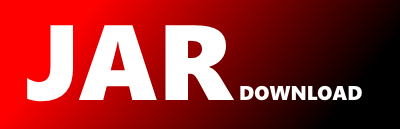
microsoft.dynamics.crm.complex.SdkMessageProcessingStepRegistration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"MessageName",
"PrimaryEntityName",
"SecondaryEntityName",
"Description",
"Stage",
"Mode",
"ImpersonatingUserId",
"SupportedDeployment",
"FilteringAttributes",
"PluginTypeFriendlyName",
"PluginTypeName",
"CustomConfiguration",
"InvocationSource",
"Images"})
@JsonInclude(Include.NON_NULL)
public class SdkMessageProcessingStepRegistration implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("MessageName")
protected String messageName;
@JsonProperty("PrimaryEntityName")
protected String primaryEntityName;
@JsonProperty("SecondaryEntityName")
protected String secondaryEntityName;
@JsonProperty("Description")
protected String description;
@JsonProperty("Stage")
protected Integer stage;
@JsonProperty("Mode")
protected Integer mode;
@JsonProperty("ImpersonatingUserId")
protected String impersonatingUserId;
@JsonProperty("SupportedDeployment")
protected Integer supportedDeployment;
@JsonProperty("FilteringAttributes")
protected String filteringAttributes;
@JsonProperty("PluginTypeFriendlyName")
protected String pluginTypeFriendlyName;
@JsonProperty("PluginTypeName")
protected String pluginTypeName;
@JsonProperty("CustomConfiguration")
protected String customConfiguration;
@JsonProperty("InvocationSource")
protected Integer invocationSource;
@JsonProperty("Images")
protected List images;
@JsonProperty("Images@nextLink")
protected String imagesNextLink;
protected SdkMessageProcessingStepRegistration() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration";
}
@Property(name="MessageName")
@JsonIgnore
public Optional getMessageName() {
return Optional.ofNullable(messageName);
}
public SdkMessageProcessingStepRegistration withMessageName(String messageName) {
Checks.checkIsAscii(messageName);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.messageName = messageName;
return _x;
}
@Property(name="PrimaryEntityName")
@JsonIgnore
public Optional getPrimaryEntityName() {
return Optional.ofNullable(primaryEntityName);
}
public SdkMessageProcessingStepRegistration withPrimaryEntityName(String primaryEntityName) {
Checks.checkIsAscii(primaryEntityName);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.primaryEntityName = primaryEntityName;
return _x;
}
@Property(name="SecondaryEntityName")
@JsonIgnore
public Optional getSecondaryEntityName() {
return Optional.ofNullable(secondaryEntityName);
}
public SdkMessageProcessingStepRegistration withSecondaryEntityName(String secondaryEntityName) {
Checks.checkIsAscii(secondaryEntityName);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.secondaryEntityName = secondaryEntityName;
return _x;
}
@Property(name="Description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public SdkMessageProcessingStepRegistration withDescription(String description) {
Checks.checkIsAscii(description);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.description = description;
return _x;
}
@Property(name="Stage")
@JsonIgnore
public Optional getStage() {
return Optional.ofNullable(stage);
}
public SdkMessageProcessingStepRegistration withStage(Integer stage) {
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.stage = stage;
return _x;
}
@Property(name="Mode")
@JsonIgnore
public Optional getMode() {
return Optional.ofNullable(mode);
}
public SdkMessageProcessingStepRegistration withMode(Integer mode) {
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.mode = mode;
return _x;
}
@Property(name="ImpersonatingUserId")
@JsonIgnore
public Optional getImpersonatingUserId() {
return Optional.ofNullable(impersonatingUserId);
}
public SdkMessageProcessingStepRegistration withImpersonatingUserId(String impersonatingUserId) {
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.impersonatingUserId = impersonatingUserId;
return _x;
}
@Property(name="SupportedDeployment")
@JsonIgnore
public Optional getSupportedDeployment() {
return Optional.ofNullable(supportedDeployment);
}
public SdkMessageProcessingStepRegistration withSupportedDeployment(Integer supportedDeployment) {
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.supportedDeployment = supportedDeployment;
return _x;
}
@Property(name="FilteringAttributes")
@JsonIgnore
public Optional getFilteringAttributes() {
return Optional.ofNullable(filteringAttributes);
}
public SdkMessageProcessingStepRegistration withFilteringAttributes(String filteringAttributes) {
Checks.checkIsAscii(filteringAttributes);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.filteringAttributes = filteringAttributes;
return _x;
}
@Property(name="PluginTypeFriendlyName")
@JsonIgnore
public Optional getPluginTypeFriendlyName() {
return Optional.ofNullable(pluginTypeFriendlyName);
}
public SdkMessageProcessingStepRegistration withPluginTypeFriendlyName(String pluginTypeFriendlyName) {
Checks.checkIsAscii(pluginTypeFriendlyName);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.pluginTypeFriendlyName = pluginTypeFriendlyName;
return _x;
}
@Property(name="PluginTypeName")
@JsonIgnore
public Optional getPluginTypeName() {
return Optional.ofNullable(pluginTypeName);
}
public SdkMessageProcessingStepRegistration withPluginTypeName(String pluginTypeName) {
Checks.checkIsAscii(pluginTypeName);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.pluginTypeName = pluginTypeName;
return _x;
}
@Property(name="CustomConfiguration")
@JsonIgnore
public Optional getCustomConfiguration() {
return Optional.ofNullable(customConfiguration);
}
public SdkMessageProcessingStepRegistration withCustomConfiguration(String customConfiguration) {
Checks.checkIsAscii(customConfiguration);
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.customConfiguration = customConfiguration;
return _x;
}
@Property(name="InvocationSource")
@JsonIgnore
public Optional getInvocationSource() {
return Optional.ofNullable(invocationSource);
}
public SdkMessageProcessingStepRegistration withInvocationSource(Integer invocationSource) {
SdkMessageProcessingStepRegistration _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration");
_x.invocationSource = invocationSource;
return _x;
}
@Property(name="Images")
@JsonIgnore
public CollectionPage getImages() {
return new CollectionPage(contextPath, SdkMessageProcessingStepImageRegistration.class, this.images, Optional.ofNullable(imagesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="Images")
@JsonIgnore
public CollectionPage getImages(HttpRequestOptions options) {
return new CollectionPage(contextPath, SdkMessageProcessingStepImageRegistration.class, this.images, Optional.ofNullable(imagesNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String messageName;
private String primaryEntityName;
private String secondaryEntityName;
private String description;
private Integer stage;
private Integer mode;
private String impersonatingUserId;
private Integer supportedDeployment;
private String filteringAttributes;
private String pluginTypeFriendlyName;
private String pluginTypeName;
private String customConfiguration;
private Integer invocationSource;
private List images;
private String imagesNextLink;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder messageName(String messageName) {
this.messageName = messageName;
this.changedFields = changedFields.add("MessageName");
return this;
}
public Builder primaryEntityName(String primaryEntityName) {
this.primaryEntityName = primaryEntityName;
this.changedFields = changedFields.add("PrimaryEntityName");
return this;
}
public Builder secondaryEntityName(String secondaryEntityName) {
this.secondaryEntityName = secondaryEntityName;
this.changedFields = changedFields.add("SecondaryEntityName");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder stage(Integer stage) {
this.stage = stage;
this.changedFields = changedFields.add("Stage");
return this;
}
public Builder mode(Integer mode) {
this.mode = mode;
this.changedFields = changedFields.add("Mode");
return this;
}
public Builder impersonatingUserId(String impersonatingUserId) {
this.impersonatingUserId = impersonatingUserId;
this.changedFields = changedFields.add("ImpersonatingUserId");
return this;
}
public Builder supportedDeployment(Integer supportedDeployment) {
this.supportedDeployment = supportedDeployment;
this.changedFields = changedFields.add("SupportedDeployment");
return this;
}
public Builder filteringAttributes(String filteringAttributes) {
this.filteringAttributes = filteringAttributes;
this.changedFields = changedFields.add("FilteringAttributes");
return this;
}
public Builder pluginTypeFriendlyName(String pluginTypeFriendlyName) {
this.pluginTypeFriendlyName = pluginTypeFriendlyName;
this.changedFields = changedFields.add("PluginTypeFriendlyName");
return this;
}
public Builder pluginTypeName(String pluginTypeName) {
this.pluginTypeName = pluginTypeName;
this.changedFields = changedFields.add("PluginTypeName");
return this;
}
public Builder customConfiguration(String customConfiguration) {
this.customConfiguration = customConfiguration;
this.changedFields = changedFields.add("CustomConfiguration");
return this;
}
public Builder invocationSource(Integer invocationSource) {
this.invocationSource = invocationSource;
this.changedFields = changedFields.add("InvocationSource");
return this;
}
public Builder images(List images) {
this.images = images;
this.changedFields = changedFields.add("Images");
return this;
}
public Builder images(SdkMessageProcessingStepImageRegistration... images) {
return images(Arrays.asList(images));
}
public Builder imagesNextLink(String imagesNextLink) {
this.imagesNextLink = imagesNextLink;
this.changedFields = changedFields.add("Images");
return this;
}
public SdkMessageProcessingStepRegistration build() {
SdkMessageProcessingStepRegistration _x = new SdkMessageProcessingStepRegistration();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.SdkMessageProcessingStepRegistration";
_x.messageName = messageName;
_x.primaryEntityName = primaryEntityName;
_x.secondaryEntityName = secondaryEntityName;
_x.description = description;
_x.stage = stage;
_x.mode = mode;
_x.impersonatingUserId = impersonatingUserId;
_x.supportedDeployment = supportedDeployment;
_x.filteringAttributes = filteringAttributes;
_x.pluginTypeFriendlyName = pluginTypeFriendlyName;
_x.pluginTypeName = pluginTypeName;
_x.customConfiguration = customConfiguration;
_x.invocationSource = invocationSource;
_x.images = images;
_x.imagesNextLink = imagesNextLink;
return _x;
}
}
private SdkMessageProcessingStepRegistration _copy() {
SdkMessageProcessingStepRegistration _x = new SdkMessageProcessingStepRegistration();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.messageName = messageName;
_x.primaryEntityName = primaryEntityName;
_x.secondaryEntityName = secondaryEntityName;
_x.description = description;
_x.stage = stage;
_x.mode = mode;
_x.impersonatingUserId = impersonatingUserId;
_x.supportedDeployment = supportedDeployment;
_x.filteringAttributes = filteringAttributes;
_x.pluginTypeFriendlyName = pluginTypeFriendlyName;
_x.pluginTypeName = pluginTypeName;
_x.customConfiguration = customConfiguration;
_x.invocationSource = invocationSource;
_x.images = images;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SdkMessageProcessingStepRegistration[");
b.append("MessageName=");
b.append(this.messageName);
b.append(", ");
b.append("PrimaryEntityName=");
b.append(this.primaryEntityName);
b.append(", ");
b.append("SecondaryEntityName=");
b.append(this.secondaryEntityName);
b.append(", ");
b.append("Description=");
b.append(this.description);
b.append(", ");
b.append("Stage=");
b.append(this.stage);
b.append(", ");
b.append("Mode=");
b.append(this.mode);
b.append(", ");
b.append("ImpersonatingUserId=");
b.append(this.impersonatingUserId);
b.append(", ");
b.append("SupportedDeployment=");
b.append(this.supportedDeployment);
b.append(", ");
b.append("FilteringAttributes=");
b.append(this.filteringAttributes);
b.append(", ");
b.append("PluginTypeFriendlyName=");
b.append(this.pluginTypeFriendlyName);
b.append(", ");
b.append("PluginTypeName=");
b.append(this.pluginTypeName);
b.append(", ");
b.append("CustomConfiguration=");
b.append(this.customConfiguration);
b.append(", ");
b.append("InvocationSource=");
b.append(this.invocationSource);
b.append(", ");
b.append("Images=");
b.append(this.images);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy