CalendarDateRequest(contextPath.addSegment("RevisedOn"), RequestHelper.getValue(unmappedFields, "RevisedOn"));
}
@NavigationProperty(name="Teams")
@JsonIgnore
public TeamCollectionRequest getTeams() {
return new TeamCollectionRequest(
contextPath.addSegment("Teams"), RequestHelper.getValue(unmappedFields, "Teams"));
}
@NavigationProperty(name="Project")
@JsonIgnore
public ProjectRequest getProject() {
return new ProjectRequest(contextPath.addSegment("Project"), RequestHelper.getValue(unmappedFields, "Project"));
}
@NavigationProperty(name="Area")
@JsonIgnore
public AreaRequest getArea() {
return new AreaRequest(contextPath.addSegment("Area"), RequestHelper.getValue(unmappedFields, "Area"));
}
@NavigationProperty(name="Iteration")
@JsonIgnore
public IterationRequest getIteration() {
return new IterationRequest(contextPath.addSegment("Iteration"), RequestHelper.getValue(unmappedFields, "Iteration"));
}
@NavigationProperty(name="AssignedTo")
@JsonIgnore
public UserRequest getAssignedTo() {
return new UserRequest(contextPath.addSegment("AssignedTo"), RequestHelper.getValue(unmappedFields, "AssignedTo"));
}
@NavigationProperty(name="ChangedBy")
@JsonIgnore
public UserRequest getChangedBy() {
return new UserRequest(contextPath.addSegment("ChangedBy"), RequestHelper.getValue(unmappedFields, "ChangedBy"));
}
@NavigationProperty(name="CreatedBy")
@JsonIgnore
public UserRequest getCreatedBy() {
return new UserRequest(contextPath.addSegment("CreatedBy"), RequestHelper.getValue(unmappedFields, "CreatedBy"));
}
@NavigationProperty(name="ActivatedBy")
@JsonIgnore
public UserRequest getActivatedBy() {
return new UserRequest(contextPath.addSegment("ActivatedBy"), RequestHelper.getValue(unmappedFields, "ActivatedBy"));
}
@NavigationProperty(name="ClosedBy")
@JsonIgnore
public UserRequest getClosedBy() {
return new UserRequest(contextPath.addSegment("ClosedBy"), RequestHelper.getValue(unmappedFields, "ClosedBy"));
}
@NavigationProperty(name="ResolvedBy")
@JsonIgnore
public UserRequest getResolvedBy() {
return new UserRequest(contextPath.addSegment("ResolvedBy"), RequestHelper.getValue(unmappedFields, "ResolvedBy"));
}
@NavigationProperty(name="Tags")
@JsonIgnore
public TagCollectionRequest getTags() {
return new TagCollectionRequest(
contextPath.addSegment("Tags"), RequestHelper.getValue(unmappedFields, "Tags"));
}
@NavigationProperty(name="ChangedOn")
@JsonIgnore
public CalendarDateRequest getChangedOn() {
return new CalendarDateRequest(contextPath.addSegment("ChangedOn"), RequestHelper.getValue(unmappedFields, "ChangedOn"));
}
@NavigationProperty(name="ClosedOn")
@JsonIgnore
public CalendarDateRequest getClosedOn() {
return new CalendarDateRequest(contextPath.addSegment("ClosedOn"), RequestHelper.getValue(unmappedFields, "ClosedOn"));
}
@NavigationProperty(name="CreatedOn")
@JsonIgnore
public CalendarDateRequest getCreatedOn() {
return new CalendarDateRequest(contextPath.addSegment("CreatedOn"), RequestHelper.getValue(unmappedFields, "CreatedOn"));
}
@NavigationProperty(name="ResolvedOn")
@JsonIgnore
public CalendarDateRequest getResolvedOn() {
return new CalendarDateRequest(contextPath.addSegment("ResolvedOn"), RequestHelper.getValue(unmappedFields, "ResolvedOn"));
}
@NavigationProperty(name="StateChangeOn")
@JsonIgnore
public CalendarDateRequest getStateChangeOn() {
return new CalendarDateRequest(contextPath.addSegment("StateChangeOn"), RequestHelper.getValue(unmappedFields, "StateChangeOn"));
}
@NavigationProperty(name="InProgressOn")
@JsonIgnore
public CalendarDateRequest getInProgressOn() {
return new CalendarDateRequest(contextPath.addSegment("InProgressOn"), RequestHelper.getValue(unmappedFields, "InProgressOn"));
}
@NavigationProperty(name="CompletedOn")
@JsonIgnore
public CalendarDateRequest getCompletedOn() {
return new CalendarDateRequest(contextPath.addSegment("CompletedOn"), RequestHelper.getValue(unmappedFields, "CompletedOn"));
}
@NavigationProperty(name="Microsoft_VSTS_CodeReview_AcceptedBy")
@JsonIgnore
public UserRequest getMicrosoft_VSTS_CodeReview_AcceptedBy() {
return new UserRequest(contextPath.addSegment("Microsoft_VSTS_CodeReview_AcceptedBy"), RequestHelper.getValue(unmappedFields, "Microsoft_VSTS_CodeReview_AcceptedBy"));
}
@NavigationProperty(name="Microsoft_VSTS_Common_ReviewedBy")
@JsonIgnore
public UserRequest getMicrosoft_VSTS_Common_ReviewedBy() {
return new UserRequest(contextPath.addSegment("Microsoft_VSTS_Common_ReviewedBy"), RequestHelper.getValue(unmappedFields, "Microsoft_VSTS_Common_ReviewedBy"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WorkItemSnapshot patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WorkItemSnapshot _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WorkItemSnapshot put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WorkItemSnapshot _x = _copy();
_x.changedFields = null;
return _x;
}
private WorkItemSnapshot _copy() {
WorkItemSnapshot _x = new WorkItemSnapshot();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.workItemId = workItemId;
_x.dateSK = dateSK;
_x.dateValue = dateValue;
_x.isLastDayOfPeriod = isLastDayOfPeriod;
_x.revisedDate = revisedDate;
_x.revisedDateSK = revisedDateSK;
_x.projectSK = projectSK;
_x.workItemRevisionSK = workItemRevisionSK;
_x.areaSK = areaSK;
_x.iterationSK = iterationSK;
_x.assignedToUserSK = assignedToUserSK;
_x.changedByUserSK = changedByUserSK;
_x.createdByUserSK = createdByUserSK;
_x.activatedByUserSK = activatedByUserSK;
_x.closedByUserSK = closedByUserSK;
_x.resolvedByUserSK = resolvedByUserSK;
_x.activatedDateSK = activatedDateSK;
_x.changedDateSK = changedDateSK;
_x.closedDateSK = closedDateSK;
_x.createdDateSK = createdDateSK;
_x.resolvedDateSK = resolvedDateSK;
_x.stateChangeDateSK = stateChangeDateSK;
_x.inProgressDateSK = inProgressDateSK;
_x.completedDateSK = completedDateSK;
_x.revision = revision;
_x.watermark = watermark;
_x.title = title;
_x.workItemType = workItemType;
_x.changedDate = changedDate;
_x.createdDate = createdDate;
_x.state = state;
_x.reason = reason;
_x.foundIn = foundIn;
_x.integrationBuild = integrationBuild;
_x.activatedDate = activatedDate;
_x.activity = activity;
_x.backlogPriority = backlogPriority;
_x.businessValue = businessValue;
_x.closedDate = closedDate;
_x.issue = issue;
_x.priority = priority;
_x.rating = rating;
_x.resolvedDate = resolvedDate;
_x.resolvedReason = resolvedReason;
_x.risk = risk;
_x.severity = severity;
_x.stackRank = stackRank;
_x.timeCriticality = timeCriticality;
_x.valueArea = valueArea;
_x.completedWork = completedWork;
_x.dueDate = dueDate;
_x.effort = effort;
_x.finishDate = finishDate;
_x.originalEstimate = originalEstimate;
_x.remainingWork = remainingWork;
_x.startDate = startDate;
_x.storyPoints = storyPoints;
_x.targetDate = targetDate;
_x.blocked = blocked;
_x.parentWorkItemId = parentWorkItemId;
_x.tagNames = tagNames;
_x.stateCategory = stateCategory;
_x.inProgressDate = inProgressDate;
_x.completedDate = completedDate;
_x.leadTimeDays = leadTimeDays;
_x.cycleTimeDays = cycleTimeDays;
_x.automatedTestId = automatedTestId;
_x.automatedTestName = automatedTestName;
_x.automatedTestStorage = automatedTestStorage;
_x.automatedTestType = automatedTestType;
_x.automationStatus = automationStatus;
_x.stateChangeDate = stateChangeDate;
_x.count = count;
_x.commentCount = commentCount;
_x.microsoft_VSTS_CodeReview_AcceptedBySK = microsoft_VSTS_CodeReview_AcceptedBySK;
_x.microsoft_VSTS_CodeReview_AcceptedDate = microsoft_VSTS_CodeReview_AcceptedDate;
_x.microsoft_VSTS_CodeReview_ClosedStatus = microsoft_VSTS_CodeReview_ClosedStatus;
_x.microsoft_VSTS_CodeReview_ClosedStatusCode = microsoft_VSTS_CodeReview_ClosedStatusCode;
_x.microsoft_VSTS_CodeReview_ClosingComment = microsoft_VSTS_CodeReview_ClosingComment;
_x.microsoft_VSTS_CodeReview_Context = microsoft_VSTS_CodeReview_Context;
_x.microsoft_VSTS_CodeReview_ContextCode = microsoft_VSTS_CodeReview_ContextCode;
_x.microsoft_VSTS_CodeReview_ContextOwner = microsoft_VSTS_CodeReview_ContextOwner;
_x.microsoft_VSTS_CodeReview_ContextType = microsoft_VSTS_CodeReview_ContextType;
_x.microsoft_VSTS_Common_ReviewedBySK = microsoft_VSTS_Common_ReviewedBySK;
_x.microsoft_VSTS_Common_StateCode = microsoft_VSTS_Common_StateCode;
_x.microsoft_VSTS_Feedback_ApplicationType = microsoft_VSTS_Feedback_ApplicationType;
_x.microsoft_VSTS_TCM_TestSuiteType = microsoft_VSTS_TCM_TestSuiteType;
_x.microsoft_VSTS_TCM_TestSuiteTypeId = microsoft_VSTS_TCM_TestSuiteTypeId;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WorkItemSnapshot[");
b.append("WorkItemId=");
b.append(this.workItemId);
b.append(", ");
b.append("DateSK=");
b.append(this.dateSK);
b.append(", ");
b.append("DateValue=");
b.append(this.dateValue);
b.append(", ");
b.append("IsLastDayOfPeriod=");
b.append(this.isLastDayOfPeriod);
b.append(", ");
b.append("RevisedDate=");
b.append(this.revisedDate);
b.append(", ");
b.append("RevisedDateSK=");
b.append(this.revisedDateSK);
b.append(", ");
b.append("ProjectSK=");
b.append(this.projectSK);
b.append(", ");
b.append("WorkItemRevisionSK=");
b.append(this.workItemRevisionSK);
b.append(", ");
b.append("AreaSK=");
b.append(this.areaSK);
b.append(", ");
b.append("IterationSK=");
b.append(this.iterationSK);
b.append(", ");
b.append("AssignedToUserSK=");
b.append(this.assignedToUserSK);
b.append(", ");
b.append("ChangedByUserSK=");
b.append(this.changedByUserSK);
b.append(", ");
b.append("CreatedByUserSK=");
b.append(this.createdByUserSK);
b.append(", ");
b.append("ActivatedByUserSK=");
b.append(this.activatedByUserSK);
b.append(", ");
b.append("ClosedByUserSK=");
b.append(this.closedByUserSK);
b.append(", ");
b.append("ResolvedByUserSK=");
b.append(this.resolvedByUserSK);
b.append(", ");
b.append("ActivatedDateSK=");
b.append(this.activatedDateSK);
b.append(", ");
b.append("ChangedDateSK=");
b.append(this.changedDateSK);
b.append(", ");
b.append("ClosedDateSK=");
b.append(this.closedDateSK);
b.append(", ");
b.append("CreatedDateSK=");
b.append(this.createdDateSK);
b.append(", ");
b.append("ResolvedDateSK=");
b.append(this.resolvedDateSK);
b.append(", ");
b.append("StateChangeDateSK=");
b.append(this.stateChangeDateSK);
b.append(", ");
b.append("InProgressDateSK=");
b.append(this.inProgressDateSK);
b.append(", ");
b.append("CompletedDateSK=");
b.append(this.completedDateSK);
b.append(", ");
b.append("Revision=");
b.append(this.revision);
b.append(", ");
b.append("Watermark=");
b.append(this.watermark);
b.append(", ");
b.append("Title=");
b.append(this.title);
b.append(", ");
b.append("WorkItemType=");
b.append(this.workItemType);
b.append(", ");
b.append("ChangedDate=");
b.append(this.changedDate);
b.append(", ");
b.append("CreatedDate=");
b.append(this.createdDate);
b.append(", ");
b.append("State=");
b.append(this.state);
b.append(", ");
b.append("Reason=");
b.append(this.reason);
b.append(", ");
b.append("FoundIn=");
b.append(this.foundIn);
b.append(", ");
b.append("IntegrationBuild=");
b.append(this.integrationBuild);
b.append(", ");
b.append("ActivatedDate=");
b.append(this.activatedDate);
b.append(", ");
b.append("Activity=");
b.append(this.activity);
b.append(", ");
b.append("BacklogPriority=");
b.append(this.backlogPriority);
b.append(", ");
b.append("BusinessValue=");
b.append(this.businessValue);
b.append(", ");
b.append("ClosedDate=");
b.append(this.closedDate);
b.append(", ");
b.append("Issue=");
b.append(this.issue);
b.append(", ");
b.append("Priority=");
b.append(this.priority);
b.append(", ");
b.append("Rating=");
b.append(this.rating);
b.append(", ");
b.append("ResolvedDate=");
b.append(this.resolvedDate);
b.append(", ");
b.append("ResolvedReason=");
b.append(this.resolvedReason);
b.append(", ");
b.append("Risk=");
b.append(this.risk);
b.append(", ");
b.append("Severity=");
b.append(this.severity);
b.append(", ");
b.append("StackRank=");
b.append(this.stackRank);
b.append(", ");
b.append("TimeCriticality=");
b.append(this.timeCriticality);
b.append(", ");
b.append("ValueArea=");
b.append(this.valueArea);
b.append(", ");
b.append("CompletedWork=");
b.append(this.completedWork);
b.append(", ");
b.append("DueDate=");
b.append(this.dueDate);
b.append(", ");
b.append("Effort=");
b.append(this.effort);
b.append(", ");
b.append("FinishDate=");
b.append(this.finishDate);
b.append(", ");
b.append("OriginalEstimate=");
b.append(this.originalEstimate);
b.append(", ");
b.append("RemainingWork=");
b.append(this.remainingWork);
b.append(", ");
b.append("StartDate=");
b.append(this.startDate);
b.append(", ");
b.append("StoryPoints=");
b.append(this.storyPoints);
b.append(", ");
b.append("TargetDate=");
b.append(this.targetDate);
b.append(", ");
b.append("Blocked=");
b.append(this.blocked);
b.append(", ");
b.append("ParentWorkItemId=");
b.append(this.parentWorkItemId);
b.append(", ");
b.append("TagNames=");
b.append(this.tagNames);
b.append(", ");
b.append("StateCategory=");
b.append(this.stateCategory);
b.append(", ");
b.append("InProgressDate=");
b.append(this.inProgressDate);
b.append(", ");
b.append("CompletedDate=");
b.append(this.completedDate);
b.append(", ");
b.append("LeadTimeDays=");
b.append(this.leadTimeDays);
b.append(", ");
b.append("CycleTimeDays=");
b.append(this.cycleTimeDays);
b.append(", ");
b.append("AutomatedTestId=");
b.append(this.automatedTestId);
b.append(", ");
b.append("AutomatedTestName=");
b.append(this.automatedTestName);
b.append(", ");
b.append("AutomatedTestStorage=");
b.append(this.automatedTestStorage);
b.append(", ");
b.append("AutomatedTestType=");
b.append(this.automatedTestType);
b.append(", ");
b.append("AutomationStatus=");
b.append(this.automationStatus);
b.append(", ");
b.append("StateChangeDate=");
b.append(this.stateChangeDate);
b.append(", ");
b.append("Count=");
b.append(this.count);
b.append(", ");
b.append("CommentCount=");
b.append(this.commentCount);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_AcceptedBySK=");
b.append(this.microsoft_VSTS_CodeReview_AcceptedBySK);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_AcceptedDate=");
b.append(this.microsoft_VSTS_CodeReview_AcceptedDate);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_ClosedStatus=");
b.append(this.microsoft_VSTS_CodeReview_ClosedStatus);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_ClosedStatusCode=");
b.append(this.microsoft_VSTS_CodeReview_ClosedStatusCode);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_ClosingComment=");
b.append(this.microsoft_VSTS_CodeReview_ClosingComment);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_Context=");
b.append(this.microsoft_VSTS_CodeReview_Context);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_ContextCode=");
b.append(this.microsoft_VSTS_CodeReview_ContextCode);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_ContextOwner=");
b.append(this.microsoft_VSTS_CodeReview_ContextOwner);
b.append(", ");
b.append("Microsoft_VSTS_CodeReview_ContextType=");
b.append(this.microsoft_VSTS_CodeReview_ContextType);
b.append(", ");
b.append("Microsoft_VSTS_Common_ReviewedBySK=");
b.append(this.microsoft_VSTS_Common_ReviewedBySK);
b.append(", ");
b.append("Microsoft_VSTS_Common_StateCode=");
b.append(this.microsoft_VSTS_Common_StateCode);
b.append(", ");
b.append("Microsoft_VSTS_Feedback_ApplicationType=");
b.append(this.microsoft_VSTS_Feedback_ApplicationType);
b.append(", ");
b.append("Microsoft_VSTS_TCM_TestSuiteType=");
b.append(this.microsoft_VSTS_TCM_TestSuiteType);
b.append(", ");
b.append("Microsoft_VSTS_TCM_TestSuiteTypeId=");
b.append(this.microsoft_VSTS_TCM_TestSuiteTypeId);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy