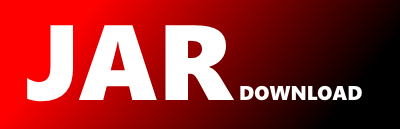
microsoft.dynamics.crm.complex.AttributeMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.EdmSchemaInfo;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"AttributeMappingId",
"MappingName",
"AttributeCrmName",
"AttributeExchangeName",
"SyncDirection",
"DefaultSyncDirection",
"AllowedSyncDirection",
"IsComputed",
"EntityTypeCode",
"ComputedProperties",
"AttributeCrmDisplayName",
"AttributeExchangeDisplayName"})
@JsonInclude(Include.NON_NULL)
public class AttributeMapping implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("AttributeMappingId")
protected String attributeMappingId;
@JsonProperty("MappingName")
protected String mappingName;
@JsonProperty("AttributeCrmName")
protected String attributeCrmName;
@JsonProperty("AttributeExchangeName")
protected String attributeExchangeName;
@JsonProperty("SyncDirection")
protected Integer syncDirection;
@JsonProperty("DefaultSyncDirection")
protected Integer defaultSyncDirection;
@JsonProperty("AllowedSyncDirection")
protected Integer allowedSyncDirection;
@JsonProperty("IsComputed")
protected Boolean isComputed;
@JsonProperty("EntityTypeCode")
protected Integer entityTypeCode;
@JsonProperty("ComputedProperties")
protected List computedProperties;
@JsonProperty("ComputedProperties@nextLink")
protected String computedPropertiesNextLink;
@JsonProperty("AttributeCrmDisplayName")
protected String attributeCrmDisplayName;
@JsonProperty("AttributeExchangeDisplayName")
protected String attributeExchangeDisplayName;
protected AttributeMapping() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.AttributeMapping";
}
@Property(name="AttributeMappingId")
@JsonIgnore
public Optional getAttributeMappingId() {
return Optional.ofNullable(attributeMappingId);
}
public AttributeMapping withAttributeMappingId(String attributeMappingId) {
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.attributeMappingId = attributeMappingId;
return _x;
}
@Property(name="MappingName")
@JsonIgnore
public Optional getMappingName() {
return Optional.ofNullable(mappingName);
}
public AttributeMapping withMappingName(String mappingName) {
Checks.checkIsAscii(mappingName);
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.mappingName = mappingName;
return _x;
}
@Property(name="AttributeCrmName")
@JsonIgnore
public Optional getAttributeCrmName() {
return Optional.ofNullable(attributeCrmName);
}
public AttributeMapping withAttributeCrmName(String attributeCrmName) {
Checks.checkIsAscii(attributeCrmName);
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.attributeCrmName = attributeCrmName;
return _x;
}
@Property(name="AttributeExchangeName")
@JsonIgnore
public Optional getAttributeExchangeName() {
return Optional.ofNullable(attributeExchangeName);
}
public AttributeMapping withAttributeExchangeName(String attributeExchangeName) {
Checks.checkIsAscii(attributeExchangeName);
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.attributeExchangeName = attributeExchangeName;
return _x;
}
@Property(name="SyncDirection")
@JsonIgnore
public Optional getSyncDirection() {
return Optional.ofNullable(syncDirection);
}
public AttributeMapping withSyncDirection(Integer syncDirection) {
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.syncDirection = syncDirection;
return _x;
}
@Property(name="DefaultSyncDirection")
@JsonIgnore
public Optional getDefaultSyncDirection() {
return Optional.ofNullable(defaultSyncDirection);
}
public AttributeMapping withDefaultSyncDirection(Integer defaultSyncDirection) {
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.defaultSyncDirection = defaultSyncDirection;
return _x;
}
@Property(name="AllowedSyncDirection")
@JsonIgnore
public Optional getAllowedSyncDirection() {
return Optional.ofNullable(allowedSyncDirection);
}
public AttributeMapping withAllowedSyncDirection(Integer allowedSyncDirection) {
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.allowedSyncDirection = allowedSyncDirection;
return _x;
}
@Property(name="IsComputed")
@JsonIgnore
public Optional getIsComputed() {
return Optional.ofNullable(isComputed);
}
public AttributeMapping withIsComputed(Boolean isComputed) {
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.isComputed = isComputed;
return _x;
}
@Property(name="EntityTypeCode")
@JsonIgnore
public Optional getEntityTypeCode() {
return Optional.ofNullable(entityTypeCode);
}
public AttributeMapping withEntityTypeCode(Integer entityTypeCode) {
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.entityTypeCode = entityTypeCode;
return _x;
}
@Property(name="ComputedProperties")
@JsonIgnore
public CollectionPage getComputedProperties() {
return new CollectionPage(contextPath, String.class, this.computedProperties, Optional.ofNullable(computedPropertiesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="ComputedProperties")
@JsonIgnore
public CollectionPage getComputedProperties(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.computedProperties, Optional.ofNullable(computedPropertiesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="AttributeCrmDisplayName")
@JsonIgnore
public Optional getAttributeCrmDisplayName() {
return Optional.ofNullable(attributeCrmDisplayName);
}
public AttributeMapping withAttributeCrmDisplayName(String attributeCrmDisplayName) {
Checks.checkIsAscii(attributeCrmDisplayName);
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.attributeCrmDisplayName = attributeCrmDisplayName;
return _x;
}
@Property(name="AttributeExchangeDisplayName")
@JsonIgnore
public Optional getAttributeExchangeDisplayName() {
return Optional.ofNullable(attributeExchangeDisplayName);
}
public AttributeMapping withAttributeExchangeDisplayName(String attributeExchangeDisplayName) {
Checks.checkIsAscii(attributeExchangeDisplayName);
AttributeMapping _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.AttributeMapping");
_x.attributeExchangeDisplayName = attributeExchangeDisplayName;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String attributeMappingId;
private String mappingName;
private String attributeCrmName;
private String attributeExchangeName;
private Integer syncDirection;
private Integer defaultSyncDirection;
private Integer allowedSyncDirection;
private Boolean isComputed;
private Integer entityTypeCode;
private List computedProperties;
private String computedPropertiesNextLink;
private String attributeCrmDisplayName;
private String attributeExchangeDisplayName;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder attributeMappingId(String attributeMappingId) {
this.attributeMappingId = attributeMappingId;
this.changedFields = changedFields.add("AttributeMappingId");
return this;
}
public Builder mappingName(String mappingName) {
this.mappingName = mappingName;
this.changedFields = changedFields.add("MappingName");
return this;
}
public Builder attributeCrmName(String attributeCrmName) {
this.attributeCrmName = attributeCrmName;
this.changedFields = changedFields.add("AttributeCrmName");
return this;
}
public Builder attributeExchangeName(String attributeExchangeName) {
this.attributeExchangeName = attributeExchangeName;
this.changedFields = changedFields.add("AttributeExchangeName");
return this;
}
public Builder syncDirection(Integer syncDirection) {
this.syncDirection = syncDirection;
this.changedFields = changedFields.add("SyncDirection");
return this;
}
public Builder defaultSyncDirection(Integer defaultSyncDirection) {
this.defaultSyncDirection = defaultSyncDirection;
this.changedFields = changedFields.add("DefaultSyncDirection");
return this;
}
public Builder allowedSyncDirection(Integer allowedSyncDirection) {
this.allowedSyncDirection = allowedSyncDirection;
this.changedFields = changedFields.add("AllowedSyncDirection");
return this;
}
public Builder isComputed(Boolean isComputed) {
this.isComputed = isComputed;
this.changedFields = changedFields.add("IsComputed");
return this;
}
public Builder entityTypeCode(Integer entityTypeCode) {
this.entityTypeCode = entityTypeCode;
this.changedFields = changedFields.add("EntityTypeCode");
return this;
}
public Builder computedProperties(List computedProperties) {
this.computedProperties = computedProperties;
this.changedFields = changedFields.add("ComputedProperties");
return this;
}
public Builder computedProperties(String... computedProperties) {
return computedProperties(Arrays.asList(computedProperties));
}
public Builder computedPropertiesNextLink(String computedPropertiesNextLink) {
this.computedPropertiesNextLink = computedPropertiesNextLink;
this.changedFields = changedFields.add("ComputedProperties");
return this;
}
public Builder attributeCrmDisplayName(String attributeCrmDisplayName) {
this.attributeCrmDisplayName = attributeCrmDisplayName;
this.changedFields = changedFields.add("AttributeCrmDisplayName");
return this;
}
public Builder attributeExchangeDisplayName(String attributeExchangeDisplayName) {
this.attributeExchangeDisplayName = attributeExchangeDisplayName;
this.changedFields = changedFields.add("AttributeExchangeDisplayName");
return this;
}
public AttributeMapping build() {
AttributeMapping _x = new AttributeMapping();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.AttributeMapping";
_x.attributeMappingId = attributeMappingId;
_x.mappingName = mappingName;
_x.attributeCrmName = attributeCrmName;
_x.attributeExchangeName = attributeExchangeName;
_x.syncDirection = syncDirection;
_x.defaultSyncDirection = defaultSyncDirection;
_x.allowedSyncDirection = allowedSyncDirection;
_x.isComputed = isComputed;
_x.entityTypeCode = entityTypeCode;
_x.computedProperties = computedProperties;
_x.computedPropertiesNextLink = computedPropertiesNextLink;
_x.attributeCrmDisplayName = attributeCrmDisplayName;
_x.attributeExchangeDisplayName = attributeExchangeDisplayName;
return _x;
}
}
private AttributeMapping _copy() {
AttributeMapping _x = new AttributeMapping();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.attributeMappingId = attributeMappingId;
_x.mappingName = mappingName;
_x.attributeCrmName = attributeCrmName;
_x.attributeExchangeName = attributeExchangeName;
_x.syncDirection = syncDirection;
_x.defaultSyncDirection = defaultSyncDirection;
_x.allowedSyncDirection = allowedSyncDirection;
_x.isComputed = isComputed;
_x.entityTypeCode = entityTypeCode;
_x.computedProperties = computedProperties;
_x.attributeCrmDisplayName = attributeCrmDisplayName;
_x.attributeExchangeDisplayName = attributeExchangeDisplayName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AttributeMapping[");
b.append("AttributeMappingId=");
b.append(this.attributeMappingId);
b.append(", ");
b.append("MappingName=");
b.append(this.mappingName);
b.append(", ");
b.append("AttributeCrmName=");
b.append(this.attributeCrmName);
b.append(", ");
b.append("AttributeExchangeName=");
b.append(this.attributeExchangeName);
b.append(", ");
b.append("SyncDirection=");
b.append(this.syncDirection);
b.append(", ");
b.append("DefaultSyncDirection=");
b.append(this.defaultSyncDirection);
b.append(", ");
b.append("AllowedSyncDirection=");
b.append(this.allowedSyncDirection);
b.append(", ");
b.append("IsComputed=");
b.append(this.isComputed);
b.append(", ");
b.append("EntityTypeCode=");
b.append(this.entityTypeCode);
b.append(", ");
b.append("ComputedProperties=");
b.append(this.computedProperties);
b.append(", ");
b.append("AttributeCrmDisplayName=");
b.append(this.attributeCrmDisplayName);
b.append(", ");
b.append("AttributeExchangeDisplayName=");
b.append(this.attributeExchangeDisplayName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy