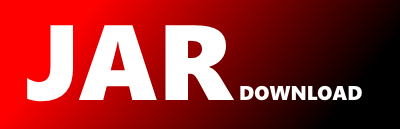
microsoft.dynamics.crm.entity.Contact Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AccountCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActioncardCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypointerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppointmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ContactCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CustomeraddressCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FaxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FeedbackCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.LetterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxtrackingfolderCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PhonecallCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostfollowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostregardingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurringappointmentmasterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlakpiinstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialactivityCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialprofileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TaskCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ProcessstageRequest;
import microsoft.dynamics.crm.entity.request.SlaRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"spousesname",
"emailaddress3",
"address3_telephone3",
"entityimage_timestamp",
"address2_shippingmethodcode",
"mobilephone",
"address3_shippingmethodcode",
"address3_postalcode",
"versionnumber",
"annualincome",
"fax",
"telephone3",
"preferredappointmentdaycode",
"address3_city",
"lastonholdtime",
"address2_stateorprovince",
"_createdby_value",
"address2_line1",
"assistantphone",
"lastusedincampaign",
"address3_freighttermscode",
"pager",
"employeeid",
"territorycode",
"_parentcustomerid_value",
"managername",
"birthdate",
"address1_name",
"department",
"address3_country",
"address2_telephone1",
"address1_postofficebox",
"address2_primarycontactname",
"address2_latitude",
"_owningteam_value",
"timezoneruleversionnumber",
"home2",
"utcconversiontimezonecode",
"address2_postalcode",
"_owninguser_value",
"merged",
"_masterid_value",
"_createdonbehalfby_value",
"address3_postofficebox",
"customertypecode",
"business2",
"address3_county",
"address1_latitude",
"address1_freighttermscode",
"traversedpath",
"address3_addresstypecode",
"address1_longitude",
"address1_addresstypecode",
"statuscode",
"yomifullname",
"aging90_base",
"_accountid_value",
"address3_primarycontactname",
"familystatuscode",
"address3_addressid",
"leadsourcecode",
"statecode",
"address2_freighttermscode",
"address1_composite",
"telephone2",
"yomimiddlename",
"_modifiedonbehalfby_value",
"jobtitle",
"address2_addresstypecode",
"educationcode",
"address1_telephone3",
"followemail",
"importsequencenumber",
"gendercode",
"address2_line2",
"creditlimit_base",
"annualincome_base",
"donotfax",
"address3_line1",
"address1_county",
"_owningbusinessunit_value",
"_createdbyexternalparty_value",
"entityimageid",
"processid",
"address1_telephone2",
"description",
"address1_fax",
"marketingonly",
"address3_line2",
"address1_addressid",
"donotpostalmail",
"address2_utcoffset",
"exchangerate",
"aging30_base",
"callback",
"emailaddress2",
"address2_line3",
"managerphone",
"websiteurl",
"overriddencreatedon",
"firstname",
"address1_telephone1",
"address3_composite",
"address3_fax",
"childrensnames",
"preferredcontactmethodcode",
"numberofchildren",
"subscriptionid",
"yomilastname",
"address2_postofficebox",
"aging90",
"donotbulkpostalmail",
"emailaddress1",
"donotbulkemail",
"customersizecode",
"address1_city",
"preferredappointmenttimecode",
"address3_latitude",
"aging60_base",
"_transactioncurrencyid_value",
"entityimage",
"_modifiedbyexternalparty_value",
"paymenttermscode",
"address3_name",
"participatesinworkflow",
"address1_shippingmethodcode",
"_modifiedby_value",
"ftpsiteurl",
"_preferredsystemuserid_value",
"address2_telephone2",
"_slainvokedid_value",
"address3_utcoffset",
"address3_stateorprovince",
"address3_telephone1",
"nickname",
"fullname",
"assistantname",
"address2_country",
"aging60",
"modifiedon",
"externaluseridentifier",
"address2_name",
"stageid",
"creditonhold",
"address3_longitude",
"onholdtime",
"address2_telephone3",
"donotphone",
"address3_upszone",
"contactid",
"aging30",
"address2_upszone",
"address1_upszone",
"creditlimit",
"accountrolecode",
"salutation",
"suffix",
"address1_primarycontactname",
"address1_utcoffset",
"address1_postalcode",
"governmentid",
"donotsendmm",
"address1_country",
"lastname",
"address2_city",
"donotemail",
"company",
"address1_line2",
"address2_longitude",
"address1_stateorprovince",
"yomifirstname",
"telephone1",
"address1_line1",
"address2_addressid",
"isbackofficecustomer",
"address2_county",
"shippingmethodcode",
"anniversary",
"_ownerid_value",
"_parentcontactid_value",
"haschildrencode",
"address2_fax",
"address2_composite",
"createdon",
"entityimage_url",
"address1_line3",
"_slaid_value",
"middlename",
"address3_line3",
"address3_telephone2",
"timespentbymeonemailandmeetings"})
@JsonInclude(Include.NON_NULL)
public class Contact extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.contact";
}
@JsonProperty("spousesname")
protected String spousesname;
@JsonProperty("emailaddress3")
protected String emailaddress3;
@JsonProperty("address3_telephone3")
protected String address3_telephone3;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("address2_shippingmethodcode")
protected Integer address2_shippingmethodcode;
@JsonProperty("mobilephone")
protected String mobilephone;
@JsonProperty("address3_shippingmethodcode")
protected Integer address3_shippingmethodcode;
@JsonProperty("address3_postalcode")
protected String address3_postalcode;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("annualincome")
protected BigDecimal annualincome;
@JsonProperty("fax")
protected String fax;
@JsonProperty("telephone3")
protected String telephone3;
@JsonProperty("preferredappointmentdaycode")
protected Integer preferredappointmentdaycode;
@JsonProperty("address3_city")
protected String address3_city;
@JsonProperty("lastonholdtime")
protected OffsetDateTime lastonholdtime;
@JsonProperty("address2_stateorprovince")
protected String address2_stateorprovince;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("address2_line1")
protected String address2_line1;
@JsonProperty("assistantphone")
protected String assistantphone;
@JsonProperty("lastusedincampaign")
protected OffsetDateTime lastusedincampaign;
@JsonProperty("address3_freighttermscode")
protected Integer address3_freighttermscode;
@JsonProperty("pager")
protected String pager;
@JsonProperty("employeeid")
protected String employeeid;
@JsonProperty("territorycode")
protected Integer territorycode;
@JsonProperty("_parentcustomerid_value")
protected String _parentcustomerid_value;
@JsonProperty("managername")
protected String managername;
@JsonProperty("birthdate")
protected LocalDate birthdate;
@JsonProperty("address1_name")
protected String address1_name;
@JsonProperty("department")
protected String department;
@JsonProperty("address3_country")
protected String address3_country;
@JsonProperty("address2_telephone1")
protected String address2_telephone1;
@JsonProperty("address1_postofficebox")
protected String address1_postofficebox;
@JsonProperty("address2_primarycontactname")
protected String address2_primarycontactname;
@JsonProperty("address2_latitude")
protected Double address2_latitude;
@JsonProperty("_owningteam_value")
protected String _owningteam_value;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("home2")
protected String home2;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("address2_postalcode")
protected String address2_postalcode;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("merged")
protected Boolean merged;
@JsonProperty("_masterid_value")
protected String _masterid_value;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("address3_postofficebox")
protected String address3_postofficebox;
@JsonProperty("customertypecode")
protected Integer customertypecode;
@JsonProperty("business2")
protected String business2;
@JsonProperty("address3_county")
protected String address3_county;
@JsonProperty("address1_latitude")
protected Double address1_latitude;
@JsonProperty("address1_freighttermscode")
protected Integer address1_freighttermscode;
@JsonProperty("traversedpath")
protected String traversedpath;
@JsonProperty("address3_addresstypecode")
protected Integer address3_addresstypecode;
@JsonProperty("address1_longitude")
protected Double address1_longitude;
@JsonProperty("address1_addresstypecode")
protected Integer address1_addresstypecode;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("yomifullname")
protected String yomifullname;
@JsonProperty("aging90_base")
protected BigDecimal aging90_base;
@JsonProperty("_accountid_value")
protected String _accountid_value;
@JsonProperty("address3_primarycontactname")
protected String address3_primarycontactname;
@JsonProperty("familystatuscode")
protected Integer familystatuscode;
@JsonProperty("address3_addressid")
protected String address3_addressid;
@JsonProperty("leadsourcecode")
protected Integer leadsourcecode;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("address2_freighttermscode")
protected Integer address2_freighttermscode;
@JsonProperty("address1_composite")
protected String address1_composite;
@JsonProperty("telephone2")
protected String telephone2;
@JsonProperty("yomimiddlename")
protected String yomimiddlename;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("jobtitle")
protected String jobtitle;
@JsonProperty("address2_addresstypecode")
protected Integer address2_addresstypecode;
@JsonProperty("educationcode")
protected Integer educationcode;
@JsonProperty("address1_telephone3")
protected String address1_telephone3;
@JsonProperty("followemail")
protected Boolean followemail;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("gendercode")
protected Integer gendercode;
@JsonProperty("address2_line2")
protected String address2_line2;
@JsonProperty("creditlimit_base")
protected BigDecimal creditlimit_base;
@JsonProperty("annualincome_base")
protected BigDecimal annualincome_base;
@JsonProperty("donotfax")
protected Boolean donotfax;
@JsonProperty("address3_line1")
protected String address3_line1;
@JsonProperty("address1_county")
protected String address1_county;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("_createdbyexternalparty_value")
protected String _createdbyexternalparty_value;
@JsonProperty("entityimageid")
protected String entityimageid;
@JsonProperty("processid")
protected String processid;
@JsonProperty("address1_telephone2")
protected String address1_telephone2;
@JsonProperty("description")
protected String description;
@JsonProperty("address1_fax")
protected String address1_fax;
@JsonProperty("marketingonly")
protected Boolean marketingonly;
@JsonProperty("address3_line2")
protected String address3_line2;
@JsonProperty("address1_addressid")
protected String address1_addressid;
@JsonProperty("donotpostalmail")
protected Boolean donotpostalmail;
@JsonProperty("address2_utcoffset")
protected Integer address2_utcoffset;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("aging30_base")
protected BigDecimal aging30_base;
@JsonProperty("callback")
protected String callback;
@JsonProperty("emailaddress2")
protected String emailaddress2;
@JsonProperty("address2_line3")
protected String address2_line3;
@JsonProperty("managerphone")
protected String managerphone;
@JsonProperty("websiteurl")
protected String websiteurl;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("firstname")
protected String firstname;
@JsonProperty("address1_telephone1")
protected String address1_telephone1;
@JsonProperty("address3_composite")
protected String address3_composite;
@JsonProperty("address3_fax")
protected String address3_fax;
@JsonProperty("childrensnames")
protected String childrensnames;
@JsonProperty("preferredcontactmethodcode")
protected Integer preferredcontactmethodcode;
@JsonProperty("numberofchildren")
protected Integer numberofchildren;
@JsonProperty("subscriptionid")
protected String subscriptionid;
@JsonProperty("yomilastname")
protected String yomilastname;
@JsonProperty("address2_postofficebox")
protected String address2_postofficebox;
@JsonProperty("aging90")
protected BigDecimal aging90;
@JsonProperty("donotbulkpostalmail")
protected Boolean donotbulkpostalmail;
@JsonProperty("emailaddress1")
protected String emailaddress1;
@JsonProperty("donotbulkemail")
protected Boolean donotbulkemail;
@JsonProperty("customersizecode")
protected Integer customersizecode;
@JsonProperty("address1_city")
protected String address1_city;
@JsonProperty("preferredappointmenttimecode")
protected Integer preferredappointmenttimecode;
@JsonProperty("address3_latitude")
protected Double address3_latitude;
@JsonProperty("aging60_base")
protected BigDecimal aging60_base;
@JsonProperty("_transactioncurrencyid_value")
protected String _transactioncurrencyid_value;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("_modifiedbyexternalparty_value")
protected String _modifiedbyexternalparty_value;
@JsonProperty("paymenttermscode")
protected Integer paymenttermscode;
@JsonProperty("address3_name")
protected String address3_name;
@JsonProperty("participatesinworkflow")
protected Boolean participatesinworkflow;
@JsonProperty("address1_shippingmethodcode")
protected Integer address1_shippingmethodcode;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("ftpsiteurl")
protected String ftpsiteurl;
@JsonProperty("_preferredsystemuserid_value")
protected String _preferredsystemuserid_value;
@JsonProperty("address2_telephone2")
protected String address2_telephone2;
@JsonProperty("_slainvokedid_value")
protected String _slainvokedid_value;
@JsonProperty("address3_utcoffset")
protected Integer address3_utcoffset;
@JsonProperty("address3_stateorprovince")
protected String address3_stateorprovince;
@JsonProperty("address3_telephone1")
protected String address3_telephone1;
@JsonProperty("nickname")
protected String nickname;
@JsonProperty("fullname")
protected String fullname;
@JsonProperty("assistantname")
protected String assistantname;
@JsonProperty("address2_country")
protected String address2_country;
@JsonProperty("aging60")
protected BigDecimal aging60;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("externaluseridentifier")
protected String externaluseridentifier;
@JsonProperty("address2_name")
protected String address2_name;
@JsonProperty("stageid")
protected String stageid;
@JsonProperty("creditonhold")
protected Boolean creditonhold;
@JsonProperty("address3_longitude")
protected Double address3_longitude;
@JsonProperty("onholdtime")
protected Integer onholdtime;
@JsonProperty("address2_telephone3")
protected String address2_telephone3;
@JsonProperty("donotphone")
protected Boolean donotphone;
@JsonProperty("address3_upszone")
protected String address3_upszone;
@JsonProperty("contactid")
protected String contactid;
@JsonProperty("aging30")
protected BigDecimal aging30;
@JsonProperty("address2_upszone")
protected String address2_upszone;
@JsonProperty("address1_upszone")
protected String address1_upszone;
@JsonProperty("creditlimit")
protected BigDecimal creditlimit;
@JsonProperty("accountrolecode")
protected Integer accountrolecode;
@JsonProperty("salutation")
protected String salutation;
@JsonProperty("suffix")
protected String suffix;
@JsonProperty("address1_primarycontactname")
protected String address1_primarycontactname;
@JsonProperty("address1_utcoffset")
protected Integer address1_utcoffset;
@JsonProperty("address1_postalcode")
protected String address1_postalcode;
@JsonProperty("governmentid")
protected String governmentid;
@JsonProperty("donotsendmm")
protected Boolean donotsendmm;
@JsonProperty("address1_country")
protected String address1_country;
@JsonProperty("lastname")
protected String lastname;
@JsonProperty("address2_city")
protected String address2_city;
@JsonProperty("donotemail")
protected Boolean donotemail;
@JsonProperty("company")
protected String company;
@JsonProperty("address1_line2")
protected String address1_line2;
@JsonProperty("address2_longitude")
protected Double address2_longitude;
@JsonProperty("address1_stateorprovince")
protected String address1_stateorprovince;
@JsonProperty("yomifirstname")
protected String yomifirstname;
@JsonProperty("telephone1")
protected String telephone1;
@JsonProperty("address1_line1")
protected String address1_line1;
@JsonProperty("address2_addressid")
protected String address2_addressid;
@JsonProperty("isbackofficecustomer")
protected Boolean isbackofficecustomer;
@JsonProperty("address2_county")
protected String address2_county;
@JsonProperty("shippingmethodcode")
protected Integer shippingmethodcode;
@JsonProperty("anniversary")
protected LocalDate anniversary;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("_parentcontactid_value")
protected String _parentcontactid_value;
@JsonProperty("haschildrencode")
protected Integer haschildrencode;
@JsonProperty("address2_fax")
protected String address2_fax;
@JsonProperty("address2_composite")
protected String address2_composite;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("address1_line3")
protected String address1_line3;
@JsonProperty("_slaid_value")
protected String _slaid_value;
@JsonProperty("middlename")
protected String middlename;
@JsonProperty("address3_line3")
protected String address3_line3;
@JsonProperty("address3_telephone2")
protected String address3_telephone2;
@JsonProperty("timespentbymeonemailandmeetings")
protected String timespentbymeonemailandmeetings;
protected Contact() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderContact() {
return new Builder();
}
public static final class Builder {
private String spousesname;
private String emailaddress3;
private String address3_telephone3;
private Long entityimage_timestamp;
private Integer address2_shippingmethodcode;
private String mobilephone;
private Integer address3_shippingmethodcode;
private String address3_postalcode;
private Long versionnumber;
private BigDecimal annualincome;
private String fax;
private String telephone3;
private Integer preferredappointmentdaycode;
private String address3_city;
private OffsetDateTime lastonholdtime;
private String address2_stateorprovince;
private String _createdby_value;
private String address2_line1;
private String assistantphone;
private OffsetDateTime lastusedincampaign;
private Integer address3_freighttermscode;
private String pager;
private String employeeid;
private Integer territorycode;
private String _parentcustomerid_value;
private String managername;
private LocalDate birthdate;
private String address1_name;
private String department;
private String address3_country;
private String address2_telephone1;
private String address1_postofficebox;
private String address2_primarycontactname;
private Double address2_latitude;
private String _owningteam_value;
private Integer timezoneruleversionnumber;
private String home2;
private Integer utcconversiontimezonecode;
private String address2_postalcode;
private String _owninguser_value;
private Boolean merged;
private String _masterid_value;
private String _createdonbehalfby_value;
private String address3_postofficebox;
private Integer customertypecode;
private String business2;
private String address3_county;
private Double address1_latitude;
private Integer address1_freighttermscode;
private String traversedpath;
private Integer address3_addresstypecode;
private Double address1_longitude;
private Integer address1_addresstypecode;
private Integer statuscode;
private String yomifullname;
private BigDecimal aging90_base;
private String _accountid_value;
private String address3_primarycontactname;
private Integer familystatuscode;
private String address3_addressid;
private Integer leadsourcecode;
private Integer statecode;
private Integer address2_freighttermscode;
private String address1_composite;
private String telephone2;
private String yomimiddlename;
private String _modifiedonbehalfby_value;
private String jobtitle;
private Integer address2_addresstypecode;
private Integer educationcode;
private String address1_telephone3;
private Boolean followemail;
private Integer importsequencenumber;
private Integer gendercode;
private String address2_line2;
private BigDecimal creditlimit_base;
private BigDecimal annualincome_base;
private Boolean donotfax;
private String address3_line1;
private String address1_county;
private String _owningbusinessunit_value;
private String _createdbyexternalparty_value;
private String entityimageid;
private String processid;
private String address1_telephone2;
private String description;
private String address1_fax;
private Boolean marketingonly;
private String address3_line2;
private String address1_addressid;
private Boolean donotpostalmail;
private Integer address2_utcoffset;
private BigDecimal exchangerate;
private BigDecimal aging30_base;
private String callback;
private String emailaddress2;
private String address2_line3;
private String managerphone;
private String websiteurl;
private OffsetDateTime overriddencreatedon;
private String firstname;
private String address1_telephone1;
private String address3_composite;
private String address3_fax;
private String childrensnames;
private Integer preferredcontactmethodcode;
private Integer numberofchildren;
private String subscriptionid;
private String yomilastname;
private String address2_postofficebox;
private BigDecimal aging90;
private Boolean donotbulkpostalmail;
private String emailaddress1;
private Boolean donotbulkemail;
private Integer customersizecode;
private String address1_city;
private Integer preferredappointmenttimecode;
private Double address3_latitude;
private BigDecimal aging60_base;
private String _transactioncurrencyid_value;
private byte[] entityimage;
private String _modifiedbyexternalparty_value;
private Integer paymenttermscode;
private String address3_name;
private Boolean participatesinworkflow;
private Integer address1_shippingmethodcode;
private String _modifiedby_value;
private String ftpsiteurl;
private String _preferredsystemuserid_value;
private String address2_telephone2;
private String _slainvokedid_value;
private Integer address3_utcoffset;
private String address3_stateorprovince;
private String address3_telephone1;
private String nickname;
private String fullname;
private String assistantname;
private String address2_country;
private BigDecimal aging60;
private OffsetDateTime modifiedon;
private String externaluseridentifier;
private String address2_name;
private String stageid;
private Boolean creditonhold;
private Double address3_longitude;
private Integer onholdtime;
private String address2_telephone3;
private Boolean donotphone;
private String address3_upszone;
private String contactid;
private BigDecimal aging30;
private String address2_upszone;
private String address1_upszone;
private BigDecimal creditlimit;
private Integer accountrolecode;
private String salutation;
private String suffix;
private String address1_primarycontactname;
private Integer address1_utcoffset;
private String address1_postalcode;
private String governmentid;
private Boolean donotsendmm;
private String address1_country;
private String lastname;
private String address2_city;
private Boolean donotemail;
private String company;
private String address1_line2;
private Double address2_longitude;
private String address1_stateorprovince;
private String yomifirstname;
private String telephone1;
private String address1_line1;
private String address2_addressid;
private Boolean isbackofficecustomer;
private String address2_county;
private Integer shippingmethodcode;
private LocalDate anniversary;
private String _ownerid_value;
private String _parentcontactid_value;
private Integer haschildrencode;
private String address2_fax;
private String address2_composite;
private OffsetDateTime createdon;
private String entityimage_url;
private String address1_line3;
private String _slaid_value;
private String middlename;
private String address3_line3;
private String address3_telephone2;
private String timespentbymeonemailandmeetings;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder spousesname(String spousesname) {
this.spousesname = spousesname;
this.changedFields = changedFields.add("spousesname");
return this;
}
public Builder emailaddress3(String emailaddress3) {
this.emailaddress3 = emailaddress3;
this.changedFields = changedFields.add("emailaddress3");
return this;
}
public Builder address3_telephone3(String address3_telephone3) {
this.address3_telephone3 = address3_telephone3;
this.changedFields = changedFields.add("address3_telephone3");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder address2_shippingmethodcode(Integer address2_shippingmethodcode) {
this.address2_shippingmethodcode = address2_shippingmethodcode;
this.changedFields = changedFields.add("address2_shippingmethodcode");
return this;
}
public Builder mobilephone(String mobilephone) {
this.mobilephone = mobilephone;
this.changedFields = changedFields.add("mobilephone");
return this;
}
public Builder address3_shippingmethodcode(Integer address3_shippingmethodcode) {
this.address3_shippingmethodcode = address3_shippingmethodcode;
this.changedFields = changedFields.add("address3_shippingmethodcode");
return this;
}
public Builder address3_postalcode(String address3_postalcode) {
this.address3_postalcode = address3_postalcode;
this.changedFields = changedFields.add("address3_postalcode");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder annualincome(BigDecimal annualincome) {
this.annualincome = annualincome;
this.changedFields = changedFields.add("annualincome");
return this;
}
public Builder fax(String fax) {
this.fax = fax;
this.changedFields = changedFields.add("fax");
return this;
}
public Builder telephone3(String telephone3) {
this.telephone3 = telephone3;
this.changedFields = changedFields.add("telephone3");
return this;
}
public Builder preferredappointmentdaycode(Integer preferredappointmentdaycode) {
this.preferredappointmentdaycode = preferredappointmentdaycode;
this.changedFields = changedFields.add("preferredappointmentdaycode");
return this;
}
public Builder address3_city(String address3_city) {
this.address3_city = address3_city;
this.changedFields = changedFields.add("address3_city");
return this;
}
public Builder lastonholdtime(OffsetDateTime lastonholdtime) {
this.lastonholdtime = lastonholdtime;
this.changedFields = changedFields.add("lastonholdtime");
return this;
}
public Builder address2_stateorprovince(String address2_stateorprovince) {
this.address2_stateorprovince = address2_stateorprovince;
this.changedFields = changedFields.add("address2_stateorprovince");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder address2_line1(String address2_line1) {
this.address2_line1 = address2_line1;
this.changedFields = changedFields.add("address2_line1");
return this;
}
public Builder assistantphone(String assistantphone) {
this.assistantphone = assistantphone;
this.changedFields = changedFields.add("assistantphone");
return this;
}
public Builder lastusedincampaign(OffsetDateTime lastusedincampaign) {
this.lastusedincampaign = lastusedincampaign;
this.changedFields = changedFields.add("lastusedincampaign");
return this;
}
public Builder address3_freighttermscode(Integer address3_freighttermscode) {
this.address3_freighttermscode = address3_freighttermscode;
this.changedFields = changedFields.add("address3_freighttermscode");
return this;
}
public Builder pager(String pager) {
this.pager = pager;
this.changedFields = changedFields.add("pager");
return this;
}
public Builder employeeid(String employeeid) {
this.employeeid = employeeid;
this.changedFields = changedFields.add("employeeid");
return this;
}
public Builder territorycode(Integer territorycode) {
this.territorycode = territorycode;
this.changedFields = changedFields.add("territorycode");
return this;
}
public Builder _parentcustomerid_value(String _parentcustomerid_value) {
this._parentcustomerid_value = _parentcustomerid_value;
this.changedFields = changedFields.add("_parentcustomerid_value");
return this;
}
public Builder managername(String managername) {
this.managername = managername;
this.changedFields = changedFields.add("managername");
return this;
}
public Builder birthdate(LocalDate birthdate) {
this.birthdate = birthdate;
this.changedFields = changedFields.add("birthdate");
return this;
}
public Builder address1_name(String address1_name) {
this.address1_name = address1_name;
this.changedFields = changedFields.add("address1_name");
return this;
}
public Builder department(String department) {
this.department = department;
this.changedFields = changedFields.add("department");
return this;
}
public Builder address3_country(String address3_country) {
this.address3_country = address3_country;
this.changedFields = changedFields.add("address3_country");
return this;
}
public Builder address2_telephone1(String address2_telephone1) {
this.address2_telephone1 = address2_telephone1;
this.changedFields = changedFields.add("address2_telephone1");
return this;
}
public Builder address1_postofficebox(String address1_postofficebox) {
this.address1_postofficebox = address1_postofficebox;
this.changedFields = changedFields.add("address1_postofficebox");
return this;
}
public Builder address2_primarycontactname(String address2_primarycontactname) {
this.address2_primarycontactname = address2_primarycontactname;
this.changedFields = changedFields.add("address2_primarycontactname");
return this;
}
public Builder address2_latitude(Double address2_latitude) {
this.address2_latitude = address2_latitude;
this.changedFields = changedFields.add("address2_latitude");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder home2(String home2) {
this.home2 = home2;
this.changedFields = changedFields.add("home2");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder address2_postalcode(String address2_postalcode) {
this.address2_postalcode = address2_postalcode;
this.changedFields = changedFields.add("address2_postalcode");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder merged(Boolean merged) {
this.merged = merged;
this.changedFields = changedFields.add("merged");
return this;
}
public Builder _masterid_value(String _masterid_value) {
this._masterid_value = _masterid_value;
this.changedFields = changedFields.add("_masterid_value");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder address3_postofficebox(String address3_postofficebox) {
this.address3_postofficebox = address3_postofficebox;
this.changedFields = changedFields.add("address3_postofficebox");
return this;
}
public Builder customertypecode(Integer customertypecode) {
this.customertypecode = customertypecode;
this.changedFields = changedFields.add("customertypecode");
return this;
}
public Builder business2(String business2) {
this.business2 = business2;
this.changedFields = changedFields.add("business2");
return this;
}
public Builder address3_county(String address3_county) {
this.address3_county = address3_county;
this.changedFields = changedFields.add("address3_county");
return this;
}
public Builder address1_latitude(Double address1_latitude) {
this.address1_latitude = address1_latitude;
this.changedFields = changedFields.add("address1_latitude");
return this;
}
public Builder address1_freighttermscode(Integer address1_freighttermscode) {
this.address1_freighttermscode = address1_freighttermscode;
this.changedFields = changedFields.add("address1_freighttermscode");
return this;
}
public Builder traversedpath(String traversedpath) {
this.traversedpath = traversedpath;
this.changedFields = changedFields.add("traversedpath");
return this;
}
public Builder address3_addresstypecode(Integer address3_addresstypecode) {
this.address3_addresstypecode = address3_addresstypecode;
this.changedFields = changedFields.add("address3_addresstypecode");
return this;
}
public Builder address1_longitude(Double address1_longitude) {
this.address1_longitude = address1_longitude;
this.changedFields = changedFields.add("address1_longitude");
return this;
}
public Builder address1_addresstypecode(Integer address1_addresstypecode) {
this.address1_addresstypecode = address1_addresstypecode;
this.changedFields = changedFields.add("address1_addresstypecode");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder yomifullname(String yomifullname) {
this.yomifullname = yomifullname;
this.changedFields = changedFields.add("yomifullname");
return this;
}
public Builder aging90_base(BigDecimal aging90_base) {
this.aging90_base = aging90_base;
this.changedFields = changedFields.add("aging90_base");
return this;
}
public Builder _accountid_value(String _accountid_value) {
this._accountid_value = _accountid_value;
this.changedFields = changedFields.add("_accountid_value");
return this;
}
public Builder address3_primarycontactname(String address3_primarycontactname) {
this.address3_primarycontactname = address3_primarycontactname;
this.changedFields = changedFields.add("address3_primarycontactname");
return this;
}
public Builder familystatuscode(Integer familystatuscode) {
this.familystatuscode = familystatuscode;
this.changedFields = changedFields.add("familystatuscode");
return this;
}
public Builder address3_addressid(String address3_addressid) {
this.address3_addressid = address3_addressid;
this.changedFields = changedFields.add("address3_addressid");
return this;
}
public Builder leadsourcecode(Integer leadsourcecode) {
this.leadsourcecode = leadsourcecode;
this.changedFields = changedFields.add("leadsourcecode");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder address2_freighttermscode(Integer address2_freighttermscode) {
this.address2_freighttermscode = address2_freighttermscode;
this.changedFields = changedFields.add("address2_freighttermscode");
return this;
}
public Builder address1_composite(String address1_composite) {
this.address1_composite = address1_composite;
this.changedFields = changedFields.add("address1_composite");
return this;
}
public Builder telephone2(String telephone2) {
this.telephone2 = telephone2;
this.changedFields = changedFields.add("telephone2");
return this;
}
public Builder yomimiddlename(String yomimiddlename) {
this.yomimiddlename = yomimiddlename;
this.changedFields = changedFields.add("yomimiddlename");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder jobtitle(String jobtitle) {
this.jobtitle = jobtitle;
this.changedFields = changedFields.add("jobtitle");
return this;
}
public Builder address2_addresstypecode(Integer address2_addresstypecode) {
this.address2_addresstypecode = address2_addresstypecode;
this.changedFields = changedFields.add("address2_addresstypecode");
return this;
}
public Builder educationcode(Integer educationcode) {
this.educationcode = educationcode;
this.changedFields = changedFields.add("educationcode");
return this;
}
public Builder address1_telephone3(String address1_telephone3) {
this.address1_telephone3 = address1_telephone3;
this.changedFields = changedFields.add("address1_telephone3");
return this;
}
public Builder followemail(Boolean followemail) {
this.followemail = followemail;
this.changedFields = changedFields.add("followemail");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder gendercode(Integer gendercode) {
this.gendercode = gendercode;
this.changedFields = changedFields.add("gendercode");
return this;
}
public Builder address2_line2(String address2_line2) {
this.address2_line2 = address2_line2;
this.changedFields = changedFields.add("address2_line2");
return this;
}
public Builder creditlimit_base(BigDecimal creditlimit_base) {
this.creditlimit_base = creditlimit_base;
this.changedFields = changedFields.add("creditlimit_base");
return this;
}
public Builder annualincome_base(BigDecimal annualincome_base) {
this.annualincome_base = annualincome_base;
this.changedFields = changedFields.add("annualincome_base");
return this;
}
public Builder donotfax(Boolean donotfax) {
this.donotfax = donotfax;
this.changedFields = changedFields.add("donotfax");
return this;
}
public Builder address3_line1(String address3_line1) {
this.address3_line1 = address3_line1;
this.changedFields = changedFields.add("address3_line1");
return this;
}
public Builder address1_county(String address1_county) {
this.address1_county = address1_county;
this.changedFields = changedFields.add("address1_county");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder _createdbyexternalparty_value(String _createdbyexternalparty_value) {
this._createdbyexternalparty_value = _createdbyexternalparty_value;
this.changedFields = changedFields.add("_createdbyexternalparty_value");
return this;
}
public Builder entityimageid(String entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder processid(String processid) {
this.processid = processid;
this.changedFields = changedFields.add("processid");
return this;
}
public Builder address1_telephone2(String address1_telephone2) {
this.address1_telephone2 = address1_telephone2;
this.changedFields = changedFields.add("address1_telephone2");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder address1_fax(String address1_fax) {
this.address1_fax = address1_fax;
this.changedFields = changedFields.add("address1_fax");
return this;
}
public Builder marketingonly(Boolean marketingonly) {
this.marketingonly = marketingonly;
this.changedFields = changedFields.add("marketingonly");
return this;
}
public Builder address3_line2(String address3_line2) {
this.address3_line2 = address3_line2;
this.changedFields = changedFields.add("address3_line2");
return this;
}
public Builder address1_addressid(String address1_addressid) {
this.address1_addressid = address1_addressid;
this.changedFields = changedFields.add("address1_addressid");
return this;
}
public Builder donotpostalmail(Boolean donotpostalmail) {
this.donotpostalmail = donotpostalmail;
this.changedFields = changedFields.add("donotpostalmail");
return this;
}
public Builder address2_utcoffset(Integer address2_utcoffset) {
this.address2_utcoffset = address2_utcoffset;
this.changedFields = changedFields.add("address2_utcoffset");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder aging30_base(BigDecimal aging30_base) {
this.aging30_base = aging30_base;
this.changedFields = changedFields.add("aging30_base");
return this;
}
public Builder callback(String callback) {
this.callback = callback;
this.changedFields = changedFields.add("callback");
return this;
}
public Builder emailaddress2(String emailaddress2) {
this.emailaddress2 = emailaddress2;
this.changedFields = changedFields.add("emailaddress2");
return this;
}
public Builder address2_line3(String address2_line3) {
this.address2_line3 = address2_line3;
this.changedFields = changedFields.add("address2_line3");
return this;
}
public Builder managerphone(String managerphone) {
this.managerphone = managerphone;
this.changedFields = changedFields.add("managerphone");
return this;
}
public Builder websiteurl(String websiteurl) {
this.websiteurl = websiteurl;
this.changedFields = changedFields.add("websiteurl");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder firstname(String firstname) {
this.firstname = firstname;
this.changedFields = changedFields.add("firstname");
return this;
}
public Builder address1_telephone1(String address1_telephone1) {
this.address1_telephone1 = address1_telephone1;
this.changedFields = changedFields.add("address1_telephone1");
return this;
}
public Builder address3_composite(String address3_composite) {
this.address3_composite = address3_composite;
this.changedFields = changedFields.add("address3_composite");
return this;
}
public Builder address3_fax(String address3_fax) {
this.address3_fax = address3_fax;
this.changedFields = changedFields.add("address3_fax");
return this;
}
public Builder childrensnames(String childrensnames) {
this.childrensnames = childrensnames;
this.changedFields = changedFields.add("childrensnames");
return this;
}
public Builder preferredcontactmethodcode(Integer preferredcontactmethodcode) {
this.preferredcontactmethodcode = preferredcontactmethodcode;
this.changedFields = changedFields.add("preferredcontactmethodcode");
return this;
}
public Builder numberofchildren(Integer numberofchildren) {
this.numberofchildren = numberofchildren;
this.changedFields = changedFields.add("numberofchildren");
return this;
}
public Builder subscriptionid(String subscriptionid) {
this.subscriptionid = subscriptionid;
this.changedFields = changedFields.add("subscriptionid");
return this;
}
public Builder yomilastname(String yomilastname) {
this.yomilastname = yomilastname;
this.changedFields = changedFields.add("yomilastname");
return this;
}
public Builder address2_postofficebox(String address2_postofficebox) {
this.address2_postofficebox = address2_postofficebox;
this.changedFields = changedFields.add("address2_postofficebox");
return this;
}
public Builder aging90(BigDecimal aging90) {
this.aging90 = aging90;
this.changedFields = changedFields.add("aging90");
return this;
}
public Builder donotbulkpostalmail(Boolean donotbulkpostalmail) {
this.donotbulkpostalmail = donotbulkpostalmail;
this.changedFields = changedFields.add("donotbulkpostalmail");
return this;
}
public Builder emailaddress1(String emailaddress1) {
this.emailaddress1 = emailaddress1;
this.changedFields = changedFields.add("emailaddress1");
return this;
}
public Builder donotbulkemail(Boolean donotbulkemail) {
this.donotbulkemail = donotbulkemail;
this.changedFields = changedFields.add("donotbulkemail");
return this;
}
public Builder customersizecode(Integer customersizecode) {
this.customersizecode = customersizecode;
this.changedFields = changedFields.add("customersizecode");
return this;
}
public Builder address1_city(String address1_city) {
this.address1_city = address1_city;
this.changedFields = changedFields.add("address1_city");
return this;
}
public Builder preferredappointmenttimecode(Integer preferredappointmenttimecode) {
this.preferredappointmenttimecode = preferredappointmenttimecode;
this.changedFields = changedFields.add("preferredappointmenttimecode");
return this;
}
public Builder address3_latitude(Double address3_latitude) {
this.address3_latitude = address3_latitude;
this.changedFields = changedFields.add("address3_latitude");
return this;
}
public Builder aging60_base(BigDecimal aging60_base) {
this.aging60_base = aging60_base;
this.changedFields = changedFields.add("aging60_base");
return this;
}
public Builder _transactioncurrencyid_value(String _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder _modifiedbyexternalparty_value(String _modifiedbyexternalparty_value) {
this._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
this.changedFields = changedFields.add("_modifiedbyexternalparty_value");
return this;
}
public Builder paymenttermscode(Integer paymenttermscode) {
this.paymenttermscode = paymenttermscode;
this.changedFields = changedFields.add("paymenttermscode");
return this;
}
public Builder address3_name(String address3_name) {
this.address3_name = address3_name;
this.changedFields = changedFields.add("address3_name");
return this;
}
public Builder participatesinworkflow(Boolean participatesinworkflow) {
this.participatesinworkflow = participatesinworkflow;
this.changedFields = changedFields.add("participatesinworkflow");
return this;
}
public Builder address1_shippingmethodcode(Integer address1_shippingmethodcode) {
this.address1_shippingmethodcode = address1_shippingmethodcode;
this.changedFields = changedFields.add("address1_shippingmethodcode");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder ftpsiteurl(String ftpsiteurl) {
this.ftpsiteurl = ftpsiteurl;
this.changedFields = changedFields.add("ftpsiteurl");
return this;
}
public Builder _preferredsystemuserid_value(String _preferredsystemuserid_value) {
this._preferredsystemuserid_value = _preferredsystemuserid_value;
this.changedFields = changedFields.add("_preferredsystemuserid_value");
return this;
}
public Builder address2_telephone2(String address2_telephone2) {
this.address2_telephone2 = address2_telephone2;
this.changedFields = changedFields.add("address2_telephone2");
return this;
}
public Builder _slainvokedid_value(String _slainvokedid_value) {
this._slainvokedid_value = _slainvokedid_value;
this.changedFields = changedFields.add("_slainvokedid_value");
return this;
}
public Builder address3_utcoffset(Integer address3_utcoffset) {
this.address3_utcoffset = address3_utcoffset;
this.changedFields = changedFields.add("address3_utcoffset");
return this;
}
public Builder address3_stateorprovince(String address3_stateorprovince) {
this.address3_stateorprovince = address3_stateorprovince;
this.changedFields = changedFields.add("address3_stateorprovince");
return this;
}
public Builder address3_telephone1(String address3_telephone1) {
this.address3_telephone1 = address3_telephone1;
this.changedFields = changedFields.add("address3_telephone1");
return this;
}
public Builder nickname(String nickname) {
this.nickname = nickname;
this.changedFields = changedFields.add("nickname");
return this;
}
public Builder fullname(String fullname) {
this.fullname = fullname;
this.changedFields = changedFields.add("fullname");
return this;
}
public Builder assistantname(String assistantname) {
this.assistantname = assistantname;
this.changedFields = changedFields.add("assistantname");
return this;
}
public Builder address2_country(String address2_country) {
this.address2_country = address2_country;
this.changedFields = changedFields.add("address2_country");
return this;
}
public Builder aging60(BigDecimal aging60) {
this.aging60 = aging60;
this.changedFields = changedFields.add("aging60");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder externaluseridentifier(String externaluseridentifier) {
this.externaluseridentifier = externaluseridentifier;
this.changedFields = changedFields.add("externaluseridentifier");
return this;
}
public Builder address2_name(String address2_name) {
this.address2_name = address2_name;
this.changedFields = changedFields.add("address2_name");
return this;
}
public Builder stageid(String stageid) {
this.stageid = stageid;
this.changedFields = changedFields.add("stageid");
return this;
}
public Builder creditonhold(Boolean creditonhold) {
this.creditonhold = creditonhold;
this.changedFields = changedFields.add("creditonhold");
return this;
}
public Builder address3_longitude(Double address3_longitude) {
this.address3_longitude = address3_longitude;
this.changedFields = changedFields.add("address3_longitude");
return this;
}
public Builder onholdtime(Integer onholdtime) {
this.onholdtime = onholdtime;
this.changedFields = changedFields.add("onholdtime");
return this;
}
public Builder address2_telephone3(String address2_telephone3) {
this.address2_telephone3 = address2_telephone3;
this.changedFields = changedFields.add("address2_telephone3");
return this;
}
public Builder donotphone(Boolean donotphone) {
this.donotphone = donotphone;
this.changedFields = changedFields.add("donotphone");
return this;
}
public Builder address3_upszone(String address3_upszone) {
this.address3_upszone = address3_upszone;
this.changedFields = changedFields.add("address3_upszone");
return this;
}
public Builder contactid(String contactid) {
this.contactid = contactid;
this.changedFields = changedFields.add("contactid");
return this;
}
public Builder aging30(BigDecimal aging30) {
this.aging30 = aging30;
this.changedFields = changedFields.add("aging30");
return this;
}
public Builder address2_upszone(String address2_upszone) {
this.address2_upszone = address2_upszone;
this.changedFields = changedFields.add("address2_upszone");
return this;
}
public Builder address1_upszone(String address1_upszone) {
this.address1_upszone = address1_upszone;
this.changedFields = changedFields.add("address1_upszone");
return this;
}
public Builder creditlimit(BigDecimal creditlimit) {
this.creditlimit = creditlimit;
this.changedFields = changedFields.add("creditlimit");
return this;
}
public Builder accountrolecode(Integer accountrolecode) {
this.accountrolecode = accountrolecode;
this.changedFields = changedFields.add("accountrolecode");
return this;
}
public Builder salutation(String salutation) {
this.salutation = salutation;
this.changedFields = changedFields.add("salutation");
return this;
}
public Builder suffix(String suffix) {
this.suffix = suffix;
this.changedFields = changedFields.add("suffix");
return this;
}
public Builder address1_primarycontactname(String address1_primarycontactname) {
this.address1_primarycontactname = address1_primarycontactname;
this.changedFields = changedFields.add("address1_primarycontactname");
return this;
}
public Builder address1_utcoffset(Integer address1_utcoffset) {
this.address1_utcoffset = address1_utcoffset;
this.changedFields = changedFields.add("address1_utcoffset");
return this;
}
public Builder address1_postalcode(String address1_postalcode) {
this.address1_postalcode = address1_postalcode;
this.changedFields = changedFields.add("address1_postalcode");
return this;
}
public Builder governmentid(String governmentid) {
this.governmentid = governmentid;
this.changedFields = changedFields.add("governmentid");
return this;
}
public Builder donotsendmm(Boolean donotsendmm) {
this.donotsendmm = donotsendmm;
this.changedFields = changedFields.add("donotsendmm");
return this;
}
public Builder address1_country(String address1_country) {
this.address1_country = address1_country;
this.changedFields = changedFields.add("address1_country");
return this;
}
public Builder lastname(String lastname) {
this.lastname = lastname;
this.changedFields = changedFields.add("lastname");
return this;
}
public Builder address2_city(String address2_city) {
this.address2_city = address2_city;
this.changedFields = changedFields.add("address2_city");
return this;
}
public Builder donotemail(Boolean donotemail) {
this.donotemail = donotemail;
this.changedFields = changedFields.add("donotemail");
return this;
}
public Builder company(String company) {
this.company = company;
this.changedFields = changedFields.add("company");
return this;
}
public Builder address1_line2(String address1_line2) {
this.address1_line2 = address1_line2;
this.changedFields = changedFields.add("address1_line2");
return this;
}
public Builder address2_longitude(Double address2_longitude) {
this.address2_longitude = address2_longitude;
this.changedFields = changedFields.add("address2_longitude");
return this;
}
public Builder address1_stateorprovince(String address1_stateorprovince) {
this.address1_stateorprovince = address1_stateorprovince;
this.changedFields = changedFields.add("address1_stateorprovince");
return this;
}
public Builder yomifirstname(String yomifirstname) {
this.yomifirstname = yomifirstname;
this.changedFields = changedFields.add("yomifirstname");
return this;
}
public Builder telephone1(String telephone1) {
this.telephone1 = telephone1;
this.changedFields = changedFields.add("telephone1");
return this;
}
public Builder address1_line1(String address1_line1) {
this.address1_line1 = address1_line1;
this.changedFields = changedFields.add("address1_line1");
return this;
}
public Builder address2_addressid(String address2_addressid) {
this.address2_addressid = address2_addressid;
this.changedFields = changedFields.add("address2_addressid");
return this;
}
public Builder isbackofficecustomer(Boolean isbackofficecustomer) {
this.isbackofficecustomer = isbackofficecustomer;
this.changedFields = changedFields.add("isbackofficecustomer");
return this;
}
public Builder address2_county(String address2_county) {
this.address2_county = address2_county;
this.changedFields = changedFields.add("address2_county");
return this;
}
public Builder shippingmethodcode(Integer shippingmethodcode) {
this.shippingmethodcode = shippingmethodcode;
this.changedFields = changedFields.add("shippingmethodcode");
return this;
}
public Builder anniversary(LocalDate anniversary) {
this.anniversary = anniversary;
this.changedFields = changedFields.add("anniversary");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder _parentcontactid_value(String _parentcontactid_value) {
this._parentcontactid_value = _parentcontactid_value;
this.changedFields = changedFields.add("_parentcontactid_value");
return this;
}
public Builder haschildrencode(Integer haschildrencode) {
this.haschildrencode = haschildrencode;
this.changedFields = changedFields.add("haschildrencode");
return this;
}
public Builder address2_fax(String address2_fax) {
this.address2_fax = address2_fax;
this.changedFields = changedFields.add("address2_fax");
return this;
}
public Builder address2_composite(String address2_composite) {
this.address2_composite = address2_composite;
this.changedFields = changedFields.add("address2_composite");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder address1_line3(String address1_line3) {
this.address1_line3 = address1_line3;
this.changedFields = changedFields.add("address1_line3");
return this;
}
public Builder _slaid_value(String _slaid_value) {
this._slaid_value = _slaid_value;
this.changedFields = changedFields.add("_slaid_value");
return this;
}
public Builder middlename(String middlename) {
this.middlename = middlename;
this.changedFields = changedFields.add("middlename");
return this;
}
public Builder address3_line3(String address3_line3) {
this.address3_line3 = address3_line3;
this.changedFields = changedFields.add("address3_line3");
return this;
}
public Builder address3_telephone2(String address3_telephone2) {
this.address3_telephone2 = address3_telephone2;
this.changedFields = changedFields.add("address3_telephone2");
return this;
}
public Builder timespentbymeonemailandmeetings(String timespentbymeonemailandmeetings) {
this.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
this.changedFields = changedFields.add("timespentbymeonemailandmeetings");
return this;
}
public Contact build() {
Contact _x = new Contact();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.contact";
_x.spousesname = spousesname;
_x.emailaddress3 = emailaddress3;
_x.address3_telephone3 = address3_telephone3;
_x.entityimage_timestamp = entityimage_timestamp;
_x.address2_shippingmethodcode = address2_shippingmethodcode;
_x.mobilephone = mobilephone;
_x.address3_shippingmethodcode = address3_shippingmethodcode;
_x.address3_postalcode = address3_postalcode;
_x.versionnumber = versionnumber;
_x.annualincome = annualincome;
_x.fax = fax;
_x.telephone3 = telephone3;
_x.preferredappointmentdaycode = preferredappointmentdaycode;
_x.address3_city = address3_city;
_x.lastonholdtime = lastonholdtime;
_x.address2_stateorprovince = address2_stateorprovince;
_x._createdby_value = _createdby_value;
_x.address2_line1 = address2_line1;
_x.assistantphone = assistantphone;
_x.lastusedincampaign = lastusedincampaign;
_x.address3_freighttermscode = address3_freighttermscode;
_x.pager = pager;
_x.employeeid = employeeid;
_x.territorycode = territorycode;
_x._parentcustomerid_value = _parentcustomerid_value;
_x.managername = managername;
_x.birthdate = birthdate;
_x.address1_name = address1_name;
_x.department = department;
_x.address3_country = address3_country;
_x.address2_telephone1 = address2_telephone1;
_x.address1_postofficebox = address1_postofficebox;
_x.address2_primarycontactname = address2_primarycontactname;
_x.address2_latitude = address2_latitude;
_x._owningteam_value = _owningteam_value;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.home2 = home2;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.address2_postalcode = address2_postalcode;
_x._owninguser_value = _owninguser_value;
_x.merged = merged;
_x._masterid_value = _masterid_value;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.address3_postofficebox = address3_postofficebox;
_x.customertypecode = customertypecode;
_x.business2 = business2;
_x.address3_county = address3_county;
_x.address1_latitude = address1_latitude;
_x.address1_freighttermscode = address1_freighttermscode;
_x.traversedpath = traversedpath;
_x.address3_addresstypecode = address3_addresstypecode;
_x.address1_longitude = address1_longitude;
_x.address1_addresstypecode = address1_addresstypecode;
_x.statuscode = statuscode;
_x.yomifullname = yomifullname;
_x.aging90_base = aging90_base;
_x._accountid_value = _accountid_value;
_x.address3_primarycontactname = address3_primarycontactname;
_x.familystatuscode = familystatuscode;
_x.address3_addressid = address3_addressid;
_x.leadsourcecode = leadsourcecode;
_x.statecode = statecode;
_x.address2_freighttermscode = address2_freighttermscode;
_x.address1_composite = address1_composite;
_x.telephone2 = telephone2;
_x.yomimiddlename = yomimiddlename;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.jobtitle = jobtitle;
_x.address2_addresstypecode = address2_addresstypecode;
_x.educationcode = educationcode;
_x.address1_telephone3 = address1_telephone3;
_x.followemail = followemail;
_x.importsequencenumber = importsequencenumber;
_x.gendercode = gendercode;
_x.address2_line2 = address2_line2;
_x.creditlimit_base = creditlimit_base;
_x.annualincome_base = annualincome_base;
_x.donotfax = donotfax;
_x.address3_line1 = address3_line1;
_x.address1_county = address1_county;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._createdbyexternalparty_value = _createdbyexternalparty_value;
_x.entityimageid = entityimageid;
_x.processid = processid;
_x.address1_telephone2 = address1_telephone2;
_x.description = description;
_x.address1_fax = address1_fax;
_x.marketingonly = marketingonly;
_x.address3_line2 = address3_line2;
_x.address1_addressid = address1_addressid;
_x.donotpostalmail = donotpostalmail;
_x.address2_utcoffset = address2_utcoffset;
_x.exchangerate = exchangerate;
_x.aging30_base = aging30_base;
_x.callback = callback;
_x.emailaddress2 = emailaddress2;
_x.address2_line3 = address2_line3;
_x.managerphone = managerphone;
_x.websiteurl = websiteurl;
_x.overriddencreatedon = overriddencreatedon;
_x.firstname = firstname;
_x.address1_telephone1 = address1_telephone1;
_x.address3_composite = address3_composite;
_x.address3_fax = address3_fax;
_x.childrensnames = childrensnames;
_x.preferredcontactmethodcode = preferredcontactmethodcode;
_x.numberofchildren = numberofchildren;
_x.subscriptionid = subscriptionid;
_x.yomilastname = yomilastname;
_x.address2_postofficebox = address2_postofficebox;
_x.aging90 = aging90;
_x.donotbulkpostalmail = donotbulkpostalmail;
_x.emailaddress1 = emailaddress1;
_x.donotbulkemail = donotbulkemail;
_x.customersizecode = customersizecode;
_x.address1_city = address1_city;
_x.preferredappointmenttimecode = preferredappointmenttimecode;
_x.address3_latitude = address3_latitude;
_x.aging60_base = aging60_base;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.entityimage = entityimage;
_x._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
_x.paymenttermscode = paymenttermscode;
_x.address3_name = address3_name;
_x.participatesinworkflow = participatesinworkflow;
_x.address1_shippingmethodcode = address1_shippingmethodcode;
_x._modifiedby_value = _modifiedby_value;
_x.ftpsiteurl = ftpsiteurl;
_x._preferredsystemuserid_value = _preferredsystemuserid_value;
_x.address2_telephone2 = address2_telephone2;
_x._slainvokedid_value = _slainvokedid_value;
_x.address3_utcoffset = address3_utcoffset;
_x.address3_stateorprovince = address3_stateorprovince;
_x.address3_telephone1 = address3_telephone1;
_x.nickname = nickname;
_x.fullname = fullname;
_x.assistantname = assistantname;
_x.address2_country = address2_country;
_x.aging60 = aging60;
_x.modifiedon = modifiedon;
_x.externaluseridentifier = externaluseridentifier;
_x.address2_name = address2_name;
_x.stageid = stageid;
_x.creditonhold = creditonhold;
_x.address3_longitude = address3_longitude;
_x.onholdtime = onholdtime;
_x.address2_telephone3 = address2_telephone3;
_x.donotphone = donotphone;
_x.address3_upszone = address3_upszone;
_x.contactid = contactid;
_x.aging30 = aging30;
_x.address2_upszone = address2_upszone;
_x.address1_upszone = address1_upszone;
_x.creditlimit = creditlimit;
_x.accountrolecode = accountrolecode;
_x.salutation = salutation;
_x.suffix = suffix;
_x.address1_primarycontactname = address1_primarycontactname;
_x.address1_utcoffset = address1_utcoffset;
_x.address1_postalcode = address1_postalcode;
_x.governmentid = governmentid;
_x.donotsendmm = donotsendmm;
_x.address1_country = address1_country;
_x.lastname = lastname;
_x.address2_city = address2_city;
_x.donotemail = donotemail;
_x.company = company;
_x.address1_line2 = address1_line2;
_x.address2_longitude = address2_longitude;
_x.address1_stateorprovince = address1_stateorprovince;
_x.yomifirstname = yomifirstname;
_x.telephone1 = telephone1;
_x.address1_line1 = address1_line1;
_x.address2_addressid = address2_addressid;
_x.isbackofficecustomer = isbackofficecustomer;
_x.address2_county = address2_county;
_x.shippingmethodcode = shippingmethodcode;
_x.anniversary = anniversary;
_x._ownerid_value = _ownerid_value;
_x._parentcontactid_value = _parentcontactid_value;
_x.haschildrencode = haschildrencode;
_x.address2_fax = address2_fax;
_x.address2_composite = address2_composite;
_x.createdon = createdon;
_x.entityimage_url = entityimage_url;
_x.address1_line3 = address1_line3;
_x._slaid_value = _slaid_value;
_x.middlename = middlename;
_x.address3_line3 = address3_line3;
_x.address3_telephone2 = address3_telephone2;
_x.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && contactid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(contactid.toString()));
}
}
@Property(name="spousesname")
@JsonIgnore
public Optional getSpousesname() {
return Optional.ofNullable(spousesname);
}
public Contact withSpousesname(String spousesname) {
Checks.checkIsAscii(spousesname);
Contact _x = _copy();
_x.changedFields = changedFields.add("spousesname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.spousesname = spousesname;
return _x;
}
@Property(name="emailaddress3")
@JsonIgnore
public Optional getEmailaddress3() {
return Optional.ofNullable(emailaddress3);
}
public Contact withEmailaddress3(String emailaddress3) {
Checks.checkIsAscii(emailaddress3);
Contact _x = _copy();
_x.changedFields = changedFields.add("emailaddress3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.emailaddress3 = emailaddress3;
return _x;
}
@Property(name="address3_telephone3")
@JsonIgnore
public Optional getAddress3_telephone3() {
return Optional.ofNullable(address3_telephone3);
}
public Contact withAddress3_telephone3(String address3_telephone3) {
Checks.checkIsAscii(address3_telephone3);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_telephone3 = address3_telephone3;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Contact withEntityimage_timestamp(Long entityimage_timestamp) {
Contact _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="address2_shippingmethodcode")
@JsonIgnore
public Optional getAddress2_shippingmethodcode() {
return Optional.ofNullable(address2_shippingmethodcode);
}
public Contact withAddress2_shippingmethodcode(Integer address2_shippingmethodcode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_shippingmethodcode = address2_shippingmethodcode;
return _x;
}
@Property(name="mobilephone")
@JsonIgnore
public Optional getMobilephone() {
return Optional.ofNullable(mobilephone);
}
public Contact withMobilephone(String mobilephone) {
Checks.checkIsAscii(mobilephone);
Contact _x = _copy();
_x.changedFields = changedFields.add("mobilephone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.mobilephone = mobilephone;
return _x;
}
@Property(name="address3_shippingmethodcode")
@JsonIgnore
public Optional getAddress3_shippingmethodcode() {
return Optional.ofNullable(address3_shippingmethodcode);
}
public Contact withAddress3_shippingmethodcode(Integer address3_shippingmethodcode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_shippingmethodcode = address3_shippingmethodcode;
return _x;
}
@Property(name="address3_postalcode")
@JsonIgnore
public Optional getAddress3_postalcode() {
return Optional.ofNullable(address3_postalcode);
}
public Contact withAddress3_postalcode(String address3_postalcode) {
Checks.checkIsAscii(address3_postalcode);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_postalcode = address3_postalcode;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Contact withVersionnumber(Long versionnumber) {
Contact _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="annualincome")
@JsonIgnore
public Optional getAnnualincome() {
return Optional.ofNullable(annualincome);
}
public Contact withAnnualincome(BigDecimal annualincome) {
Contact _x = _copy();
_x.changedFields = changedFields.add("annualincome");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.annualincome = annualincome;
return _x;
}
@Property(name="fax")
@JsonIgnore
public Optional getFax() {
return Optional.ofNullable(fax);
}
public Contact withFax(String fax) {
Checks.checkIsAscii(fax);
Contact _x = _copy();
_x.changedFields = changedFields.add("fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.fax = fax;
return _x;
}
@Property(name="telephone3")
@JsonIgnore
public Optional getTelephone3() {
return Optional.ofNullable(telephone3);
}
public Contact withTelephone3(String telephone3) {
Checks.checkIsAscii(telephone3);
Contact _x = _copy();
_x.changedFields = changedFields.add("telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.telephone3 = telephone3;
return _x;
}
@Property(name="preferredappointmentdaycode")
@JsonIgnore
public Optional getPreferredappointmentdaycode() {
return Optional.ofNullable(preferredappointmentdaycode);
}
public Contact withPreferredappointmentdaycode(Integer preferredappointmentdaycode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("preferredappointmentdaycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.preferredappointmentdaycode = preferredappointmentdaycode;
return _x;
}
@Property(name="address3_city")
@JsonIgnore
public Optional getAddress3_city() {
return Optional.ofNullable(address3_city);
}
public Contact withAddress3_city(String address3_city) {
Checks.checkIsAscii(address3_city);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_city = address3_city;
return _x;
}
@Property(name="lastonholdtime")
@JsonIgnore
public Optional getLastonholdtime() {
return Optional.ofNullable(lastonholdtime);
}
public Contact withLastonholdtime(OffsetDateTime lastonholdtime) {
Contact _x = _copy();
_x.changedFields = changedFields.add("lastonholdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.lastonholdtime = lastonholdtime;
return _x;
}
@Property(name="address2_stateorprovince")
@JsonIgnore
public Optional getAddress2_stateorprovince() {
return Optional.ofNullable(address2_stateorprovince);
}
public Contact withAddress2_stateorprovince(String address2_stateorprovince) {
Checks.checkIsAscii(address2_stateorprovince);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_stateorprovince = address2_stateorprovince;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Contact with_createdby_value(String _createdby_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="address2_line1")
@JsonIgnore
public Optional getAddress2_line1() {
return Optional.ofNullable(address2_line1);
}
public Contact withAddress2_line1(String address2_line1) {
Checks.checkIsAscii(address2_line1);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_line1 = address2_line1;
return _x;
}
@Property(name="assistantphone")
@JsonIgnore
public Optional getAssistantphone() {
return Optional.ofNullable(assistantphone);
}
public Contact withAssistantphone(String assistantphone) {
Checks.checkIsAscii(assistantphone);
Contact _x = _copy();
_x.changedFields = changedFields.add("assistantphone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.assistantphone = assistantphone;
return _x;
}
@Property(name="lastusedincampaign")
@JsonIgnore
public Optional getLastusedincampaign() {
return Optional.ofNullable(lastusedincampaign);
}
public Contact withLastusedincampaign(OffsetDateTime lastusedincampaign) {
Contact _x = _copy();
_x.changedFields = changedFields.add("lastusedincampaign");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.lastusedincampaign = lastusedincampaign;
return _x;
}
@Property(name="address3_freighttermscode")
@JsonIgnore
public Optional getAddress3_freighttermscode() {
return Optional.ofNullable(address3_freighttermscode);
}
public Contact withAddress3_freighttermscode(Integer address3_freighttermscode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_freighttermscode = address3_freighttermscode;
return _x;
}
@Property(name="pager")
@JsonIgnore
public Optional getPager() {
return Optional.ofNullable(pager);
}
public Contact withPager(String pager) {
Checks.checkIsAscii(pager);
Contact _x = _copy();
_x.changedFields = changedFields.add("pager");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.pager = pager;
return _x;
}
@Property(name="employeeid")
@JsonIgnore
public Optional getEmployeeid() {
return Optional.ofNullable(employeeid);
}
public Contact withEmployeeid(String employeeid) {
Checks.checkIsAscii(employeeid);
Contact _x = _copy();
_x.changedFields = changedFields.add("employeeid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.employeeid = employeeid;
return _x;
}
@Property(name="territorycode")
@JsonIgnore
public Optional getTerritorycode() {
return Optional.ofNullable(territorycode);
}
public Contact withTerritorycode(Integer territorycode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("territorycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.territorycode = territorycode;
return _x;
}
@Property(name="_parentcustomerid_value")
@JsonIgnore
public Optional get_parentcustomerid_value() {
return Optional.ofNullable(_parentcustomerid_value);
}
public Contact with_parentcustomerid_value(String _parentcustomerid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_parentcustomerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._parentcustomerid_value = _parentcustomerid_value;
return _x;
}
@Property(name="managername")
@JsonIgnore
public Optional getManagername() {
return Optional.ofNullable(managername);
}
public Contact withManagername(String managername) {
Checks.checkIsAscii(managername);
Contact _x = _copy();
_x.changedFields = changedFields.add("managername");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.managername = managername;
return _x;
}
@Property(name="birthdate")
@JsonIgnore
public Optional getBirthdate() {
return Optional.ofNullable(birthdate);
}
public Contact withBirthdate(LocalDate birthdate) {
Contact _x = _copy();
_x.changedFields = changedFields.add("birthdate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.birthdate = birthdate;
return _x;
}
@Property(name="address1_name")
@JsonIgnore
public Optional getAddress1_name() {
return Optional.ofNullable(address1_name);
}
public Contact withAddress1_name(String address1_name) {
Checks.checkIsAscii(address1_name);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_name = address1_name;
return _x;
}
@Property(name="department")
@JsonIgnore
public Optional getDepartment() {
return Optional.ofNullable(department);
}
public Contact withDepartment(String department) {
Checks.checkIsAscii(department);
Contact _x = _copy();
_x.changedFields = changedFields.add("department");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.department = department;
return _x;
}
@Property(name="address3_country")
@JsonIgnore
public Optional getAddress3_country() {
return Optional.ofNullable(address3_country);
}
public Contact withAddress3_country(String address3_country) {
Checks.checkIsAscii(address3_country);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_country = address3_country;
return _x;
}
@Property(name="address2_telephone1")
@JsonIgnore
public Optional getAddress2_telephone1() {
return Optional.ofNullable(address2_telephone1);
}
public Contact withAddress2_telephone1(String address2_telephone1) {
Checks.checkIsAscii(address2_telephone1);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_telephone1 = address2_telephone1;
return _x;
}
@Property(name="address1_postofficebox")
@JsonIgnore
public Optional getAddress1_postofficebox() {
return Optional.ofNullable(address1_postofficebox);
}
public Contact withAddress1_postofficebox(String address1_postofficebox) {
Checks.checkIsAscii(address1_postofficebox);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_postofficebox = address1_postofficebox;
return _x;
}
@Property(name="address2_primarycontactname")
@JsonIgnore
public Optional getAddress2_primarycontactname() {
return Optional.ofNullable(address2_primarycontactname);
}
public Contact withAddress2_primarycontactname(String address2_primarycontactname) {
Checks.checkIsAscii(address2_primarycontactname);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_primarycontactname = address2_primarycontactname;
return _x;
}
@Property(name="address2_latitude")
@JsonIgnore
public Optional getAddress2_latitude() {
return Optional.ofNullable(address2_latitude);
}
public Contact withAddress2_latitude(Double address2_latitude) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_latitude = address2_latitude;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Contact with_owningteam_value(String _owningteam_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Contact withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Contact _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="home2")
@JsonIgnore
public Optional getHome2() {
return Optional.ofNullable(home2);
}
public Contact withHome2(String home2) {
Checks.checkIsAscii(home2);
Contact _x = _copy();
_x.changedFields = changedFields.add("home2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.home2 = home2;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Contact withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="address2_postalcode")
@JsonIgnore
public Optional getAddress2_postalcode() {
return Optional.ofNullable(address2_postalcode);
}
public Contact withAddress2_postalcode(String address2_postalcode) {
Checks.checkIsAscii(address2_postalcode);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_postalcode = address2_postalcode;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Contact with_owninguser_value(String _owninguser_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="merged")
@JsonIgnore
public Optional getMerged() {
return Optional.ofNullable(merged);
}
public Contact withMerged(Boolean merged) {
Contact _x = _copy();
_x.changedFields = changedFields.add("merged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.merged = merged;
return _x;
}
@Property(name="_masterid_value")
@JsonIgnore
public Optional get_masterid_value() {
return Optional.ofNullable(_masterid_value);
}
public Contact with_masterid_value(String _masterid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_masterid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._masterid_value = _masterid_value;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Contact with_createdonbehalfby_value(String _createdonbehalfby_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="address3_postofficebox")
@JsonIgnore
public Optional getAddress3_postofficebox() {
return Optional.ofNullable(address3_postofficebox);
}
public Contact withAddress3_postofficebox(String address3_postofficebox) {
Checks.checkIsAscii(address3_postofficebox);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_postofficebox = address3_postofficebox;
return _x;
}
@Property(name="customertypecode")
@JsonIgnore
public Optional getCustomertypecode() {
return Optional.ofNullable(customertypecode);
}
public Contact withCustomertypecode(Integer customertypecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("customertypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.customertypecode = customertypecode;
return _x;
}
@Property(name="business2")
@JsonIgnore
public Optional getBusiness2() {
return Optional.ofNullable(business2);
}
public Contact withBusiness2(String business2) {
Checks.checkIsAscii(business2);
Contact _x = _copy();
_x.changedFields = changedFields.add("business2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.business2 = business2;
return _x;
}
@Property(name="address3_county")
@JsonIgnore
public Optional getAddress3_county() {
return Optional.ofNullable(address3_county);
}
public Contact withAddress3_county(String address3_county) {
Checks.checkIsAscii(address3_county);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_county = address3_county;
return _x;
}
@Property(name="address1_latitude")
@JsonIgnore
public Optional getAddress1_latitude() {
return Optional.ofNullable(address1_latitude);
}
public Contact withAddress1_latitude(Double address1_latitude) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_latitude = address1_latitude;
return _x;
}
@Property(name="address1_freighttermscode")
@JsonIgnore
public Optional getAddress1_freighttermscode() {
return Optional.ofNullable(address1_freighttermscode);
}
public Contact withAddress1_freighttermscode(Integer address1_freighttermscode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_freighttermscode = address1_freighttermscode;
return _x;
}
@Property(name="traversedpath")
@JsonIgnore
public Optional getTraversedpath() {
return Optional.ofNullable(traversedpath);
}
public Contact withTraversedpath(String traversedpath) {
Checks.checkIsAscii(traversedpath);
Contact _x = _copy();
_x.changedFields = changedFields.add("traversedpath");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.traversedpath = traversedpath;
return _x;
}
@Property(name="address3_addresstypecode")
@JsonIgnore
public Optional getAddress3_addresstypecode() {
return Optional.ofNullable(address3_addresstypecode);
}
public Contact withAddress3_addresstypecode(Integer address3_addresstypecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_addresstypecode = address3_addresstypecode;
return _x;
}
@Property(name="address1_longitude")
@JsonIgnore
public Optional getAddress1_longitude() {
return Optional.ofNullable(address1_longitude);
}
public Contact withAddress1_longitude(Double address1_longitude) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_longitude = address1_longitude;
return _x;
}
@Property(name="address1_addresstypecode")
@JsonIgnore
public Optional getAddress1_addresstypecode() {
return Optional.ofNullable(address1_addresstypecode);
}
public Contact withAddress1_addresstypecode(Integer address1_addresstypecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_addresstypecode = address1_addresstypecode;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Contact withStatuscode(Integer statuscode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.statuscode = statuscode;
return _x;
}
@Property(name="yomifullname")
@JsonIgnore
public Optional getYomifullname() {
return Optional.ofNullable(yomifullname);
}
public Contact withYomifullname(String yomifullname) {
Checks.checkIsAscii(yomifullname);
Contact _x = _copy();
_x.changedFields = changedFields.add("yomifullname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.yomifullname = yomifullname;
return _x;
}
@Property(name="aging90_base")
@JsonIgnore
public Optional getAging90_base() {
return Optional.ofNullable(aging90_base);
}
public Contact withAging90_base(BigDecimal aging90_base) {
Contact _x = _copy();
_x.changedFields = changedFields.add("aging90_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.aging90_base = aging90_base;
return _x;
}
@Property(name="_accountid_value")
@JsonIgnore
public Optional get_accountid_value() {
return Optional.ofNullable(_accountid_value);
}
public Contact with_accountid_value(String _accountid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_accountid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._accountid_value = _accountid_value;
return _x;
}
@Property(name="address3_primarycontactname")
@JsonIgnore
public Optional getAddress3_primarycontactname() {
return Optional.ofNullable(address3_primarycontactname);
}
public Contact withAddress3_primarycontactname(String address3_primarycontactname) {
Checks.checkIsAscii(address3_primarycontactname);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_primarycontactname = address3_primarycontactname;
return _x;
}
@Property(name="familystatuscode")
@JsonIgnore
public Optional getFamilystatuscode() {
return Optional.ofNullable(familystatuscode);
}
public Contact withFamilystatuscode(Integer familystatuscode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("familystatuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.familystatuscode = familystatuscode;
return _x;
}
@Property(name="address3_addressid")
@JsonIgnore
public Optional getAddress3_addressid() {
return Optional.ofNullable(address3_addressid);
}
public Contact withAddress3_addressid(String address3_addressid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_addressid = address3_addressid;
return _x;
}
@Property(name="leadsourcecode")
@JsonIgnore
public Optional getLeadsourcecode() {
return Optional.ofNullable(leadsourcecode);
}
public Contact withLeadsourcecode(Integer leadsourcecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("leadsourcecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.leadsourcecode = leadsourcecode;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Contact withStatecode(Integer statecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.statecode = statecode;
return _x;
}
@Property(name="address2_freighttermscode")
@JsonIgnore
public Optional getAddress2_freighttermscode() {
return Optional.ofNullable(address2_freighttermscode);
}
public Contact withAddress2_freighttermscode(Integer address2_freighttermscode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_freighttermscode = address2_freighttermscode;
return _x;
}
@Property(name="address1_composite")
@JsonIgnore
public Optional getAddress1_composite() {
return Optional.ofNullable(address1_composite);
}
public Contact withAddress1_composite(String address1_composite) {
Checks.checkIsAscii(address1_composite);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_composite = address1_composite;
return _x;
}
@Property(name="telephone2")
@JsonIgnore
public Optional getTelephone2() {
return Optional.ofNullable(telephone2);
}
public Contact withTelephone2(String telephone2) {
Checks.checkIsAscii(telephone2);
Contact _x = _copy();
_x.changedFields = changedFields.add("telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.telephone2 = telephone2;
return _x;
}
@Property(name="yomimiddlename")
@JsonIgnore
public Optional getYomimiddlename() {
return Optional.ofNullable(yomimiddlename);
}
public Contact withYomimiddlename(String yomimiddlename) {
Checks.checkIsAscii(yomimiddlename);
Contact _x = _copy();
_x.changedFields = changedFields.add("yomimiddlename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.yomimiddlename = yomimiddlename;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Contact with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="jobtitle")
@JsonIgnore
public Optional getJobtitle() {
return Optional.ofNullable(jobtitle);
}
public Contact withJobtitle(String jobtitle) {
Checks.checkIsAscii(jobtitle);
Contact _x = _copy();
_x.changedFields = changedFields.add("jobtitle");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.jobtitle = jobtitle;
return _x;
}
@Property(name="address2_addresstypecode")
@JsonIgnore
public Optional getAddress2_addresstypecode() {
return Optional.ofNullable(address2_addresstypecode);
}
public Contact withAddress2_addresstypecode(Integer address2_addresstypecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_addresstypecode = address2_addresstypecode;
return _x;
}
@Property(name="educationcode")
@JsonIgnore
public Optional getEducationcode() {
return Optional.ofNullable(educationcode);
}
public Contact withEducationcode(Integer educationcode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("educationcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.educationcode = educationcode;
return _x;
}
@Property(name="address1_telephone3")
@JsonIgnore
public Optional getAddress1_telephone3() {
return Optional.ofNullable(address1_telephone3);
}
public Contact withAddress1_telephone3(String address1_telephone3) {
Checks.checkIsAscii(address1_telephone3);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_telephone3 = address1_telephone3;
return _x;
}
@Property(name="followemail")
@JsonIgnore
public Optional getFollowemail() {
return Optional.ofNullable(followemail);
}
public Contact withFollowemail(Boolean followemail) {
Contact _x = _copy();
_x.changedFields = changedFields.add("followemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.followemail = followemail;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Contact withImportsequencenumber(Integer importsequencenumber) {
Contact _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="gendercode")
@JsonIgnore
public Optional getGendercode() {
return Optional.ofNullable(gendercode);
}
public Contact withGendercode(Integer gendercode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("gendercode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.gendercode = gendercode;
return _x;
}
@Property(name="address2_line2")
@JsonIgnore
public Optional getAddress2_line2() {
return Optional.ofNullable(address2_line2);
}
public Contact withAddress2_line2(String address2_line2) {
Checks.checkIsAscii(address2_line2);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_line2 = address2_line2;
return _x;
}
@Property(name="creditlimit_base")
@JsonIgnore
public Optional getCreditlimit_base() {
return Optional.ofNullable(creditlimit_base);
}
public Contact withCreditlimit_base(BigDecimal creditlimit_base) {
Contact _x = _copy();
_x.changedFields = changedFields.add("creditlimit_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.creditlimit_base = creditlimit_base;
return _x;
}
@Property(name="annualincome_base")
@JsonIgnore
public Optional getAnnualincome_base() {
return Optional.ofNullable(annualincome_base);
}
public Contact withAnnualincome_base(BigDecimal annualincome_base) {
Contact _x = _copy();
_x.changedFields = changedFields.add("annualincome_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.annualincome_base = annualincome_base;
return _x;
}
@Property(name="donotfax")
@JsonIgnore
public Optional getDonotfax() {
return Optional.ofNullable(donotfax);
}
public Contact withDonotfax(Boolean donotfax) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotfax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotfax = donotfax;
return _x;
}
@Property(name="address3_line1")
@JsonIgnore
public Optional getAddress3_line1() {
return Optional.ofNullable(address3_line1);
}
public Contact withAddress3_line1(String address3_line1) {
Checks.checkIsAscii(address3_line1);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_line1 = address3_line1;
return _x;
}
@Property(name="address1_county")
@JsonIgnore
public Optional getAddress1_county() {
return Optional.ofNullable(address1_county);
}
public Contact withAddress1_county(String address1_county) {
Checks.checkIsAscii(address1_county);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_county = address1_county;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Contact with_owningbusinessunit_value(String _owningbusinessunit_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="_createdbyexternalparty_value")
@JsonIgnore
public Optional get_createdbyexternalparty_value() {
return Optional.ofNullable(_createdbyexternalparty_value);
}
public Contact with_createdbyexternalparty_value(String _createdbyexternalparty_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_createdbyexternalparty_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._createdbyexternalparty_value = _createdbyexternalparty_value;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Contact withEntityimageid(String entityimageid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="processid")
@JsonIgnore
public Optional getProcessid() {
return Optional.ofNullable(processid);
}
public Contact withProcessid(String processid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("processid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.processid = processid;
return _x;
}
@Property(name="address1_telephone2")
@JsonIgnore
public Optional getAddress1_telephone2() {
return Optional.ofNullable(address1_telephone2);
}
public Contact withAddress1_telephone2(String address1_telephone2) {
Checks.checkIsAscii(address1_telephone2);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_telephone2 = address1_telephone2;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Contact withDescription(String description) {
Checks.checkIsAscii(description);
Contact _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.description = description;
return _x;
}
@Property(name="address1_fax")
@JsonIgnore
public Optional getAddress1_fax() {
return Optional.ofNullable(address1_fax);
}
public Contact withAddress1_fax(String address1_fax) {
Checks.checkIsAscii(address1_fax);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_fax = address1_fax;
return _x;
}
@Property(name="marketingonly")
@JsonIgnore
public Optional getMarketingonly() {
return Optional.ofNullable(marketingonly);
}
public Contact withMarketingonly(Boolean marketingonly) {
Contact _x = _copy();
_x.changedFields = changedFields.add("marketingonly");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.marketingonly = marketingonly;
return _x;
}
@Property(name="address3_line2")
@JsonIgnore
public Optional getAddress3_line2() {
return Optional.ofNullable(address3_line2);
}
public Contact withAddress3_line2(String address3_line2) {
Checks.checkIsAscii(address3_line2);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_line2 = address3_line2;
return _x;
}
@Property(name="address1_addressid")
@JsonIgnore
public Optional getAddress1_addressid() {
return Optional.ofNullable(address1_addressid);
}
public Contact withAddress1_addressid(String address1_addressid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_addressid = address1_addressid;
return _x;
}
@Property(name="donotpostalmail")
@JsonIgnore
public Optional getDonotpostalmail() {
return Optional.ofNullable(donotpostalmail);
}
public Contact withDonotpostalmail(Boolean donotpostalmail) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotpostalmail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotpostalmail = donotpostalmail;
return _x;
}
@Property(name="address2_utcoffset")
@JsonIgnore
public Optional getAddress2_utcoffset() {
return Optional.ofNullable(address2_utcoffset);
}
public Contact withAddress2_utcoffset(Integer address2_utcoffset) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_utcoffset = address2_utcoffset;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Contact withExchangerate(BigDecimal exchangerate) {
Contact _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="aging30_base")
@JsonIgnore
public Optional getAging30_base() {
return Optional.ofNullable(aging30_base);
}
public Contact withAging30_base(BigDecimal aging30_base) {
Contact _x = _copy();
_x.changedFields = changedFields.add("aging30_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.aging30_base = aging30_base;
return _x;
}
@Property(name="callback")
@JsonIgnore
public Optional getCallback() {
return Optional.ofNullable(callback);
}
public Contact withCallback(String callback) {
Checks.checkIsAscii(callback);
Contact _x = _copy();
_x.changedFields = changedFields.add("callback");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.callback = callback;
return _x;
}
@Property(name="emailaddress2")
@JsonIgnore
public Optional getEmailaddress2() {
return Optional.ofNullable(emailaddress2);
}
public Contact withEmailaddress2(String emailaddress2) {
Checks.checkIsAscii(emailaddress2);
Contact _x = _copy();
_x.changedFields = changedFields.add("emailaddress2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.emailaddress2 = emailaddress2;
return _x;
}
@Property(name="address2_line3")
@JsonIgnore
public Optional getAddress2_line3() {
return Optional.ofNullable(address2_line3);
}
public Contact withAddress2_line3(String address2_line3) {
Checks.checkIsAscii(address2_line3);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_line3 = address2_line3;
return _x;
}
@Property(name="managerphone")
@JsonIgnore
public Optional getManagerphone() {
return Optional.ofNullable(managerphone);
}
public Contact withManagerphone(String managerphone) {
Checks.checkIsAscii(managerphone);
Contact _x = _copy();
_x.changedFields = changedFields.add("managerphone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.managerphone = managerphone;
return _x;
}
@Property(name="websiteurl")
@JsonIgnore
public Optional getWebsiteurl() {
return Optional.ofNullable(websiteurl);
}
public Contact withWebsiteurl(String websiteurl) {
Checks.checkIsAscii(websiteurl);
Contact _x = _copy();
_x.changedFields = changedFields.add("websiteurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.websiteurl = websiteurl;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Contact withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Contact _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="firstname")
@JsonIgnore
public Optional getFirstname() {
return Optional.ofNullable(firstname);
}
public Contact withFirstname(String firstname) {
Checks.checkIsAscii(firstname);
Contact _x = _copy();
_x.changedFields = changedFields.add("firstname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.firstname = firstname;
return _x;
}
@Property(name="address1_telephone1")
@JsonIgnore
public Optional getAddress1_telephone1() {
return Optional.ofNullable(address1_telephone1);
}
public Contact withAddress1_telephone1(String address1_telephone1) {
Checks.checkIsAscii(address1_telephone1);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_telephone1 = address1_telephone1;
return _x;
}
@Property(name="address3_composite")
@JsonIgnore
public Optional getAddress3_composite() {
return Optional.ofNullable(address3_composite);
}
public Contact withAddress3_composite(String address3_composite) {
Checks.checkIsAscii(address3_composite);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_composite = address3_composite;
return _x;
}
@Property(name="address3_fax")
@JsonIgnore
public Optional getAddress3_fax() {
return Optional.ofNullable(address3_fax);
}
public Contact withAddress3_fax(String address3_fax) {
Checks.checkIsAscii(address3_fax);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_fax = address3_fax;
return _x;
}
@Property(name="childrensnames")
@JsonIgnore
public Optional getChildrensnames() {
return Optional.ofNullable(childrensnames);
}
public Contact withChildrensnames(String childrensnames) {
Checks.checkIsAscii(childrensnames);
Contact _x = _copy();
_x.changedFields = changedFields.add("childrensnames");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.childrensnames = childrensnames;
return _x;
}
@Property(name="preferredcontactmethodcode")
@JsonIgnore
public Optional getPreferredcontactmethodcode() {
return Optional.ofNullable(preferredcontactmethodcode);
}
public Contact withPreferredcontactmethodcode(Integer preferredcontactmethodcode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("preferredcontactmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.preferredcontactmethodcode = preferredcontactmethodcode;
return _x;
}
@Property(name="numberofchildren")
@JsonIgnore
public Optional getNumberofchildren() {
return Optional.ofNullable(numberofchildren);
}
public Contact withNumberofchildren(Integer numberofchildren) {
Contact _x = _copy();
_x.changedFields = changedFields.add("numberofchildren");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.numberofchildren = numberofchildren;
return _x;
}
@Property(name="subscriptionid")
@JsonIgnore
public Optional getSubscriptionid() {
return Optional.ofNullable(subscriptionid);
}
public Contact withSubscriptionid(String subscriptionid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("subscriptionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.subscriptionid = subscriptionid;
return _x;
}
@Property(name="yomilastname")
@JsonIgnore
public Optional getYomilastname() {
return Optional.ofNullable(yomilastname);
}
public Contact withYomilastname(String yomilastname) {
Checks.checkIsAscii(yomilastname);
Contact _x = _copy();
_x.changedFields = changedFields.add("yomilastname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.yomilastname = yomilastname;
return _x;
}
@Property(name="address2_postofficebox")
@JsonIgnore
public Optional getAddress2_postofficebox() {
return Optional.ofNullable(address2_postofficebox);
}
public Contact withAddress2_postofficebox(String address2_postofficebox) {
Checks.checkIsAscii(address2_postofficebox);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_postofficebox = address2_postofficebox;
return _x;
}
@Property(name="aging90")
@JsonIgnore
public Optional getAging90() {
return Optional.ofNullable(aging90);
}
public Contact withAging90(BigDecimal aging90) {
Contact _x = _copy();
_x.changedFields = changedFields.add("aging90");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.aging90 = aging90;
return _x;
}
@Property(name="donotbulkpostalmail")
@JsonIgnore
public Optional getDonotbulkpostalmail() {
return Optional.ofNullable(donotbulkpostalmail);
}
public Contact withDonotbulkpostalmail(Boolean donotbulkpostalmail) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotbulkpostalmail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotbulkpostalmail = donotbulkpostalmail;
return _x;
}
@Property(name="emailaddress1")
@JsonIgnore
public Optional getEmailaddress1() {
return Optional.ofNullable(emailaddress1);
}
public Contact withEmailaddress1(String emailaddress1) {
Checks.checkIsAscii(emailaddress1);
Contact _x = _copy();
_x.changedFields = changedFields.add("emailaddress1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.emailaddress1 = emailaddress1;
return _x;
}
@Property(name="donotbulkemail")
@JsonIgnore
public Optional getDonotbulkemail() {
return Optional.ofNullable(donotbulkemail);
}
public Contact withDonotbulkemail(Boolean donotbulkemail) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotbulkemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotbulkemail = donotbulkemail;
return _x;
}
@Property(name="customersizecode")
@JsonIgnore
public Optional getCustomersizecode() {
return Optional.ofNullable(customersizecode);
}
public Contact withCustomersizecode(Integer customersizecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("customersizecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.customersizecode = customersizecode;
return _x;
}
@Property(name="address1_city")
@JsonIgnore
public Optional getAddress1_city() {
return Optional.ofNullable(address1_city);
}
public Contact withAddress1_city(String address1_city) {
Checks.checkIsAscii(address1_city);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_city = address1_city;
return _x;
}
@Property(name="preferredappointmenttimecode")
@JsonIgnore
public Optional getPreferredappointmenttimecode() {
return Optional.ofNullable(preferredappointmenttimecode);
}
public Contact withPreferredappointmenttimecode(Integer preferredappointmenttimecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("preferredappointmenttimecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.preferredappointmenttimecode = preferredappointmenttimecode;
return _x;
}
@Property(name="address3_latitude")
@JsonIgnore
public Optional getAddress3_latitude() {
return Optional.ofNullable(address3_latitude);
}
public Contact withAddress3_latitude(Double address3_latitude) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_latitude = address3_latitude;
return _x;
}
@Property(name="aging60_base")
@JsonIgnore
public Optional getAging60_base() {
return Optional.ofNullable(aging60_base);
}
public Contact withAging60_base(BigDecimal aging60_base) {
Contact _x = _copy();
_x.changedFields = changedFields.add("aging60_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.aging60_base = aging60_base;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Contact with_transactioncurrencyid_value(String _transactioncurrencyid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Contact withEntityimage(byte[] entityimage) {
Contact _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.entityimage = entityimage;
return _x;
}
@Property(name="_modifiedbyexternalparty_value")
@JsonIgnore
public Optional get_modifiedbyexternalparty_value() {
return Optional.ofNullable(_modifiedbyexternalparty_value);
}
public Contact with_modifiedbyexternalparty_value(String _modifiedbyexternalparty_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_modifiedbyexternalparty_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
return _x;
}
@Property(name="paymenttermscode")
@JsonIgnore
public Optional getPaymenttermscode() {
return Optional.ofNullable(paymenttermscode);
}
public Contact withPaymenttermscode(Integer paymenttermscode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("paymenttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.paymenttermscode = paymenttermscode;
return _x;
}
@Property(name="address3_name")
@JsonIgnore
public Optional getAddress3_name() {
return Optional.ofNullable(address3_name);
}
public Contact withAddress3_name(String address3_name) {
Checks.checkIsAscii(address3_name);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_name = address3_name;
return _x;
}
@Property(name="participatesinworkflow")
@JsonIgnore
public Optional getParticipatesinworkflow() {
return Optional.ofNullable(participatesinworkflow);
}
public Contact withParticipatesinworkflow(Boolean participatesinworkflow) {
Contact _x = _copy();
_x.changedFields = changedFields.add("participatesinworkflow");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.participatesinworkflow = participatesinworkflow;
return _x;
}
@Property(name="address1_shippingmethodcode")
@JsonIgnore
public Optional getAddress1_shippingmethodcode() {
return Optional.ofNullable(address1_shippingmethodcode);
}
public Contact withAddress1_shippingmethodcode(Integer address1_shippingmethodcode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_shippingmethodcode = address1_shippingmethodcode;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Contact with_modifiedby_value(String _modifiedby_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="ftpsiteurl")
@JsonIgnore
public Optional getFtpsiteurl() {
return Optional.ofNullable(ftpsiteurl);
}
public Contact withFtpsiteurl(String ftpsiteurl) {
Checks.checkIsAscii(ftpsiteurl);
Contact _x = _copy();
_x.changedFields = changedFields.add("ftpsiteurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.ftpsiteurl = ftpsiteurl;
return _x;
}
@Property(name="_preferredsystemuserid_value")
@JsonIgnore
public Optional get_preferredsystemuserid_value() {
return Optional.ofNullable(_preferredsystemuserid_value);
}
public Contact with_preferredsystemuserid_value(String _preferredsystemuserid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_preferredsystemuserid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._preferredsystemuserid_value = _preferredsystemuserid_value;
return _x;
}
@Property(name="address2_telephone2")
@JsonIgnore
public Optional getAddress2_telephone2() {
return Optional.ofNullable(address2_telephone2);
}
public Contact withAddress2_telephone2(String address2_telephone2) {
Checks.checkIsAscii(address2_telephone2);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_telephone2 = address2_telephone2;
return _x;
}
@Property(name="_slainvokedid_value")
@JsonIgnore
public Optional get_slainvokedid_value() {
return Optional.ofNullable(_slainvokedid_value);
}
public Contact with_slainvokedid_value(String _slainvokedid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_slainvokedid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._slainvokedid_value = _slainvokedid_value;
return _x;
}
@Property(name="address3_utcoffset")
@JsonIgnore
public Optional getAddress3_utcoffset() {
return Optional.ofNullable(address3_utcoffset);
}
public Contact withAddress3_utcoffset(Integer address3_utcoffset) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_utcoffset = address3_utcoffset;
return _x;
}
@Property(name="address3_stateorprovince")
@JsonIgnore
public Optional getAddress3_stateorprovince() {
return Optional.ofNullable(address3_stateorprovince);
}
public Contact withAddress3_stateorprovince(String address3_stateorprovince) {
Checks.checkIsAscii(address3_stateorprovince);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_stateorprovince = address3_stateorprovince;
return _x;
}
@Property(name="address3_telephone1")
@JsonIgnore
public Optional getAddress3_telephone1() {
return Optional.ofNullable(address3_telephone1);
}
public Contact withAddress3_telephone1(String address3_telephone1) {
Checks.checkIsAscii(address3_telephone1);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_telephone1 = address3_telephone1;
return _x;
}
@Property(name="nickname")
@JsonIgnore
public Optional getNickname() {
return Optional.ofNullable(nickname);
}
public Contact withNickname(String nickname) {
Checks.checkIsAscii(nickname);
Contact _x = _copy();
_x.changedFields = changedFields.add("nickname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.nickname = nickname;
return _x;
}
@Property(name="fullname")
@JsonIgnore
public Optional getFullname() {
return Optional.ofNullable(fullname);
}
public Contact withFullname(String fullname) {
Checks.checkIsAscii(fullname);
Contact _x = _copy();
_x.changedFields = changedFields.add("fullname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.fullname = fullname;
return _x;
}
@Property(name="assistantname")
@JsonIgnore
public Optional getAssistantname() {
return Optional.ofNullable(assistantname);
}
public Contact withAssistantname(String assistantname) {
Checks.checkIsAscii(assistantname);
Contact _x = _copy();
_x.changedFields = changedFields.add("assistantname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.assistantname = assistantname;
return _x;
}
@Property(name="address2_country")
@JsonIgnore
public Optional getAddress2_country() {
return Optional.ofNullable(address2_country);
}
public Contact withAddress2_country(String address2_country) {
Checks.checkIsAscii(address2_country);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_country = address2_country;
return _x;
}
@Property(name="aging60")
@JsonIgnore
public Optional getAging60() {
return Optional.ofNullable(aging60);
}
public Contact withAging60(BigDecimal aging60) {
Contact _x = _copy();
_x.changedFields = changedFields.add("aging60");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.aging60 = aging60;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Contact withModifiedon(OffsetDateTime modifiedon) {
Contact _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="externaluseridentifier")
@JsonIgnore
public Optional getExternaluseridentifier() {
return Optional.ofNullable(externaluseridentifier);
}
public Contact withExternaluseridentifier(String externaluseridentifier) {
Checks.checkIsAscii(externaluseridentifier);
Contact _x = _copy();
_x.changedFields = changedFields.add("externaluseridentifier");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.externaluseridentifier = externaluseridentifier;
return _x;
}
@Property(name="address2_name")
@JsonIgnore
public Optional getAddress2_name() {
return Optional.ofNullable(address2_name);
}
public Contact withAddress2_name(String address2_name) {
Checks.checkIsAscii(address2_name);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_name = address2_name;
return _x;
}
@Property(name="stageid")
@JsonIgnore
public Optional getStageid() {
return Optional.ofNullable(stageid);
}
public Contact withStageid(String stageid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("stageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.stageid = stageid;
return _x;
}
@Property(name="creditonhold")
@JsonIgnore
public Optional getCreditonhold() {
return Optional.ofNullable(creditonhold);
}
public Contact withCreditonhold(Boolean creditonhold) {
Contact _x = _copy();
_x.changedFields = changedFields.add("creditonhold");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.creditonhold = creditonhold;
return _x;
}
@Property(name="address3_longitude")
@JsonIgnore
public Optional getAddress3_longitude() {
return Optional.ofNullable(address3_longitude);
}
public Contact withAddress3_longitude(Double address3_longitude) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_longitude = address3_longitude;
return _x;
}
@Property(name="onholdtime")
@JsonIgnore
public Optional getOnholdtime() {
return Optional.ofNullable(onholdtime);
}
public Contact withOnholdtime(Integer onholdtime) {
Contact _x = _copy();
_x.changedFields = changedFields.add("onholdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.onholdtime = onholdtime;
return _x;
}
@Property(name="address2_telephone3")
@JsonIgnore
public Optional getAddress2_telephone3() {
return Optional.ofNullable(address2_telephone3);
}
public Contact withAddress2_telephone3(String address2_telephone3) {
Checks.checkIsAscii(address2_telephone3);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_telephone3 = address2_telephone3;
return _x;
}
@Property(name="donotphone")
@JsonIgnore
public Optional getDonotphone() {
return Optional.ofNullable(donotphone);
}
public Contact withDonotphone(Boolean donotphone) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotphone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotphone = donotphone;
return _x;
}
@Property(name="address3_upszone")
@JsonIgnore
public Optional getAddress3_upszone() {
return Optional.ofNullable(address3_upszone);
}
public Contact withAddress3_upszone(String address3_upszone) {
Checks.checkIsAscii(address3_upszone);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_upszone = address3_upszone;
return _x;
}
@Property(name="contactid")
@JsonIgnore
public Optional getContactid() {
return Optional.ofNullable(contactid);
}
public Contact withContactid(String contactid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("contactid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.contactid = contactid;
return _x;
}
@Property(name="aging30")
@JsonIgnore
public Optional getAging30() {
return Optional.ofNullable(aging30);
}
public Contact withAging30(BigDecimal aging30) {
Contact _x = _copy();
_x.changedFields = changedFields.add("aging30");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.aging30 = aging30;
return _x;
}
@Property(name="address2_upszone")
@JsonIgnore
public Optional getAddress2_upszone() {
return Optional.ofNullable(address2_upszone);
}
public Contact withAddress2_upszone(String address2_upszone) {
Checks.checkIsAscii(address2_upszone);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_upszone = address2_upszone;
return _x;
}
@Property(name="address1_upszone")
@JsonIgnore
public Optional getAddress1_upszone() {
return Optional.ofNullable(address1_upszone);
}
public Contact withAddress1_upszone(String address1_upszone) {
Checks.checkIsAscii(address1_upszone);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_upszone = address1_upszone;
return _x;
}
@Property(name="creditlimit")
@JsonIgnore
public Optional getCreditlimit() {
return Optional.ofNullable(creditlimit);
}
public Contact withCreditlimit(BigDecimal creditlimit) {
Contact _x = _copy();
_x.changedFields = changedFields.add("creditlimit");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.creditlimit = creditlimit;
return _x;
}
@Property(name="accountrolecode")
@JsonIgnore
public Optional getAccountrolecode() {
return Optional.ofNullable(accountrolecode);
}
public Contact withAccountrolecode(Integer accountrolecode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("accountrolecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.accountrolecode = accountrolecode;
return _x;
}
@Property(name="salutation")
@JsonIgnore
public Optional getSalutation() {
return Optional.ofNullable(salutation);
}
public Contact withSalutation(String salutation) {
Checks.checkIsAscii(salutation);
Contact _x = _copy();
_x.changedFields = changedFields.add("salutation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.salutation = salutation;
return _x;
}
@Property(name="suffix")
@JsonIgnore
public Optional getSuffix() {
return Optional.ofNullable(suffix);
}
public Contact withSuffix(String suffix) {
Checks.checkIsAscii(suffix);
Contact _x = _copy();
_x.changedFields = changedFields.add("suffix");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.suffix = suffix;
return _x;
}
@Property(name="address1_primarycontactname")
@JsonIgnore
public Optional getAddress1_primarycontactname() {
return Optional.ofNullable(address1_primarycontactname);
}
public Contact withAddress1_primarycontactname(String address1_primarycontactname) {
Checks.checkIsAscii(address1_primarycontactname);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_primarycontactname = address1_primarycontactname;
return _x;
}
@Property(name="address1_utcoffset")
@JsonIgnore
public Optional getAddress1_utcoffset() {
return Optional.ofNullable(address1_utcoffset);
}
public Contact withAddress1_utcoffset(Integer address1_utcoffset) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_utcoffset = address1_utcoffset;
return _x;
}
@Property(name="address1_postalcode")
@JsonIgnore
public Optional getAddress1_postalcode() {
return Optional.ofNullable(address1_postalcode);
}
public Contact withAddress1_postalcode(String address1_postalcode) {
Checks.checkIsAscii(address1_postalcode);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_postalcode = address1_postalcode;
return _x;
}
@Property(name="governmentid")
@JsonIgnore
public Optional getGovernmentid() {
return Optional.ofNullable(governmentid);
}
public Contact withGovernmentid(String governmentid) {
Checks.checkIsAscii(governmentid);
Contact _x = _copy();
_x.changedFields = changedFields.add("governmentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.governmentid = governmentid;
return _x;
}
@Property(name="donotsendmm")
@JsonIgnore
public Optional getDonotsendmm() {
return Optional.ofNullable(donotsendmm);
}
public Contact withDonotsendmm(Boolean donotsendmm) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotsendmm");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotsendmm = donotsendmm;
return _x;
}
@Property(name="address1_country")
@JsonIgnore
public Optional getAddress1_country() {
return Optional.ofNullable(address1_country);
}
public Contact withAddress1_country(String address1_country) {
Checks.checkIsAscii(address1_country);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_country = address1_country;
return _x;
}
@Property(name="lastname")
@JsonIgnore
public Optional getLastname() {
return Optional.ofNullable(lastname);
}
public Contact withLastname(String lastname) {
Checks.checkIsAscii(lastname);
Contact _x = _copy();
_x.changedFields = changedFields.add("lastname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.lastname = lastname;
return _x;
}
@Property(name="address2_city")
@JsonIgnore
public Optional getAddress2_city() {
return Optional.ofNullable(address2_city);
}
public Contact withAddress2_city(String address2_city) {
Checks.checkIsAscii(address2_city);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_city = address2_city;
return _x;
}
@Property(name="donotemail")
@JsonIgnore
public Optional getDonotemail() {
return Optional.ofNullable(donotemail);
}
public Contact withDonotemail(Boolean donotemail) {
Contact _x = _copy();
_x.changedFields = changedFields.add("donotemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.donotemail = donotemail;
return _x;
}
@Property(name="company")
@JsonIgnore
public Optional getCompany() {
return Optional.ofNullable(company);
}
public Contact withCompany(String company) {
Checks.checkIsAscii(company);
Contact _x = _copy();
_x.changedFields = changedFields.add("company");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.company = company;
return _x;
}
@Property(name="address1_line2")
@JsonIgnore
public Optional getAddress1_line2() {
return Optional.ofNullable(address1_line2);
}
public Contact withAddress1_line2(String address1_line2) {
Checks.checkIsAscii(address1_line2);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_line2 = address1_line2;
return _x;
}
@Property(name="address2_longitude")
@JsonIgnore
public Optional getAddress2_longitude() {
return Optional.ofNullable(address2_longitude);
}
public Contact withAddress2_longitude(Double address2_longitude) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_longitude = address2_longitude;
return _x;
}
@Property(name="address1_stateorprovince")
@JsonIgnore
public Optional getAddress1_stateorprovince() {
return Optional.ofNullable(address1_stateorprovince);
}
public Contact withAddress1_stateorprovince(String address1_stateorprovince) {
Checks.checkIsAscii(address1_stateorprovince);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_stateorprovince = address1_stateorprovince;
return _x;
}
@Property(name="yomifirstname")
@JsonIgnore
public Optional getYomifirstname() {
return Optional.ofNullable(yomifirstname);
}
public Contact withYomifirstname(String yomifirstname) {
Checks.checkIsAscii(yomifirstname);
Contact _x = _copy();
_x.changedFields = changedFields.add("yomifirstname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.yomifirstname = yomifirstname;
return _x;
}
@Property(name="telephone1")
@JsonIgnore
public Optional getTelephone1() {
return Optional.ofNullable(telephone1);
}
public Contact withTelephone1(String telephone1) {
Checks.checkIsAscii(telephone1);
Contact _x = _copy();
_x.changedFields = changedFields.add("telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.telephone1 = telephone1;
return _x;
}
@Property(name="address1_line1")
@JsonIgnore
public Optional getAddress1_line1() {
return Optional.ofNullable(address1_line1);
}
public Contact withAddress1_line1(String address1_line1) {
Checks.checkIsAscii(address1_line1);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_line1 = address1_line1;
return _x;
}
@Property(name="address2_addressid")
@JsonIgnore
public Optional getAddress2_addressid() {
return Optional.ofNullable(address2_addressid);
}
public Contact withAddress2_addressid(String address2_addressid) {
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_addressid = address2_addressid;
return _x;
}
@Property(name="isbackofficecustomer")
@JsonIgnore
public Optional getIsbackofficecustomer() {
return Optional.ofNullable(isbackofficecustomer);
}
public Contact withIsbackofficecustomer(Boolean isbackofficecustomer) {
Contact _x = _copy();
_x.changedFields = changedFields.add("isbackofficecustomer");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.isbackofficecustomer = isbackofficecustomer;
return _x;
}
@Property(name="address2_county")
@JsonIgnore
public Optional getAddress2_county() {
return Optional.ofNullable(address2_county);
}
public Contact withAddress2_county(String address2_county) {
Checks.checkIsAscii(address2_county);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_county = address2_county;
return _x;
}
@Property(name="shippingmethodcode")
@JsonIgnore
public Optional getShippingmethodcode() {
return Optional.ofNullable(shippingmethodcode);
}
public Contact withShippingmethodcode(Integer shippingmethodcode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.shippingmethodcode = shippingmethodcode;
return _x;
}
@Property(name="anniversary")
@JsonIgnore
public Optional getAnniversary() {
return Optional.ofNullable(anniversary);
}
public Contact withAnniversary(LocalDate anniversary) {
Contact _x = _copy();
_x.changedFields = changedFields.add("anniversary");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.anniversary = anniversary;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Contact with_ownerid_value(String _ownerid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="_parentcontactid_value")
@JsonIgnore
public Optional get_parentcontactid_value() {
return Optional.ofNullable(_parentcontactid_value);
}
public Contact with_parentcontactid_value(String _parentcontactid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_parentcontactid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._parentcontactid_value = _parentcontactid_value;
return _x;
}
@Property(name="haschildrencode")
@JsonIgnore
public Optional getHaschildrencode() {
return Optional.ofNullable(haschildrencode);
}
public Contact withHaschildrencode(Integer haschildrencode) {
Contact _x = _copy();
_x.changedFields = changedFields.add("haschildrencode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.haschildrencode = haschildrencode;
return _x;
}
@Property(name="address2_fax")
@JsonIgnore
public Optional getAddress2_fax() {
return Optional.ofNullable(address2_fax);
}
public Contact withAddress2_fax(String address2_fax) {
Checks.checkIsAscii(address2_fax);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_fax = address2_fax;
return _x;
}
@Property(name="address2_composite")
@JsonIgnore
public Optional getAddress2_composite() {
return Optional.ofNullable(address2_composite);
}
public Contact withAddress2_composite(String address2_composite) {
Checks.checkIsAscii(address2_composite);
Contact _x = _copy();
_x.changedFields = changedFields.add("address2_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address2_composite = address2_composite;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Contact withCreatedon(OffsetDateTime createdon) {
Contact _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.createdon = createdon;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Contact withEntityimage_url(String entityimage_url) {
Checks.checkIsAscii(entityimage_url);
Contact _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="address1_line3")
@JsonIgnore
public Optional getAddress1_line3() {
return Optional.ofNullable(address1_line3);
}
public Contact withAddress1_line3(String address1_line3) {
Checks.checkIsAscii(address1_line3);
Contact _x = _copy();
_x.changedFields = changedFields.add("address1_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address1_line3 = address1_line3;
return _x;
}
@Property(name="_slaid_value")
@JsonIgnore
public Optional get_slaid_value() {
return Optional.ofNullable(_slaid_value);
}
public Contact with_slaid_value(String _slaid_value) {
Contact _x = _copy();
_x.changedFields = changedFields.add("_slaid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x._slaid_value = _slaid_value;
return _x;
}
@Property(name="middlename")
@JsonIgnore
public Optional getMiddlename() {
return Optional.ofNullable(middlename);
}
public Contact withMiddlename(String middlename) {
Checks.checkIsAscii(middlename);
Contact _x = _copy();
_x.changedFields = changedFields.add("middlename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.middlename = middlename;
return _x;
}
@Property(name="address3_line3")
@JsonIgnore
public Optional getAddress3_line3() {
return Optional.ofNullable(address3_line3);
}
public Contact withAddress3_line3(String address3_line3) {
Checks.checkIsAscii(address3_line3);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_line3 = address3_line3;
return _x;
}
@Property(name="address3_telephone2")
@JsonIgnore
public Optional getAddress3_telephone2() {
return Optional.ofNullable(address3_telephone2);
}
public Contact withAddress3_telephone2(String address3_telephone2) {
Checks.checkIsAscii(address3_telephone2);
Contact _x = _copy();
_x.changedFields = changedFields.add("address3_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.address3_telephone2 = address3_telephone2;
return _x;
}
@Property(name="timespentbymeonemailandmeetings")
@JsonIgnore
public Optional getTimespentbymeonemailandmeetings() {
return Optional.ofNullable(timespentbymeonemailandmeetings);
}
public Contact withTimespentbymeonemailandmeetings(String timespentbymeonemailandmeetings) {
Checks.checkIsAscii(timespentbymeonemailandmeetings);
Contact _x = _copy();
_x.changedFields = changedFields.add("timespentbymeonemailandmeetings");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.contact");
_x.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
return _x;
}
@NavigationProperty(name="contact_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getContact_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("contact_principalobjectattributeaccess"));
}
@NavigationProperty(name="contact_connections1")
@JsonIgnore
public ConnectionCollectionRequest getContact_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("contact_connections1"));
}
@NavigationProperty(name="Contact_Feedback")
@JsonIgnore
public FeedbackCollectionRequest getContact_Feedback() {
return new FeedbackCollectionRequest(
contextPath.addSegment("Contact_Feedback"));
}
@NavigationProperty(name="Contact_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getContact_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Contact_ProcessSessions"));
}
@NavigationProperty(name="stageid_processstage")
@JsonIgnore
public ProcessstageRequest getStageid_processstage() {
return new ProcessstageRequest(contextPath.addSegment("stageid_processstage"));
}
@NavigationProperty(name="Contact_ActivityPointers")
@JsonIgnore
public ActivitypointerCollectionRequest getContact_ActivityPointers() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("Contact_ActivityPointers"));
}
@NavigationProperty(name="Contact_SocialActivities")
@JsonIgnore
public SocialactivityCollectionRequest getContact_SocialActivities() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("Contact_SocialActivities"));
}
@NavigationProperty(name="lk_contact_feedback_createdonbehalfby")
@JsonIgnore
public FeedbackCollectionRequest getLk_contact_feedback_createdonbehalfby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_contact_feedback_createdonbehalfby"));
}
@NavigationProperty(name="contact_PostFollows")
@JsonIgnore
public PostfollowCollectionRequest getContact_PostFollows() {
return new PostfollowCollectionRequest(
contextPath.addSegment("contact_PostFollows"));
}
@NavigationProperty(name="contact_PostRegardings")
@JsonIgnore
public PostregardingCollectionRequest getContact_PostRegardings() {
return new PostregardingCollectionRequest(
contextPath.addSegment("contact_PostRegardings"));
}
@NavigationProperty(name="socialactivity_postauthor_contacts")
@JsonIgnore
public SocialactivityCollectionRequest getSocialactivity_postauthor_contacts() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("socialactivity_postauthor_contacts"));
}
@NavigationProperty(name="Contact_Phonecalls")
@JsonIgnore
public PhonecallCollectionRequest getContact_Phonecalls() {
return new PhonecallCollectionRequest(
contextPath.addSegment("Contact_Phonecalls"));
}
@NavigationProperty(name="Contact_Tasks")
@JsonIgnore
public TaskCollectionRequest getContact_Tasks() {
return new TaskCollectionRequest(
contextPath.addSegment("Contact_Tasks"));
}
@NavigationProperty(name="Contact_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getContact_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Contact_SyncErrors"));
}
@NavigationProperty(name="slainvokedid_contact_sla")
@JsonIgnore
public SlaRequest getSlainvokedid_contact_sla() {
return new SlaRequest(contextPath.addSegment("slainvokedid_contact_sla"));
}
@NavigationProperty(name="sla_contact_sla")
@JsonIgnore
public SlaRequest getSla_contact_sla() {
return new SlaRequest(contextPath.addSegment("sla_contact_sla"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="Contact_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getContact_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Contact_Annotation"));
}
@NavigationProperty(name="masterid")
@JsonIgnore
public ContactRequest getMasterid() {
return new ContactRequest(contextPath.addSegment("masterid"));
}
@NavigationProperty(name="contact_master_contact")
@JsonIgnore
public ContactCollectionRequest getContact_master_contact() {
return new ContactCollectionRequest(
contextPath.addSegment("contact_master_contact"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="Contact_Email_EmailSender")
@JsonIgnore
public EmailCollectionRequest getContact_Email_EmailSender() {
return new EmailCollectionRequest(
contextPath.addSegment("Contact_Email_EmailSender"));
}
@NavigationProperty(name="account_primary_contact")
@JsonIgnore
public AccountCollectionRequest getAccount_primary_contact() {
return new AccountCollectionRequest(
contextPath.addSegment("account_primary_contact"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="contact_actioncard")
@JsonIgnore
public ActioncardCollectionRequest getContact_actioncard() {
return new ActioncardCollectionRequest(
contextPath.addSegment("contact_actioncard"));
}
@NavigationProperty(name="socialactivity_postauthoraccount_contacts")
@JsonIgnore
public SocialactivityCollectionRequest getSocialactivity_postauthoraccount_contacts() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("socialactivity_postauthoraccount_contacts"));
}
@NavigationProperty(name="Socialprofile_customer_contacts")
@JsonIgnore
public SocialprofileCollectionRequest getSocialprofile_customer_contacts() {
return new SocialprofileCollectionRequest(
contextPath.addSegment("Socialprofile_customer_contacts"));
}
@NavigationProperty(name="Contact_CustomerAddress")
@JsonIgnore
public CustomeraddressCollectionRequest getContact_CustomerAddress() {
return new CustomeraddressCollectionRequest(
contextPath.addSegment("Contact_CustomerAddress"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
@NavigationProperty(name="parentcustomerid_account")
@JsonIgnore
public AccountRequest getParentcustomerid_account() {
return new AccountRequest(contextPath.addSegment("parentcustomerid_account"));
}
@NavigationProperty(name="Contact_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getContact_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Contact_DuplicateMatchingRecord"));
}
@NavigationProperty(name="lk_contact_feedback_createdby")
@JsonIgnore
public FeedbackCollectionRequest getLk_contact_feedback_createdby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_contact_feedback_createdby"));
}
@NavigationProperty(name="slakpiinstance_contact")
@JsonIgnore
public SlakpiinstanceCollectionRequest getSlakpiinstance_contact() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("slakpiinstance_contact"));
}
@NavigationProperty(name="preferredsystemuserid")
@JsonIgnore
public SystemuserRequest getPreferredsystemuserid() {
return new SystemuserRequest(contextPath.addSegment("preferredsystemuserid"));
}
@NavigationProperty(name="Contact_Faxes")
@JsonIgnore
public FaxCollectionRequest getContact_Faxes() {
return new FaxCollectionRequest(
contextPath.addSegment("Contact_Faxes"));
}
@NavigationProperty(name="Contact_MailboxTrackingFolder")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getContact_MailboxTrackingFolder() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("Contact_MailboxTrackingFolder"));
}
@NavigationProperty(name="Contact_Appointments")
@JsonIgnore
public AppointmentCollectionRequest getContact_Appointments() {
return new AppointmentCollectionRequest(
contextPath.addSegment("Contact_Appointments"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
@NavigationProperty(name="Contact_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getContact_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Contact_AsyncOperations"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"));
}
@NavigationProperty(name="Contact_RecurringAppointmentMasters")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getContact_RecurringAppointmentMasters() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("Contact_RecurringAppointmentMasters"));
}
@NavigationProperty(name="Contact_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getContact_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("Contact_BulkDeleteFailures"));
}
@NavigationProperty(name="parentcustomerid_contact")
@JsonIgnore
public ContactRequest getParentcustomerid_contact() {
return new ContactRequest(contextPath.addSegment("parentcustomerid_contact"));
}
@NavigationProperty(name="contact_customer_contacts")
@JsonIgnore
public ContactCollectionRequest getContact_customer_contacts() {
return new ContactCollectionRequest(
contextPath.addSegment("contact_customer_contacts"));
}
@NavigationProperty(name="contact_connections2")
@JsonIgnore
public ConnectionCollectionRequest getContact_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("contact_connections2"));
}
@NavigationProperty(name="Contact_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getContact_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Contact_DuplicateBaseRecord"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="Contact_Emails")
@JsonIgnore
public EmailCollectionRequest getContact_Emails() {
return new EmailCollectionRequest(
contextPath.addSegment("Contact_Emails"));
}
@NavigationProperty(name="contact_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getContact_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("contact_activity_parties"));
}
@NavigationProperty(name="Contact_Letters")
@JsonIgnore
public LetterCollectionRequest getContact_Letters() {
return new LetterCollectionRequest(
contextPath.addSegment("Contact_Letters"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Contact patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Contact _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Contact put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Contact _x = _copy();
_x.changedFields = null;
return _x;
}
private Contact _copy() {
Contact _x = new Contact();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.spousesname = spousesname;
_x.emailaddress3 = emailaddress3;
_x.address3_telephone3 = address3_telephone3;
_x.entityimage_timestamp = entityimage_timestamp;
_x.address2_shippingmethodcode = address2_shippingmethodcode;
_x.mobilephone = mobilephone;
_x.address3_shippingmethodcode = address3_shippingmethodcode;
_x.address3_postalcode = address3_postalcode;
_x.versionnumber = versionnumber;
_x.annualincome = annualincome;
_x.fax = fax;
_x.telephone3 = telephone3;
_x.preferredappointmentdaycode = preferredappointmentdaycode;
_x.address3_city = address3_city;
_x.lastonholdtime = lastonholdtime;
_x.address2_stateorprovince = address2_stateorprovince;
_x._createdby_value = _createdby_value;
_x.address2_line1 = address2_line1;
_x.assistantphone = assistantphone;
_x.lastusedincampaign = lastusedincampaign;
_x.address3_freighttermscode = address3_freighttermscode;
_x.pager = pager;
_x.employeeid = employeeid;
_x.territorycode = territorycode;
_x._parentcustomerid_value = _parentcustomerid_value;
_x.managername = managername;
_x.birthdate = birthdate;
_x.address1_name = address1_name;
_x.department = department;
_x.address3_country = address3_country;
_x.address2_telephone1 = address2_telephone1;
_x.address1_postofficebox = address1_postofficebox;
_x.address2_primarycontactname = address2_primarycontactname;
_x.address2_latitude = address2_latitude;
_x._owningteam_value = _owningteam_value;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.home2 = home2;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.address2_postalcode = address2_postalcode;
_x._owninguser_value = _owninguser_value;
_x.merged = merged;
_x._masterid_value = _masterid_value;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.address3_postofficebox = address3_postofficebox;
_x.customertypecode = customertypecode;
_x.business2 = business2;
_x.address3_county = address3_county;
_x.address1_latitude = address1_latitude;
_x.address1_freighttermscode = address1_freighttermscode;
_x.traversedpath = traversedpath;
_x.address3_addresstypecode = address3_addresstypecode;
_x.address1_longitude = address1_longitude;
_x.address1_addresstypecode = address1_addresstypecode;
_x.statuscode = statuscode;
_x.yomifullname = yomifullname;
_x.aging90_base = aging90_base;
_x._accountid_value = _accountid_value;
_x.address3_primarycontactname = address3_primarycontactname;
_x.familystatuscode = familystatuscode;
_x.address3_addressid = address3_addressid;
_x.leadsourcecode = leadsourcecode;
_x.statecode = statecode;
_x.address2_freighttermscode = address2_freighttermscode;
_x.address1_composite = address1_composite;
_x.telephone2 = telephone2;
_x.yomimiddlename = yomimiddlename;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.jobtitle = jobtitle;
_x.address2_addresstypecode = address2_addresstypecode;
_x.educationcode = educationcode;
_x.address1_telephone3 = address1_telephone3;
_x.followemail = followemail;
_x.importsequencenumber = importsequencenumber;
_x.gendercode = gendercode;
_x.address2_line2 = address2_line2;
_x.creditlimit_base = creditlimit_base;
_x.annualincome_base = annualincome_base;
_x.donotfax = donotfax;
_x.address3_line1 = address3_line1;
_x.address1_county = address1_county;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._createdbyexternalparty_value = _createdbyexternalparty_value;
_x.entityimageid = entityimageid;
_x.processid = processid;
_x.address1_telephone2 = address1_telephone2;
_x.description = description;
_x.address1_fax = address1_fax;
_x.marketingonly = marketingonly;
_x.address3_line2 = address3_line2;
_x.address1_addressid = address1_addressid;
_x.donotpostalmail = donotpostalmail;
_x.address2_utcoffset = address2_utcoffset;
_x.exchangerate = exchangerate;
_x.aging30_base = aging30_base;
_x.callback = callback;
_x.emailaddress2 = emailaddress2;
_x.address2_line3 = address2_line3;
_x.managerphone = managerphone;
_x.websiteurl = websiteurl;
_x.overriddencreatedon = overriddencreatedon;
_x.firstname = firstname;
_x.address1_telephone1 = address1_telephone1;
_x.address3_composite = address3_composite;
_x.address3_fax = address3_fax;
_x.childrensnames = childrensnames;
_x.preferredcontactmethodcode = preferredcontactmethodcode;
_x.numberofchildren = numberofchildren;
_x.subscriptionid = subscriptionid;
_x.yomilastname = yomilastname;
_x.address2_postofficebox = address2_postofficebox;
_x.aging90 = aging90;
_x.donotbulkpostalmail = donotbulkpostalmail;
_x.emailaddress1 = emailaddress1;
_x.donotbulkemail = donotbulkemail;
_x.customersizecode = customersizecode;
_x.address1_city = address1_city;
_x.preferredappointmenttimecode = preferredappointmenttimecode;
_x.address3_latitude = address3_latitude;
_x.aging60_base = aging60_base;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.entityimage = entityimage;
_x._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
_x.paymenttermscode = paymenttermscode;
_x.address3_name = address3_name;
_x.participatesinworkflow = participatesinworkflow;
_x.address1_shippingmethodcode = address1_shippingmethodcode;
_x._modifiedby_value = _modifiedby_value;
_x.ftpsiteurl = ftpsiteurl;
_x._preferredsystemuserid_value = _preferredsystemuserid_value;
_x.address2_telephone2 = address2_telephone2;
_x._slainvokedid_value = _slainvokedid_value;
_x.address3_utcoffset = address3_utcoffset;
_x.address3_stateorprovince = address3_stateorprovince;
_x.address3_telephone1 = address3_telephone1;
_x.nickname = nickname;
_x.fullname = fullname;
_x.assistantname = assistantname;
_x.address2_country = address2_country;
_x.aging60 = aging60;
_x.modifiedon = modifiedon;
_x.externaluseridentifier = externaluseridentifier;
_x.address2_name = address2_name;
_x.stageid = stageid;
_x.creditonhold = creditonhold;
_x.address3_longitude = address3_longitude;
_x.onholdtime = onholdtime;
_x.address2_telephone3 = address2_telephone3;
_x.donotphone = donotphone;
_x.address3_upszone = address3_upszone;
_x.contactid = contactid;
_x.aging30 = aging30;
_x.address2_upszone = address2_upszone;
_x.address1_upszone = address1_upszone;
_x.creditlimit = creditlimit;
_x.accountrolecode = accountrolecode;
_x.salutation = salutation;
_x.suffix = suffix;
_x.address1_primarycontactname = address1_primarycontactname;
_x.address1_utcoffset = address1_utcoffset;
_x.address1_postalcode = address1_postalcode;
_x.governmentid = governmentid;
_x.donotsendmm = donotsendmm;
_x.address1_country = address1_country;
_x.lastname = lastname;
_x.address2_city = address2_city;
_x.donotemail = donotemail;
_x.company = company;
_x.address1_line2 = address1_line2;
_x.address2_longitude = address2_longitude;
_x.address1_stateorprovince = address1_stateorprovince;
_x.yomifirstname = yomifirstname;
_x.telephone1 = telephone1;
_x.address1_line1 = address1_line1;
_x.address2_addressid = address2_addressid;
_x.isbackofficecustomer = isbackofficecustomer;
_x.address2_county = address2_county;
_x.shippingmethodcode = shippingmethodcode;
_x.anniversary = anniversary;
_x._ownerid_value = _ownerid_value;
_x._parentcontactid_value = _parentcontactid_value;
_x.haschildrencode = haschildrencode;
_x.address2_fax = address2_fax;
_x.address2_composite = address2_composite;
_x.createdon = createdon;
_x.entityimage_url = entityimage_url;
_x.address1_line3 = address1_line3;
_x._slaid_value = _slaid_value;
_x.middlename = middlename;
_x.address3_line3 = address3_line3;
_x.address3_telephone2 = address3_telephone2;
_x.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Contact[");
b.append("spousesname=");
b.append(this.spousesname);
b.append(", ");
b.append("emailaddress3=");
b.append(this.emailaddress3);
b.append(", ");
b.append("address3_telephone3=");
b.append(this.address3_telephone3);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("address2_shippingmethodcode=");
b.append(this.address2_shippingmethodcode);
b.append(", ");
b.append("mobilephone=");
b.append(this.mobilephone);
b.append(", ");
b.append("address3_shippingmethodcode=");
b.append(this.address3_shippingmethodcode);
b.append(", ");
b.append("address3_postalcode=");
b.append(this.address3_postalcode);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("annualincome=");
b.append(this.annualincome);
b.append(", ");
b.append("fax=");
b.append(this.fax);
b.append(", ");
b.append("telephone3=");
b.append(this.telephone3);
b.append(", ");
b.append("preferredappointmentdaycode=");
b.append(this.preferredappointmentdaycode);
b.append(", ");
b.append("address3_city=");
b.append(this.address3_city);
b.append(", ");
b.append("lastonholdtime=");
b.append(this.lastonholdtime);
b.append(", ");
b.append("address2_stateorprovince=");
b.append(this.address2_stateorprovince);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("address2_line1=");
b.append(this.address2_line1);
b.append(", ");
b.append("assistantphone=");
b.append(this.assistantphone);
b.append(", ");
b.append("lastusedincampaign=");
b.append(this.lastusedincampaign);
b.append(", ");
b.append("address3_freighttermscode=");
b.append(this.address3_freighttermscode);
b.append(", ");
b.append("pager=");
b.append(this.pager);
b.append(", ");
b.append("employeeid=");
b.append(this.employeeid);
b.append(", ");
b.append("territorycode=");
b.append(this.territorycode);
b.append(", ");
b.append("_parentcustomerid_value=");
b.append(this._parentcustomerid_value);
b.append(", ");
b.append("managername=");
b.append(this.managername);
b.append(", ");
b.append("birthdate=");
b.append(this.birthdate);
b.append(", ");
b.append("address1_name=");
b.append(this.address1_name);
b.append(", ");
b.append("department=");
b.append(this.department);
b.append(", ");
b.append("address3_country=");
b.append(this.address3_country);
b.append(", ");
b.append("address2_telephone1=");
b.append(this.address2_telephone1);
b.append(", ");
b.append("address1_postofficebox=");
b.append(this.address1_postofficebox);
b.append(", ");
b.append("address2_primarycontactname=");
b.append(this.address2_primarycontactname);
b.append(", ");
b.append("address2_latitude=");
b.append(this.address2_latitude);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("home2=");
b.append(this.home2);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("address2_postalcode=");
b.append(this.address2_postalcode);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("merged=");
b.append(this.merged);
b.append(", ");
b.append("_masterid_value=");
b.append(this._masterid_value);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("address3_postofficebox=");
b.append(this.address3_postofficebox);
b.append(", ");
b.append("customertypecode=");
b.append(this.customertypecode);
b.append(", ");
b.append("business2=");
b.append(this.business2);
b.append(", ");
b.append("address3_county=");
b.append(this.address3_county);
b.append(", ");
b.append("address1_latitude=");
b.append(this.address1_latitude);
b.append(", ");
b.append("address1_freighttermscode=");
b.append(this.address1_freighttermscode);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("address3_addresstypecode=");
b.append(this.address3_addresstypecode);
b.append(", ");
b.append("address1_longitude=");
b.append(this.address1_longitude);
b.append(", ");
b.append("address1_addresstypecode=");
b.append(this.address1_addresstypecode);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("yomifullname=");
b.append(this.yomifullname);
b.append(", ");
b.append("aging90_base=");
b.append(this.aging90_base);
b.append(", ");
b.append("_accountid_value=");
b.append(this._accountid_value);
b.append(", ");
b.append("address3_primarycontactname=");
b.append(this.address3_primarycontactname);
b.append(", ");
b.append("familystatuscode=");
b.append(this.familystatuscode);
b.append(", ");
b.append("address3_addressid=");
b.append(this.address3_addressid);
b.append(", ");
b.append("leadsourcecode=");
b.append(this.leadsourcecode);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("address2_freighttermscode=");
b.append(this.address2_freighttermscode);
b.append(", ");
b.append("address1_composite=");
b.append(this.address1_composite);
b.append(", ");
b.append("telephone2=");
b.append(this.telephone2);
b.append(", ");
b.append("yomimiddlename=");
b.append(this.yomimiddlename);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("jobtitle=");
b.append(this.jobtitle);
b.append(", ");
b.append("address2_addresstypecode=");
b.append(this.address2_addresstypecode);
b.append(", ");
b.append("educationcode=");
b.append(this.educationcode);
b.append(", ");
b.append("address1_telephone3=");
b.append(this.address1_telephone3);
b.append(", ");
b.append("followemail=");
b.append(this.followemail);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("gendercode=");
b.append(this.gendercode);
b.append(", ");
b.append("address2_line2=");
b.append(this.address2_line2);
b.append(", ");
b.append("creditlimit_base=");
b.append(this.creditlimit_base);
b.append(", ");
b.append("annualincome_base=");
b.append(this.annualincome_base);
b.append(", ");
b.append("donotfax=");
b.append(this.donotfax);
b.append(", ");
b.append("address3_line1=");
b.append(this.address3_line1);
b.append(", ");
b.append("address1_county=");
b.append(this.address1_county);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("_createdbyexternalparty_value=");
b.append(this._createdbyexternalparty_value);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("address1_telephone2=");
b.append(this.address1_telephone2);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("address1_fax=");
b.append(this.address1_fax);
b.append(", ");
b.append("marketingonly=");
b.append(this.marketingonly);
b.append(", ");
b.append("address3_line2=");
b.append(this.address3_line2);
b.append(", ");
b.append("address1_addressid=");
b.append(this.address1_addressid);
b.append(", ");
b.append("donotpostalmail=");
b.append(this.donotpostalmail);
b.append(", ");
b.append("address2_utcoffset=");
b.append(this.address2_utcoffset);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("aging30_base=");
b.append(this.aging30_base);
b.append(", ");
b.append("callback=");
b.append(this.callback);
b.append(", ");
b.append("emailaddress2=");
b.append(this.emailaddress2);
b.append(", ");
b.append("address2_line3=");
b.append(this.address2_line3);
b.append(", ");
b.append("managerphone=");
b.append(this.managerphone);
b.append(", ");
b.append("websiteurl=");
b.append(this.websiteurl);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("firstname=");
b.append(this.firstname);
b.append(", ");
b.append("address1_telephone1=");
b.append(this.address1_telephone1);
b.append(", ");
b.append("address3_composite=");
b.append(this.address3_composite);
b.append(", ");
b.append("address3_fax=");
b.append(this.address3_fax);
b.append(", ");
b.append("childrensnames=");
b.append(this.childrensnames);
b.append(", ");
b.append("preferredcontactmethodcode=");
b.append(this.preferredcontactmethodcode);
b.append(", ");
b.append("numberofchildren=");
b.append(this.numberofchildren);
b.append(", ");
b.append("subscriptionid=");
b.append(this.subscriptionid);
b.append(", ");
b.append("yomilastname=");
b.append(this.yomilastname);
b.append(", ");
b.append("address2_postofficebox=");
b.append(this.address2_postofficebox);
b.append(", ");
b.append("aging90=");
b.append(this.aging90);
b.append(", ");
b.append("donotbulkpostalmail=");
b.append(this.donotbulkpostalmail);
b.append(", ");
b.append("emailaddress1=");
b.append(this.emailaddress1);
b.append(", ");
b.append("donotbulkemail=");
b.append(this.donotbulkemail);
b.append(", ");
b.append("customersizecode=");
b.append(this.customersizecode);
b.append(", ");
b.append("address1_city=");
b.append(this.address1_city);
b.append(", ");
b.append("preferredappointmenttimecode=");
b.append(this.preferredappointmenttimecode);
b.append(", ");
b.append("address3_latitude=");
b.append(this.address3_latitude);
b.append(", ");
b.append("aging60_base=");
b.append(this.aging60_base);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("_modifiedbyexternalparty_value=");
b.append(this._modifiedbyexternalparty_value);
b.append(", ");
b.append("paymenttermscode=");
b.append(this.paymenttermscode);
b.append(", ");
b.append("address3_name=");
b.append(this.address3_name);
b.append(", ");
b.append("participatesinworkflow=");
b.append(this.participatesinworkflow);
b.append(", ");
b.append("address1_shippingmethodcode=");
b.append(this.address1_shippingmethodcode);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("ftpsiteurl=");
b.append(this.ftpsiteurl);
b.append(", ");
b.append("_preferredsystemuserid_value=");
b.append(this._preferredsystemuserid_value);
b.append(", ");
b.append("address2_telephone2=");
b.append(this.address2_telephone2);
b.append(", ");
b.append("_slainvokedid_value=");
b.append(this._slainvokedid_value);
b.append(", ");
b.append("address3_utcoffset=");
b.append(this.address3_utcoffset);
b.append(", ");
b.append("address3_stateorprovince=");
b.append(this.address3_stateorprovince);
b.append(", ");
b.append("address3_telephone1=");
b.append(this.address3_telephone1);
b.append(", ");
b.append("nickname=");
b.append(this.nickname);
b.append(", ");
b.append("fullname=");
b.append(this.fullname);
b.append(", ");
b.append("assistantname=");
b.append(this.assistantname);
b.append(", ");
b.append("address2_country=");
b.append(this.address2_country);
b.append(", ");
b.append("aging60=");
b.append(this.aging60);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("externaluseridentifier=");
b.append(this.externaluseridentifier);
b.append(", ");
b.append("address2_name=");
b.append(this.address2_name);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("creditonhold=");
b.append(this.creditonhold);
b.append(", ");
b.append("address3_longitude=");
b.append(this.address3_longitude);
b.append(", ");
b.append("onholdtime=");
b.append(this.onholdtime);
b.append(", ");
b.append("address2_telephone3=");
b.append(this.address2_telephone3);
b.append(", ");
b.append("donotphone=");
b.append(this.donotphone);
b.append(", ");
b.append("address3_upszone=");
b.append(this.address3_upszone);
b.append(", ");
b.append("contactid=");
b.append(this.contactid);
b.append(", ");
b.append("aging30=");
b.append(this.aging30);
b.append(", ");
b.append("address2_upszone=");
b.append(this.address2_upszone);
b.append(", ");
b.append("address1_upszone=");
b.append(this.address1_upszone);
b.append(", ");
b.append("creditlimit=");
b.append(this.creditlimit);
b.append(", ");
b.append("accountrolecode=");
b.append(this.accountrolecode);
b.append(", ");
b.append("salutation=");
b.append(this.salutation);
b.append(", ");
b.append("suffix=");
b.append(this.suffix);
b.append(", ");
b.append("address1_primarycontactname=");
b.append(this.address1_primarycontactname);
b.append(", ");
b.append("address1_utcoffset=");
b.append(this.address1_utcoffset);
b.append(", ");
b.append("address1_postalcode=");
b.append(this.address1_postalcode);
b.append(", ");
b.append("governmentid=");
b.append(this.governmentid);
b.append(", ");
b.append("donotsendmm=");
b.append(this.donotsendmm);
b.append(", ");
b.append("address1_country=");
b.append(this.address1_country);
b.append(", ");
b.append("lastname=");
b.append(this.lastname);
b.append(", ");
b.append("address2_city=");
b.append(this.address2_city);
b.append(", ");
b.append("donotemail=");
b.append(this.donotemail);
b.append(", ");
b.append("company=");
b.append(this.company);
b.append(", ");
b.append("address1_line2=");
b.append(this.address1_line2);
b.append(", ");
b.append("address2_longitude=");
b.append(this.address2_longitude);
b.append(", ");
b.append("address1_stateorprovince=");
b.append(this.address1_stateorprovince);
b.append(", ");
b.append("yomifirstname=");
b.append(this.yomifirstname);
b.append(", ");
b.append("telephone1=");
b.append(this.telephone1);
b.append(", ");
b.append("address1_line1=");
b.append(this.address1_line1);
b.append(", ");
b.append("address2_addressid=");
b.append(this.address2_addressid);
b.append(", ");
b.append("isbackofficecustomer=");
b.append(this.isbackofficecustomer);
b.append(", ");
b.append("address2_county=");
b.append(this.address2_county);
b.append(", ");
b.append("shippingmethodcode=");
b.append(this.shippingmethodcode);
b.append(", ");
b.append("anniversary=");
b.append(this.anniversary);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("_parentcontactid_value=");
b.append(this._parentcontactid_value);
b.append(", ");
b.append("haschildrencode=");
b.append(this.haschildrencode);
b.append(", ");
b.append("address2_fax=");
b.append(this.address2_fax);
b.append(", ");
b.append("address2_composite=");
b.append(this.address2_composite);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("address1_line3=");
b.append(this.address1_line3);
b.append(", ");
b.append("_slaid_value=");
b.append(this._slaid_value);
b.append(", ");
b.append("middlename=");
b.append(this.middlename);
b.append(", ");
b.append("address3_line3=");
b.append(this.address3_line3);
b.append(", ");
b.append("address3_telephone2=");
b.append(this.address3_telephone2);
b.append(", ");
b.append("timespentbymeonemailandmeetings=");
b.append(this.timespentbymeonemailandmeetings);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy