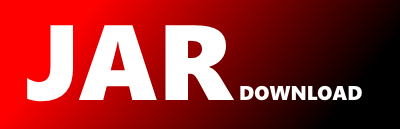
microsoft.dynamics.crm.complex.AppModuleInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"AppId",
"AppDisplayName",
"PublisherName",
"PublishedOn",
"AppUniqueName",
"AppVersion",
"Description",
"WebResourceUri",
"WelcomePageUri",
"CreatedOn",
"CreatedBy",
"ModifiedOn",
"ModifiedBy",
"AppUri",
"IsDefault",
"ClientType",
"NavigationType",
"OptimizedFor",
"AppSettingDetails"})
@JsonInclude(Include.NON_NULL)
public class AppModuleInfo implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("AppId")
protected String appId;
@JsonProperty("AppDisplayName")
protected String appDisplayName;
@JsonProperty("PublisherName")
protected String publisherName;
@JsonProperty("PublishedOn")
protected String publishedOn;
@JsonProperty("AppUniqueName")
protected String appUniqueName;
@JsonProperty("AppVersion")
protected String appVersion;
@JsonProperty("Description")
protected String description;
@JsonProperty("WebResourceUri")
protected String webResourceUri;
@JsonProperty("WelcomePageUri")
protected String welcomePageUri;
@JsonProperty("CreatedOn")
protected String createdOn;
@JsonProperty("CreatedBy")
protected String createdBy;
@JsonProperty("ModifiedOn")
protected String modifiedOn;
@JsonProperty("ModifiedBy")
protected String modifiedBy;
@JsonProperty("AppUri")
protected String appUri;
@JsonProperty("IsDefault")
protected Boolean isDefault;
@JsonProperty("ClientType")
protected Integer clientType;
@JsonProperty("NavigationType")
protected Integer navigationType;
@JsonProperty("OptimizedFor")
protected String optimizedFor;
@JsonProperty("AppSettingDetails")
protected List appSettingDetails;
@JsonProperty("AppSettingDetails@nextLink")
protected String appSettingDetailsNextLink;
protected AppModuleInfo() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.AppModuleInfo";
}
@Property(name="AppId")
@JsonIgnore
public Optional getAppId() {
return Optional.ofNullable(appId);
}
public AppModuleInfo withAppId(String appId) {
Checks.checkIsAscii(appId);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.appId = appId;
return _x;
}
@Property(name="AppDisplayName")
@JsonIgnore
public Optional getAppDisplayName() {
return Optional.ofNullable(appDisplayName);
}
public AppModuleInfo withAppDisplayName(String appDisplayName) {
Checks.checkIsAscii(appDisplayName);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.appDisplayName = appDisplayName;
return _x;
}
@Property(name="PublisherName")
@JsonIgnore
public Optional getPublisherName() {
return Optional.ofNullable(publisherName);
}
public AppModuleInfo withPublisherName(String publisherName) {
Checks.checkIsAscii(publisherName);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.publisherName = publisherName;
return _x;
}
@Property(name="PublishedOn")
@JsonIgnore
public Optional getPublishedOn() {
return Optional.ofNullable(publishedOn);
}
public AppModuleInfo withPublishedOn(String publishedOn) {
Checks.checkIsAscii(publishedOn);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.publishedOn = publishedOn;
return _x;
}
@Property(name="AppUniqueName")
@JsonIgnore
public Optional getAppUniqueName() {
return Optional.ofNullable(appUniqueName);
}
public AppModuleInfo withAppUniqueName(String appUniqueName) {
Checks.checkIsAscii(appUniqueName);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.appUniqueName = appUniqueName;
return _x;
}
@Property(name="AppVersion")
@JsonIgnore
public Optional getAppVersion() {
return Optional.ofNullable(appVersion);
}
public AppModuleInfo withAppVersion(String appVersion) {
Checks.checkIsAscii(appVersion);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.appVersion = appVersion;
return _x;
}
@Property(name="Description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public AppModuleInfo withDescription(String description) {
Checks.checkIsAscii(description);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.description = description;
return _x;
}
@Property(name="WebResourceUri")
@JsonIgnore
public Optional getWebResourceUri() {
return Optional.ofNullable(webResourceUri);
}
public AppModuleInfo withWebResourceUri(String webResourceUri) {
Checks.checkIsAscii(webResourceUri);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.webResourceUri = webResourceUri;
return _x;
}
@Property(name="WelcomePageUri")
@JsonIgnore
public Optional getWelcomePageUri() {
return Optional.ofNullable(welcomePageUri);
}
public AppModuleInfo withWelcomePageUri(String welcomePageUri) {
Checks.checkIsAscii(welcomePageUri);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.welcomePageUri = welcomePageUri;
return _x;
}
@Property(name="CreatedOn")
@JsonIgnore
public Optional getCreatedOn() {
return Optional.ofNullable(createdOn);
}
public AppModuleInfo withCreatedOn(String createdOn) {
Checks.checkIsAscii(createdOn);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.createdOn = createdOn;
return _x;
}
@Property(name="CreatedBy")
@JsonIgnore
public Optional getCreatedBy() {
return Optional.ofNullable(createdBy);
}
public AppModuleInfo withCreatedBy(String createdBy) {
Checks.checkIsAscii(createdBy);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.createdBy = createdBy;
return _x;
}
@Property(name="ModifiedOn")
@JsonIgnore
public Optional getModifiedOn() {
return Optional.ofNullable(modifiedOn);
}
public AppModuleInfo withModifiedOn(String modifiedOn) {
Checks.checkIsAscii(modifiedOn);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.modifiedOn = modifiedOn;
return _x;
}
@Property(name="ModifiedBy")
@JsonIgnore
public Optional getModifiedBy() {
return Optional.ofNullable(modifiedBy);
}
public AppModuleInfo withModifiedBy(String modifiedBy) {
Checks.checkIsAscii(modifiedBy);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.modifiedBy = modifiedBy;
return _x;
}
@Property(name="AppUri")
@JsonIgnore
public Optional getAppUri() {
return Optional.ofNullable(appUri);
}
public AppModuleInfo withAppUri(String appUri) {
Checks.checkIsAscii(appUri);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.appUri = appUri;
return _x;
}
@Property(name="IsDefault")
@JsonIgnore
public Optional getIsDefault() {
return Optional.ofNullable(isDefault);
}
public AppModuleInfo withIsDefault(Boolean isDefault) {
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.isDefault = isDefault;
return _x;
}
@Property(name="ClientType")
@JsonIgnore
public Optional getClientType() {
return Optional.ofNullable(clientType);
}
public AppModuleInfo withClientType(Integer clientType) {
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.clientType = clientType;
return _x;
}
@Property(name="NavigationType")
@JsonIgnore
public Optional getNavigationType() {
return Optional.ofNullable(navigationType);
}
public AppModuleInfo withNavigationType(Integer navigationType) {
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.navigationType = navigationType;
return _x;
}
@Property(name="OptimizedFor")
@JsonIgnore
public Optional getOptimizedFor() {
return Optional.ofNullable(optimizedFor);
}
public AppModuleInfo withOptimizedFor(String optimizedFor) {
Checks.checkIsAscii(optimizedFor);
AppModuleInfo _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppModuleInfo");
_x.optimizedFor = optimizedFor;
return _x;
}
@Property(name="AppSettingDetails")
@JsonIgnore
public CollectionPage getAppSettingDetails() {
return new CollectionPage(contextPath, AppSettingDetails.class, this.appSettingDetails, Optional.ofNullable(appSettingDetailsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="AppSettingDetails")
@JsonIgnore
public CollectionPage getAppSettingDetails(HttpRequestOptions options) {
return new CollectionPage(contextPath, AppSettingDetails.class, this.appSettingDetails, Optional.ofNullable(appSettingDetailsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String appId;
private String appDisplayName;
private String publisherName;
private String publishedOn;
private String appUniqueName;
private String appVersion;
private String description;
private String webResourceUri;
private String welcomePageUri;
private String createdOn;
private String createdBy;
private String modifiedOn;
private String modifiedBy;
private String appUri;
private Boolean isDefault;
private Integer clientType;
private Integer navigationType;
private String optimizedFor;
private List appSettingDetails;
private String appSettingDetailsNextLink;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder appId(String appId) {
this.appId = appId;
this.changedFields = changedFields.add("AppId");
return this;
}
public Builder appDisplayName(String appDisplayName) {
this.appDisplayName = appDisplayName;
this.changedFields = changedFields.add("AppDisplayName");
return this;
}
public Builder publisherName(String publisherName) {
this.publisherName = publisherName;
this.changedFields = changedFields.add("PublisherName");
return this;
}
public Builder publishedOn(String publishedOn) {
this.publishedOn = publishedOn;
this.changedFields = changedFields.add("PublishedOn");
return this;
}
public Builder appUniqueName(String appUniqueName) {
this.appUniqueName = appUniqueName;
this.changedFields = changedFields.add("AppUniqueName");
return this;
}
public Builder appVersion(String appVersion) {
this.appVersion = appVersion;
this.changedFields = changedFields.add("AppVersion");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder webResourceUri(String webResourceUri) {
this.webResourceUri = webResourceUri;
this.changedFields = changedFields.add("WebResourceUri");
return this;
}
public Builder welcomePageUri(String welcomePageUri) {
this.welcomePageUri = welcomePageUri;
this.changedFields = changedFields.add("WelcomePageUri");
return this;
}
public Builder createdOn(String createdOn) {
this.createdOn = createdOn;
this.changedFields = changedFields.add("CreatedOn");
return this;
}
public Builder createdBy(String createdBy) {
this.createdBy = createdBy;
this.changedFields = changedFields.add("CreatedBy");
return this;
}
public Builder modifiedOn(String modifiedOn) {
this.modifiedOn = modifiedOn;
this.changedFields = changedFields.add("ModifiedOn");
return this;
}
public Builder modifiedBy(String modifiedBy) {
this.modifiedBy = modifiedBy;
this.changedFields = changedFields.add("ModifiedBy");
return this;
}
public Builder appUri(String appUri) {
this.appUri = appUri;
this.changedFields = changedFields.add("AppUri");
return this;
}
public Builder isDefault(Boolean isDefault) {
this.isDefault = isDefault;
this.changedFields = changedFields.add("IsDefault");
return this;
}
public Builder clientType(Integer clientType) {
this.clientType = clientType;
this.changedFields = changedFields.add("ClientType");
return this;
}
public Builder navigationType(Integer navigationType) {
this.navigationType = navigationType;
this.changedFields = changedFields.add("NavigationType");
return this;
}
public Builder optimizedFor(String optimizedFor) {
this.optimizedFor = optimizedFor;
this.changedFields = changedFields.add("OptimizedFor");
return this;
}
public Builder appSettingDetails(List appSettingDetails) {
this.appSettingDetails = appSettingDetails;
this.changedFields = changedFields.add("AppSettingDetails");
return this;
}
public Builder appSettingDetails(AppSettingDetails... appSettingDetails) {
return appSettingDetails(Arrays.asList(appSettingDetails));
}
public Builder appSettingDetailsNextLink(String appSettingDetailsNextLink) {
this.appSettingDetailsNextLink = appSettingDetailsNextLink;
this.changedFields = changedFields.add("AppSettingDetails");
return this;
}
public AppModuleInfo build() {
AppModuleInfo _x = new AppModuleInfo();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.AppModuleInfo";
_x.appId = appId;
_x.appDisplayName = appDisplayName;
_x.publisherName = publisherName;
_x.publishedOn = publishedOn;
_x.appUniqueName = appUniqueName;
_x.appVersion = appVersion;
_x.description = description;
_x.webResourceUri = webResourceUri;
_x.welcomePageUri = welcomePageUri;
_x.createdOn = createdOn;
_x.createdBy = createdBy;
_x.modifiedOn = modifiedOn;
_x.modifiedBy = modifiedBy;
_x.appUri = appUri;
_x.isDefault = isDefault;
_x.clientType = clientType;
_x.navigationType = navigationType;
_x.optimizedFor = optimizedFor;
_x.appSettingDetails = appSettingDetails;
_x.appSettingDetailsNextLink = appSettingDetailsNextLink;
return _x;
}
}
private AppModuleInfo _copy() {
AppModuleInfo _x = new AppModuleInfo();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.appId = appId;
_x.appDisplayName = appDisplayName;
_x.publisherName = publisherName;
_x.publishedOn = publishedOn;
_x.appUniqueName = appUniqueName;
_x.appVersion = appVersion;
_x.description = description;
_x.webResourceUri = webResourceUri;
_x.welcomePageUri = welcomePageUri;
_x.createdOn = createdOn;
_x.createdBy = createdBy;
_x.modifiedOn = modifiedOn;
_x.modifiedBy = modifiedBy;
_x.appUri = appUri;
_x.isDefault = isDefault;
_x.clientType = clientType;
_x.navigationType = navigationType;
_x.optimizedFor = optimizedFor;
_x.appSettingDetails = appSettingDetails;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AppModuleInfo[");
b.append("AppId=");
b.append(this.appId);
b.append(", ");
b.append("AppDisplayName=");
b.append(this.appDisplayName);
b.append(", ");
b.append("PublisherName=");
b.append(this.publisherName);
b.append(", ");
b.append("PublishedOn=");
b.append(this.publishedOn);
b.append(", ");
b.append("AppUniqueName=");
b.append(this.appUniqueName);
b.append(", ");
b.append("AppVersion=");
b.append(this.appVersion);
b.append(", ");
b.append("Description=");
b.append(this.description);
b.append(", ");
b.append("WebResourceUri=");
b.append(this.webResourceUri);
b.append(", ");
b.append("WelcomePageUri=");
b.append(this.welcomePageUri);
b.append(", ");
b.append("CreatedOn=");
b.append(this.createdOn);
b.append(", ");
b.append("CreatedBy=");
b.append(this.createdBy);
b.append(", ");
b.append("ModifiedOn=");
b.append(this.modifiedOn);
b.append(", ");
b.append("ModifiedBy=");
b.append(this.modifiedBy);
b.append(", ");
b.append("AppUri=");
b.append(this.appUri);
b.append(", ");
b.append("IsDefault=");
b.append(this.isDefault);
b.append(", ");
b.append("ClientType=");
b.append(this.clientType);
b.append(", ");
b.append("NavigationType=");
b.append(this.navigationType);
b.append(", ");
b.append("OptimizedFor=");
b.append(this.optimizedFor);
b.append(", ");
b.append("AppSettingDetails=");
b.append(this.appSettingDetails);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy