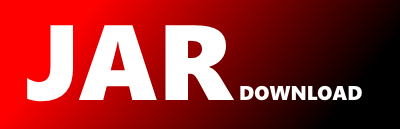
microsoft.dynamics.crm.complex.EntityRelationsWithDependantEntityMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"EntityId",
"LogicalName",
"LogicalCollectionName",
"ObjectTypeCode",
"DisplayName",
"PrimaryNameAttribute",
"PrimaryIdAttribute",
"AttributesMetadata",
"EntityRelationshipCollection",
"DependantEntitiesCollection"})
@JsonInclude(Include.NON_NULL)
public class EntityRelationsWithDependantEntityMetadata implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("EntityId")
protected String entityId;
@JsonProperty("LogicalName")
protected String logicalName;
@JsonProperty("LogicalCollectionName")
protected String logicalCollectionName;
@JsonProperty("ObjectTypeCode")
protected Integer objectTypeCode;
@JsonProperty("DisplayName")
protected String displayName;
@JsonProperty("PrimaryNameAttribute")
protected String primaryNameAttribute;
@JsonProperty("PrimaryIdAttribute")
protected String primaryIdAttribute;
@JsonProperty("AttributesMetadata")
protected DependentAttributeMetadataCollection attributesMetadata;
@JsonProperty("EntityRelationshipCollection")
protected DependentRelationshipCollection entityRelationshipCollection;
@JsonProperty("DependantEntitiesCollection")
protected DependentEntityMetadataCollection dependantEntitiesCollection;
protected EntityRelationsWithDependantEntityMetadata() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata";
}
@Property(name="EntityId")
@JsonIgnore
public Optional getEntityId() {
return Optional.ofNullable(entityId);
}
public EntityRelationsWithDependantEntityMetadata withEntityId(String entityId) {
Checks.checkIsAscii(entityId);
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.entityId = entityId;
return _x;
}
@Property(name="LogicalName")
@JsonIgnore
public Optional getLogicalName() {
return Optional.ofNullable(logicalName);
}
public EntityRelationsWithDependantEntityMetadata withLogicalName(String logicalName) {
Checks.checkIsAscii(logicalName);
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.logicalName = logicalName;
return _x;
}
@Property(name="LogicalCollectionName")
@JsonIgnore
public Optional getLogicalCollectionName() {
return Optional.ofNullable(logicalCollectionName);
}
public EntityRelationsWithDependantEntityMetadata withLogicalCollectionName(String logicalCollectionName) {
Checks.checkIsAscii(logicalCollectionName);
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.logicalCollectionName = logicalCollectionName;
return _x;
}
@Property(name="ObjectTypeCode")
@JsonIgnore
public Optional getObjectTypeCode() {
return Optional.ofNullable(objectTypeCode);
}
public EntityRelationsWithDependantEntityMetadata withObjectTypeCode(Integer objectTypeCode) {
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.objectTypeCode = objectTypeCode;
return _x;
}
@Property(name="DisplayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public EntityRelationsWithDependantEntityMetadata withDisplayName(String displayName) {
Checks.checkIsAscii(displayName);
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.displayName = displayName;
return _x;
}
@Property(name="PrimaryNameAttribute")
@JsonIgnore
public Optional getPrimaryNameAttribute() {
return Optional.ofNullable(primaryNameAttribute);
}
public EntityRelationsWithDependantEntityMetadata withPrimaryNameAttribute(String primaryNameAttribute) {
Checks.checkIsAscii(primaryNameAttribute);
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.primaryNameAttribute = primaryNameAttribute;
return _x;
}
@Property(name="PrimaryIdAttribute")
@JsonIgnore
public Optional getPrimaryIdAttribute() {
return Optional.ofNullable(primaryIdAttribute);
}
public EntityRelationsWithDependantEntityMetadata withPrimaryIdAttribute(String primaryIdAttribute) {
Checks.checkIsAscii(primaryIdAttribute);
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.primaryIdAttribute = primaryIdAttribute;
return _x;
}
@Property(name="AttributesMetadata")
@JsonIgnore
public Optional getAttributesMetadata() {
return Optional.ofNullable(attributesMetadata);
}
public EntityRelationsWithDependantEntityMetadata withAttributesMetadata(DependentAttributeMetadataCollection attributesMetadata) {
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.attributesMetadata = attributesMetadata;
return _x;
}
@Property(name="EntityRelationshipCollection")
@JsonIgnore
public Optional getEntityRelationshipCollection() {
return Optional.ofNullable(entityRelationshipCollection);
}
public EntityRelationsWithDependantEntityMetadata withEntityRelationshipCollection(DependentRelationshipCollection entityRelationshipCollection) {
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.entityRelationshipCollection = entityRelationshipCollection;
return _x;
}
@Property(name="DependantEntitiesCollection")
@JsonIgnore
public Optional getDependantEntitiesCollection() {
return Optional.ofNullable(dependantEntitiesCollection);
}
public EntityRelationsWithDependantEntityMetadata withDependantEntitiesCollection(DependentEntityMetadataCollection dependantEntitiesCollection) {
EntityRelationsWithDependantEntityMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata");
_x.dependantEntitiesCollection = dependantEntitiesCollection;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String entityId;
private String logicalName;
private String logicalCollectionName;
private Integer objectTypeCode;
private String displayName;
private String primaryNameAttribute;
private String primaryIdAttribute;
private DependentAttributeMetadataCollection attributesMetadata;
private DependentRelationshipCollection entityRelationshipCollection;
private DependentEntityMetadataCollection dependantEntitiesCollection;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder entityId(String entityId) {
this.entityId = entityId;
this.changedFields = changedFields.add("EntityId");
return this;
}
public Builder logicalName(String logicalName) {
this.logicalName = logicalName;
this.changedFields = changedFields.add("LogicalName");
return this;
}
public Builder logicalCollectionName(String logicalCollectionName) {
this.logicalCollectionName = logicalCollectionName;
this.changedFields = changedFields.add("LogicalCollectionName");
return this;
}
public Builder objectTypeCode(Integer objectTypeCode) {
this.objectTypeCode = objectTypeCode;
this.changedFields = changedFields.add("ObjectTypeCode");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder primaryNameAttribute(String primaryNameAttribute) {
this.primaryNameAttribute = primaryNameAttribute;
this.changedFields = changedFields.add("PrimaryNameAttribute");
return this;
}
public Builder primaryIdAttribute(String primaryIdAttribute) {
this.primaryIdAttribute = primaryIdAttribute;
this.changedFields = changedFields.add("PrimaryIdAttribute");
return this;
}
public Builder attributesMetadata(DependentAttributeMetadataCollection attributesMetadata) {
this.attributesMetadata = attributesMetadata;
this.changedFields = changedFields.add("AttributesMetadata");
return this;
}
public Builder entityRelationshipCollection(DependentRelationshipCollection entityRelationshipCollection) {
this.entityRelationshipCollection = entityRelationshipCollection;
this.changedFields = changedFields.add("EntityRelationshipCollection");
return this;
}
public Builder dependantEntitiesCollection(DependentEntityMetadataCollection dependantEntitiesCollection) {
this.dependantEntitiesCollection = dependantEntitiesCollection;
this.changedFields = changedFields.add("DependantEntitiesCollection");
return this;
}
public EntityRelationsWithDependantEntityMetadata build() {
EntityRelationsWithDependantEntityMetadata _x = new EntityRelationsWithDependantEntityMetadata();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.EntityRelationsWithDependantEntityMetadata";
_x.entityId = entityId;
_x.logicalName = logicalName;
_x.logicalCollectionName = logicalCollectionName;
_x.objectTypeCode = objectTypeCode;
_x.displayName = displayName;
_x.primaryNameAttribute = primaryNameAttribute;
_x.primaryIdAttribute = primaryIdAttribute;
_x.attributesMetadata = attributesMetadata;
_x.entityRelationshipCollection = entityRelationshipCollection;
_x.dependantEntitiesCollection = dependantEntitiesCollection;
return _x;
}
}
private EntityRelationsWithDependantEntityMetadata _copy() {
EntityRelationsWithDependantEntityMetadata _x = new EntityRelationsWithDependantEntityMetadata();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.entityId = entityId;
_x.logicalName = logicalName;
_x.logicalCollectionName = logicalCollectionName;
_x.objectTypeCode = objectTypeCode;
_x.displayName = displayName;
_x.primaryNameAttribute = primaryNameAttribute;
_x.primaryIdAttribute = primaryIdAttribute;
_x.attributesMetadata = attributesMetadata;
_x.entityRelationshipCollection = entityRelationshipCollection;
_x.dependantEntitiesCollection = dependantEntitiesCollection;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("EntityRelationsWithDependantEntityMetadata[");
b.append("EntityId=");
b.append(this.entityId);
b.append(", ");
b.append("LogicalName=");
b.append(this.logicalName);
b.append(", ");
b.append("LogicalCollectionName=");
b.append(this.logicalCollectionName);
b.append(", ");
b.append("ObjectTypeCode=");
b.append(this.objectTypeCode);
b.append(", ");
b.append("DisplayName=");
b.append(this.displayName);
b.append(", ");
b.append("PrimaryNameAttribute=");
b.append(this.primaryNameAttribute);
b.append(", ");
b.append("PrimaryIdAttribute=");
b.append(this.primaryIdAttribute);
b.append(", ");
b.append("AttributesMetadata=");
b.append(this.attributesMetadata);
b.append(", ");
b.append("EntityRelationshipCollection=");
b.append(this.entityRelationshipCollection);
b.append(", ");
b.append("DependantEntitiesCollection=");
b.append(this.dependantEntitiesCollection);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy