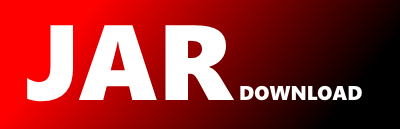
microsoft.dynamics.crm.complex.ValidationIssue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import microsoft.dynamics.crm.enums.ErrorType;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonPropertyOrder({
"@odata.type",
"ErrorType",
"Message",
"DisplayName",
"ComponentId",
"ComponentType",
"ComponentSubType",
"ParentEntityId",
"ParentEntityName",
"RequiredComponents",
"CRMErrorCode"})
@JsonInclude(Include.NON_NULL)
public class ValidationIssue implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("ErrorType")
protected ErrorType errorType;
@JsonProperty("Message")
protected String message;
@JsonProperty("DisplayName")
protected String displayName;
@JsonProperty("ComponentId")
protected String componentId;
@JsonProperty("ComponentType")
protected Integer componentType;
@JsonProperty("ComponentSubType")
protected Integer componentSubType;
@JsonProperty("ParentEntityId")
protected String parentEntityId;
@JsonProperty("ParentEntityName")
protected String parentEntityName;
@JsonProperty("RequiredComponents")
protected List requiredComponents;
@JsonProperty("RequiredComponents@nextLink")
protected String requiredComponentsNextLink;
@JsonProperty("CRMErrorCode")
protected Integer cRMErrorCode;
protected ValidationIssue() {
}
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.ValidationIssue";
}
@Property(name="ErrorType")
@JsonIgnore
public Optional getErrorType() {
return Optional.ofNullable(errorType);
}
public ValidationIssue withErrorType(ErrorType errorType) {
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.errorType = errorType;
return _x;
}
@Property(name="Message")
@JsonIgnore
public Optional getMessage() {
return Optional.ofNullable(message);
}
public ValidationIssue withMessage(String message) {
Checks.checkIsAscii(message);
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.message = message;
return _x;
}
@Property(name="DisplayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public ValidationIssue withDisplayName(String displayName) {
Checks.checkIsAscii(displayName);
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.displayName = displayName;
return _x;
}
@Property(name="ComponentId")
@JsonIgnore
public Optional getComponentId() {
return Optional.ofNullable(componentId);
}
public ValidationIssue withComponentId(String componentId) {
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.componentId = componentId;
return _x;
}
@Property(name="ComponentType")
@JsonIgnore
public Optional getComponentType() {
return Optional.ofNullable(componentType);
}
public ValidationIssue withComponentType(Integer componentType) {
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.componentType = componentType;
return _x;
}
@Property(name="ComponentSubType")
@JsonIgnore
public Optional getComponentSubType() {
return Optional.ofNullable(componentSubType);
}
public ValidationIssue withComponentSubType(Integer componentSubType) {
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.componentSubType = componentSubType;
return _x;
}
@Property(name="ParentEntityId")
@JsonIgnore
public Optional getParentEntityId() {
return Optional.ofNullable(parentEntityId);
}
public ValidationIssue withParentEntityId(String parentEntityId) {
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.parentEntityId = parentEntityId;
return _x;
}
@Property(name="ParentEntityName")
@JsonIgnore
public Optional getParentEntityName() {
return Optional.ofNullable(parentEntityName);
}
public ValidationIssue withParentEntityName(String parentEntityName) {
Checks.checkIsAscii(parentEntityName);
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.parentEntityName = parentEntityName;
return _x;
}
@Property(name="RequiredComponents")
@JsonIgnore
public CollectionPage getRequiredComponents() {
return new CollectionPage(contextPath, Component.class, this.requiredComponents, Optional.ofNullable(requiredComponentsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="RequiredComponents")
@JsonIgnore
public CollectionPage getRequiredComponents(HttpRequestOptions options) {
return new CollectionPage(contextPath, Component.class, this.requiredComponents, Optional.ofNullable(requiredComponentsNextLink), SchemaInfo.INSTANCE, Collections.emptyList(), options);
}
@Property(name="CRMErrorCode")
@JsonIgnore
public Optional getCRMErrorCode() {
return Optional.ofNullable(cRMErrorCode);
}
public ValidationIssue withCRMErrorCode(Integer cRMErrorCode) {
ValidationIssue _x = _copy();
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ValidationIssue");
_x.cRMErrorCode = cRMErrorCode;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private ErrorType errorType;
private String message;
private String displayName;
private String componentId;
private Integer componentType;
private Integer componentSubType;
private String parentEntityId;
private String parentEntityName;
private List requiredComponents;
private String requiredComponentsNextLink;
private Integer cRMErrorCode;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder errorType(ErrorType errorType) {
this.errorType = errorType;
this.changedFields = changedFields.add("ErrorType");
return this;
}
public Builder message(String message) {
this.message = message;
this.changedFields = changedFields.add("Message");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder componentId(String componentId) {
this.componentId = componentId;
this.changedFields = changedFields.add("ComponentId");
return this;
}
public Builder componentType(Integer componentType) {
this.componentType = componentType;
this.changedFields = changedFields.add("ComponentType");
return this;
}
public Builder componentSubType(Integer componentSubType) {
this.componentSubType = componentSubType;
this.changedFields = changedFields.add("ComponentSubType");
return this;
}
public Builder parentEntityId(String parentEntityId) {
this.parentEntityId = parentEntityId;
this.changedFields = changedFields.add("ParentEntityId");
return this;
}
public Builder parentEntityName(String parentEntityName) {
this.parentEntityName = parentEntityName;
this.changedFields = changedFields.add("ParentEntityName");
return this;
}
public Builder requiredComponents(List requiredComponents) {
this.requiredComponents = requiredComponents;
this.changedFields = changedFields.add("RequiredComponents");
return this;
}
public Builder requiredComponents(Component... requiredComponents) {
return requiredComponents(Arrays.asList(requiredComponents));
}
public Builder requiredComponentsNextLink(String requiredComponentsNextLink) {
this.requiredComponentsNextLink = requiredComponentsNextLink;
this.changedFields = changedFields.add("RequiredComponents");
return this;
}
public Builder cRMErrorCode(Integer cRMErrorCode) {
this.cRMErrorCode = cRMErrorCode;
this.changedFields = changedFields.add("CRMErrorCode");
return this;
}
public ValidationIssue build() {
ValidationIssue _x = new ValidationIssue();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.ValidationIssue";
_x.errorType = errorType;
_x.message = message;
_x.displayName = displayName;
_x.componentId = componentId;
_x.componentType = componentType;
_x.componentSubType = componentSubType;
_x.parentEntityId = parentEntityId;
_x.parentEntityName = parentEntityName;
_x.requiredComponents = requiredComponents;
_x.requiredComponentsNextLink = requiredComponentsNextLink;
_x.cRMErrorCode = cRMErrorCode;
return _x;
}
}
private ValidationIssue _copy() {
ValidationIssue _x = new ValidationIssue();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.errorType = errorType;
_x.message = message;
_x.displayName = displayName;
_x.componentId = componentId;
_x.componentType = componentType;
_x.componentSubType = componentSubType;
_x.parentEntityId = parentEntityId;
_x.parentEntityName = parentEntityName;
_x.requiredComponents = requiredComponents;
_x.cRMErrorCode = cRMErrorCode;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ValidationIssue[");
b.append("ErrorType=");
b.append(this.errorType);
b.append(", ");
b.append("Message=");
b.append(this.message);
b.append(", ");
b.append("DisplayName=");
b.append(this.displayName);
b.append(", ");
b.append("ComponentId=");
b.append(this.componentId);
b.append(", ");
b.append("ComponentType=");
b.append(this.componentType);
b.append(", ");
b.append("ComponentSubType=");
b.append(this.componentSubType);
b.append(", ");
b.append("ParentEntityId=");
b.append(this.parentEntityId);
b.append(", ");
b.append("ParentEntityName=");
b.append(this.parentEntityName);
b.append(", ");
b.append("RequiredComponents=");
b.append(this.requiredComponents);
b.append(", ");
b.append("CRMErrorCode=");
b.append(this.cRMErrorCode);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy