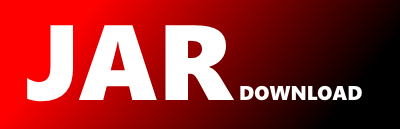
microsoft.dynamics.crm.complex.ComplexManyToManyRelationshipMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
import microsoft.dynamics.crm.enums.RelationshipType;
import microsoft.dynamics.crm.enums.SecurityTypes;
@JsonPropertyOrder({
"@odata.type",
"Entity1AssociatedMenuConfiguration",
"Entity2AssociatedMenuConfiguration",
"Entity1LogicalName",
"Entity2LogicalName",
"IntersectEntityName",
"Entity1IntersectAttribute",
"Entity2IntersectAttribute",
"Entity1NavigationPropertyName",
"Entity2NavigationPropertyName",
"IsCustomRelationship",
"IsCustomizable",
"IsValidForAdvancedFind",
"SchemaName",
"SecurityTypes",
"IsManaged",
"RelationshipType",
"IntroducedVersion",
"MetadataId",
"HasChanged"})
@JsonInclude(Include.NON_NULL)
public class ComplexManyToManyRelationshipMetadata implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("Entity1AssociatedMenuConfiguration")
protected AssociatedMenuConfiguration entity1AssociatedMenuConfiguration;
@JsonProperty("Entity2AssociatedMenuConfiguration")
protected AssociatedMenuConfiguration entity2AssociatedMenuConfiguration;
@JsonProperty("Entity1LogicalName")
protected String entity1LogicalName;
@JsonProperty("Entity2LogicalName")
protected String entity2LogicalName;
@JsonProperty("IntersectEntityName")
protected String intersectEntityName;
@JsonProperty("Entity1IntersectAttribute")
protected String entity1IntersectAttribute;
@JsonProperty("Entity2IntersectAttribute")
protected String entity2IntersectAttribute;
@JsonProperty("Entity1NavigationPropertyName")
protected String entity1NavigationPropertyName;
@JsonProperty("Entity2NavigationPropertyName")
protected String entity2NavigationPropertyName;
@JsonProperty("IsCustomRelationship")
protected Boolean isCustomRelationship;
@JsonProperty("IsCustomizable")
protected BooleanManagedProperty isCustomizable;
@JsonProperty("IsValidForAdvancedFind")
protected Boolean isValidForAdvancedFind;
@JsonProperty("SchemaName")
protected String schemaName;
@JsonProperty("SecurityTypes")
protected SecurityTypes securityTypes;
@JsonProperty("IsManaged")
protected Boolean isManaged;
@JsonProperty("RelationshipType")
protected RelationshipType relationshipType;
@JsonProperty("IntroducedVersion")
protected String introducedVersion;
@JsonProperty("MetadataId")
protected String metadataId;
@JsonProperty("HasChanged")
protected Boolean hasChanged;
protected ComplexManyToManyRelationshipMetadata() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata";
}
@Property(name="Entity1AssociatedMenuConfiguration")
@JsonIgnore
public Optional getEntity1AssociatedMenuConfiguration() {
return Optional.ofNullable(entity1AssociatedMenuConfiguration);
}
public ComplexManyToManyRelationshipMetadata withEntity1AssociatedMenuConfiguration(AssociatedMenuConfiguration entity1AssociatedMenuConfiguration) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity1AssociatedMenuConfiguration = entity1AssociatedMenuConfiguration;
return _x;
}
@Property(name="Entity2AssociatedMenuConfiguration")
@JsonIgnore
public Optional getEntity2AssociatedMenuConfiguration() {
return Optional.ofNullable(entity2AssociatedMenuConfiguration);
}
public ComplexManyToManyRelationshipMetadata withEntity2AssociatedMenuConfiguration(AssociatedMenuConfiguration entity2AssociatedMenuConfiguration) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity2AssociatedMenuConfiguration = entity2AssociatedMenuConfiguration;
return _x;
}
@Property(name="Entity1LogicalName")
@JsonIgnore
public Optional getEntity1LogicalName() {
return Optional.ofNullable(entity1LogicalName);
}
public ComplexManyToManyRelationshipMetadata withEntity1LogicalName(String entity1LogicalName) {
Checks.checkIsAscii(entity1LogicalName);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity1LogicalName = entity1LogicalName;
return _x;
}
@Property(name="Entity2LogicalName")
@JsonIgnore
public Optional getEntity2LogicalName() {
return Optional.ofNullable(entity2LogicalName);
}
public ComplexManyToManyRelationshipMetadata withEntity2LogicalName(String entity2LogicalName) {
Checks.checkIsAscii(entity2LogicalName);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity2LogicalName = entity2LogicalName;
return _x;
}
@Property(name="IntersectEntityName")
@JsonIgnore
public Optional getIntersectEntityName() {
return Optional.ofNullable(intersectEntityName);
}
public ComplexManyToManyRelationshipMetadata withIntersectEntityName(String intersectEntityName) {
Checks.checkIsAscii(intersectEntityName);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.intersectEntityName = intersectEntityName;
return _x;
}
@Property(name="Entity1IntersectAttribute")
@JsonIgnore
public Optional getEntity1IntersectAttribute() {
return Optional.ofNullable(entity1IntersectAttribute);
}
public ComplexManyToManyRelationshipMetadata withEntity1IntersectAttribute(String entity1IntersectAttribute) {
Checks.checkIsAscii(entity1IntersectAttribute);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity1IntersectAttribute = entity1IntersectAttribute;
return _x;
}
@Property(name="Entity2IntersectAttribute")
@JsonIgnore
public Optional getEntity2IntersectAttribute() {
return Optional.ofNullable(entity2IntersectAttribute);
}
public ComplexManyToManyRelationshipMetadata withEntity2IntersectAttribute(String entity2IntersectAttribute) {
Checks.checkIsAscii(entity2IntersectAttribute);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity2IntersectAttribute = entity2IntersectAttribute;
return _x;
}
@Property(name="Entity1NavigationPropertyName")
@JsonIgnore
public Optional getEntity1NavigationPropertyName() {
return Optional.ofNullable(entity1NavigationPropertyName);
}
public ComplexManyToManyRelationshipMetadata withEntity1NavigationPropertyName(String entity1NavigationPropertyName) {
Checks.checkIsAscii(entity1NavigationPropertyName);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity1NavigationPropertyName = entity1NavigationPropertyName;
return _x;
}
@Property(name="Entity2NavigationPropertyName")
@JsonIgnore
public Optional getEntity2NavigationPropertyName() {
return Optional.ofNullable(entity2NavigationPropertyName);
}
public ComplexManyToManyRelationshipMetadata withEntity2NavigationPropertyName(String entity2NavigationPropertyName) {
Checks.checkIsAscii(entity2NavigationPropertyName);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.entity2NavigationPropertyName = entity2NavigationPropertyName;
return _x;
}
@Property(name="IsCustomRelationship")
@JsonIgnore
public Optional getIsCustomRelationship() {
return Optional.ofNullable(isCustomRelationship);
}
public ComplexManyToManyRelationshipMetadata withIsCustomRelationship(Boolean isCustomRelationship) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.isCustomRelationship = isCustomRelationship;
return _x;
}
@Property(name="IsCustomizable")
@JsonIgnore
public Optional getIsCustomizable() {
return Optional.ofNullable(isCustomizable);
}
public ComplexManyToManyRelationshipMetadata withIsCustomizable(BooleanManagedProperty isCustomizable) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.isCustomizable = isCustomizable;
return _x;
}
@Property(name="IsValidForAdvancedFind")
@JsonIgnore
public Optional getIsValidForAdvancedFind() {
return Optional.ofNullable(isValidForAdvancedFind);
}
public ComplexManyToManyRelationshipMetadata withIsValidForAdvancedFind(Boolean isValidForAdvancedFind) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.isValidForAdvancedFind = isValidForAdvancedFind;
return _x;
}
@Property(name="SchemaName")
@JsonIgnore
public Optional getSchemaName() {
return Optional.ofNullable(schemaName);
}
public ComplexManyToManyRelationshipMetadata withSchemaName(String schemaName) {
Checks.checkIsAscii(schemaName);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.schemaName = schemaName;
return _x;
}
@Property(name="SecurityTypes")
@JsonIgnore
public Optional getSecurityTypes() {
return Optional.ofNullable(securityTypes);
}
public ComplexManyToManyRelationshipMetadata withSecurityTypes(SecurityTypes securityTypes) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.securityTypes = securityTypes;
return _x;
}
@Property(name="IsManaged")
@JsonIgnore
public Optional getIsManaged() {
return Optional.ofNullable(isManaged);
}
public ComplexManyToManyRelationshipMetadata withIsManaged(Boolean isManaged) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.isManaged = isManaged;
return _x;
}
@Property(name="RelationshipType")
@JsonIgnore
public Optional getRelationshipType() {
return Optional.ofNullable(relationshipType);
}
public ComplexManyToManyRelationshipMetadata withRelationshipType(RelationshipType relationshipType) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.relationshipType = relationshipType;
return _x;
}
@Property(name="IntroducedVersion")
@JsonIgnore
public Optional getIntroducedVersion() {
return Optional.ofNullable(introducedVersion);
}
public ComplexManyToManyRelationshipMetadata withIntroducedVersion(String introducedVersion) {
Checks.checkIsAscii(introducedVersion);
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.introducedVersion = introducedVersion;
return _x;
}
@Property(name="MetadataId")
@JsonIgnore
public Optional getMetadataId() {
return Optional.ofNullable(metadataId);
}
public ComplexManyToManyRelationshipMetadata withMetadataId(String metadataId) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.metadataId = metadataId;
return _x;
}
@Property(name="HasChanged")
@JsonIgnore
public Optional getHasChanged() {
return Optional.ofNullable(hasChanged);
}
public ComplexManyToManyRelationshipMetadata withHasChanged(Boolean hasChanged) {
ComplexManyToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata");
_x.hasChanged = hasChanged;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private AssociatedMenuConfiguration entity1AssociatedMenuConfiguration;
private AssociatedMenuConfiguration entity2AssociatedMenuConfiguration;
private String entity1LogicalName;
private String entity2LogicalName;
private String intersectEntityName;
private String entity1IntersectAttribute;
private String entity2IntersectAttribute;
private String entity1NavigationPropertyName;
private String entity2NavigationPropertyName;
private Boolean isCustomRelationship;
private BooleanManagedProperty isCustomizable;
private Boolean isValidForAdvancedFind;
private String schemaName;
private SecurityTypes securityTypes;
private Boolean isManaged;
private RelationshipType relationshipType;
private String introducedVersion;
private String metadataId;
private Boolean hasChanged;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder entity1AssociatedMenuConfiguration(AssociatedMenuConfiguration entity1AssociatedMenuConfiguration) {
this.entity1AssociatedMenuConfiguration = entity1AssociatedMenuConfiguration;
this.changedFields = changedFields.add("Entity1AssociatedMenuConfiguration");
return this;
}
public Builder entity2AssociatedMenuConfiguration(AssociatedMenuConfiguration entity2AssociatedMenuConfiguration) {
this.entity2AssociatedMenuConfiguration = entity2AssociatedMenuConfiguration;
this.changedFields = changedFields.add("Entity2AssociatedMenuConfiguration");
return this;
}
public Builder entity1LogicalName(String entity1LogicalName) {
this.entity1LogicalName = entity1LogicalName;
this.changedFields = changedFields.add("Entity1LogicalName");
return this;
}
public Builder entity2LogicalName(String entity2LogicalName) {
this.entity2LogicalName = entity2LogicalName;
this.changedFields = changedFields.add("Entity2LogicalName");
return this;
}
public Builder intersectEntityName(String intersectEntityName) {
this.intersectEntityName = intersectEntityName;
this.changedFields = changedFields.add("IntersectEntityName");
return this;
}
public Builder entity1IntersectAttribute(String entity1IntersectAttribute) {
this.entity1IntersectAttribute = entity1IntersectAttribute;
this.changedFields = changedFields.add("Entity1IntersectAttribute");
return this;
}
public Builder entity2IntersectAttribute(String entity2IntersectAttribute) {
this.entity2IntersectAttribute = entity2IntersectAttribute;
this.changedFields = changedFields.add("Entity2IntersectAttribute");
return this;
}
public Builder entity1NavigationPropertyName(String entity1NavigationPropertyName) {
this.entity1NavigationPropertyName = entity1NavigationPropertyName;
this.changedFields = changedFields.add("Entity1NavigationPropertyName");
return this;
}
public Builder entity2NavigationPropertyName(String entity2NavigationPropertyName) {
this.entity2NavigationPropertyName = entity2NavigationPropertyName;
this.changedFields = changedFields.add("Entity2NavigationPropertyName");
return this;
}
public Builder isCustomRelationship(Boolean isCustomRelationship) {
this.isCustomRelationship = isCustomRelationship;
this.changedFields = changedFields.add("IsCustomRelationship");
return this;
}
public Builder isCustomizable(BooleanManagedProperty isCustomizable) {
this.isCustomizable = isCustomizable;
this.changedFields = changedFields.add("IsCustomizable");
return this;
}
public Builder isValidForAdvancedFind(Boolean isValidForAdvancedFind) {
this.isValidForAdvancedFind = isValidForAdvancedFind;
this.changedFields = changedFields.add("IsValidForAdvancedFind");
return this;
}
public Builder schemaName(String schemaName) {
this.schemaName = schemaName;
this.changedFields = changedFields.add("SchemaName");
return this;
}
public Builder securityTypes(SecurityTypes securityTypes) {
this.securityTypes = securityTypes;
this.changedFields = changedFields.add("SecurityTypes");
return this;
}
public Builder isManaged(Boolean isManaged) {
this.isManaged = isManaged;
this.changedFields = changedFields.add("IsManaged");
return this;
}
public Builder relationshipType(RelationshipType relationshipType) {
this.relationshipType = relationshipType;
this.changedFields = changedFields.add("RelationshipType");
return this;
}
public Builder introducedVersion(String introducedVersion) {
this.introducedVersion = introducedVersion;
this.changedFields = changedFields.add("IntroducedVersion");
return this;
}
public Builder metadataId(String metadataId) {
this.metadataId = metadataId;
this.changedFields = changedFields.add("MetadataId");
return this;
}
public Builder hasChanged(Boolean hasChanged) {
this.hasChanged = hasChanged;
this.changedFields = changedFields.add("HasChanged");
return this;
}
public ComplexManyToManyRelationshipMetadata build() {
ComplexManyToManyRelationshipMetadata _x = new ComplexManyToManyRelationshipMetadata();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.ComplexManyToManyRelationshipMetadata";
_x.entity1AssociatedMenuConfiguration = entity1AssociatedMenuConfiguration;
_x.entity2AssociatedMenuConfiguration = entity2AssociatedMenuConfiguration;
_x.entity1LogicalName = entity1LogicalName;
_x.entity2LogicalName = entity2LogicalName;
_x.intersectEntityName = intersectEntityName;
_x.entity1IntersectAttribute = entity1IntersectAttribute;
_x.entity2IntersectAttribute = entity2IntersectAttribute;
_x.entity1NavigationPropertyName = entity1NavigationPropertyName;
_x.entity2NavigationPropertyName = entity2NavigationPropertyName;
_x.isCustomRelationship = isCustomRelationship;
_x.isCustomizable = isCustomizable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.schemaName = schemaName;
_x.securityTypes = securityTypes;
_x.isManaged = isManaged;
_x.relationshipType = relationshipType;
_x.introducedVersion = introducedVersion;
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
return _x;
}
}
private ComplexManyToManyRelationshipMetadata _copy() {
ComplexManyToManyRelationshipMetadata _x = new ComplexManyToManyRelationshipMetadata();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.entity1AssociatedMenuConfiguration = entity1AssociatedMenuConfiguration;
_x.entity2AssociatedMenuConfiguration = entity2AssociatedMenuConfiguration;
_x.entity1LogicalName = entity1LogicalName;
_x.entity2LogicalName = entity2LogicalName;
_x.intersectEntityName = intersectEntityName;
_x.entity1IntersectAttribute = entity1IntersectAttribute;
_x.entity2IntersectAttribute = entity2IntersectAttribute;
_x.entity1NavigationPropertyName = entity1NavigationPropertyName;
_x.entity2NavigationPropertyName = entity2NavigationPropertyName;
_x.isCustomRelationship = isCustomRelationship;
_x.isCustomizable = isCustomizable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.schemaName = schemaName;
_x.securityTypes = securityTypes;
_x.isManaged = isManaged;
_x.relationshipType = relationshipType;
_x.introducedVersion = introducedVersion;
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ComplexManyToManyRelationshipMetadata[");
b.append("Entity1AssociatedMenuConfiguration=");
b.append(this.entity1AssociatedMenuConfiguration);
b.append(", ");
b.append("Entity2AssociatedMenuConfiguration=");
b.append(this.entity2AssociatedMenuConfiguration);
b.append(", ");
b.append("Entity1LogicalName=");
b.append(this.entity1LogicalName);
b.append(", ");
b.append("Entity2LogicalName=");
b.append(this.entity2LogicalName);
b.append(", ");
b.append("IntersectEntityName=");
b.append(this.intersectEntityName);
b.append(", ");
b.append("Entity1IntersectAttribute=");
b.append(this.entity1IntersectAttribute);
b.append(", ");
b.append("Entity2IntersectAttribute=");
b.append(this.entity2IntersectAttribute);
b.append(", ");
b.append("Entity1NavigationPropertyName=");
b.append(this.entity1NavigationPropertyName);
b.append(", ");
b.append("Entity2NavigationPropertyName=");
b.append(this.entity2NavigationPropertyName);
b.append(", ");
b.append("IsCustomRelationship=");
b.append(this.isCustomRelationship);
b.append(", ");
b.append("IsCustomizable=");
b.append(this.isCustomizable);
b.append(", ");
b.append("IsValidForAdvancedFind=");
b.append(this.isValidForAdvancedFind);
b.append(", ");
b.append("SchemaName=");
b.append(this.schemaName);
b.append(", ");
b.append("SecurityTypes=");
b.append(this.securityTypes);
b.append(", ");
b.append("IsManaged=");
b.append(this.isManaged);
b.append(", ");
b.append("RelationshipType=");
b.append(this.relationshipType);
b.append(", ");
b.append("IntroducedVersion=");
b.append(this.introducedVersion);
b.append(", ");
b.append("MetadataId=");
b.append(this.metadataId);
b.append(", ");
b.append("HasChanged=");
b.append(this.hasChanged);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy