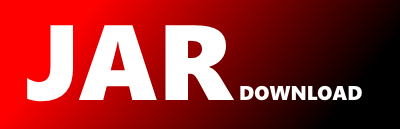
microsoft.vs.analytics.v4.model.entity.PipelineRun Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-analytics Show documentation
Show all versions of odata-client-microsoft-analytics Show documentation
Java client as template for Microsoft Analytics organisation endpoints
package microsoft.vs.analytics.v4.model.entity;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.vs.analytics.v4.model.entity.request.BranchRequest;
import microsoft.vs.analytics.v4.model.entity.request.CalendarDateRequest;
import microsoft.vs.analytics.v4.model.entity.request.PipelineRequest;
import microsoft.vs.analytics.v4.model.entity.request.ProjectRequest;
import microsoft.vs.analytics.v4.model.enums.PipelineRunOutcome;
import microsoft.vs.analytics.v4.model.enums.PipelineRunReason;
@JsonPropertyOrder({
"@odata.type",
"ProjectSK",
"PipelineRunSK",
"PipelineRunId",
"PipelineId",
"PipelineSK",
"BranchSK",
"RunNumber",
"RunNumberRevision",
"RunReason",
"RunOutcome",
"QueuedDate",
"QueuedDateSK",
"StartedDate",
"StartedDateSK",
"CompletedDate",
"CompletedDateSK",
"RunDurationSeconds",
"QueueDurationSeconds",
"TotalDurationSeconds",
"SucceededCount",
"PartiallySucceededCount",
"FailedCount",
"CanceledCount"})
@JsonInclude(Include.NON_NULL)
public class PipelineRun implements ODataEntityType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JacksonInject
@JsonIgnore
protected ChangedFields changedFields;
@Override
public String odataTypeName() {
return "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun";
}
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("ProjectSK")
protected String projectSK;
@JsonProperty("PipelineRunSK")
protected Integer pipelineRunSK;
@JsonProperty("PipelineRunId")
protected Integer pipelineRunId;
@JsonProperty("PipelineId")
protected Integer pipelineId;
@JsonProperty("PipelineSK")
protected Integer pipelineSK;
@JsonProperty("BranchSK")
protected Integer branchSK;
@JsonProperty("RunNumber")
protected String runNumber;
@JsonProperty("RunNumberRevision")
protected Integer runNumberRevision;
@JsonProperty("RunReason")
protected PipelineRunReason runReason;
@JsonProperty("RunOutcome")
protected PipelineRunOutcome runOutcome;
@JsonProperty("QueuedDate")
protected OffsetDateTime queuedDate;
@JsonProperty("QueuedDateSK")
protected Integer queuedDateSK;
@JsonProperty("StartedDate")
protected OffsetDateTime startedDate;
@JsonProperty("StartedDateSK")
protected Integer startedDateSK;
@JsonProperty("CompletedDate")
protected OffsetDateTime completedDate;
@JsonProperty("CompletedDateSK")
protected Integer completedDateSK;
@JsonProperty("RunDurationSeconds")
protected BigDecimal runDurationSeconds;
@JsonProperty("QueueDurationSeconds")
protected BigDecimal queueDurationSeconds;
@JsonProperty("TotalDurationSeconds")
protected BigDecimal totalDurationSeconds;
@JsonProperty("SucceededCount")
protected Integer succeededCount;
@JsonProperty("PartiallySucceededCount")
protected Integer partiallySucceededCount;
@JsonProperty("FailedCount")
protected Integer failedCount;
@JsonProperty("CanceledCount")
protected Integer canceledCount;
protected PipelineRun() {
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String projectSK;
private Integer pipelineRunSK;
private Integer pipelineRunId;
private Integer pipelineId;
private Integer pipelineSK;
private Integer branchSK;
private String runNumber;
private Integer runNumberRevision;
private PipelineRunReason runReason;
private PipelineRunOutcome runOutcome;
private OffsetDateTime queuedDate;
private Integer queuedDateSK;
private OffsetDateTime startedDate;
private Integer startedDateSK;
private OffsetDateTime completedDate;
private Integer completedDateSK;
private BigDecimal runDurationSeconds;
private BigDecimal queueDurationSeconds;
private BigDecimal totalDurationSeconds;
private Integer succeededCount;
private Integer partiallySucceededCount;
private Integer failedCount;
private Integer canceledCount;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder projectSK(String projectSK) {
this.projectSK = projectSK;
this.changedFields = changedFields.add("ProjectSK");
return this;
}
public Builder pipelineRunSK(Integer pipelineRunSK) {
this.pipelineRunSK = pipelineRunSK;
this.changedFields = changedFields.add("PipelineRunSK");
return this;
}
public Builder pipelineRunId(Integer pipelineRunId) {
this.pipelineRunId = pipelineRunId;
this.changedFields = changedFields.add("PipelineRunId");
return this;
}
public Builder pipelineId(Integer pipelineId) {
this.pipelineId = pipelineId;
this.changedFields = changedFields.add("PipelineId");
return this;
}
public Builder pipelineSK(Integer pipelineSK) {
this.pipelineSK = pipelineSK;
this.changedFields = changedFields.add("PipelineSK");
return this;
}
public Builder branchSK(Integer branchSK) {
this.branchSK = branchSK;
this.changedFields = changedFields.add("BranchSK");
return this;
}
public Builder runNumber(String runNumber) {
this.runNumber = runNumber;
this.changedFields = changedFields.add("RunNumber");
return this;
}
public Builder runNumberRevision(Integer runNumberRevision) {
this.runNumberRevision = runNumberRevision;
this.changedFields = changedFields.add("RunNumberRevision");
return this;
}
public Builder runReason(PipelineRunReason runReason) {
this.runReason = runReason;
this.changedFields = changedFields.add("RunReason");
return this;
}
public Builder runOutcome(PipelineRunOutcome runOutcome) {
this.runOutcome = runOutcome;
this.changedFields = changedFields.add("RunOutcome");
return this;
}
public Builder queuedDate(OffsetDateTime queuedDate) {
this.queuedDate = queuedDate;
this.changedFields = changedFields.add("QueuedDate");
return this;
}
public Builder queuedDateSK(Integer queuedDateSK) {
this.queuedDateSK = queuedDateSK;
this.changedFields = changedFields.add("QueuedDateSK");
return this;
}
public Builder startedDate(OffsetDateTime startedDate) {
this.startedDate = startedDate;
this.changedFields = changedFields.add("StartedDate");
return this;
}
public Builder startedDateSK(Integer startedDateSK) {
this.startedDateSK = startedDateSK;
this.changedFields = changedFields.add("StartedDateSK");
return this;
}
public Builder completedDate(OffsetDateTime completedDate) {
this.completedDate = completedDate;
this.changedFields = changedFields.add("CompletedDate");
return this;
}
public Builder completedDateSK(Integer completedDateSK) {
this.completedDateSK = completedDateSK;
this.changedFields = changedFields.add("CompletedDateSK");
return this;
}
public Builder runDurationSeconds(BigDecimal runDurationSeconds) {
this.runDurationSeconds = runDurationSeconds;
this.changedFields = changedFields.add("RunDurationSeconds");
return this;
}
public Builder queueDurationSeconds(BigDecimal queueDurationSeconds) {
this.queueDurationSeconds = queueDurationSeconds;
this.changedFields = changedFields.add("QueueDurationSeconds");
return this;
}
public Builder totalDurationSeconds(BigDecimal totalDurationSeconds) {
this.totalDurationSeconds = totalDurationSeconds;
this.changedFields = changedFields.add("TotalDurationSeconds");
return this;
}
public Builder succeededCount(Integer succeededCount) {
this.succeededCount = succeededCount;
this.changedFields = changedFields.add("SucceededCount");
return this;
}
public Builder partiallySucceededCount(Integer partiallySucceededCount) {
this.partiallySucceededCount = partiallySucceededCount;
this.changedFields = changedFields.add("PartiallySucceededCount");
return this;
}
public Builder failedCount(Integer failedCount) {
this.failedCount = failedCount;
this.changedFields = changedFields.add("FailedCount");
return this;
}
public Builder canceledCount(Integer canceledCount) {
this.canceledCount = canceledCount;
this.changedFields = changedFields.add("CanceledCount");
return this;
}
public PipelineRun build() {
PipelineRun _x = new PipelineRun();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun";
_x.projectSK = projectSK;
_x.pipelineRunSK = pipelineRunSK;
_x.pipelineRunId = pipelineRunId;
_x.pipelineId = pipelineId;
_x.pipelineSK = pipelineSK;
_x.branchSK = branchSK;
_x.runNumber = runNumber;
_x.runNumberRevision = runNumberRevision;
_x.runReason = runReason;
_x.runOutcome = runOutcome;
_x.queuedDate = queuedDate;
_x.queuedDateSK = queuedDateSK;
_x.startedDate = startedDate;
_x.startedDateSK = startedDateSK;
_x.completedDate = completedDate;
_x.completedDateSK = completedDateSK;
_x.runDurationSeconds = runDurationSeconds;
_x.queueDurationSeconds = queueDurationSeconds;
_x.totalDurationSeconds = totalDurationSeconds;
_x.succeededCount = succeededCount;
_x.partiallySucceededCount = partiallySucceededCount;
_x.failedCount = failedCount;
_x.canceledCount = canceledCount;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && pipelineRunSK != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(pipelineRunSK.toString()));
}
}
@Property(name="ProjectSK")
@JsonIgnore
public Optional getProjectSK() {
return Optional.ofNullable(projectSK);
}
public PipelineRun withProjectSK(String projectSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("ProjectSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.projectSK = projectSK;
return _x;
}
@Property(name="PipelineRunSK")
@JsonIgnore
public Optional getPipelineRunSK() {
return Optional.ofNullable(pipelineRunSK);
}
public PipelineRun withPipelineRunSK(Integer pipelineRunSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("PipelineRunSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.pipelineRunSK = pipelineRunSK;
return _x;
}
@Property(name="PipelineRunId")
@JsonIgnore
public Optional getPipelineRunId() {
return Optional.ofNullable(pipelineRunId);
}
public PipelineRun withPipelineRunId(Integer pipelineRunId) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("PipelineRunId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.pipelineRunId = pipelineRunId;
return _x;
}
@Property(name="PipelineId")
@JsonIgnore
public Optional getPipelineId() {
return Optional.ofNullable(pipelineId);
}
public PipelineRun withPipelineId(Integer pipelineId) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("PipelineId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.pipelineId = pipelineId;
return _x;
}
@Property(name="PipelineSK")
@JsonIgnore
public Optional getPipelineSK() {
return Optional.ofNullable(pipelineSK);
}
public PipelineRun withPipelineSK(Integer pipelineSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("PipelineSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.pipelineSK = pipelineSK;
return _x;
}
@Property(name="BranchSK")
@JsonIgnore
public Optional getBranchSK() {
return Optional.ofNullable(branchSK);
}
public PipelineRun withBranchSK(Integer branchSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("BranchSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.branchSK = branchSK;
return _x;
}
@Property(name="RunNumber")
@JsonIgnore
public Optional getRunNumber() {
return Optional.ofNullable(runNumber);
}
public PipelineRun withRunNumber(String runNumber) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("RunNumber");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.runNumber = runNumber;
return _x;
}
@Property(name="RunNumberRevision")
@JsonIgnore
public Optional getRunNumberRevision() {
return Optional.ofNullable(runNumberRevision);
}
public PipelineRun withRunNumberRevision(Integer runNumberRevision) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("RunNumberRevision");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.runNumberRevision = runNumberRevision;
return _x;
}
@Property(name="RunReason")
@JsonIgnore
public Optional getRunReason() {
return Optional.ofNullable(runReason);
}
public PipelineRun withRunReason(PipelineRunReason runReason) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("RunReason");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.runReason = runReason;
return _x;
}
@Property(name="RunOutcome")
@JsonIgnore
public Optional getRunOutcome() {
return Optional.ofNullable(runOutcome);
}
public PipelineRun withRunOutcome(PipelineRunOutcome runOutcome) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("RunOutcome");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.runOutcome = runOutcome;
return _x;
}
@Property(name="QueuedDate")
@JsonIgnore
public Optional getQueuedDate() {
return Optional.ofNullable(queuedDate);
}
public PipelineRun withQueuedDate(OffsetDateTime queuedDate) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("QueuedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.queuedDate = queuedDate;
return _x;
}
@Property(name="QueuedDateSK")
@JsonIgnore
public Optional getQueuedDateSK() {
return Optional.ofNullable(queuedDateSK);
}
public PipelineRun withQueuedDateSK(Integer queuedDateSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("QueuedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.queuedDateSK = queuedDateSK;
return _x;
}
@Property(name="StartedDate")
@JsonIgnore
public Optional getStartedDate() {
return Optional.ofNullable(startedDate);
}
public PipelineRun withStartedDate(OffsetDateTime startedDate) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("StartedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.startedDate = startedDate;
return _x;
}
@Property(name="StartedDateSK")
@JsonIgnore
public Optional getStartedDateSK() {
return Optional.ofNullable(startedDateSK);
}
public PipelineRun withStartedDateSK(Integer startedDateSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("StartedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.startedDateSK = startedDateSK;
return _x;
}
@Property(name="CompletedDate")
@JsonIgnore
public Optional getCompletedDate() {
return Optional.ofNullable(completedDate);
}
public PipelineRun withCompletedDate(OffsetDateTime completedDate) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("CompletedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.completedDate = completedDate;
return _x;
}
@Property(name="CompletedDateSK")
@JsonIgnore
public Optional getCompletedDateSK() {
return Optional.ofNullable(completedDateSK);
}
public PipelineRun withCompletedDateSK(Integer completedDateSK) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("CompletedDateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.completedDateSK = completedDateSK;
return _x;
}
@Property(name="RunDurationSeconds")
@JsonIgnore
public Optional getRunDurationSeconds() {
return Optional.ofNullable(runDurationSeconds);
}
public PipelineRun withRunDurationSeconds(BigDecimal runDurationSeconds) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("RunDurationSeconds");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.runDurationSeconds = runDurationSeconds;
return _x;
}
@Property(name="QueueDurationSeconds")
@JsonIgnore
public Optional getQueueDurationSeconds() {
return Optional.ofNullable(queueDurationSeconds);
}
public PipelineRun withQueueDurationSeconds(BigDecimal queueDurationSeconds) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("QueueDurationSeconds");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.queueDurationSeconds = queueDurationSeconds;
return _x;
}
@Property(name="TotalDurationSeconds")
@JsonIgnore
public Optional getTotalDurationSeconds() {
return Optional.ofNullable(totalDurationSeconds);
}
public PipelineRun withTotalDurationSeconds(BigDecimal totalDurationSeconds) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("TotalDurationSeconds");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.totalDurationSeconds = totalDurationSeconds;
return _x;
}
@Property(name="SucceededCount")
@JsonIgnore
public Optional getSucceededCount() {
return Optional.ofNullable(succeededCount);
}
public PipelineRun withSucceededCount(Integer succeededCount) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("SucceededCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.succeededCount = succeededCount;
return _x;
}
@Property(name="PartiallySucceededCount")
@JsonIgnore
public Optional getPartiallySucceededCount() {
return Optional.ofNullable(partiallySucceededCount);
}
public PipelineRun withPartiallySucceededCount(Integer partiallySucceededCount) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("PartiallySucceededCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.partiallySucceededCount = partiallySucceededCount;
return _x;
}
@Property(name="FailedCount")
@JsonIgnore
public Optional getFailedCount() {
return Optional.ofNullable(failedCount);
}
public PipelineRun withFailedCount(Integer failedCount) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("FailedCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.failedCount = failedCount;
return _x;
}
@Property(name="CanceledCount")
@JsonIgnore
public Optional getCanceledCount() {
return Optional.ofNullable(canceledCount);
}
public PipelineRun withCanceledCount(Integer canceledCount) {
PipelineRun _x = _copy();
_x.changedFields = changedFields.add("CanceledCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.PipelineRun");
_x.canceledCount = canceledCount;
return _x;
}
public PipelineRun withUnmappedField(String name, String value) {
PipelineRun _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="Project")
@JsonIgnore
public ProjectRequest getProject() {
return new ProjectRequest(contextPath.addSegment("Project"), RequestHelper.getValue(unmappedFields, "Project"));
}
@NavigationProperty(name="Pipeline")
@JsonIgnore
public PipelineRequest getPipeline() {
return new PipelineRequest(contextPath.addSegment("Pipeline"), RequestHelper.getValue(unmappedFields, "Pipeline"));
}
@NavigationProperty(name="Branch")
@JsonIgnore
public BranchRequest getBranch() {
return new BranchRequest(contextPath.addSegment("Branch"), RequestHelper.getValue(unmappedFields, "Branch"));
}
@NavigationProperty(name="QueuedOn")
@JsonIgnore
public CalendarDateRequest getQueuedOn() {
return new CalendarDateRequest(contextPath.addSegment("QueuedOn"), RequestHelper.getValue(unmappedFields, "QueuedOn"));
}
@NavigationProperty(name="StartedOn")
@JsonIgnore
public CalendarDateRequest getStartedOn() {
return new CalendarDateRequest(contextPath.addSegment("StartedOn"), RequestHelper.getValue(unmappedFields, "StartedOn"));
}
@NavigationProperty(name="CompletedOn")
@JsonIgnore
public CalendarDateRequest getCompletedOn() {
return new CalendarDateRequest(contextPath.addSegment("CompletedOn"), RequestHelper.getValue(unmappedFields, "CompletedOn"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public PipelineRun patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
PipelineRun _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public PipelineRun put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
PipelineRun _x = _copy();
_x.changedFields = null;
return _x;
}
private PipelineRun _copy() {
PipelineRun _x = new PipelineRun();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.projectSK = projectSK;
_x.pipelineRunSK = pipelineRunSK;
_x.pipelineRunId = pipelineRunId;
_x.pipelineId = pipelineId;
_x.pipelineSK = pipelineSK;
_x.branchSK = branchSK;
_x.runNumber = runNumber;
_x.runNumberRevision = runNumberRevision;
_x.runReason = runReason;
_x.runOutcome = runOutcome;
_x.queuedDate = queuedDate;
_x.queuedDateSK = queuedDateSK;
_x.startedDate = startedDate;
_x.startedDateSK = startedDateSK;
_x.completedDate = completedDate;
_x.completedDateSK = completedDateSK;
_x.runDurationSeconds = runDurationSeconds;
_x.queueDurationSeconds = queueDurationSeconds;
_x.totalDurationSeconds = totalDurationSeconds;
_x.succeededCount = succeededCount;
_x.partiallySucceededCount = partiallySucceededCount;
_x.failedCount = failedCount;
_x.canceledCount = canceledCount;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("PipelineRun[");
b.append("ProjectSK=");
b.append(this.projectSK);
b.append(", ");
b.append("PipelineRunSK=");
b.append(this.pipelineRunSK);
b.append(", ");
b.append("PipelineRunId=");
b.append(this.pipelineRunId);
b.append(", ");
b.append("PipelineId=");
b.append(this.pipelineId);
b.append(", ");
b.append("PipelineSK=");
b.append(this.pipelineSK);
b.append(", ");
b.append("BranchSK=");
b.append(this.branchSK);
b.append(", ");
b.append("RunNumber=");
b.append(this.runNumber);
b.append(", ");
b.append("RunNumberRevision=");
b.append(this.runNumberRevision);
b.append(", ");
b.append("RunReason=");
b.append(this.runReason);
b.append(", ");
b.append("RunOutcome=");
b.append(this.runOutcome);
b.append(", ");
b.append("QueuedDate=");
b.append(this.queuedDate);
b.append(", ");
b.append("QueuedDateSK=");
b.append(this.queuedDateSK);
b.append(", ");
b.append("StartedDate=");
b.append(this.startedDate);
b.append(", ");
b.append("StartedDateSK=");
b.append(this.startedDateSK);
b.append(", ");
b.append("CompletedDate=");
b.append(this.completedDate);
b.append(", ");
b.append("CompletedDateSK=");
b.append(this.completedDateSK);
b.append(", ");
b.append("RunDurationSeconds=");
b.append(this.runDurationSeconds);
b.append(", ");
b.append("QueueDurationSeconds=");
b.append(this.queueDurationSeconds);
b.append(", ");
b.append("TotalDurationSeconds=");
b.append(this.totalDurationSeconds);
b.append(", ");
b.append("SucceededCount=");
b.append(this.succeededCount);
b.append(", ");
b.append("PartiallySucceededCount=");
b.append(this.partiallySucceededCount);
b.append(", ");
b.append("FailedCount=");
b.append(this.failedCount);
b.append(", ");
b.append("CanceledCount=");
b.append(this.canceledCount);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy