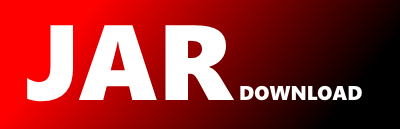
microsoft.dynamics.crm.complex.ComplexOneToManyRelationshipMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.enums.RelationshipType;
import microsoft.dynamics.crm.enums.SecurityTypes;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"AssociatedMenuConfiguration",
"CascadeConfiguration",
"ReferencedAttribute",
"ReferencedEntity",
"ReferencingAttribute",
"ReferencingEntity",
"IsHierarchical",
"EntityKey",
"RelationshipAttributes",
"ReferencedEntityNavigationPropertyName",
"ReferencingEntityNavigationPropertyName",
"RelationshipBehavior",
"IsCustomRelationship",
"IsCustomizable",
"IsValidForAdvancedFind",
"SchemaName",
"SecurityTypes",
"IsManaged",
"RelationshipType",
"IntroducedVersion",
"MetadataId",
"HasChanged"})
@JsonInclude(Include.NON_NULL)
public class ComplexOneToManyRelationshipMetadata implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("AssociatedMenuConfiguration")
protected AssociatedMenuConfiguration associatedMenuConfiguration;
@JsonProperty("CascadeConfiguration")
protected CascadeConfiguration cascadeConfiguration;
@JsonProperty("ReferencedAttribute")
protected String referencedAttribute;
@JsonProperty("ReferencedEntity")
protected String referencedEntity;
@JsonProperty("ReferencingAttribute")
protected String referencingAttribute;
@JsonProperty("ReferencingEntity")
protected String referencingEntity;
@JsonProperty("IsHierarchical")
protected Boolean isHierarchical;
@JsonProperty("EntityKey")
protected String entityKey;
@JsonProperty("RelationshipAttributes")
protected List relationshipAttributes;
@JsonProperty("RelationshipAttributes@nextLink")
protected String relationshipAttributesNextLink;
@JsonProperty("ReferencedEntityNavigationPropertyName")
protected String referencedEntityNavigationPropertyName;
@JsonProperty("ReferencingEntityNavigationPropertyName")
protected String referencingEntityNavigationPropertyName;
@JsonProperty("RelationshipBehavior")
protected Integer relationshipBehavior;
@JsonProperty("IsCustomRelationship")
protected Boolean isCustomRelationship;
@JsonProperty("IsCustomizable")
protected BooleanManagedProperty isCustomizable;
@JsonProperty("IsValidForAdvancedFind")
protected Boolean isValidForAdvancedFind;
@JsonProperty("SchemaName")
protected String schemaName;
@JsonProperty("SecurityTypes")
protected SecurityTypes securityTypes;
@JsonProperty("IsManaged")
protected Boolean isManaged;
@JsonProperty("RelationshipType")
protected RelationshipType relationshipType;
@JsonProperty("IntroducedVersion")
protected String introducedVersion;
@JsonProperty("MetadataId")
protected String metadataId;
@JsonProperty("HasChanged")
protected Boolean hasChanged;
protected ComplexOneToManyRelationshipMetadata() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata";
}
@Property(name="AssociatedMenuConfiguration")
@JsonIgnore
public Optional getAssociatedMenuConfiguration() {
return Optional.ofNullable(associatedMenuConfiguration);
}
public ComplexOneToManyRelationshipMetadata withAssociatedMenuConfiguration(AssociatedMenuConfiguration associatedMenuConfiguration) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.associatedMenuConfiguration = associatedMenuConfiguration;
return _x;
}
@Property(name="CascadeConfiguration")
@JsonIgnore
public Optional getCascadeConfiguration() {
return Optional.ofNullable(cascadeConfiguration);
}
public ComplexOneToManyRelationshipMetadata withCascadeConfiguration(CascadeConfiguration cascadeConfiguration) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.cascadeConfiguration = cascadeConfiguration;
return _x;
}
@Property(name="ReferencedAttribute")
@JsonIgnore
public Optional getReferencedAttribute() {
return Optional.ofNullable(referencedAttribute);
}
public ComplexOneToManyRelationshipMetadata withReferencedAttribute(String referencedAttribute) {
Checks.checkIsAscii(referencedAttribute);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.referencedAttribute = referencedAttribute;
return _x;
}
@Property(name="ReferencedEntity")
@JsonIgnore
public Optional getReferencedEntity() {
return Optional.ofNullable(referencedEntity);
}
public ComplexOneToManyRelationshipMetadata withReferencedEntity(String referencedEntity) {
Checks.checkIsAscii(referencedEntity);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.referencedEntity = referencedEntity;
return _x;
}
@Property(name="ReferencingAttribute")
@JsonIgnore
public Optional getReferencingAttribute() {
return Optional.ofNullable(referencingAttribute);
}
public ComplexOneToManyRelationshipMetadata withReferencingAttribute(String referencingAttribute) {
Checks.checkIsAscii(referencingAttribute);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.referencingAttribute = referencingAttribute;
return _x;
}
@Property(name="ReferencingEntity")
@JsonIgnore
public Optional getReferencingEntity() {
return Optional.ofNullable(referencingEntity);
}
public ComplexOneToManyRelationshipMetadata withReferencingEntity(String referencingEntity) {
Checks.checkIsAscii(referencingEntity);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.referencingEntity = referencingEntity;
return _x;
}
@Property(name="IsHierarchical")
@JsonIgnore
public Optional getIsHierarchical() {
return Optional.ofNullable(isHierarchical);
}
public ComplexOneToManyRelationshipMetadata withIsHierarchical(Boolean isHierarchical) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.isHierarchical = isHierarchical;
return _x;
}
@Property(name="EntityKey")
@JsonIgnore
public Optional getEntityKey() {
return Optional.ofNullable(entityKey);
}
public ComplexOneToManyRelationshipMetadata withEntityKey(String entityKey) {
Checks.checkIsAscii(entityKey);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.entityKey = entityKey;
return _x;
}
@Property(name="RelationshipAttributes")
@JsonIgnore
public CollectionPage getRelationshipAttributes() {
return new CollectionPage(contextPath, RelationshipAttribute.class, this.relationshipAttributes, Optional.ofNullable(relationshipAttributesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="RelationshipAttributes")
@JsonIgnore
public CollectionPage getRelationshipAttributes(HttpRequestOptions options) {
return new CollectionPage(contextPath, RelationshipAttribute.class, this.relationshipAttributes, Optional.ofNullable(relationshipAttributesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="ReferencedEntityNavigationPropertyName")
@JsonIgnore
public Optional getReferencedEntityNavigationPropertyName() {
return Optional.ofNullable(referencedEntityNavigationPropertyName);
}
public ComplexOneToManyRelationshipMetadata withReferencedEntityNavigationPropertyName(String referencedEntityNavigationPropertyName) {
Checks.checkIsAscii(referencedEntityNavigationPropertyName);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.referencedEntityNavigationPropertyName = referencedEntityNavigationPropertyName;
return _x;
}
@Property(name="ReferencingEntityNavigationPropertyName")
@JsonIgnore
public Optional getReferencingEntityNavigationPropertyName() {
return Optional.ofNullable(referencingEntityNavigationPropertyName);
}
public ComplexOneToManyRelationshipMetadata withReferencingEntityNavigationPropertyName(String referencingEntityNavigationPropertyName) {
Checks.checkIsAscii(referencingEntityNavigationPropertyName);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.referencingEntityNavigationPropertyName = referencingEntityNavigationPropertyName;
return _x;
}
@Property(name="RelationshipBehavior")
@JsonIgnore
public Optional getRelationshipBehavior() {
return Optional.ofNullable(relationshipBehavior);
}
public ComplexOneToManyRelationshipMetadata withRelationshipBehavior(Integer relationshipBehavior) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.relationshipBehavior = relationshipBehavior;
return _x;
}
@Property(name="IsCustomRelationship")
@JsonIgnore
public Optional getIsCustomRelationship() {
return Optional.ofNullable(isCustomRelationship);
}
public ComplexOneToManyRelationshipMetadata withIsCustomRelationship(Boolean isCustomRelationship) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.isCustomRelationship = isCustomRelationship;
return _x;
}
@Property(name="IsCustomizable")
@JsonIgnore
public Optional getIsCustomizable() {
return Optional.ofNullable(isCustomizable);
}
public ComplexOneToManyRelationshipMetadata withIsCustomizable(BooleanManagedProperty isCustomizable) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.isCustomizable = isCustomizable;
return _x;
}
@Property(name="IsValidForAdvancedFind")
@JsonIgnore
public Optional getIsValidForAdvancedFind() {
return Optional.ofNullable(isValidForAdvancedFind);
}
public ComplexOneToManyRelationshipMetadata withIsValidForAdvancedFind(Boolean isValidForAdvancedFind) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.isValidForAdvancedFind = isValidForAdvancedFind;
return _x;
}
@Property(name="SchemaName")
@JsonIgnore
public Optional getSchemaName() {
return Optional.ofNullable(schemaName);
}
public ComplexOneToManyRelationshipMetadata withSchemaName(String schemaName) {
Checks.checkIsAscii(schemaName);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.schemaName = schemaName;
return _x;
}
@Property(name="SecurityTypes")
@JsonIgnore
public Optional getSecurityTypes() {
return Optional.ofNullable(securityTypes);
}
public ComplexOneToManyRelationshipMetadata withSecurityTypes(SecurityTypes securityTypes) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.securityTypes = securityTypes;
return _x;
}
@Property(name="IsManaged")
@JsonIgnore
public Optional getIsManaged() {
return Optional.ofNullable(isManaged);
}
public ComplexOneToManyRelationshipMetadata withIsManaged(Boolean isManaged) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.isManaged = isManaged;
return _x;
}
@Property(name="RelationshipType")
@JsonIgnore
public Optional getRelationshipType() {
return Optional.ofNullable(relationshipType);
}
public ComplexOneToManyRelationshipMetadata withRelationshipType(RelationshipType relationshipType) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.relationshipType = relationshipType;
return _x;
}
@Property(name="IntroducedVersion")
@JsonIgnore
public Optional getIntroducedVersion() {
return Optional.ofNullable(introducedVersion);
}
public ComplexOneToManyRelationshipMetadata withIntroducedVersion(String introducedVersion) {
Checks.checkIsAscii(introducedVersion);
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.introducedVersion = introducedVersion;
return _x;
}
@Property(name="MetadataId")
@JsonIgnore
public Optional getMetadataId() {
return Optional.ofNullable(metadataId);
}
public ComplexOneToManyRelationshipMetadata withMetadataId(String metadataId) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.metadataId = metadataId;
return _x;
}
@Property(name="HasChanged")
@JsonIgnore
public Optional getHasChanged() {
return Optional.ofNullable(hasChanged);
}
public ComplexOneToManyRelationshipMetadata withHasChanged(Boolean hasChanged) {
ComplexOneToManyRelationshipMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata");
_x.hasChanged = hasChanged;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private AssociatedMenuConfiguration associatedMenuConfiguration;
private CascadeConfiguration cascadeConfiguration;
private String referencedAttribute;
private String referencedEntity;
private String referencingAttribute;
private String referencingEntity;
private Boolean isHierarchical;
private String entityKey;
private List relationshipAttributes;
private String relationshipAttributesNextLink;
private String referencedEntityNavigationPropertyName;
private String referencingEntityNavigationPropertyName;
private Integer relationshipBehavior;
private Boolean isCustomRelationship;
private BooleanManagedProperty isCustomizable;
private Boolean isValidForAdvancedFind;
private String schemaName;
private SecurityTypes securityTypes;
private Boolean isManaged;
private RelationshipType relationshipType;
private String introducedVersion;
private String metadataId;
private Boolean hasChanged;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder associatedMenuConfiguration(AssociatedMenuConfiguration associatedMenuConfiguration) {
this.associatedMenuConfiguration = associatedMenuConfiguration;
this.changedFields = changedFields.add("AssociatedMenuConfiguration");
return this;
}
public Builder cascadeConfiguration(CascadeConfiguration cascadeConfiguration) {
this.cascadeConfiguration = cascadeConfiguration;
this.changedFields = changedFields.add("CascadeConfiguration");
return this;
}
public Builder referencedAttribute(String referencedAttribute) {
this.referencedAttribute = referencedAttribute;
this.changedFields = changedFields.add("ReferencedAttribute");
return this;
}
public Builder referencedEntity(String referencedEntity) {
this.referencedEntity = referencedEntity;
this.changedFields = changedFields.add("ReferencedEntity");
return this;
}
public Builder referencingAttribute(String referencingAttribute) {
this.referencingAttribute = referencingAttribute;
this.changedFields = changedFields.add("ReferencingAttribute");
return this;
}
public Builder referencingEntity(String referencingEntity) {
this.referencingEntity = referencingEntity;
this.changedFields = changedFields.add("ReferencingEntity");
return this;
}
public Builder isHierarchical(Boolean isHierarchical) {
this.isHierarchical = isHierarchical;
this.changedFields = changedFields.add("IsHierarchical");
return this;
}
public Builder entityKey(String entityKey) {
this.entityKey = entityKey;
this.changedFields = changedFields.add("EntityKey");
return this;
}
public Builder relationshipAttributes(List relationshipAttributes) {
this.relationshipAttributes = relationshipAttributes;
this.changedFields = changedFields.add("RelationshipAttributes");
return this;
}
public Builder relationshipAttributes(RelationshipAttribute... relationshipAttributes) {
return relationshipAttributes(Arrays.asList(relationshipAttributes));
}
public Builder relationshipAttributesNextLink(String relationshipAttributesNextLink) {
this.relationshipAttributesNextLink = relationshipAttributesNextLink;
this.changedFields = changedFields.add("RelationshipAttributes");
return this;
}
public Builder referencedEntityNavigationPropertyName(String referencedEntityNavigationPropertyName) {
this.referencedEntityNavigationPropertyName = referencedEntityNavigationPropertyName;
this.changedFields = changedFields.add("ReferencedEntityNavigationPropertyName");
return this;
}
public Builder referencingEntityNavigationPropertyName(String referencingEntityNavigationPropertyName) {
this.referencingEntityNavigationPropertyName = referencingEntityNavigationPropertyName;
this.changedFields = changedFields.add("ReferencingEntityNavigationPropertyName");
return this;
}
public Builder relationshipBehavior(Integer relationshipBehavior) {
this.relationshipBehavior = relationshipBehavior;
this.changedFields = changedFields.add("RelationshipBehavior");
return this;
}
public Builder isCustomRelationship(Boolean isCustomRelationship) {
this.isCustomRelationship = isCustomRelationship;
this.changedFields = changedFields.add("IsCustomRelationship");
return this;
}
public Builder isCustomizable(BooleanManagedProperty isCustomizable) {
this.isCustomizable = isCustomizable;
this.changedFields = changedFields.add("IsCustomizable");
return this;
}
public Builder isValidForAdvancedFind(Boolean isValidForAdvancedFind) {
this.isValidForAdvancedFind = isValidForAdvancedFind;
this.changedFields = changedFields.add("IsValidForAdvancedFind");
return this;
}
public Builder schemaName(String schemaName) {
this.schemaName = schemaName;
this.changedFields = changedFields.add("SchemaName");
return this;
}
public Builder securityTypes(SecurityTypes securityTypes) {
this.securityTypes = securityTypes;
this.changedFields = changedFields.add("SecurityTypes");
return this;
}
public Builder isManaged(Boolean isManaged) {
this.isManaged = isManaged;
this.changedFields = changedFields.add("IsManaged");
return this;
}
public Builder relationshipType(RelationshipType relationshipType) {
this.relationshipType = relationshipType;
this.changedFields = changedFields.add("RelationshipType");
return this;
}
public Builder introducedVersion(String introducedVersion) {
this.introducedVersion = introducedVersion;
this.changedFields = changedFields.add("IntroducedVersion");
return this;
}
public Builder metadataId(String metadataId) {
this.metadataId = metadataId;
this.changedFields = changedFields.add("MetadataId");
return this;
}
public Builder hasChanged(Boolean hasChanged) {
this.hasChanged = hasChanged;
this.changedFields = changedFields.add("HasChanged");
return this;
}
public ComplexOneToManyRelationshipMetadata build() {
ComplexOneToManyRelationshipMetadata _x = new ComplexOneToManyRelationshipMetadata();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.ComplexOneToManyRelationshipMetadata";
_x.associatedMenuConfiguration = associatedMenuConfiguration;
_x.cascadeConfiguration = cascadeConfiguration;
_x.referencedAttribute = referencedAttribute;
_x.referencedEntity = referencedEntity;
_x.referencingAttribute = referencingAttribute;
_x.referencingEntity = referencingEntity;
_x.isHierarchical = isHierarchical;
_x.entityKey = entityKey;
_x.relationshipAttributes = relationshipAttributes;
_x.relationshipAttributesNextLink = relationshipAttributesNextLink;
_x.referencedEntityNavigationPropertyName = referencedEntityNavigationPropertyName;
_x.referencingEntityNavigationPropertyName = referencingEntityNavigationPropertyName;
_x.relationshipBehavior = relationshipBehavior;
_x.isCustomRelationship = isCustomRelationship;
_x.isCustomizable = isCustomizable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.schemaName = schemaName;
_x.securityTypes = securityTypes;
_x.isManaged = isManaged;
_x.relationshipType = relationshipType;
_x.introducedVersion = introducedVersion;
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
return _x;
}
}
private ComplexOneToManyRelationshipMetadata _copy() {
ComplexOneToManyRelationshipMetadata _x = new ComplexOneToManyRelationshipMetadata();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.associatedMenuConfiguration = associatedMenuConfiguration;
_x.cascadeConfiguration = cascadeConfiguration;
_x.referencedAttribute = referencedAttribute;
_x.referencedEntity = referencedEntity;
_x.referencingAttribute = referencingAttribute;
_x.referencingEntity = referencingEntity;
_x.isHierarchical = isHierarchical;
_x.entityKey = entityKey;
_x.relationshipAttributes = relationshipAttributes;
_x.referencedEntityNavigationPropertyName = referencedEntityNavigationPropertyName;
_x.referencingEntityNavigationPropertyName = referencingEntityNavigationPropertyName;
_x.relationshipBehavior = relationshipBehavior;
_x.isCustomRelationship = isCustomRelationship;
_x.isCustomizable = isCustomizable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.schemaName = schemaName;
_x.securityTypes = securityTypes;
_x.isManaged = isManaged;
_x.relationshipType = relationshipType;
_x.introducedVersion = introducedVersion;
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ComplexOneToManyRelationshipMetadata[");
b.append("AssociatedMenuConfiguration=");
b.append(this.associatedMenuConfiguration);
b.append(", ");
b.append("CascadeConfiguration=");
b.append(this.cascadeConfiguration);
b.append(", ");
b.append("ReferencedAttribute=");
b.append(this.referencedAttribute);
b.append(", ");
b.append("ReferencedEntity=");
b.append(this.referencedEntity);
b.append(", ");
b.append("ReferencingAttribute=");
b.append(this.referencingAttribute);
b.append(", ");
b.append("ReferencingEntity=");
b.append(this.referencingEntity);
b.append(", ");
b.append("IsHierarchical=");
b.append(this.isHierarchical);
b.append(", ");
b.append("EntityKey=");
b.append(this.entityKey);
b.append(", ");
b.append("RelationshipAttributes=");
b.append(this.relationshipAttributes);
b.append(", ");
b.append("ReferencedEntityNavigationPropertyName=");
b.append(this.referencedEntityNavigationPropertyName);
b.append(", ");
b.append("ReferencingEntityNavigationPropertyName=");
b.append(this.referencingEntityNavigationPropertyName);
b.append(", ");
b.append("RelationshipBehavior=");
b.append(this.relationshipBehavior);
b.append(", ");
b.append("IsCustomRelationship=");
b.append(this.isCustomRelationship);
b.append(", ");
b.append("IsCustomizable=");
b.append(this.isCustomizable);
b.append(", ");
b.append("IsValidForAdvancedFind=");
b.append(this.isValidForAdvancedFind);
b.append(", ");
b.append("SchemaName=");
b.append(this.schemaName);
b.append(", ");
b.append("SecurityTypes=");
b.append(this.securityTypes);
b.append(", ");
b.append("IsManaged=");
b.append(this.isManaged);
b.append(", ");
b.append("RelationshipType=");
b.append(this.relationshipType);
b.append(", ");
b.append("IntroducedVersion=");
b.append(this.introducedVersion);
b.append(", ");
b.append("MetadataId=");
b.append(this.metadataId);
b.append(", ");
b.append("HasChanged=");
b.append(this.hasChanged);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy