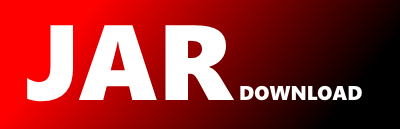
microsoft.dynamics.crm.complex.DependentAttributeMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.EdmSchemaInfo;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"AttributeId",
"LogicalName",
"DisplayName",
"AttributeType",
"AttributeFormatType",
"EntityName",
"IsCustomAttribute",
"IsValidForAdvancedFind",
"IsValidForGrid",
"YomiOf",
"AttributeOf",
"OptionSet",
"Targets",
"IsRangeBased",
"MinValue",
"MaxValue",
"Precision",
"MaxLength"})
@JsonInclude(Include.NON_NULL)
public class DependentAttributeMetadata implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("AttributeId")
protected String attributeId;
@JsonProperty("LogicalName")
protected String logicalName;
@JsonProperty("DisplayName")
protected String displayName;
@JsonProperty("AttributeType")
protected String attributeType;
@JsonProperty("AttributeFormatType")
protected String attributeFormatType;
@JsonProperty("EntityName")
protected String entityName;
@JsonProperty("IsCustomAttribute")
protected Boolean isCustomAttribute;
@JsonProperty("IsValidForAdvancedFind")
protected Boolean isValidForAdvancedFind;
@JsonProperty("IsValidForGrid")
protected Boolean isValidForGrid;
@JsonProperty("YomiOf")
protected String yomiOf;
@JsonProperty("AttributeOf")
protected String attributeOf;
@JsonProperty("OptionSet")
protected DependentOptionSetMetadata optionSet;
@JsonProperty("Targets")
protected List targets;
@JsonProperty("Targets@nextLink")
protected String targetsNextLink;
@JsonProperty("IsRangeBased")
protected Boolean isRangeBased;
@JsonProperty("MinValue")
protected Double minValue;
@JsonProperty("MaxValue")
protected Double maxValue;
@JsonProperty("Precision")
protected Integer precision;
@JsonProperty("MaxLength")
protected Integer maxLength;
protected DependentAttributeMetadata() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.DependentAttributeMetadata";
}
@Property(name="AttributeId")
@JsonIgnore
public Optional getAttributeId() {
return Optional.ofNullable(attributeId);
}
public DependentAttributeMetadata withAttributeId(String attributeId) {
Checks.checkIsAscii(attributeId);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.attributeId = attributeId;
return _x;
}
@Property(name="LogicalName")
@JsonIgnore
public Optional getLogicalName() {
return Optional.ofNullable(logicalName);
}
public DependentAttributeMetadata withLogicalName(String logicalName) {
Checks.checkIsAscii(logicalName);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.logicalName = logicalName;
return _x;
}
@Property(name="DisplayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public DependentAttributeMetadata withDisplayName(String displayName) {
Checks.checkIsAscii(displayName);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.displayName = displayName;
return _x;
}
@Property(name="AttributeType")
@JsonIgnore
public Optional getAttributeType() {
return Optional.ofNullable(attributeType);
}
public DependentAttributeMetadata withAttributeType(String attributeType) {
Checks.checkIsAscii(attributeType);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.attributeType = attributeType;
return _x;
}
@Property(name="AttributeFormatType")
@JsonIgnore
public Optional getAttributeFormatType() {
return Optional.ofNullable(attributeFormatType);
}
public DependentAttributeMetadata withAttributeFormatType(String attributeFormatType) {
Checks.checkIsAscii(attributeFormatType);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.attributeFormatType = attributeFormatType;
return _x;
}
@Property(name="EntityName")
@JsonIgnore
public Optional getEntityName() {
return Optional.ofNullable(entityName);
}
public DependentAttributeMetadata withEntityName(String entityName) {
Checks.checkIsAscii(entityName);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.entityName = entityName;
return _x;
}
@Property(name="IsCustomAttribute")
@JsonIgnore
public Optional getIsCustomAttribute() {
return Optional.ofNullable(isCustomAttribute);
}
public DependentAttributeMetadata withIsCustomAttribute(Boolean isCustomAttribute) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.isCustomAttribute = isCustomAttribute;
return _x;
}
@Property(name="IsValidForAdvancedFind")
@JsonIgnore
public Optional getIsValidForAdvancedFind() {
return Optional.ofNullable(isValidForAdvancedFind);
}
public DependentAttributeMetadata withIsValidForAdvancedFind(Boolean isValidForAdvancedFind) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.isValidForAdvancedFind = isValidForAdvancedFind;
return _x;
}
@Property(name="IsValidForGrid")
@JsonIgnore
public Optional getIsValidForGrid() {
return Optional.ofNullable(isValidForGrid);
}
public DependentAttributeMetadata withIsValidForGrid(Boolean isValidForGrid) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.isValidForGrid = isValidForGrid;
return _x;
}
@Property(name="YomiOf")
@JsonIgnore
public Optional getYomiOf() {
return Optional.ofNullable(yomiOf);
}
public DependentAttributeMetadata withYomiOf(String yomiOf) {
Checks.checkIsAscii(yomiOf);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.yomiOf = yomiOf;
return _x;
}
@Property(name="AttributeOf")
@JsonIgnore
public Optional getAttributeOf() {
return Optional.ofNullable(attributeOf);
}
public DependentAttributeMetadata withAttributeOf(String attributeOf) {
Checks.checkIsAscii(attributeOf);
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.attributeOf = attributeOf;
return _x;
}
@Property(name="OptionSet")
@JsonIgnore
public Optional getOptionSet() {
return Optional.ofNullable(optionSet);
}
public DependentAttributeMetadata withOptionSet(DependentOptionSetMetadata optionSet) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.optionSet = optionSet;
return _x;
}
@Property(name="Targets")
@JsonIgnore
public CollectionPage getTargets() {
return new CollectionPage(contextPath, String.class, this.targets, Optional.ofNullable(targetsNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Targets")
@JsonIgnore
public CollectionPage getTargets(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.targets, Optional.ofNullable(targetsNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="IsRangeBased")
@JsonIgnore
public Optional getIsRangeBased() {
return Optional.ofNullable(isRangeBased);
}
public DependentAttributeMetadata withIsRangeBased(Boolean isRangeBased) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.isRangeBased = isRangeBased;
return _x;
}
@Property(name="MinValue")
@JsonIgnore
public Optional getMinValue() {
return Optional.ofNullable(minValue);
}
public DependentAttributeMetadata withMinValue(Double minValue) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.minValue = minValue;
return _x;
}
@Property(name="MaxValue")
@JsonIgnore
public Optional getMaxValue() {
return Optional.ofNullable(maxValue);
}
public DependentAttributeMetadata withMaxValue(Double maxValue) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.maxValue = maxValue;
return _x;
}
@Property(name="Precision")
@JsonIgnore
public Optional getPrecision() {
return Optional.ofNullable(precision);
}
public DependentAttributeMetadata withPrecision(Integer precision) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.precision = precision;
return _x;
}
@Property(name="MaxLength")
@JsonIgnore
public Optional getMaxLength() {
return Optional.ofNullable(maxLength);
}
public DependentAttributeMetadata withMaxLength(Integer maxLength) {
DependentAttributeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.DependentAttributeMetadata");
_x.maxLength = maxLength;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String attributeId;
private String logicalName;
private String displayName;
private String attributeType;
private String attributeFormatType;
private String entityName;
private Boolean isCustomAttribute;
private Boolean isValidForAdvancedFind;
private Boolean isValidForGrid;
private String yomiOf;
private String attributeOf;
private DependentOptionSetMetadata optionSet;
private List targets;
private String targetsNextLink;
private Boolean isRangeBased;
private Double minValue;
private Double maxValue;
private Integer precision;
private Integer maxLength;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder attributeId(String attributeId) {
this.attributeId = attributeId;
this.changedFields = changedFields.add("AttributeId");
return this;
}
public Builder logicalName(String logicalName) {
this.logicalName = logicalName;
this.changedFields = changedFields.add("LogicalName");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder attributeType(String attributeType) {
this.attributeType = attributeType;
this.changedFields = changedFields.add("AttributeType");
return this;
}
public Builder attributeFormatType(String attributeFormatType) {
this.attributeFormatType = attributeFormatType;
this.changedFields = changedFields.add("AttributeFormatType");
return this;
}
public Builder entityName(String entityName) {
this.entityName = entityName;
this.changedFields = changedFields.add("EntityName");
return this;
}
public Builder isCustomAttribute(Boolean isCustomAttribute) {
this.isCustomAttribute = isCustomAttribute;
this.changedFields = changedFields.add("IsCustomAttribute");
return this;
}
public Builder isValidForAdvancedFind(Boolean isValidForAdvancedFind) {
this.isValidForAdvancedFind = isValidForAdvancedFind;
this.changedFields = changedFields.add("IsValidForAdvancedFind");
return this;
}
public Builder isValidForGrid(Boolean isValidForGrid) {
this.isValidForGrid = isValidForGrid;
this.changedFields = changedFields.add("IsValidForGrid");
return this;
}
public Builder yomiOf(String yomiOf) {
this.yomiOf = yomiOf;
this.changedFields = changedFields.add("YomiOf");
return this;
}
public Builder attributeOf(String attributeOf) {
this.attributeOf = attributeOf;
this.changedFields = changedFields.add("AttributeOf");
return this;
}
public Builder optionSet(DependentOptionSetMetadata optionSet) {
this.optionSet = optionSet;
this.changedFields = changedFields.add("OptionSet");
return this;
}
public Builder targets(List targets) {
this.targets = targets;
this.changedFields = changedFields.add("Targets");
return this;
}
public Builder targets(String... targets) {
return targets(Arrays.asList(targets));
}
public Builder targetsNextLink(String targetsNextLink) {
this.targetsNextLink = targetsNextLink;
this.changedFields = changedFields.add("Targets");
return this;
}
public Builder isRangeBased(Boolean isRangeBased) {
this.isRangeBased = isRangeBased;
this.changedFields = changedFields.add("IsRangeBased");
return this;
}
public Builder minValue(Double minValue) {
this.minValue = minValue;
this.changedFields = changedFields.add("MinValue");
return this;
}
public Builder maxValue(Double maxValue) {
this.maxValue = maxValue;
this.changedFields = changedFields.add("MaxValue");
return this;
}
public Builder precision(Integer precision) {
this.precision = precision;
this.changedFields = changedFields.add("Precision");
return this;
}
public Builder maxLength(Integer maxLength) {
this.maxLength = maxLength;
this.changedFields = changedFields.add("MaxLength");
return this;
}
public DependentAttributeMetadata build() {
DependentAttributeMetadata _x = new DependentAttributeMetadata();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.DependentAttributeMetadata";
_x.attributeId = attributeId;
_x.logicalName = logicalName;
_x.displayName = displayName;
_x.attributeType = attributeType;
_x.attributeFormatType = attributeFormatType;
_x.entityName = entityName;
_x.isCustomAttribute = isCustomAttribute;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.isValidForGrid = isValidForGrid;
_x.yomiOf = yomiOf;
_x.attributeOf = attributeOf;
_x.optionSet = optionSet;
_x.targets = targets;
_x.targetsNextLink = targetsNextLink;
_x.isRangeBased = isRangeBased;
_x.minValue = minValue;
_x.maxValue = maxValue;
_x.precision = precision;
_x.maxLength = maxLength;
return _x;
}
}
private DependentAttributeMetadata _copy() {
DependentAttributeMetadata _x = new DependentAttributeMetadata();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.attributeId = attributeId;
_x.logicalName = logicalName;
_x.displayName = displayName;
_x.attributeType = attributeType;
_x.attributeFormatType = attributeFormatType;
_x.entityName = entityName;
_x.isCustomAttribute = isCustomAttribute;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.isValidForGrid = isValidForGrid;
_x.yomiOf = yomiOf;
_x.attributeOf = attributeOf;
_x.optionSet = optionSet;
_x.targets = targets;
_x.isRangeBased = isRangeBased;
_x.minValue = minValue;
_x.maxValue = maxValue;
_x.precision = precision;
_x.maxLength = maxLength;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("DependentAttributeMetadata[");
b.append("AttributeId=");
b.append(this.attributeId);
b.append(", ");
b.append("LogicalName=");
b.append(this.logicalName);
b.append(", ");
b.append("DisplayName=");
b.append(this.displayName);
b.append(", ");
b.append("AttributeType=");
b.append(this.attributeType);
b.append(", ");
b.append("AttributeFormatType=");
b.append(this.attributeFormatType);
b.append(", ");
b.append("EntityName=");
b.append(this.entityName);
b.append(", ");
b.append("IsCustomAttribute=");
b.append(this.isCustomAttribute);
b.append(", ");
b.append("IsValidForAdvancedFind=");
b.append(this.isValidForAdvancedFind);
b.append(", ");
b.append("IsValidForGrid=");
b.append(this.isValidForGrid);
b.append(", ");
b.append("YomiOf=");
b.append(this.yomiOf);
b.append(", ");
b.append("AttributeOf=");
b.append(this.attributeOf);
b.append(", ");
b.append("OptionSet=");
b.append(this.optionSet);
b.append(", ");
b.append("Targets=");
b.append(this.targets);
b.append(", ");
b.append("IsRangeBased=");
b.append(this.isRangeBased);
b.append(", ");
b.append("MinValue=");
b.append(this.minValue);
b.append(", ");
b.append("MaxValue=");
b.append(this.maxValue);
b.append(", ");
b.append("Precision=");
b.append(this.precision);
b.append(", ");
b.append("MaxLength=");
b.append(this.maxLength);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy