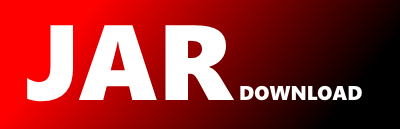
microsoft.vs.analytics.v4.model.entity.TestResultDaily Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-analytics Show documentation
Show all versions of odata-client-microsoft-analytics Show documentation
Java client as template for Microsoft Analytics organisation endpoints
package microsoft.vs.analytics.v4.model.entity;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.vs.analytics.v4.model.entity.request.BranchRequest;
import microsoft.vs.analytics.v4.model.entity.request.CalendarDateRequest;
import microsoft.vs.analytics.v4.model.entity.request.PipelineRequest;
import microsoft.vs.analytics.v4.model.entity.request.ProjectRequest;
import microsoft.vs.analytics.v4.model.entity.request.TestRequest;
import microsoft.vs.analytics.v4.model.enums.SourceWorkflow;
import microsoft.vs.analytics.v4.model.enums.TestRunType;
@JsonPropertyOrder({
"@odata.type",
"AnalyticsUpdatedDate",
"ProjectSK",
"TestResultDailySK",
"TestSK",
"PipelineSK",
"BranchSK",
"DateSK",
"ResultDurationSeconds",
"ResultCount",
"ResultPassCount",
"ResultFailCount",
"ResultFlakyCount",
"ResultNoneCount",
"ResultInconclusiveCount",
"ResultTimeoutCount",
"ResultAbortedCount",
"ResultBlockedCount",
"ResultNotExecutedCount",
"ResultWarningCount",
"ResultErrorCount",
"ResultNotApplicableCount",
"ResultNotImpactedCount",
"TestRunType",
"Workflow",
"ReleasePipelineId",
"ReleaseStageId"})
@JsonInclude(Include.NON_NULL)
public class TestResultDaily implements ODataEntityType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JacksonInject
@JsonIgnore
protected ChangedFields changedFields;
@Override
public String odataTypeName() {
return "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily";
}
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("AnalyticsUpdatedDate")
protected OffsetDateTime analyticsUpdatedDate;
@JsonProperty("ProjectSK")
protected String projectSK;
@JsonProperty("TestResultDailySK")
protected Long testResultDailySK;
@JsonProperty("TestSK")
protected Integer testSK;
@JsonProperty("PipelineSK")
protected Integer pipelineSK;
@JsonProperty("BranchSK")
protected Integer branchSK;
@JsonProperty("DateSK")
protected Integer dateSK;
@JsonProperty("ResultDurationSeconds")
protected BigDecimal resultDurationSeconds;
@JsonProperty("ResultCount")
protected Integer resultCount;
@JsonProperty("ResultPassCount")
protected Integer resultPassCount;
@JsonProperty("ResultFailCount")
protected Integer resultFailCount;
@JsonProperty("ResultFlakyCount")
protected Integer resultFlakyCount;
@JsonProperty("ResultNoneCount")
protected Integer resultNoneCount;
@JsonProperty("ResultInconclusiveCount")
protected Integer resultInconclusiveCount;
@JsonProperty("ResultTimeoutCount")
protected Integer resultTimeoutCount;
@JsonProperty("ResultAbortedCount")
protected Integer resultAbortedCount;
@JsonProperty("ResultBlockedCount")
protected Integer resultBlockedCount;
@JsonProperty("ResultNotExecutedCount")
protected Integer resultNotExecutedCount;
@JsonProperty("ResultWarningCount")
protected Integer resultWarningCount;
@JsonProperty("ResultErrorCount")
protected Integer resultErrorCount;
@JsonProperty("ResultNotApplicableCount")
protected Integer resultNotApplicableCount;
@JsonProperty("ResultNotImpactedCount")
protected Integer resultNotImpactedCount;
@JsonProperty("TestRunType")
protected TestRunType testRunType;
@JsonProperty("Workflow")
protected SourceWorkflow workflow;
@JsonProperty("ReleasePipelineId")
protected Integer releasePipelineId;
@JsonProperty("ReleaseStageId")
protected Integer releaseStageId;
protected TestResultDaily() {
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private OffsetDateTime analyticsUpdatedDate;
private String projectSK;
private Long testResultDailySK;
private Integer testSK;
private Integer pipelineSK;
private Integer branchSK;
private Integer dateSK;
private BigDecimal resultDurationSeconds;
private Integer resultCount;
private Integer resultPassCount;
private Integer resultFailCount;
private Integer resultFlakyCount;
private Integer resultNoneCount;
private Integer resultInconclusiveCount;
private Integer resultTimeoutCount;
private Integer resultAbortedCount;
private Integer resultBlockedCount;
private Integer resultNotExecutedCount;
private Integer resultWarningCount;
private Integer resultErrorCount;
private Integer resultNotApplicableCount;
private Integer resultNotImpactedCount;
private TestRunType testRunType;
private SourceWorkflow workflow;
private Integer releasePipelineId;
private Integer releaseStageId;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder analyticsUpdatedDate(OffsetDateTime analyticsUpdatedDate) {
this.analyticsUpdatedDate = analyticsUpdatedDate;
this.changedFields = changedFields.add("AnalyticsUpdatedDate");
return this;
}
public Builder projectSK(String projectSK) {
this.projectSK = projectSK;
this.changedFields = changedFields.add("ProjectSK");
return this;
}
public Builder testResultDailySK(Long testResultDailySK) {
this.testResultDailySK = testResultDailySK;
this.changedFields = changedFields.add("TestResultDailySK");
return this;
}
public Builder testSK(Integer testSK) {
this.testSK = testSK;
this.changedFields = changedFields.add("TestSK");
return this;
}
public Builder pipelineSK(Integer pipelineSK) {
this.pipelineSK = pipelineSK;
this.changedFields = changedFields.add("PipelineSK");
return this;
}
public Builder branchSK(Integer branchSK) {
this.branchSK = branchSK;
this.changedFields = changedFields.add("BranchSK");
return this;
}
public Builder dateSK(Integer dateSK) {
this.dateSK = dateSK;
this.changedFields = changedFields.add("DateSK");
return this;
}
public Builder resultDurationSeconds(BigDecimal resultDurationSeconds) {
this.resultDurationSeconds = resultDurationSeconds;
this.changedFields = changedFields.add("ResultDurationSeconds");
return this;
}
public Builder resultCount(Integer resultCount) {
this.resultCount = resultCount;
this.changedFields = changedFields.add("ResultCount");
return this;
}
public Builder resultPassCount(Integer resultPassCount) {
this.resultPassCount = resultPassCount;
this.changedFields = changedFields.add("ResultPassCount");
return this;
}
public Builder resultFailCount(Integer resultFailCount) {
this.resultFailCount = resultFailCount;
this.changedFields = changedFields.add("ResultFailCount");
return this;
}
public Builder resultFlakyCount(Integer resultFlakyCount) {
this.resultFlakyCount = resultFlakyCount;
this.changedFields = changedFields.add("ResultFlakyCount");
return this;
}
public Builder resultNoneCount(Integer resultNoneCount) {
this.resultNoneCount = resultNoneCount;
this.changedFields = changedFields.add("ResultNoneCount");
return this;
}
public Builder resultInconclusiveCount(Integer resultInconclusiveCount) {
this.resultInconclusiveCount = resultInconclusiveCount;
this.changedFields = changedFields.add("ResultInconclusiveCount");
return this;
}
public Builder resultTimeoutCount(Integer resultTimeoutCount) {
this.resultTimeoutCount = resultTimeoutCount;
this.changedFields = changedFields.add("ResultTimeoutCount");
return this;
}
public Builder resultAbortedCount(Integer resultAbortedCount) {
this.resultAbortedCount = resultAbortedCount;
this.changedFields = changedFields.add("ResultAbortedCount");
return this;
}
public Builder resultBlockedCount(Integer resultBlockedCount) {
this.resultBlockedCount = resultBlockedCount;
this.changedFields = changedFields.add("ResultBlockedCount");
return this;
}
public Builder resultNotExecutedCount(Integer resultNotExecutedCount) {
this.resultNotExecutedCount = resultNotExecutedCount;
this.changedFields = changedFields.add("ResultNotExecutedCount");
return this;
}
public Builder resultWarningCount(Integer resultWarningCount) {
this.resultWarningCount = resultWarningCount;
this.changedFields = changedFields.add("ResultWarningCount");
return this;
}
public Builder resultErrorCount(Integer resultErrorCount) {
this.resultErrorCount = resultErrorCount;
this.changedFields = changedFields.add("ResultErrorCount");
return this;
}
public Builder resultNotApplicableCount(Integer resultNotApplicableCount) {
this.resultNotApplicableCount = resultNotApplicableCount;
this.changedFields = changedFields.add("ResultNotApplicableCount");
return this;
}
public Builder resultNotImpactedCount(Integer resultNotImpactedCount) {
this.resultNotImpactedCount = resultNotImpactedCount;
this.changedFields = changedFields.add("ResultNotImpactedCount");
return this;
}
public Builder testRunType(TestRunType testRunType) {
this.testRunType = testRunType;
this.changedFields = changedFields.add("TestRunType");
return this;
}
public Builder workflow(SourceWorkflow workflow) {
this.workflow = workflow;
this.changedFields = changedFields.add("Workflow");
return this;
}
public Builder releasePipelineId(Integer releasePipelineId) {
this.releasePipelineId = releasePipelineId;
this.changedFields = changedFields.add("ReleasePipelineId");
return this;
}
public Builder releaseStageId(Integer releaseStageId) {
this.releaseStageId = releaseStageId;
this.changedFields = changedFields.add("ReleaseStageId");
return this;
}
public TestResultDaily build() {
TestResultDaily _x = new TestResultDaily();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily";
_x.analyticsUpdatedDate = analyticsUpdatedDate;
_x.projectSK = projectSK;
_x.testResultDailySK = testResultDailySK;
_x.testSK = testSK;
_x.pipelineSK = pipelineSK;
_x.branchSK = branchSK;
_x.dateSK = dateSK;
_x.resultDurationSeconds = resultDurationSeconds;
_x.resultCount = resultCount;
_x.resultPassCount = resultPassCount;
_x.resultFailCount = resultFailCount;
_x.resultFlakyCount = resultFlakyCount;
_x.resultNoneCount = resultNoneCount;
_x.resultInconclusiveCount = resultInconclusiveCount;
_x.resultTimeoutCount = resultTimeoutCount;
_x.resultAbortedCount = resultAbortedCount;
_x.resultBlockedCount = resultBlockedCount;
_x.resultNotExecutedCount = resultNotExecutedCount;
_x.resultWarningCount = resultWarningCount;
_x.resultErrorCount = resultErrorCount;
_x.resultNotApplicableCount = resultNotApplicableCount;
_x.resultNotImpactedCount = resultNotImpactedCount;
_x.testRunType = testRunType;
_x.workflow = workflow;
_x.releasePipelineId = releasePipelineId;
_x.releaseStageId = releaseStageId;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && testResultDailySK != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(testResultDailySK.toString()));
}
}
@Property(name="AnalyticsUpdatedDate")
@JsonIgnore
public Optional getAnalyticsUpdatedDate() {
return Optional.ofNullable(analyticsUpdatedDate);
}
public TestResultDaily withAnalyticsUpdatedDate(OffsetDateTime analyticsUpdatedDate) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("AnalyticsUpdatedDate");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.analyticsUpdatedDate = analyticsUpdatedDate;
return _x;
}
@Property(name="ProjectSK")
@JsonIgnore
public Optional getProjectSK() {
return Optional.ofNullable(projectSK);
}
public TestResultDaily withProjectSK(String projectSK) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ProjectSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.projectSK = projectSK;
return _x;
}
@Property(name="TestResultDailySK")
@JsonIgnore
public Optional getTestResultDailySK() {
return Optional.ofNullable(testResultDailySK);
}
public TestResultDaily withTestResultDailySK(Long testResultDailySK) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("TestResultDailySK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.testResultDailySK = testResultDailySK;
return _x;
}
@Property(name="TestSK")
@JsonIgnore
public Optional getTestSK() {
return Optional.ofNullable(testSK);
}
public TestResultDaily withTestSK(Integer testSK) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("TestSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.testSK = testSK;
return _x;
}
@Property(name="PipelineSK")
@JsonIgnore
public Optional getPipelineSK() {
return Optional.ofNullable(pipelineSK);
}
public TestResultDaily withPipelineSK(Integer pipelineSK) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("PipelineSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.pipelineSK = pipelineSK;
return _x;
}
@Property(name="BranchSK")
@JsonIgnore
public Optional getBranchSK() {
return Optional.ofNullable(branchSK);
}
public TestResultDaily withBranchSK(Integer branchSK) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("BranchSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.branchSK = branchSK;
return _x;
}
@Property(name="DateSK")
@JsonIgnore
public Optional getDateSK() {
return Optional.ofNullable(dateSK);
}
public TestResultDaily withDateSK(Integer dateSK) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("DateSK");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.dateSK = dateSK;
return _x;
}
@Property(name="ResultDurationSeconds")
@JsonIgnore
public Optional getResultDurationSeconds() {
return Optional.ofNullable(resultDurationSeconds);
}
public TestResultDaily withResultDurationSeconds(BigDecimal resultDurationSeconds) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultDurationSeconds");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultDurationSeconds = resultDurationSeconds;
return _x;
}
@Property(name="ResultCount")
@JsonIgnore
public Optional getResultCount() {
return Optional.ofNullable(resultCount);
}
public TestResultDaily withResultCount(Integer resultCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultCount = resultCount;
return _x;
}
@Property(name="ResultPassCount")
@JsonIgnore
public Optional getResultPassCount() {
return Optional.ofNullable(resultPassCount);
}
public TestResultDaily withResultPassCount(Integer resultPassCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultPassCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultPassCount = resultPassCount;
return _x;
}
@Property(name="ResultFailCount")
@JsonIgnore
public Optional getResultFailCount() {
return Optional.ofNullable(resultFailCount);
}
public TestResultDaily withResultFailCount(Integer resultFailCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultFailCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultFailCount = resultFailCount;
return _x;
}
@Property(name="ResultFlakyCount")
@JsonIgnore
public Optional getResultFlakyCount() {
return Optional.ofNullable(resultFlakyCount);
}
public TestResultDaily withResultFlakyCount(Integer resultFlakyCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultFlakyCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultFlakyCount = resultFlakyCount;
return _x;
}
@Property(name="ResultNoneCount")
@JsonIgnore
public Optional getResultNoneCount() {
return Optional.ofNullable(resultNoneCount);
}
public TestResultDaily withResultNoneCount(Integer resultNoneCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultNoneCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultNoneCount = resultNoneCount;
return _x;
}
@Property(name="ResultInconclusiveCount")
@JsonIgnore
public Optional getResultInconclusiveCount() {
return Optional.ofNullable(resultInconclusiveCount);
}
public TestResultDaily withResultInconclusiveCount(Integer resultInconclusiveCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultInconclusiveCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultInconclusiveCount = resultInconclusiveCount;
return _x;
}
@Property(name="ResultTimeoutCount")
@JsonIgnore
public Optional getResultTimeoutCount() {
return Optional.ofNullable(resultTimeoutCount);
}
public TestResultDaily withResultTimeoutCount(Integer resultTimeoutCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultTimeoutCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultTimeoutCount = resultTimeoutCount;
return _x;
}
@Property(name="ResultAbortedCount")
@JsonIgnore
public Optional getResultAbortedCount() {
return Optional.ofNullable(resultAbortedCount);
}
public TestResultDaily withResultAbortedCount(Integer resultAbortedCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultAbortedCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultAbortedCount = resultAbortedCount;
return _x;
}
@Property(name="ResultBlockedCount")
@JsonIgnore
public Optional getResultBlockedCount() {
return Optional.ofNullable(resultBlockedCount);
}
public TestResultDaily withResultBlockedCount(Integer resultBlockedCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultBlockedCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultBlockedCount = resultBlockedCount;
return _x;
}
@Property(name="ResultNotExecutedCount")
@JsonIgnore
public Optional getResultNotExecutedCount() {
return Optional.ofNullable(resultNotExecutedCount);
}
public TestResultDaily withResultNotExecutedCount(Integer resultNotExecutedCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultNotExecutedCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultNotExecutedCount = resultNotExecutedCount;
return _x;
}
@Property(name="ResultWarningCount")
@JsonIgnore
public Optional getResultWarningCount() {
return Optional.ofNullable(resultWarningCount);
}
public TestResultDaily withResultWarningCount(Integer resultWarningCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultWarningCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultWarningCount = resultWarningCount;
return _x;
}
@Property(name="ResultErrorCount")
@JsonIgnore
public Optional getResultErrorCount() {
return Optional.ofNullable(resultErrorCount);
}
public TestResultDaily withResultErrorCount(Integer resultErrorCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultErrorCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultErrorCount = resultErrorCount;
return _x;
}
@Property(name="ResultNotApplicableCount")
@JsonIgnore
public Optional getResultNotApplicableCount() {
return Optional.ofNullable(resultNotApplicableCount);
}
public TestResultDaily withResultNotApplicableCount(Integer resultNotApplicableCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultNotApplicableCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultNotApplicableCount = resultNotApplicableCount;
return _x;
}
@Property(name="ResultNotImpactedCount")
@JsonIgnore
public Optional getResultNotImpactedCount() {
return Optional.ofNullable(resultNotImpactedCount);
}
public TestResultDaily withResultNotImpactedCount(Integer resultNotImpactedCount) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ResultNotImpactedCount");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.resultNotImpactedCount = resultNotImpactedCount;
return _x;
}
@Property(name="TestRunType")
@JsonIgnore
public Optional getTestRunType() {
return Optional.ofNullable(testRunType);
}
public TestResultDaily withTestRunType(TestRunType testRunType) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("TestRunType");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.testRunType = testRunType;
return _x;
}
@Property(name="Workflow")
@JsonIgnore
public Optional getWorkflow() {
return Optional.ofNullable(workflow);
}
public TestResultDaily withWorkflow(SourceWorkflow workflow) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("Workflow");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.workflow = workflow;
return _x;
}
@Property(name="ReleasePipelineId")
@JsonIgnore
public Optional getReleasePipelineId() {
return Optional.ofNullable(releasePipelineId);
}
public TestResultDaily withReleasePipelineId(Integer releasePipelineId) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ReleasePipelineId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.releasePipelineId = releasePipelineId;
return _x;
}
@Property(name="ReleaseStageId")
@JsonIgnore
public Optional getReleaseStageId() {
return Optional.ofNullable(releaseStageId);
}
public TestResultDaily withReleaseStageId(Integer releaseStageId) {
TestResultDaily _x = _copy();
_x.changedFields = changedFields.add("ReleaseStageId");
_x.odataType = Util.nvl(odataType, "Microsoft.VisualStudio.Services.Analytics.Model.TestResultDaily");
_x.releaseStageId = releaseStageId;
return _x;
}
public TestResultDaily withUnmappedField(String name, String value) {
TestResultDaily _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="Project")
@JsonIgnore
public ProjectRequest getProject() {
return new ProjectRequest(contextPath.addSegment("Project"), RequestHelper.getValue(unmappedFields, "Project"));
}
@NavigationProperty(name="Test")
@JsonIgnore
public TestRequest getTest() {
return new TestRequest(contextPath.addSegment("Test"), RequestHelper.getValue(unmappedFields, "Test"));
}
@NavigationProperty(name="Pipeline")
@JsonIgnore
public PipelineRequest getPipeline() {
return new PipelineRequest(contextPath.addSegment("Pipeline"), RequestHelper.getValue(unmappedFields, "Pipeline"));
}
@NavigationProperty(name="Branch")
@JsonIgnore
public BranchRequest getBranch() {
return new BranchRequest(contextPath.addSegment("Branch"), RequestHelper.getValue(unmappedFields, "Branch"));
}
@NavigationProperty(name="Date")
@JsonIgnore
public CalendarDateRequest getDate() {
return new CalendarDateRequest(contextPath.addSegment("Date"), RequestHelper.getValue(unmappedFields, "Date"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public TestResultDaily patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
TestResultDaily _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public TestResultDaily put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
TestResultDaily _x = _copy();
_x.changedFields = null;
return _x;
}
private TestResultDaily _copy() {
TestResultDaily _x = new TestResultDaily();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.analyticsUpdatedDate = analyticsUpdatedDate;
_x.projectSK = projectSK;
_x.testResultDailySK = testResultDailySK;
_x.testSK = testSK;
_x.pipelineSK = pipelineSK;
_x.branchSK = branchSK;
_x.dateSK = dateSK;
_x.resultDurationSeconds = resultDurationSeconds;
_x.resultCount = resultCount;
_x.resultPassCount = resultPassCount;
_x.resultFailCount = resultFailCount;
_x.resultFlakyCount = resultFlakyCount;
_x.resultNoneCount = resultNoneCount;
_x.resultInconclusiveCount = resultInconclusiveCount;
_x.resultTimeoutCount = resultTimeoutCount;
_x.resultAbortedCount = resultAbortedCount;
_x.resultBlockedCount = resultBlockedCount;
_x.resultNotExecutedCount = resultNotExecutedCount;
_x.resultWarningCount = resultWarningCount;
_x.resultErrorCount = resultErrorCount;
_x.resultNotApplicableCount = resultNotApplicableCount;
_x.resultNotImpactedCount = resultNotImpactedCount;
_x.testRunType = testRunType;
_x.workflow = workflow;
_x.releasePipelineId = releasePipelineId;
_x.releaseStageId = releaseStageId;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("TestResultDaily[");
b.append("AnalyticsUpdatedDate=");
b.append(this.analyticsUpdatedDate);
b.append(", ");
b.append("ProjectSK=");
b.append(this.projectSK);
b.append(", ");
b.append("TestResultDailySK=");
b.append(this.testResultDailySK);
b.append(", ");
b.append("TestSK=");
b.append(this.testSK);
b.append(", ");
b.append("PipelineSK=");
b.append(this.pipelineSK);
b.append(", ");
b.append("BranchSK=");
b.append(this.branchSK);
b.append(", ");
b.append("DateSK=");
b.append(this.dateSK);
b.append(", ");
b.append("ResultDurationSeconds=");
b.append(this.resultDurationSeconds);
b.append(", ");
b.append("ResultCount=");
b.append(this.resultCount);
b.append(", ");
b.append("ResultPassCount=");
b.append(this.resultPassCount);
b.append(", ");
b.append("ResultFailCount=");
b.append(this.resultFailCount);
b.append(", ");
b.append("ResultFlakyCount=");
b.append(this.resultFlakyCount);
b.append(", ");
b.append("ResultNoneCount=");
b.append(this.resultNoneCount);
b.append(", ");
b.append("ResultInconclusiveCount=");
b.append(this.resultInconclusiveCount);
b.append(", ");
b.append("ResultTimeoutCount=");
b.append(this.resultTimeoutCount);
b.append(", ");
b.append("ResultAbortedCount=");
b.append(this.resultAbortedCount);
b.append(", ");
b.append("ResultBlockedCount=");
b.append(this.resultBlockedCount);
b.append(", ");
b.append("ResultNotExecutedCount=");
b.append(this.resultNotExecutedCount);
b.append(", ");
b.append("ResultWarningCount=");
b.append(this.resultWarningCount);
b.append(", ");
b.append("ResultErrorCount=");
b.append(this.resultErrorCount);
b.append(", ");
b.append("ResultNotApplicableCount=");
b.append(this.resultNotApplicableCount);
b.append(", ");
b.append("ResultNotImpactedCount=");
b.append(this.resultNotImpactedCount);
b.append(", ");
b.append("TestRunType=");
b.append(this.testRunType);
b.append(", ");
b.append("Workflow=");
b.append(this.workflow);
b.append(", ");
b.append("ReleasePipelineId=");
b.append(this.releasePipelineId);
b.append(", ");
b.append("ReleaseStageId=");
b.append(this.releaseStageId);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy