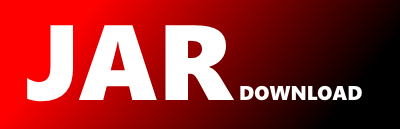
microsoft.vs.analytics.v3.model.entity.TestPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-analytics Show documentation
Show all versions of odata-client-microsoft-analytics Show documentation
Java client as template for Microsoft Analytics organisation endpoints
package microsoft.vs.analytics.v3.model.entity;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.vs.analytics.v3.model.entity.request.CalendarDateRequest;
import microsoft.vs.analytics.v3.model.entity.request.ProjectRequest;
import microsoft.vs.analytics.v3.model.entity.request.TestConfigurationRequest;
import microsoft.vs.analytics.v3.model.entity.request.TestSuiteRequest;
import microsoft.vs.analytics.v3.model.entity.request.UserRequest;
import microsoft.vs.analytics.v3.model.entity.request.WorkItemRequest;
import microsoft.vs.analytics.v3.model.enums.TestOutcome;
import microsoft.vs.analytics.v3.model.enums.TestResultState;
@JsonPropertyOrder({
"@odata.type",
"LastResultState",
"LastResultOutcome",
"ChangedDate",
"ChangedDateSK",
"TestPointSK",
"AnalyticsUpdatedDate",
"ProjectSK",
"TestSuiteSK",
"TestPlanId",
"TestSuiteId",
"TestPointId",
"TestConfigurationSK",
"TestConfigurationId",
"TestCaseId",
"TesterUserSK",
"AssignedToUserSK",
"Priority",
"AutomationStatus"})
@JsonInclude(Include.NON_NULL)
public class TestPoint implements ODataEntityType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JacksonInject
@JsonIgnore
protected ChangedFields changedFields;
@Override
public String odataTypeName() {
return "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint";
}
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("LastResultState")
protected TestResultState lastResultState;
@JsonProperty("LastResultOutcome")
protected TestOutcome lastResultOutcome;
@JsonProperty("ChangedDate")
protected OffsetDateTime changedDate;
@JsonProperty("ChangedDateSK")
protected Integer changedDateSK;
@JsonProperty("TestPointSK")
protected Integer testPointSK;
@JsonProperty("AnalyticsUpdatedDate")
protected OffsetDateTime analyticsUpdatedDate;
@JsonProperty("ProjectSK")
protected String projectSK;
@JsonProperty("TestSuiteSK")
protected Integer testSuiteSK;
@JsonProperty("TestPlanId")
protected Integer testPlanId;
@JsonProperty("TestSuiteId")
protected Integer testSuiteId;
@JsonProperty("TestPointId")
protected Integer testPointId;
@JsonProperty("TestConfigurationSK")
protected Integer testConfigurationSK;
@JsonProperty("TestConfigurationId")
protected Integer testConfigurationId;
@JsonProperty("TestCaseId")
protected Integer testCaseId;
@JsonProperty("TesterUserSK")
protected String testerUserSK;
@JsonProperty("AssignedToUserSK")
protected String assignedToUserSK;
@JsonProperty("Priority")
protected Integer priority;
@JsonProperty("AutomationStatus")
protected String automationStatus;
protected TestPoint() {
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private TestResultState lastResultState;
private TestOutcome lastResultOutcome;
private OffsetDateTime changedDate;
private Integer changedDateSK;
private Integer testPointSK;
private OffsetDateTime analyticsUpdatedDate;
private String projectSK;
private Integer testSuiteSK;
private Integer testPlanId;
private Integer testSuiteId;
private Integer testPointId;
private Integer testConfigurationSK;
private Integer testConfigurationId;
private Integer testCaseId;
private String testerUserSK;
private String assignedToUserSK;
private Integer priority;
private String automationStatus;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder lastResultState(TestResultState lastResultState) {
this.lastResultState = lastResultState;
this.changedFields = changedFields.add("LastResultState");
return this;
}
public Builder lastResultOutcome(TestOutcome lastResultOutcome) {
this.lastResultOutcome = lastResultOutcome;
this.changedFields = changedFields.add("LastResultOutcome");
return this;
}
public Builder changedDate(OffsetDateTime changedDate) {
this.changedDate = changedDate;
this.changedFields = changedFields.add("ChangedDate");
return this;
}
public Builder changedDateSK(Integer changedDateSK) {
this.changedDateSK = changedDateSK;
this.changedFields = changedFields.add("ChangedDateSK");
return this;
}
public Builder testPointSK(Integer testPointSK) {
this.testPointSK = testPointSK;
this.changedFields = changedFields.add("TestPointSK");
return this;
}
public Builder analyticsUpdatedDate(OffsetDateTime analyticsUpdatedDate) {
this.analyticsUpdatedDate = analyticsUpdatedDate;
this.changedFields = changedFields.add("AnalyticsUpdatedDate");
return this;
}
public Builder projectSK(String projectSK) {
this.projectSK = projectSK;
this.changedFields = changedFields.add("ProjectSK");
return this;
}
public Builder testSuiteSK(Integer testSuiteSK) {
this.testSuiteSK = testSuiteSK;
this.changedFields = changedFields.add("TestSuiteSK");
return this;
}
public Builder testPlanId(Integer testPlanId) {
this.testPlanId = testPlanId;
this.changedFields = changedFields.add("TestPlanId");
return this;
}
public Builder testSuiteId(Integer testSuiteId) {
this.testSuiteId = testSuiteId;
this.changedFields = changedFields.add("TestSuiteId");
return this;
}
public Builder testPointId(Integer testPointId) {
this.testPointId = testPointId;
this.changedFields = changedFields.add("TestPointId");
return this;
}
public Builder testConfigurationSK(Integer testConfigurationSK) {
this.testConfigurationSK = testConfigurationSK;
this.changedFields = changedFields.add("TestConfigurationSK");
return this;
}
public Builder testConfigurationId(Integer testConfigurationId) {
this.testConfigurationId = testConfigurationId;
this.changedFields = changedFields.add("TestConfigurationId");
return this;
}
public Builder testCaseId(Integer testCaseId) {
this.testCaseId = testCaseId;
this.changedFields = changedFields.add("TestCaseId");
return this;
}
public Builder testerUserSK(String testerUserSK) {
this.testerUserSK = testerUserSK;
this.changedFields = changedFields.add("TesterUserSK");
return this;
}
public Builder assignedToUserSK(String assignedToUserSK) {
this.assignedToUserSK = assignedToUserSK;
this.changedFields = changedFields.add("AssignedToUserSK");
return this;
}
public Builder priority(Integer priority) {
this.priority = priority;
this.changedFields = changedFields.add("Priority");
return this;
}
public Builder automationStatus(String automationStatus) {
this.automationStatus = automationStatus;
this.changedFields = changedFields.add("AutomationStatus");
return this;
}
public TestPoint build() {
TestPoint _x = new TestPoint();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint";
_x.lastResultState = lastResultState;
_x.lastResultOutcome = lastResultOutcome;
_x.changedDate = changedDate;
_x.changedDateSK = changedDateSK;
_x.testPointSK = testPointSK;
_x.analyticsUpdatedDate = analyticsUpdatedDate;
_x.projectSK = projectSK;
_x.testSuiteSK = testSuiteSK;
_x.testPlanId = testPlanId;
_x.testSuiteId = testSuiteId;
_x.testPointId = testPointId;
_x.testConfigurationSK = testConfigurationSK;
_x.testConfigurationId = testConfigurationId;
_x.testCaseId = testCaseId;
_x.testerUserSK = testerUserSK;
_x.assignedToUserSK = assignedToUserSK;
_x.priority = priority;
_x.automationStatus = automationStatus;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && testPointSK != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(testPointSK.toString()));
}
}
@Property(name="LastResultState")
@JsonIgnore
public Optional getLastResultState() {
return Optional.ofNullable(lastResultState);
}
public TestPoint withLastResultState(TestResultState lastResultState) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("LastResultState");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.lastResultState = lastResultState;
return _x;
}
@Property(name="LastResultOutcome")
@JsonIgnore
public Optional getLastResultOutcome() {
return Optional.ofNullable(lastResultOutcome);
}
public TestPoint withLastResultOutcome(TestOutcome lastResultOutcome) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("LastResultOutcome");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.lastResultOutcome = lastResultOutcome;
return _x;
}
@Property(name="ChangedDate")
@JsonIgnore
public Optional getChangedDate() {
return Optional.ofNullable(changedDate);
}
public TestPoint withChangedDate(OffsetDateTime changedDate) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("ChangedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.changedDate = changedDate;
return _x;
}
@Property(name="ChangedDateSK")
@JsonIgnore
public Optional getChangedDateSK() {
return Optional.ofNullable(changedDateSK);
}
public TestPoint withChangedDateSK(Integer changedDateSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("ChangedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.changedDateSK = changedDateSK;
return _x;
}
@Property(name="TestPointSK")
@JsonIgnore
public Optional getTestPointSK() {
return Optional.ofNullable(testPointSK);
}
public TestPoint withTestPointSK(Integer testPointSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestPointSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testPointSK = testPointSK;
return _x;
}
@Property(name="AnalyticsUpdatedDate")
@JsonIgnore
public Optional getAnalyticsUpdatedDate() {
return Optional.ofNullable(analyticsUpdatedDate);
}
public TestPoint withAnalyticsUpdatedDate(OffsetDateTime analyticsUpdatedDate) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("AnalyticsUpdatedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.analyticsUpdatedDate = analyticsUpdatedDate;
return _x;
}
@Property(name="ProjectSK")
@JsonIgnore
public Optional getProjectSK() {
return Optional.ofNullable(projectSK);
}
public TestPoint withProjectSK(String projectSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("ProjectSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.projectSK = projectSK;
return _x;
}
@Property(name="TestSuiteSK")
@JsonIgnore
public Optional getTestSuiteSK() {
return Optional.ofNullable(testSuiteSK);
}
public TestPoint withTestSuiteSK(Integer testSuiteSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestSuiteSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testSuiteSK = testSuiteSK;
return _x;
}
@Property(name="TestPlanId")
@JsonIgnore
public Optional getTestPlanId() {
return Optional.ofNullable(testPlanId);
}
public TestPoint withTestPlanId(Integer testPlanId) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestPlanId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testPlanId = testPlanId;
return _x;
}
@Property(name="TestSuiteId")
@JsonIgnore
public Optional getTestSuiteId() {
return Optional.ofNullable(testSuiteId);
}
public TestPoint withTestSuiteId(Integer testSuiteId) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestSuiteId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testSuiteId = testSuiteId;
return _x;
}
@Property(name="TestPointId")
@JsonIgnore
public Optional getTestPointId() {
return Optional.ofNullable(testPointId);
}
public TestPoint withTestPointId(Integer testPointId) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestPointId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testPointId = testPointId;
return _x;
}
@Property(name="TestConfigurationSK")
@JsonIgnore
public Optional getTestConfigurationSK() {
return Optional.ofNullable(testConfigurationSK);
}
public TestPoint withTestConfigurationSK(Integer testConfigurationSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestConfigurationSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testConfigurationSK = testConfigurationSK;
return _x;
}
@Property(name="TestConfigurationId")
@JsonIgnore
public Optional getTestConfigurationId() {
return Optional.ofNullable(testConfigurationId);
}
public TestPoint withTestConfigurationId(Integer testConfigurationId) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestConfigurationId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testConfigurationId = testConfigurationId;
return _x;
}
@Property(name="TestCaseId")
@JsonIgnore
public Optional getTestCaseId() {
return Optional.ofNullable(testCaseId);
}
public TestPoint withTestCaseId(Integer testCaseId) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TestCaseId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testCaseId = testCaseId;
return _x;
}
@Property(name="TesterUserSK")
@JsonIgnore
public Optional getTesterUserSK() {
return Optional.ofNullable(testerUserSK);
}
public TestPoint withTesterUserSK(String testerUserSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("TesterUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.testerUserSK = testerUserSK;
return _x;
}
@Property(name="AssignedToUserSK")
@JsonIgnore
public Optional getAssignedToUserSK() {
return Optional.ofNullable(assignedToUserSK);
}
public TestPoint withAssignedToUserSK(String assignedToUserSK) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("AssignedToUserSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.assignedToUserSK = assignedToUserSK;
return _x;
}
@Property(name="Priority")
@JsonIgnore
public Optional getPriority() {
return Optional.ofNullable(priority);
}
public TestPoint withPriority(Integer priority) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("Priority");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.priority = priority;
return _x;
}
@Property(name="AutomationStatus")
@JsonIgnore
public Optional getAutomationStatus() {
return Optional.ofNullable(automationStatus);
}
public TestPoint withAutomationStatus(String automationStatus) {
TestPoint _x = _copy();
_x.changedFields = changedFields.add("AutomationStatus");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestPoint");
_x.automationStatus = automationStatus;
return _x;
}
public TestPoint withUnmappedField(String name, String value) {
TestPoint _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="ChangedOn")
@JsonIgnore
public CalendarDateRequest getChangedOn() {
return new CalendarDateRequest(contextPath.addSegment("ChangedOn"), RequestHelper.getValue(unmappedFields, "ChangedOn"));
}
@NavigationProperty(name="Project")
@JsonIgnore
public ProjectRequest getProject() {
return new ProjectRequest(contextPath.addSegment("Project"), RequestHelper.getValue(unmappedFields, "Project"));
}
@NavigationProperty(name="TestSuite")
@JsonIgnore
public TestSuiteRequest getTestSuite() {
return new TestSuiteRequest(contextPath.addSegment("TestSuite"), RequestHelper.getValue(unmappedFields, "TestSuite"));
}
@NavigationProperty(name="TestConfiguration")
@JsonIgnore
public TestConfigurationRequest getTestConfiguration() {
return new TestConfigurationRequest(contextPath.addSegment("TestConfiguration"), RequestHelper.getValue(unmappedFields, "TestConfiguration"));
}
@NavigationProperty(name="TestCase")
@JsonIgnore
public WorkItemRequest getTestCase() {
return new WorkItemRequest(contextPath.addSegment("TestCase"), RequestHelper.getValue(unmappedFields, "TestCase"));
}
@NavigationProperty(name="Tester")
@JsonIgnore
public UserRequest getTester() {
return new UserRequest(contextPath.addSegment("Tester"), RequestHelper.getValue(unmappedFields, "Tester"));
}
@NavigationProperty(name="AssignedTo")
@JsonIgnore
public UserRequest getAssignedTo() {
return new UserRequest(contextPath.addSegment("AssignedTo"), RequestHelper.getValue(unmappedFields, "AssignedTo"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public TestPoint patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
TestPoint _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public TestPoint put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
TestPoint _x = _copy();
_x.changedFields = null;
return _x;
}
private TestPoint _copy() {
TestPoint _x = new TestPoint();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.lastResultState = lastResultState;
_x.lastResultOutcome = lastResultOutcome;
_x.changedDate = changedDate;
_x.changedDateSK = changedDateSK;
_x.testPointSK = testPointSK;
_x.analyticsUpdatedDate = analyticsUpdatedDate;
_x.projectSK = projectSK;
_x.testSuiteSK = testSuiteSK;
_x.testPlanId = testPlanId;
_x.testSuiteId = testSuiteId;
_x.testPointId = testPointId;
_x.testConfigurationSK = testConfigurationSK;
_x.testConfigurationId = testConfigurationId;
_x.testCaseId = testCaseId;
_x.testerUserSK = testerUserSK;
_x.assignedToUserSK = assignedToUserSK;
_x.priority = priority;
_x.automationStatus = automationStatus;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("TestPoint[");
b.append("LastResultState=");
b.append(this.lastResultState);
b.append(", ");
b.append("LastResultOutcome=");
b.append(this.lastResultOutcome);
b.append(", ");
b.append("ChangedDate=");
b.append(this.changedDate);
b.append(", ");
b.append("ChangedDateSK=");
b.append(this.changedDateSK);
b.append(", ");
b.append("TestPointSK=");
b.append(this.testPointSK);
b.append(", ");
b.append("AnalyticsUpdatedDate=");
b.append(this.analyticsUpdatedDate);
b.append(", ");
b.append("ProjectSK=");
b.append(this.projectSK);
b.append(", ");
b.append("TestSuiteSK=");
b.append(this.testSuiteSK);
b.append(", ");
b.append("TestPlanId=");
b.append(this.testPlanId);
b.append(", ");
b.append("TestSuiteId=");
b.append(this.testSuiteId);
b.append(", ");
b.append("TestPointId=");
b.append(this.testPointId);
b.append(", ");
b.append("TestConfigurationSK=");
b.append(this.testConfigurationSK);
b.append(", ");
b.append("TestConfigurationId=");
b.append(this.testConfigurationId);
b.append(", ");
b.append("TestCaseId=");
b.append(this.testCaseId);
b.append(", ");
b.append("TesterUserSK=");
b.append(this.testerUserSK);
b.append(", ");
b.append("AssignedToUserSK=");
b.append(this.assignedToUserSK);
b.append(", ");
b.append("Priority=");
b.append(this.priority);
b.append(", ");
b.append("AutomationStatus=");
b.append(this.automationStatus);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy