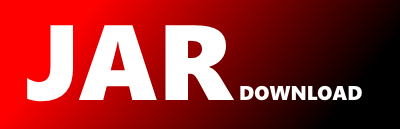
microsoft.dynamics.crm.entity.Activitymimeattachment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.ActivitypointerRequest;
import microsoft.dynamics.crm.entity.request.AppointmentRequest;
import microsoft.dynamics.crm.entity.request.AttachmentRequest;
import microsoft.dynamics.crm.entity.request.EmailRequest;
import microsoft.dynamics.crm.entity.request.TemplateRequest;
@JsonPropertyOrder({
"@odata.type",
"componentstate",
"versionnumber",
"_ownerid_value",
"subject",
"filesize",
"isfollowed",
"ismanaged",
"activitymimeattachmentid",
"_owninguser_value",
"attachmentcontentid",
"_attachmentid_value",
"objecttypecode",
"_owningbusinessunit_value",
"_objectid_value",
"activitymimeattachmentidunique",
"mimetype",
"activitysubject",
"solutionid",
"attachmentnumber",
"anonymouslink",
"filename",
"body",
"body_binary",
"overwritetime"})
@JsonInclude(Include.NON_NULL)
public class Activitymimeattachment extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.activitymimeattachment";
}
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("subject")
protected String subject;
@JsonProperty("filesize")
protected Integer filesize;
@JsonProperty("isfollowed")
protected Boolean isfollowed;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("activitymimeattachmentid")
protected String activitymimeattachmentid;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("attachmentcontentid")
protected String attachmentcontentid;
@JsonProperty("_attachmentid_value")
protected String _attachmentid_value;
@JsonProperty("objecttypecode")
protected String objecttypecode;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("_objectid_value")
protected String _objectid_value;
@JsonProperty("activitymimeattachmentidunique")
protected String activitymimeattachmentidunique;
@JsonProperty("mimetype")
protected String mimetype;
@JsonProperty("activitysubject")
protected String activitysubject;
@JsonProperty("solutionid")
protected String solutionid;
@JsonProperty("attachmentnumber")
protected Integer attachmentnumber;
@JsonProperty("anonymouslink")
protected String anonymouslink;
@JsonProperty("filename")
protected String filename;
@JsonProperty("body")
protected String body;
@JsonProperty("body_binary")
protected byte[] body_binary;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
protected Activitymimeattachment() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderActivitymimeattachment() {
return new Builder();
}
public static final class Builder {
private Integer componentstate;
private Long versionnumber;
private String _ownerid_value;
private String subject;
private Integer filesize;
private Boolean isfollowed;
private Boolean ismanaged;
private String activitymimeattachmentid;
private String _owninguser_value;
private String attachmentcontentid;
private String _attachmentid_value;
private String objecttypecode;
private String _owningbusinessunit_value;
private String _objectid_value;
private String activitymimeattachmentidunique;
private String mimetype;
private String activitysubject;
private String solutionid;
private Integer attachmentnumber;
private String anonymouslink;
private String filename;
private String body;
private byte[] body_binary;
private OffsetDateTime overwritetime;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder subject(String subject) {
this.subject = subject;
this.changedFields = changedFields.add("subject");
return this;
}
public Builder filesize(Integer filesize) {
this.filesize = filesize;
this.changedFields = changedFields.add("filesize");
return this;
}
public Builder isfollowed(Boolean isfollowed) {
this.isfollowed = isfollowed;
this.changedFields = changedFields.add("isfollowed");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder activitymimeattachmentid(String activitymimeattachmentid) {
this.activitymimeattachmentid = activitymimeattachmentid;
this.changedFields = changedFields.add("activitymimeattachmentid");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder attachmentcontentid(String attachmentcontentid) {
this.attachmentcontentid = attachmentcontentid;
this.changedFields = changedFields.add("attachmentcontentid");
return this;
}
public Builder _attachmentid_value(String _attachmentid_value) {
this._attachmentid_value = _attachmentid_value;
this.changedFields = changedFields.add("_attachmentid_value");
return this;
}
public Builder objecttypecode(String objecttypecode) {
this.objecttypecode = objecttypecode;
this.changedFields = changedFields.add("objecttypecode");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder _objectid_value(String _objectid_value) {
this._objectid_value = _objectid_value;
this.changedFields = changedFields.add("_objectid_value");
return this;
}
public Builder activitymimeattachmentidunique(String activitymimeattachmentidunique) {
this.activitymimeattachmentidunique = activitymimeattachmentidunique;
this.changedFields = changedFields.add("activitymimeattachmentidunique");
return this;
}
public Builder mimetype(String mimetype) {
this.mimetype = mimetype;
this.changedFields = changedFields.add("mimetype");
return this;
}
public Builder activitysubject(String activitysubject) {
this.activitysubject = activitysubject;
this.changedFields = changedFields.add("activitysubject");
return this;
}
public Builder solutionid(String solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder attachmentnumber(Integer attachmentnumber) {
this.attachmentnumber = attachmentnumber;
this.changedFields = changedFields.add("attachmentnumber");
return this;
}
public Builder anonymouslink(String anonymouslink) {
this.anonymouslink = anonymouslink;
this.changedFields = changedFields.add("anonymouslink");
return this;
}
public Builder filename(String filename) {
this.filename = filename;
this.changedFields = changedFields.add("filename");
return this;
}
public Builder body(String body) {
this.body = body;
this.changedFields = changedFields.add("body");
return this;
}
public Builder body_binary(byte[] body_binary) {
this.body_binary = body_binary;
this.changedFields = changedFields.add("body_binary");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Activitymimeattachment build() {
Activitymimeattachment _x = new Activitymimeattachment();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.activitymimeattachment";
_x.componentstate = componentstate;
_x.versionnumber = versionnumber;
_x._ownerid_value = _ownerid_value;
_x.subject = subject;
_x.filesize = filesize;
_x.isfollowed = isfollowed;
_x.ismanaged = ismanaged;
_x.activitymimeattachmentid = activitymimeattachmentid;
_x._owninguser_value = _owninguser_value;
_x.attachmentcontentid = attachmentcontentid;
_x._attachmentid_value = _attachmentid_value;
_x.objecttypecode = objecttypecode;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._objectid_value = _objectid_value;
_x.activitymimeattachmentidunique = activitymimeattachmentidunique;
_x.mimetype = mimetype;
_x.activitysubject = activitysubject;
_x.solutionid = solutionid;
_x.attachmentnumber = attachmentnumber;
_x.anonymouslink = anonymouslink;
_x.filename = filename;
_x.body = body;
_x.body_binary = body_binary;
_x.overwritetime = overwritetime;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && activitymimeattachmentid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(activitymimeattachmentid.toString()));
}
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Activitymimeattachment withComponentstate(Integer componentstate) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.componentstate = componentstate;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Activitymimeattachment withVersionnumber(Long versionnumber) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Activitymimeattachment with_ownerid_value(String _ownerid_value) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="subject")
@JsonIgnore
public Optional getSubject() {
return Optional.ofNullable(subject);
}
public Activitymimeattachment withSubject(String subject) {
Checks.checkIsAscii(subject);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("subject");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.subject = subject;
return _x;
}
@Property(name="filesize")
@JsonIgnore
public Optional getFilesize() {
return Optional.ofNullable(filesize);
}
public Activitymimeattachment withFilesize(Integer filesize) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("filesize");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.filesize = filesize;
return _x;
}
@Property(name="isfollowed")
@JsonIgnore
public Optional getIsfollowed() {
return Optional.ofNullable(isfollowed);
}
public Activitymimeattachment withIsfollowed(Boolean isfollowed) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("isfollowed");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.isfollowed = isfollowed;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Activitymimeattachment withIsmanaged(Boolean ismanaged) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="activitymimeattachmentid")
@JsonIgnore
public Optional getActivitymimeattachmentid() {
return Optional.ofNullable(activitymimeattachmentid);
}
public Activitymimeattachment withActivitymimeattachmentid(String activitymimeattachmentid) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("activitymimeattachmentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.activitymimeattachmentid = activitymimeattachmentid;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Activitymimeattachment with_owninguser_value(String _owninguser_value) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="attachmentcontentid")
@JsonIgnore
public Optional getAttachmentcontentid() {
return Optional.ofNullable(attachmentcontentid);
}
public Activitymimeattachment withAttachmentcontentid(String attachmentcontentid) {
Checks.checkIsAscii(attachmentcontentid);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("attachmentcontentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.attachmentcontentid = attachmentcontentid;
return _x;
}
@Property(name="_attachmentid_value")
@JsonIgnore
public Optional get_attachmentid_value() {
return Optional.ofNullable(_attachmentid_value);
}
public Activitymimeattachment with_attachmentid_value(String _attachmentid_value) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("_attachmentid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x._attachmentid_value = _attachmentid_value;
return _x;
}
@Property(name="objecttypecode")
@JsonIgnore
public Optional getObjecttypecode() {
return Optional.ofNullable(objecttypecode);
}
public Activitymimeattachment withObjecttypecode(String objecttypecode) {
Checks.checkIsAscii(objecttypecode);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("objecttypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.objecttypecode = objecttypecode;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Activitymimeattachment with_owningbusinessunit_value(String _owningbusinessunit_value) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="_objectid_value")
@JsonIgnore
public Optional get_objectid_value() {
return Optional.ofNullable(_objectid_value);
}
public Activitymimeattachment with_objectid_value(String _objectid_value) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("_objectid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x._objectid_value = _objectid_value;
return _x;
}
@Property(name="activitymimeattachmentidunique")
@JsonIgnore
public Optional getActivitymimeattachmentidunique() {
return Optional.ofNullable(activitymimeattachmentidunique);
}
public Activitymimeattachment withActivitymimeattachmentidunique(String activitymimeattachmentidunique) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("activitymimeattachmentidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.activitymimeattachmentidunique = activitymimeattachmentidunique;
return _x;
}
@Property(name="mimetype")
@JsonIgnore
public Optional getMimetype() {
return Optional.ofNullable(mimetype);
}
public Activitymimeattachment withMimetype(String mimetype) {
Checks.checkIsAscii(mimetype);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("mimetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.mimetype = mimetype;
return _x;
}
@Property(name="activitysubject")
@JsonIgnore
public Optional getActivitysubject() {
return Optional.ofNullable(activitysubject);
}
public Activitymimeattachment withActivitysubject(String activitysubject) {
Checks.checkIsAscii(activitysubject);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("activitysubject");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.activitysubject = activitysubject;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Activitymimeattachment withSolutionid(String solutionid) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.solutionid = solutionid;
return _x;
}
@Property(name="attachmentnumber")
@JsonIgnore
public Optional getAttachmentnumber() {
return Optional.ofNullable(attachmentnumber);
}
public Activitymimeattachment withAttachmentnumber(Integer attachmentnumber) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("attachmentnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.attachmentnumber = attachmentnumber;
return _x;
}
@Property(name="anonymouslink")
@JsonIgnore
public Optional getAnonymouslink() {
return Optional.ofNullable(anonymouslink);
}
public Activitymimeattachment withAnonymouslink(String anonymouslink) {
Checks.checkIsAscii(anonymouslink);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("anonymouslink");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.anonymouslink = anonymouslink;
return _x;
}
@Property(name="filename")
@JsonIgnore
public Optional getFilename() {
return Optional.ofNullable(filename);
}
public Activitymimeattachment withFilename(String filename) {
Checks.checkIsAscii(filename);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("filename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.filename = filename;
return _x;
}
@Property(name="body")
@JsonIgnore
public Optional getBody() {
return Optional.ofNullable(body);
}
public Activitymimeattachment withBody(String body) {
Checks.checkIsAscii(body);
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("body");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.body = body;
return _x;
}
@Property(name="body_binary")
@JsonIgnore
public Optional getBody_binary() {
return Optional.ofNullable(body_binary);
}
public Activitymimeattachment withBody_binary(byte[] body_binary) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("body_binary");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.body_binary = body_binary;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Activitymimeattachment withOverwritetime(OffsetDateTime overwritetime) {
Activitymimeattachment _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitymimeattachment");
_x.overwritetime = overwritetime;
return _x;
}
@NavigationProperty(name="ActivityMimeAttachment_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getActivityMimeAttachment_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("ActivityMimeAttachment_SyncErrors"));
}
@NavigationProperty(name="objectid_email")
@JsonIgnore
public EmailRequest getObjectid_email() {
return new EmailRequest(contextPath.addSegment("objectid_email"));
}
@NavigationProperty(name="objectid_activitypointer")
@JsonIgnore
public ActivitypointerRequest getObjectid_activitypointer() {
return new ActivitypointerRequest(contextPath.addSegment("objectid_activitypointer"));
}
@NavigationProperty(name="objectid_template")
@JsonIgnore
public TemplateRequest getObjectid_template() {
return new TemplateRequest(contextPath.addSegment("objectid_template"));
}
@NavigationProperty(name="attachmentid")
@JsonIgnore
public AttachmentRequest getAttachmentid() {
return new AttachmentRequest(contextPath.addSegment("attachmentid"));
}
@NavigationProperty(name="objectid_appointment")
@JsonIgnore
public AppointmentRequest getObjectid_appointment() {
return new AppointmentRequest(contextPath.addSegment("objectid_appointment"));
}
@NavigationProperty(name="ActivityMimeAttachment_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getActivityMimeAttachment_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("ActivityMimeAttachment_AsyncOperations"));
}
@NavigationProperty(name="ActivityMimeAttachment_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getActivityMimeAttachment_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("ActivityMimeAttachment_BulkDeleteFailures"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Activitymimeattachment patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Activitymimeattachment _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Activitymimeattachment put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Activitymimeattachment _x = _copy();
_x.changedFields = null;
return _x;
}
private Activitymimeattachment _copy() {
Activitymimeattachment _x = new Activitymimeattachment();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.componentstate = componentstate;
_x.versionnumber = versionnumber;
_x._ownerid_value = _ownerid_value;
_x.subject = subject;
_x.filesize = filesize;
_x.isfollowed = isfollowed;
_x.ismanaged = ismanaged;
_x.activitymimeattachmentid = activitymimeattachmentid;
_x._owninguser_value = _owninguser_value;
_x.attachmentcontentid = attachmentcontentid;
_x._attachmentid_value = _attachmentid_value;
_x.objecttypecode = objecttypecode;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._objectid_value = _objectid_value;
_x.activitymimeattachmentidunique = activitymimeattachmentidunique;
_x.mimetype = mimetype;
_x.activitysubject = activitysubject;
_x.solutionid = solutionid;
_x.attachmentnumber = attachmentnumber;
_x.anonymouslink = anonymouslink;
_x.filename = filename;
_x.body = body;
_x.body_binary = body_binary;
_x.overwritetime = overwritetime;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Activitymimeattachment[");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("subject=");
b.append(this.subject);
b.append(", ");
b.append("filesize=");
b.append(this.filesize);
b.append(", ");
b.append("isfollowed=");
b.append(this.isfollowed);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("activitymimeattachmentid=");
b.append(this.activitymimeattachmentid);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("attachmentcontentid=");
b.append(this.attachmentcontentid);
b.append(", ");
b.append("_attachmentid_value=");
b.append(this._attachmentid_value);
b.append(", ");
b.append("objecttypecode=");
b.append(this.objecttypecode);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("_objectid_value=");
b.append(this._objectid_value);
b.append(", ");
b.append("activitymimeattachmentidunique=");
b.append(this.activitymimeattachmentidunique);
b.append(", ");
b.append("mimetype=");
b.append(this.mimetype);
b.append(", ");
b.append("activitysubject=");
b.append(this.activitysubject);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("attachmentnumber=");
b.append(this.attachmentnumber);
b.append(", ");
b.append("anonymouslink=");
b.append(this.anonymouslink);
b.append(", ");
b.append("filename=");
b.append(this.filename);
b.append(", ");
b.append("body=");
b.append(this.body);
b.append(", ");
b.append("body_binary=");
b.append(this.body_binary);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy