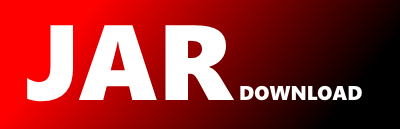
microsoft.dynamics.crm.complex.AppModuleDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"AppModuleId",
"AppDisplayName",
"AppUniqueName",
"AppVersion",
"Description",
"IconWebResource",
"WelcomePageWebResource",
"CreatedBy",
"CreatedOn",
"ModifiedBy",
"ModifiedOn",
"AppUri",
"IsDefault",
"ClientType",
"NavigationType",
"OptimizedFor",
"PublisherUniqueName",
"PublisherName",
"PublishedOn",
"MetadataVersionNumber",
"IsFirstPartyApplication",
"CanvasApps"})
@JsonInclude(Include.NON_NULL)
public class AppModuleDetails implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("AppModuleId")
protected String appModuleId;
@JsonProperty("AppDisplayName")
protected LocalizedLabelDetails appDisplayName;
@JsonProperty("AppUniqueName")
protected String appUniqueName;
@JsonProperty("AppVersion")
protected String appVersion;
@JsonProperty("Description")
protected LocalizedLabelDetails description;
@JsonProperty("IconWebResource")
protected WebResourceDetails iconWebResource;
@JsonProperty("WelcomePageWebResource")
protected WebResourceDetails welcomePageWebResource;
@JsonProperty("CreatedBy")
protected UserDetails createdBy;
@JsonProperty("CreatedOn")
protected OffsetDateTime createdOn;
@JsonProperty("ModifiedBy")
protected UserDetails modifiedBy;
@JsonProperty("ModifiedOn")
protected OffsetDateTime modifiedOn;
@JsonProperty("AppUri")
protected String appUri;
@JsonProperty("IsDefault")
protected Boolean isDefault;
@JsonProperty("ClientType")
protected Integer clientType;
@JsonProperty("NavigationType")
protected Integer navigationType;
@JsonProperty("OptimizedFor")
protected String optimizedFor;
@JsonProperty("PublisherUniqueName")
protected String publisherUniqueName;
@JsonProperty("PublisherName")
protected String publisherName;
@JsonProperty("PublishedOn")
protected OffsetDateTime publishedOn;
@JsonProperty("MetadataVersionNumber")
protected Long metadataVersionNumber;
@JsonProperty("IsFirstPartyApplication")
protected Boolean isFirstPartyApplication;
@JsonProperty("CanvasApps")
protected List canvasApps;
@JsonProperty("CanvasApps@nextLink")
protected String canvasAppsNextLink;
protected AppModuleDetails() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.AppModuleDetails";
}
@Property(name="AppModuleId")
@JsonIgnore
public Optional getAppModuleId() {
return Optional.ofNullable(appModuleId);
}
public AppModuleDetails withAppModuleId(String appModuleId) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.appModuleId = appModuleId;
return _x;
}
@Property(name="AppDisplayName")
@JsonIgnore
public Optional getAppDisplayName() {
return Optional.ofNullable(appDisplayName);
}
public AppModuleDetails withAppDisplayName(LocalizedLabelDetails appDisplayName) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.appDisplayName = appDisplayName;
return _x;
}
@Property(name="AppUniqueName")
@JsonIgnore
public Optional getAppUniqueName() {
return Optional.ofNullable(appUniqueName);
}
public AppModuleDetails withAppUniqueName(String appUniqueName) {
Checks.checkIsAscii(appUniqueName);
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.appUniqueName = appUniqueName;
return _x;
}
@Property(name="AppVersion")
@JsonIgnore
public Optional getAppVersion() {
return Optional.ofNullable(appVersion);
}
public AppModuleDetails withAppVersion(String appVersion) {
Checks.checkIsAscii(appVersion);
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.appVersion = appVersion;
return _x;
}
@Property(name="Description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public AppModuleDetails withDescription(LocalizedLabelDetails description) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.description = description;
return _x;
}
@Property(name="IconWebResource")
@JsonIgnore
public Optional getIconWebResource() {
return Optional.ofNullable(iconWebResource);
}
public AppModuleDetails withIconWebResource(WebResourceDetails iconWebResource) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.iconWebResource = iconWebResource;
return _x;
}
@Property(name="WelcomePageWebResource")
@JsonIgnore
public Optional getWelcomePageWebResource() {
return Optional.ofNullable(welcomePageWebResource);
}
public AppModuleDetails withWelcomePageWebResource(WebResourceDetails welcomePageWebResource) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.welcomePageWebResource = welcomePageWebResource;
return _x;
}
@Property(name="CreatedBy")
@JsonIgnore
public Optional getCreatedBy() {
return Optional.ofNullable(createdBy);
}
public AppModuleDetails withCreatedBy(UserDetails createdBy) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.createdBy = createdBy;
return _x;
}
@Property(name="CreatedOn")
@JsonIgnore
public Optional getCreatedOn() {
return Optional.ofNullable(createdOn);
}
public AppModuleDetails withCreatedOn(OffsetDateTime createdOn) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.createdOn = createdOn;
return _x;
}
@Property(name="ModifiedBy")
@JsonIgnore
public Optional getModifiedBy() {
return Optional.ofNullable(modifiedBy);
}
public AppModuleDetails withModifiedBy(UserDetails modifiedBy) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.modifiedBy = modifiedBy;
return _x;
}
@Property(name="ModifiedOn")
@JsonIgnore
public Optional getModifiedOn() {
return Optional.ofNullable(modifiedOn);
}
public AppModuleDetails withModifiedOn(OffsetDateTime modifiedOn) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.modifiedOn = modifiedOn;
return _x;
}
@Property(name="AppUri")
@JsonIgnore
public Optional getAppUri() {
return Optional.ofNullable(appUri);
}
public AppModuleDetails withAppUri(String appUri) {
Checks.checkIsAscii(appUri);
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.appUri = appUri;
return _x;
}
@Property(name="IsDefault")
@JsonIgnore
public Optional getIsDefault() {
return Optional.ofNullable(isDefault);
}
public AppModuleDetails withIsDefault(Boolean isDefault) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.isDefault = isDefault;
return _x;
}
@Property(name="ClientType")
@JsonIgnore
public Optional getClientType() {
return Optional.ofNullable(clientType);
}
public AppModuleDetails withClientType(Integer clientType) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.clientType = clientType;
return _x;
}
@Property(name="NavigationType")
@JsonIgnore
public Optional getNavigationType() {
return Optional.ofNullable(navigationType);
}
public AppModuleDetails withNavigationType(Integer navigationType) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.navigationType = navigationType;
return _x;
}
@Property(name="OptimizedFor")
@JsonIgnore
public Optional getOptimizedFor() {
return Optional.ofNullable(optimizedFor);
}
public AppModuleDetails withOptimizedFor(String optimizedFor) {
Checks.checkIsAscii(optimizedFor);
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.optimizedFor = optimizedFor;
return _x;
}
@Property(name="PublisherUniqueName")
@JsonIgnore
public Optional getPublisherUniqueName() {
return Optional.ofNullable(publisherUniqueName);
}
public AppModuleDetails withPublisherUniqueName(String publisherUniqueName) {
Checks.checkIsAscii(publisherUniqueName);
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.publisherUniqueName = publisherUniqueName;
return _x;
}
@Property(name="PublisherName")
@JsonIgnore
public Optional getPublisherName() {
return Optional.ofNullable(publisherName);
}
public AppModuleDetails withPublisherName(String publisherName) {
Checks.checkIsAscii(publisherName);
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.publisherName = publisherName;
return _x;
}
@Property(name="PublishedOn")
@JsonIgnore
public Optional getPublishedOn() {
return Optional.ofNullable(publishedOn);
}
public AppModuleDetails withPublishedOn(OffsetDateTime publishedOn) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.publishedOn = publishedOn;
return _x;
}
@Property(name="MetadataVersionNumber")
@JsonIgnore
public Optional getMetadataVersionNumber() {
return Optional.ofNullable(metadataVersionNumber);
}
public AppModuleDetails withMetadataVersionNumber(Long metadataVersionNumber) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.metadataVersionNumber = metadataVersionNumber;
return _x;
}
@Property(name="IsFirstPartyApplication")
@JsonIgnore
public Optional getIsFirstPartyApplication() {
return Optional.ofNullable(isFirstPartyApplication);
}
public AppModuleDetails withIsFirstPartyApplication(Boolean isFirstPartyApplication) {
AppModuleDetails _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleDetails");
_x.isFirstPartyApplication = isFirstPartyApplication;
return _x;
}
@Property(name="CanvasApps")
@JsonIgnore
public CollectionPage getCanvasApps() {
return new CollectionPage(contextPath, CanvasAppsDetails.class, this.canvasApps, Optional.ofNullable(canvasAppsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="CanvasApps")
@JsonIgnore
public CollectionPage getCanvasApps(HttpRequestOptions options) {
return new CollectionPage(contextPath, CanvasAppsDetails.class, this.canvasApps, Optional.ofNullable(canvasAppsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String appModuleId;
private LocalizedLabelDetails appDisplayName;
private String appUniqueName;
private String appVersion;
private LocalizedLabelDetails description;
private WebResourceDetails iconWebResource;
private WebResourceDetails welcomePageWebResource;
private UserDetails createdBy;
private OffsetDateTime createdOn;
private UserDetails modifiedBy;
private OffsetDateTime modifiedOn;
private String appUri;
private Boolean isDefault;
private Integer clientType;
private Integer navigationType;
private String optimizedFor;
private String publisherUniqueName;
private String publisherName;
private OffsetDateTime publishedOn;
private Long metadataVersionNumber;
private Boolean isFirstPartyApplication;
private List canvasApps;
private String canvasAppsNextLink;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder appModuleId(String appModuleId) {
this.appModuleId = appModuleId;
this.changedFields = changedFields.add("AppModuleId");
return this;
}
public Builder appDisplayName(LocalizedLabelDetails appDisplayName) {
this.appDisplayName = appDisplayName;
this.changedFields = changedFields.add("AppDisplayName");
return this;
}
public Builder appUniqueName(String appUniqueName) {
this.appUniqueName = appUniqueName;
this.changedFields = changedFields.add("AppUniqueName");
return this;
}
public Builder appVersion(String appVersion) {
this.appVersion = appVersion;
this.changedFields = changedFields.add("AppVersion");
return this;
}
public Builder description(LocalizedLabelDetails description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder iconWebResource(WebResourceDetails iconWebResource) {
this.iconWebResource = iconWebResource;
this.changedFields = changedFields.add("IconWebResource");
return this;
}
public Builder welcomePageWebResource(WebResourceDetails welcomePageWebResource) {
this.welcomePageWebResource = welcomePageWebResource;
this.changedFields = changedFields.add("WelcomePageWebResource");
return this;
}
public Builder createdBy(UserDetails createdBy) {
this.createdBy = createdBy;
this.changedFields = changedFields.add("CreatedBy");
return this;
}
public Builder createdOn(OffsetDateTime createdOn) {
this.createdOn = createdOn;
this.changedFields = changedFields.add("CreatedOn");
return this;
}
public Builder modifiedBy(UserDetails modifiedBy) {
this.modifiedBy = modifiedBy;
this.changedFields = changedFields.add("ModifiedBy");
return this;
}
public Builder modifiedOn(OffsetDateTime modifiedOn) {
this.modifiedOn = modifiedOn;
this.changedFields = changedFields.add("ModifiedOn");
return this;
}
public Builder appUri(String appUri) {
this.appUri = appUri;
this.changedFields = changedFields.add("AppUri");
return this;
}
public Builder isDefault(Boolean isDefault) {
this.isDefault = isDefault;
this.changedFields = changedFields.add("IsDefault");
return this;
}
public Builder clientType(Integer clientType) {
this.clientType = clientType;
this.changedFields = changedFields.add("ClientType");
return this;
}
public Builder navigationType(Integer navigationType) {
this.navigationType = navigationType;
this.changedFields = changedFields.add("NavigationType");
return this;
}
public Builder optimizedFor(String optimizedFor) {
this.optimizedFor = optimizedFor;
this.changedFields = changedFields.add("OptimizedFor");
return this;
}
public Builder publisherUniqueName(String publisherUniqueName) {
this.publisherUniqueName = publisherUniqueName;
this.changedFields = changedFields.add("PublisherUniqueName");
return this;
}
public Builder publisherName(String publisherName) {
this.publisherName = publisherName;
this.changedFields = changedFields.add("PublisherName");
return this;
}
public Builder publishedOn(OffsetDateTime publishedOn) {
this.publishedOn = publishedOn;
this.changedFields = changedFields.add("PublishedOn");
return this;
}
public Builder metadataVersionNumber(Long metadataVersionNumber) {
this.metadataVersionNumber = metadataVersionNumber;
this.changedFields = changedFields.add("MetadataVersionNumber");
return this;
}
public Builder isFirstPartyApplication(Boolean isFirstPartyApplication) {
this.isFirstPartyApplication = isFirstPartyApplication;
this.changedFields = changedFields.add("IsFirstPartyApplication");
return this;
}
public Builder canvasApps(List canvasApps) {
this.canvasApps = canvasApps;
this.changedFields = changedFields.add("CanvasApps");
return this;
}
public Builder canvasApps(CanvasAppsDetails... canvasApps) {
return canvasApps(Arrays.asList(canvasApps));
}
public Builder canvasAppsNextLink(String canvasAppsNextLink) {
this.canvasAppsNextLink = canvasAppsNextLink;
this.changedFields = changedFields.add("CanvasApps");
return this;
}
public AppModuleDetails build() {
AppModuleDetails _x = new AppModuleDetails();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.AppModuleDetails";
_x.appModuleId = appModuleId;
_x.appDisplayName = appDisplayName;
_x.appUniqueName = appUniqueName;
_x.appVersion = appVersion;
_x.description = description;
_x.iconWebResource = iconWebResource;
_x.welcomePageWebResource = welcomePageWebResource;
_x.createdBy = createdBy;
_x.createdOn = createdOn;
_x.modifiedBy = modifiedBy;
_x.modifiedOn = modifiedOn;
_x.appUri = appUri;
_x.isDefault = isDefault;
_x.clientType = clientType;
_x.navigationType = navigationType;
_x.optimizedFor = optimizedFor;
_x.publisherUniqueName = publisherUniqueName;
_x.publisherName = publisherName;
_x.publishedOn = publishedOn;
_x.metadataVersionNumber = metadataVersionNumber;
_x.isFirstPartyApplication = isFirstPartyApplication;
_x.canvasApps = canvasApps;
_x.canvasAppsNextLink = canvasAppsNextLink;
return _x;
}
}
private AppModuleDetails _copy() {
AppModuleDetails _x = new AppModuleDetails();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.appModuleId = appModuleId;
_x.appDisplayName = appDisplayName;
_x.appUniqueName = appUniqueName;
_x.appVersion = appVersion;
_x.description = description;
_x.iconWebResource = iconWebResource;
_x.welcomePageWebResource = welcomePageWebResource;
_x.createdBy = createdBy;
_x.createdOn = createdOn;
_x.modifiedBy = modifiedBy;
_x.modifiedOn = modifiedOn;
_x.appUri = appUri;
_x.isDefault = isDefault;
_x.clientType = clientType;
_x.navigationType = navigationType;
_x.optimizedFor = optimizedFor;
_x.publisherUniqueName = publisherUniqueName;
_x.publisherName = publisherName;
_x.publishedOn = publishedOn;
_x.metadataVersionNumber = metadataVersionNumber;
_x.isFirstPartyApplication = isFirstPartyApplication;
_x.canvasApps = canvasApps;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AppModuleDetails[");
b.append("AppModuleId=");
b.append(this.appModuleId);
b.append(", ");
b.append("AppDisplayName=");
b.append(this.appDisplayName);
b.append(", ");
b.append("AppUniqueName=");
b.append(this.appUniqueName);
b.append(", ");
b.append("AppVersion=");
b.append(this.appVersion);
b.append(", ");
b.append("Description=");
b.append(this.description);
b.append(", ");
b.append("IconWebResource=");
b.append(this.iconWebResource);
b.append(", ");
b.append("WelcomePageWebResource=");
b.append(this.welcomePageWebResource);
b.append(", ");
b.append("CreatedBy=");
b.append(this.createdBy);
b.append(", ");
b.append("CreatedOn=");
b.append(this.createdOn);
b.append(", ");
b.append("ModifiedBy=");
b.append(this.modifiedBy);
b.append(", ");
b.append("ModifiedOn=");
b.append(this.modifiedOn);
b.append(", ");
b.append("AppUri=");
b.append(this.appUri);
b.append(", ");
b.append("IsDefault=");
b.append(this.isDefault);
b.append(", ");
b.append("ClientType=");
b.append(this.clientType);
b.append(", ");
b.append("NavigationType=");
b.append(this.navigationType);
b.append(", ");
b.append("OptimizedFor=");
b.append(this.optimizedFor);
b.append(", ");
b.append("PublisherUniqueName=");
b.append(this.publisherUniqueName);
b.append(", ");
b.append("PublisherName=");
b.append(this.publisherName);
b.append(", ");
b.append("PublishedOn=");
b.append(this.publishedOn);
b.append(", ");
b.append("MetadataVersionNumber=");
b.append(this.metadataVersionNumber);
b.append(", ");
b.append("IsFirstPartyApplication=");
b.append(this.isFirstPartyApplication);
b.append(", ");
b.append("CanvasApps=");
b.append(this.canvasApps);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy