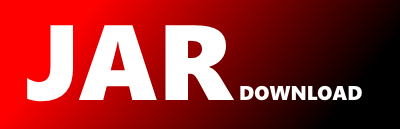
microsoft.dynamics.crm.complex.AppEntityInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.EdmSchemaInfo;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"EntityId",
"ChartIds",
"Forms",
"Views",
"EntityDashboards"})
@JsonInclude(Include.NON_NULL)
public class AppEntityInfo implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("EntityId")
protected String entityId;
@JsonProperty("ChartIds")
protected List chartIds;
@JsonProperty("ChartIds@nextLink")
protected String chartIdsNextLink;
@JsonProperty("Forms")
protected List forms;
@JsonProperty("Forms@nextLink")
protected String formsNextLink;
@JsonProperty("Views")
protected List views;
@JsonProperty("Views@nextLink")
protected String viewsNextLink;
@JsonProperty("EntityDashboards")
protected List entityDashboards;
@JsonProperty("EntityDashboards@nextLink")
protected String entityDashboardsNextLink;
protected AppEntityInfo() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.AppEntityInfo";
}
@Property(name="EntityId")
@JsonIgnore
public Optional getEntityId() {
return Optional.ofNullable(entityId);
}
public AppEntityInfo withEntityId(String entityId) {
Checks.checkIsAscii(entityId);
AppEntityInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppEntityInfo");
_x.entityId = entityId;
return _x;
}
@Property(name="ChartIds")
@JsonIgnore
public CollectionPage getChartIds() {
return new CollectionPage(contextPath, String.class, this.chartIds, Optional.ofNullable(chartIdsNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="ChartIds")
@JsonIgnore
public CollectionPage getChartIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.chartIds, Optional.ofNullable(chartIdsNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="Forms")
@JsonIgnore
public CollectionPage getForms() {
return new CollectionPage(contextPath, ArtifactIdType.class, this.forms, Optional.ofNullable(formsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Forms")
@JsonIgnore
public CollectionPage getForms(HttpRequestOptions options) {
return new CollectionPage(contextPath, ArtifactIdType.class, this.forms, Optional.ofNullable(formsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="Views")
@JsonIgnore
public CollectionPage getViews() {
return new CollectionPage(contextPath, ArtifactIdType.class, this.views, Optional.ofNullable(viewsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Views")
@JsonIgnore
public CollectionPage getViews(HttpRequestOptions options) {
return new CollectionPage(contextPath, ArtifactIdType.class, this.views, Optional.ofNullable(viewsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="EntityDashboards")
@JsonIgnore
public CollectionPage getEntityDashboards() {
return new CollectionPage(contextPath, ArtifactIdType.class, this.entityDashboards, Optional.ofNullable(entityDashboardsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="EntityDashboards")
@JsonIgnore
public CollectionPage getEntityDashboards(HttpRequestOptions options) {
return new CollectionPage(contextPath, ArtifactIdType.class, this.entityDashboards, Optional.ofNullable(entityDashboardsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String entityId;
private List chartIds;
private String chartIdsNextLink;
private List forms;
private String formsNextLink;
private List views;
private String viewsNextLink;
private List entityDashboards;
private String entityDashboardsNextLink;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder entityId(String entityId) {
this.entityId = entityId;
this.changedFields = changedFields.add("EntityId");
return this;
}
public Builder chartIds(List chartIds) {
this.chartIds = chartIds;
this.changedFields = changedFields.add("ChartIds");
return this;
}
public Builder chartIds(String... chartIds) {
return chartIds(Arrays.asList(chartIds));
}
public Builder chartIdsNextLink(String chartIdsNextLink) {
this.chartIdsNextLink = chartIdsNextLink;
this.changedFields = changedFields.add("ChartIds");
return this;
}
public Builder forms(List forms) {
this.forms = forms;
this.changedFields = changedFields.add("Forms");
return this;
}
public Builder forms(ArtifactIdType... forms) {
return forms(Arrays.asList(forms));
}
public Builder formsNextLink(String formsNextLink) {
this.formsNextLink = formsNextLink;
this.changedFields = changedFields.add("Forms");
return this;
}
public Builder views(List views) {
this.views = views;
this.changedFields = changedFields.add("Views");
return this;
}
public Builder views(ArtifactIdType... views) {
return views(Arrays.asList(views));
}
public Builder viewsNextLink(String viewsNextLink) {
this.viewsNextLink = viewsNextLink;
this.changedFields = changedFields.add("Views");
return this;
}
public Builder entityDashboards(List entityDashboards) {
this.entityDashboards = entityDashboards;
this.changedFields = changedFields.add("EntityDashboards");
return this;
}
public Builder entityDashboards(ArtifactIdType... entityDashboards) {
return entityDashboards(Arrays.asList(entityDashboards));
}
public Builder entityDashboardsNextLink(String entityDashboardsNextLink) {
this.entityDashboardsNextLink = entityDashboardsNextLink;
this.changedFields = changedFields.add("EntityDashboards");
return this;
}
public AppEntityInfo build() {
AppEntityInfo _x = new AppEntityInfo();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.AppEntityInfo";
_x.entityId = entityId;
_x.chartIds = chartIds;
_x.chartIdsNextLink = chartIdsNextLink;
_x.forms = forms;
_x.formsNextLink = formsNextLink;
_x.views = views;
_x.viewsNextLink = viewsNextLink;
_x.entityDashboards = entityDashboards;
_x.entityDashboardsNextLink = entityDashboardsNextLink;
return _x;
}
}
private AppEntityInfo _copy() {
AppEntityInfo _x = new AppEntityInfo();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.entityId = entityId;
_x.chartIds = chartIds;
_x.forms = forms;
_x.views = views;
_x.entityDashboards = entityDashboards;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AppEntityInfo[");
b.append("EntityId=");
b.append(this.entityId);
b.append(", ");
b.append("ChartIds=");
b.append(this.chartIds);
b.append(", ");
b.append("Forms=");
b.append(this.forms);
b.append(", ");
b.append("Views=");
b.append(this.views);
b.append(", ");
b.append("EntityDashboards=");
b.append(this.entityDashboards);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy