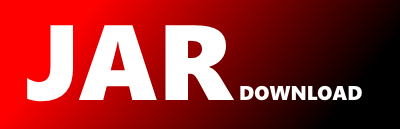
microsoft.dynamics.crm.complex.ComponentDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"Type",
"SchemaName",
"DisplayName",
"Id",
"ParentSchemaName",
"ParentDisplayName",
"ParentId",
"Solution"})
@JsonInclude(Include.NON_NULL)
public class ComponentDetail implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("Type")
protected Integer type;
@JsonProperty("SchemaName")
protected String schemaName;
@JsonProperty("DisplayName")
protected String displayName;
@JsonProperty("Id")
protected String id;
@JsonProperty("ParentSchemaName")
protected String parentSchemaName;
@JsonProperty("ParentDisplayName")
protected String parentDisplayName;
@JsonProperty("ParentId")
protected String parentId;
@JsonProperty("Solution")
protected String solution;
protected ComponentDetail() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.ComponentDetail";
}
@Property(name="Type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public ComponentDetail withType(Integer type) {
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.type = type;
return _x;
}
@Property(name="SchemaName")
@JsonIgnore
public Optional getSchemaName() {
return Optional.ofNullable(schemaName);
}
public ComponentDetail withSchemaName(String schemaName) {
Checks.checkIsAscii(schemaName);
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.schemaName = schemaName;
return _x;
}
@Property(name="DisplayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public ComponentDetail withDisplayName(String displayName) {
Checks.checkIsAscii(displayName);
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.displayName = displayName;
return _x;
}
@Property(name="Id")
@JsonIgnore
public Optional getId() {
return Optional.ofNullable(id);
}
public ComponentDetail withId(String id) {
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.id = id;
return _x;
}
@Property(name="ParentSchemaName")
@JsonIgnore
public Optional getParentSchemaName() {
return Optional.ofNullable(parentSchemaName);
}
public ComponentDetail withParentSchemaName(String parentSchemaName) {
Checks.checkIsAscii(parentSchemaName);
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.parentSchemaName = parentSchemaName;
return _x;
}
@Property(name="ParentDisplayName")
@JsonIgnore
public Optional getParentDisplayName() {
return Optional.ofNullable(parentDisplayName);
}
public ComponentDetail withParentDisplayName(String parentDisplayName) {
Checks.checkIsAscii(parentDisplayName);
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.parentDisplayName = parentDisplayName;
return _x;
}
@Property(name="ParentId")
@JsonIgnore
public Optional getParentId() {
return Optional.ofNullable(parentId);
}
public ComponentDetail withParentId(String parentId) {
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.parentId = parentId;
return _x;
}
@Property(name="Solution")
@JsonIgnore
public Optional getSolution() {
return Optional.ofNullable(solution);
}
public ComponentDetail withSolution(String solution) {
Checks.checkIsAscii(solution);
ComponentDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ComponentDetail");
_x.solution = solution;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Integer type;
private String schemaName;
private String displayName;
private String id;
private String parentSchemaName;
private String parentDisplayName;
private String parentId;
private String solution;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder type(Integer type) {
this.type = type;
this.changedFields = changedFields.add("Type");
return this;
}
public Builder schemaName(String schemaName) {
this.schemaName = schemaName;
this.changedFields = changedFields.add("SchemaName");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("Id");
return this;
}
public Builder parentSchemaName(String parentSchemaName) {
this.parentSchemaName = parentSchemaName;
this.changedFields = changedFields.add("ParentSchemaName");
return this;
}
public Builder parentDisplayName(String parentDisplayName) {
this.parentDisplayName = parentDisplayName;
this.changedFields = changedFields.add("ParentDisplayName");
return this;
}
public Builder parentId(String parentId) {
this.parentId = parentId;
this.changedFields = changedFields.add("ParentId");
return this;
}
public Builder solution(String solution) {
this.solution = solution;
this.changedFields = changedFields.add("Solution");
return this;
}
public ComponentDetail build() {
ComponentDetail _x = new ComponentDetail();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.ComponentDetail";
_x.type = type;
_x.schemaName = schemaName;
_x.displayName = displayName;
_x.id = id;
_x.parentSchemaName = parentSchemaName;
_x.parentDisplayName = parentDisplayName;
_x.parentId = parentId;
_x.solution = solution;
return _x;
}
}
private ComponentDetail _copy() {
ComponentDetail _x = new ComponentDetail();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.type = type;
_x.schemaName = schemaName;
_x.displayName = displayName;
_x.id = id;
_x.parentSchemaName = parentSchemaName;
_x.parentDisplayName = parentDisplayName;
_x.parentId = parentId;
_x.solution = solution;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ComponentDetail[");
b.append("Type=");
b.append(this.type);
b.append(", ");
b.append("SchemaName=");
b.append(this.schemaName);
b.append(", ");
b.append("DisplayName=");
b.append(this.displayName);
b.append(", ");
b.append("Id=");
b.append(this.id);
b.append(", ");
b.append("ParentSchemaName=");
b.append(this.parentSchemaName);
b.append(", ");
b.append("ParentDisplayName=");
b.append(this.parentDisplayName);
b.append(", ");
b.append("ParentId=");
b.append(this.parentId);
b.append(", ");
b.append("Solution=");
b.append(this.solution);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy