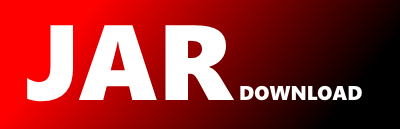
microsoft.dynamics.crm.complex.SecurityPrivilegeMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
import microsoft.dynamics.crm.enums.PrivilegeType;
@JsonPropertyOrder({
"@odata.type",
"CanBeBasic",
"CanBeDeep",
"CanBeGlobal",
"CanBeLocal",
"CanBeEntityReference",
"CanBeParentEntityReference",
"Name",
"PrivilegeId",
"PrivilegeType"})
@JsonInclude(Include.NON_NULL)
public class SecurityPrivilegeMetadata implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("CanBeBasic")
protected Boolean canBeBasic;
@JsonProperty("CanBeDeep")
protected Boolean canBeDeep;
@JsonProperty("CanBeGlobal")
protected Boolean canBeGlobal;
@JsonProperty("CanBeLocal")
protected Boolean canBeLocal;
@JsonProperty("CanBeEntityReference")
protected Boolean canBeEntityReference;
@JsonProperty("CanBeParentEntityReference")
protected Boolean canBeParentEntityReference;
@JsonProperty("Name")
protected String name;
@JsonProperty("PrivilegeId")
protected String privilegeId;
@JsonProperty("PrivilegeType")
protected PrivilegeType privilegeType;
protected SecurityPrivilegeMetadata() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata";
}
@Property(name="CanBeBasic")
@JsonIgnore
public Optional getCanBeBasic() {
return Optional.ofNullable(canBeBasic);
}
public SecurityPrivilegeMetadata withCanBeBasic(Boolean canBeBasic) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.canBeBasic = canBeBasic;
return _x;
}
@Property(name="CanBeDeep")
@JsonIgnore
public Optional getCanBeDeep() {
return Optional.ofNullable(canBeDeep);
}
public SecurityPrivilegeMetadata withCanBeDeep(Boolean canBeDeep) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.canBeDeep = canBeDeep;
return _x;
}
@Property(name="CanBeGlobal")
@JsonIgnore
public Optional getCanBeGlobal() {
return Optional.ofNullable(canBeGlobal);
}
public SecurityPrivilegeMetadata withCanBeGlobal(Boolean canBeGlobal) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.canBeGlobal = canBeGlobal;
return _x;
}
@Property(name="CanBeLocal")
@JsonIgnore
public Optional getCanBeLocal() {
return Optional.ofNullable(canBeLocal);
}
public SecurityPrivilegeMetadata withCanBeLocal(Boolean canBeLocal) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.canBeLocal = canBeLocal;
return _x;
}
@Property(name="CanBeEntityReference")
@JsonIgnore
public Optional getCanBeEntityReference() {
return Optional.ofNullable(canBeEntityReference);
}
public SecurityPrivilegeMetadata withCanBeEntityReference(Boolean canBeEntityReference) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.canBeEntityReference = canBeEntityReference;
return _x;
}
@Property(name="CanBeParentEntityReference")
@JsonIgnore
public Optional getCanBeParentEntityReference() {
return Optional.ofNullable(canBeParentEntityReference);
}
public SecurityPrivilegeMetadata withCanBeParentEntityReference(Boolean canBeParentEntityReference) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.canBeParentEntityReference = canBeParentEntityReference;
return _x;
}
@Property(name="Name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public SecurityPrivilegeMetadata withName(String name) {
Checks.checkIsAscii(name);
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.name = name;
return _x;
}
@Property(name="PrivilegeId")
@JsonIgnore
public Optional getPrivilegeId() {
return Optional.ofNullable(privilegeId);
}
public SecurityPrivilegeMetadata withPrivilegeId(String privilegeId) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.privilegeId = privilegeId;
return _x;
}
@Property(name="PrivilegeType")
@JsonIgnore
public Optional getPrivilegeType() {
return Optional.ofNullable(privilegeType);
}
public SecurityPrivilegeMetadata withPrivilegeType(PrivilegeType privilegeType) {
SecurityPrivilegeMetadata _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata");
_x.privilegeType = privilegeType;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Boolean canBeBasic;
private Boolean canBeDeep;
private Boolean canBeGlobal;
private Boolean canBeLocal;
private Boolean canBeEntityReference;
private Boolean canBeParentEntityReference;
private String name;
private String privilegeId;
private PrivilegeType privilegeType;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder canBeBasic(Boolean canBeBasic) {
this.canBeBasic = canBeBasic;
this.changedFields = changedFields.add("CanBeBasic");
return this;
}
public Builder canBeDeep(Boolean canBeDeep) {
this.canBeDeep = canBeDeep;
this.changedFields = changedFields.add("CanBeDeep");
return this;
}
public Builder canBeGlobal(Boolean canBeGlobal) {
this.canBeGlobal = canBeGlobal;
this.changedFields = changedFields.add("CanBeGlobal");
return this;
}
public Builder canBeLocal(Boolean canBeLocal) {
this.canBeLocal = canBeLocal;
this.changedFields = changedFields.add("CanBeLocal");
return this;
}
public Builder canBeEntityReference(Boolean canBeEntityReference) {
this.canBeEntityReference = canBeEntityReference;
this.changedFields = changedFields.add("CanBeEntityReference");
return this;
}
public Builder canBeParentEntityReference(Boolean canBeParentEntityReference) {
this.canBeParentEntityReference = canBeParentEntityReference;
this.changedFields = changedFields.add("CanBeParentEntityReference");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("Name");
return this;
}
public Builder privilegeId(String privilegeId) {
this.privilegeId = privilegeId;
this.changedFields = changedFields.add("PrivilegeId");
return this;
}
public Builder privilegeType(PrivilegeType privilegeType) {
this.privilegeType = privilegeType;
this.changedFields = changedFields.add("PrivilegeType");
return this;
}
public SecurityPrivilegeMetadata build() {
SecurityPrivilegeMetadata _x = new SecurityPrivilegeMetadata();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.SecurityPrivilegeMetadata";
_x.canBeBasic = canBeBasic;
_x.canBeDeep = canBeDeep;
_x.canBeGlobal = canBeGlobal;
_x.canBeLocal = canBeLocal;
_x.canBeEntityReference = canBeEntityReference;
_x.canBeParentEntityReference = canBeParentEntityReference;
_x.name = name;
_x.privilegeId = privilegeId;
_x.privilegeType = privilegeType;
return _x;
}
}
private SecurityPrivilegeMetadata _copy() {
SecurityPrivilegeMetadata _x = new SecurityPrivilegeMetadata();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.canBeBasic = canBeBasic;
_x.canBeDeep = canBeDeep;
_x.canBeGlobal = canBeGlobal;
_x.canBeLocal = canBeLocal;
_x.canBeEntityReference = canBeEntityReference;
_x.canBeParentEntityReference = canBeParentEntityReference;
_x.name = name;
_x.privilegeId = privilegeId;
_x.privilegeType = privilegeType;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SecurityPrivilegeMetadata[");
b.append("CanBeBasic=");
b.append(this.canBeBasic);
b.append(", ");
b.append("CanBeDeep=");
b.append(this.canBeDeep);
b.append(", ");
b.append("CanBeGlobal=");
b.append(this.canBeGlobal);
b.append(", ");
b.append("CanBeLocal=");
b.append(this.canBeLocal);
b.append(", ");
b.append("CanBeEntityReference=");
b.append(this.canBeEntityReference);
b.append(", ");
b.append("CanBeParentEntityReference=");
b.append(this.canBeParentEntityReference);
b.append(", ");
b.append("Name=");
b.append(this.name);
b.append(", ");
b.append("PrivilegeId=");
b.append(this.privilegeId);
b.append(", ");
b.append("PrivilegeType=");
b.append(this.privilegeType);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy