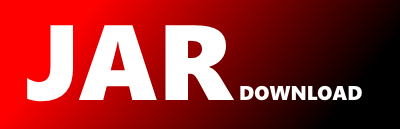
microsoft.dynamics.crm.complex.AppointmentRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.EdmSchemaInfo;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.enums.SearchDirection;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"ServiceId",
"AnchorOffset",
"UserTimeZoneCode",
"RecurrenceDuration",
"RecurrenceTimeZoneCode",
"AppointmentsToIgnore",
"RequiredResources",
"SearchWindowStart",
"SearchWindowEnd",
"SearchRecurrenceStart",
"SearchRecurrenceRule",
"Duration",
"Constraints",
"Objectives",
"Direction",
"NumberOfResults",
"Sites"})
@JsonInclude(Include.NON_NULL)
public class AppointmentRequest implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("ServiceId")
protected String serviceId;
@JsonProperty("AnchorOffset")
protected Integer anchorOffset;
@JsonProperty("UserTimeZoneCode")
protected Integer userTimeZoneCode;
@JsonProperty("RecurrenceDuration")
protected Integer recurrenceDuration;
@JsonProperty("RecurrenceTimeZoneCode")
protected Integer recurrenceTimeZoneCode;
@JsonProperty("AppointmentsToIgnore")
protected List appointmentsToIgnore;
@JsonProperty("AppointmentsToIgnore@nextLink")
protected String appointmentsToIgnoreNextLink;
@JsonProperty("RequiredResources")
protected List requiredResources;
@JsonProperty("RequiredResources@nextLink")
protected String requiredResourcesNextLink;
@JsonProperty("SearchWindowStart")
protected OffsetDateTime searchWindowStart;
@JsonProperty("SearchWindowEnd")
protected OffsetDateTime searchWindowEnd;
@JsonProperty("SearchRecurrenceStart")
protected OffsetDateTime searchRecurrenceStart;
@JsonProperty("SearchRecurrenceRule")
protected String searchRecurrenceRule;
@JsonProperty("Duration")
protected Integer duration;
@JsonProperty("Constraints")
protected List constraints;
@JsonProperty("Constraints@nextLink")
protected String constraintsNextLink;
@JsonProperty("Objectives")
protected List objectives;
@JsonProperty("Objectives@nextLink")
protected String objectivesNextLink;
@JsonProperty("Direction")
protected SearchDirection direction;
@JsonProperty("NumberOfResults")
protected Integer numberOfResults;
@JsonProperty("Sites")
protected List sites;
@JsonProperty("Sites@nextLink")
protected String sitesNextLink;
protected AppointmentRequest() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.AppointmentRequest";
}
@Property(name="ServiceId")
@JsonIgnore
public Optional getServiceId() {
return Optional.ofNullable(serviceId);
}
public AppointmentRequest withServiceId(String serviceId) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.serviceId = serviceId;
return _x;
}
@Property(name="AnchorOffset")
@JsonIgnore
public Optional getAnchorOffset() {
return Optional.ofNullable(anchorOffset);
}
public AppointmentRequest withAnchorOffset(Integer anchorOffset) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.anchorOffset = anchorOffset;
return _x;
}
@Property(name="UserTimeZoneCode")
@JsonIgnore
public Optional getUserTimeZoneCode() {
return Optional.ofNullable(userTimeZoneCode);
}
public AppointmentRequest withUserTimeZoneCode(Integer userTimeZoneCode) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.userTimeZoneCode = userTimeZoneCode;
return _x;
}
@Property(name="RecurrenceDuration")
@JsonIgnore
public Optional getRecurrenceDuration() {
return Optional.ofNullable(recurrenceDuration);
}
public AppointmentRequest withRecurrenceDuration(Integer recurrenceDuration) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.recurrenceDuration = recurrenceDuration;
return _x;
}
@Property(name="RecurrenceTimeZoneCode")
@JsonIgnore
public Optional getRecurrenceTimeZoneCode() {
return Optional.ofNullable(recurrenceTimeZoneCode);
}
public AppointmentRequest withRecurrenceTimeZoneCode(Integer recurrenceTimeZoneCode) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.recurrenceTimeZoneCode = recurrenceTimeZoneCode;
return _x;
}
@Property(name="AppointmentsToIgnore")
@JsonIgnore
public CollectionPage getAppointmentsToIgnore() {
return new CollectionPage(contextPath, AppointmentsToIgnore.class, this.appointmentsToIgnore, Optional.ofNullable(appointmentsToIgnoreNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="AppointmentsToIgnore")
@JsonIgnore
public CollectionPage getAppointmentsToIgnore(HttpRequestOptions options) {
return new CollectionPage(contextPath, AppointmentsToIgnore.class, this.appointmentsToIgnore, Optional.ofNullable(appointmentsToIgnoreNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="RequiredResources")
@JsonIgnore
public CollectionPage getRequiredResources() {
return new CollectionPage(contextPath, RequiredResource.class, this.requiredResources, Optional.ofNullable(requiredResourcesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="RequiredResources")
@JsonIgnore
public CollectionPage getRequiredResources(HttpRequestOptions options) {
return new CollectionPage(contextPath, RequiredResource.class, this.requiredResources, Optional.ofNullable(requiredResourcesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="SearchWindowStart")
@JsonIgnore
public Optional getSearchWindowStart() {
return Optional.ofNullable(searchWindowStart);
}
public AppointmentRequest withSearchWindowStart(OffsetDateTime searchWindowStart) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.searchWindowStart = searchWindowStart;
return _x;
}
@Property(name="SearchWindowEnd")
@JsonIgnore
public Optional getSearchWindowEnd() {
return Optional.ofNullable(searchWindowEnd);
}
public AppointmentRequest withSearchWindowEnd(OffsetDateTime searchWindowEnd) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.searchWindowEnd = searchWindowEnd;
return _x;
}
@Property(name="SearchRecurrenceStart")
@JsonIgnore
public Optional getSearchRecurrenceStart() {
return Optional.ofNullable(searchRecurrenceStart);
}
public AppointmentRequest withSearchRecurrenceStart(OffsetDateTime searchRecurrenceStart) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.searchRecurrenceStart = searchRecurrenceStart;
return _x;
}
@Property(name="SearchRecurrenceRule")
@JsonIgnore
public Optional getSearchRecurrenceRule() {
return Optional.ofNullable(searchRecurrenceRule);
}
public AppointmentRequest withSearchRecurrenceRule(String searchRecurrenceRule) {
Checks.checkIsAscii(searchRecurrenceRule);
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.searchRecurrenceRule = searchRecurrenceRule;
return _x;
}
@Property(name="Duration")
@JsonIgnore
public Optional getDuration() {
return Optional.ofNullable(duration);
}
public AppointmentRequest withDuration(Integer duration) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.duration = duration;
return _x;
}
@Property(name="Constraints")
@JsonIgnore
public CollectionPage getConstraints() {
return new CollectionPage(contextPath, ConstraintRelation.class, this.constraints, Optional.ofNullable(constraintsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Constraints")
@JsonIgnore
public CollectionPage getConstraints(HttpRequestOptions options) {
return new CollectionPage(contextPath, ConstraintRelation.class, this.constraints, Optional.ofNullable(constraintsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="Objectives")
@JsonIgnore
public CollectionPage getObjectives() {
return new CollectionPage(contextPath, ObjectiveRelation.class, this.objectives, Optional.ofNullable(objectivesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Objectives")
@JsonIgnore
public CollectionPage getObjectives(HttpRequestOptions options) {
return new CollectionPage(contextPath, ObjectiveRelation.class, this.objectives, Optional.ofNullable(objectivesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="Direction")
@JsonIgnore
public Optional getDirection() {
return Optional.ofNullable(direction);
}
public AppointmentRequest withDirection(SearchDirection direction) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.direction = direction;
return _x;
}
@Property(name="NumberOfResults")
@JsonIgnore
public Optional getNumberOfResults() {
return Optional.ofNullable(numberOfResults);
}
public AppointmentRequest withNumberOfResults(Integer numberOfResults) {
AppointmentRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AppointmentRequest");
_x.numberOfResults = numberOfResults;
return _x;
}
@Property(name="Sites")
@JsonIgnore
public CollectionPage getSites() {
return new CollectionPage(contextPath, String.class, this.sites, Optional.ofNullable(sitesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Sites")
@JsonIgnore
public CollectionPage getSites(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.sites, Optional.ofNullable(sitesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String serviceId;
private Integer anchorOffset;
private Integer userTimeZoneCode;
private Integer recurrenceDuration;
private Integer recurrenceTimeZoneCode;
private List appointmentsToIgnore;
private String appointmentsToIgnoreNextLink;
private List requiredResources;
private String requiredResourcesNextLink;
private OffsetDateTime searchWindowStart;
private OffsetDateTime searchWindowEnd;
private OffsetDateTime searchRecurrenceStart;
private String searchRecurrenceRule;
private Integer duration;
private List constraints;
private String constraintsNextLink;
private List objectives;
private String objectivesNextLink;
private SearchDirection direction;
private Integer numberOfResults;
private List sites;
private String sitesNextLink;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder serviceId(String serviceId) {
this.serviceId = serviceId;
this.changedFields = changedFields.add("ServiceId");
return this;
}
public Builder anchorOffset(Integer anchorOffset) {
this.anchorOffset = anchorOffset;
this.changedFields = changedFields.add("AnchorOffset");
return this;
}
public Builder userTimeZoneCode(Integer userTimeZoneCode) {
this.userTimeZoneCode = userTimeZoneCode;
this.changedFields = changedFields.add("UserTimeZoneCode");
return this;
}
public Builder recurrenceDuration(Integer recurrenceDuration) {
this.recurrenceDuration = recurrenceDuration;
this.changedFields = changedFields.add("RecurrenceDuration");
return this;
}
public Builder recurrenceTimeZoneCode(Integer recurrenceTimeZoneCode) {
this.recurrenceTimeZoneCode = recurrenceTimeZoneCode;
this.changedFields = changedFields.add("RecurrenceTimeZoneCode");
return this;
}
public Builder appointmentsToIgnore(List appointmentsToIgnore) {
this.appointmentsToIgnore = appointmentsToIgnore;
this.changedFields = changedFields.add("AppointmentsToIgnore");
return this;
}
public Builder appointmentsToIgnore(AppointmentsToIgnore... appointmentsToIgnore) {
return appointmentsToIgnore(Arrays.asList(appointmentsToIgnore));
}
public Builder appointmentsToIgnoreNextLink(String appointmentsToIgnoreNextLink) {
this.appointmentsToIgnoreNextLink = appointmentsToIgnoreNextLink;
this.changedFields = changedFields.add("AppointmentsToIgnore");
return this;
}
public Builder requiredResources(List requiredResources) {
this.requiredResources = requiredResources;
this.changedFields = changedFields.add("RequiredResources");
return this;
}
public Builder requiredResources(RequiredResource... requiredResources) {
return requiredResources(Arrays.asList(requiredResources));
}
public Builder requiredResourcesNextLink(String requiredResourcesNextLink) {
this.requiredResourcesNextLink = requiredResourcesNextLink;
this.changedFields = changedFields.add("RequiredResources");
return this;
}
public Builder searchWindowStart(OffsetDateTime searchWindowStart) {
this.searchWindowStart = searchWindowStart;
this.changedFields = changedFields.add("SearchWindowStart");
return this;
}
public Builder searchWindowEnd(OffsetDateTime searchWindowEnd) {
this.searchWindowEnd = searchWindowEnd;
this.changedFields = changedFields.add("SearchWindowEnd");
return this;
}
public Builder searchRecurrenceStart(OffsetDateTime searchRecurrenceStart) {
this.searchRecurrenceStart = searchRecurrenceStart;
this.changedFields = changedFields.add("SearchRecurrenceStart");
return this;
}
public Builder searchRecurrenceRule(String searchRecurrenceRule) {
this.searchRecurrenceRule = searchRecurrenceRule;
this.changedFields = changedFields.add("SearchRecurrenceRule");
return this;
}
public Builder duration(Integer duration) {
this.duration = duration;
this.changedFields = changedFields.add("Duration");
return this;
}
public Builder constraints(List constraints) {
this.constraints = constraints;
this.changedFields = changedFields.add("Constraints");
return this;
}
public Builder constraints(ConstraintRelation... constraints) {
return constraints(Arrays.asList(constraints));
}
public Builder constraintsNextLink(String constraintsNextLink) {
this.constraintsNextLink = constraintsNextLink;
this.changedFields = changedFields.add("Constraints");
return this;
}
public Builder objectives(List objectives) {
this.objectives = objectives;
this.changedFields = changedFields.add("Objectives");
return this;
}
public Builder objectives(ObjectiveRelation... objectives) {
return objectives(Arrays.asList(objectives));
}
public Builder objectivesNextLink(String objectivesNextLink) {
this.objectivesNextLink = objectivesNextLink;
this.changedFields = changedFields.add("Objectives");
return this;
}
public Builder direction(SearchDirection direction) {
this.direction = direction;
this.changedFields = changedFields.add("Direction");
return this;
}
public Builder numberOfResults(Integer numberOfResults) {
this.numberOfResults = numberOfResults;
this.changedFields = changedFields.add("NumberOfResults");
return this;
}
public Builder sites(List sites) {
this.sites = sites;
this.changedFields = changedFields.add("Sites");
return this;
}
public Builder sites(String... sites) {
return sites(Arrays.asList(sites));
}
public Builder sitesNextLink(String sitesNextLink) {
this.sitesNextLink = sitesNextLink;
this.changedFields = changedFields.add("Sites");
return this;
}
public AppointmentRequest build() {
AppointmentRequest _x = new AppointmentRequest();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.AppointmentRequest";
_x.serviceId = serviceId;
_x.anchorOffset = anchorOffset;
_x.userTimeZoneCode = userTimeZoneCode;
_x.recurrenceDuration = recurrenceDuration;
_x.recurrenceTimeZoneCode = recurrenceTimeZoneCode;
_x.appointmentsToIgnore = appointmentsToIgnore;
_x.appointmentsToIgnoreNextLink = appointmentsToIgnoreNextLink;
_x.requiredResources = requiredResources;
_x.requiredResourcesNextLink = requiredResourcesNextLink;
_x.searchWindowStart = searchWindowStart;
_x.searchWindowEnd = searchWindowEnd;
_x.searchRecurrenceStart = searchRecurrenceStart;
_x.searchRecurrenceRule = searchRecurrenceRule;
_x.duration = duration;
_x.constraints = constraints;
_x.constraintsNextLink = constraintsNextLink;
_x.objectives = objectives;
_x.objectivesNextLink = objectivesNextLink;
_x.direction = direction;
_x.numberOfResults = numberOfResults;
_x.sites = sites;
_x.sitesNextLink = sitesNextLink;
return _x;
}
}
private AppointmentRequest _copy() {
AppointmentRequest _x = new AppointmentRequest();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.serviceId = serviceId;
_x.anchorOffset = anchorOffset;
_x.userTimeZoneCode = userTimeZoneCode;
_x.recurrenceDuration = recurrenceDuration;
_x.recurrenceTimeZoneCode = recurrenceTimeZoneCode;
_x.appointmentsToIgnore = appointmentsToIgnore;
_x.requiredResources = requiredResources;
_x.searchWindowStart = searchWindowStart;
_x.searchWindowEnd = searchWindowEnd;
_x.searchRecurrenceStart = searchRecurrenceStart;
_x.searchRecurrenceRule = searchRecurrenceRule;
_x.duration = duration;
_x.constraints = constraints;
_x.objectives = objectives;
_x.direction = direction;
_x.numberOfResults = numberOfResults;
_x.sites = sites;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AppointmentRequest[");
b.append("ServiceId=");
b.append(this.serviceId);
b.append(", ");
b.append("AnchorOffset=");
b.append(this.anchorOffset);
b.append(", ");
b.append("UserTimeZoneCode=");
b.append(this.userTimeZoneCode);
b.append(", ");
b.append("RecurrenceDuration=");
b.append(this.recurrenceDuration);
b.append(", ");
b.append("RecurrenceTimeZoneCode=");
b.append(this.recurrenceTimeZoneCode);
b.append(", ");
b.append("AppointmentsToIgnore=");
b.append(this.appointmentsToIgnore);
b.append(", ");
b.append("RequiredResources=");
b.append(this.requiredResources);
b.append(", ");
b.append("SearchWindowStart=");
b.append(this.searchWindowStart);
b.append(", ");
b.append("SearchWindowEnd=");
b.append(this.searchWindowEnd);
b.append(", ");
b.append("SearchRecurrenceStart=");
b.append(this.searchRecurrenceStart);
b.append(", ");
b.append("SearchRecurrenceRule=");
b.append(this.searchRecurrenceRule);
b.append(", ");
b.append("Duration=");
b.append(this.duration);
b.append(", ");
b.append("Constraints=");
b.append(this.constraints);
b.append(", ");
b.append("Objectives=");
b.append(this.objectives);
b.append(", ");
b.append("Direction=");
b.append(this.direction);
b.append(", ");
b.append("NumberOfResults=");
b.append(this.numberOfResults);
b.append(", ");
b.append("Sites=");
b.append(this.sites);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy