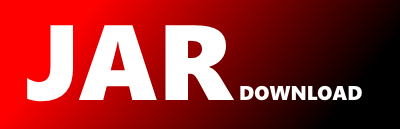
microsoft.dynamics.crm.entity.Customeraddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"_modifiedby_value",
"addresstypecode",
"_ownerid_value",
"customeraddressid",
"_createdonbehalfby_value",
"line2",
"utcconversiontimezonecode",
"addressnumber",
"utcoffset",
"line3",
"shippingmethodcode",
"importsequencenumber",
"line1",
"versionnumber",
"_parentid_value",
"postalcode",
"freighttermscode",
"exchangerate",
"country",
"county",
"createdon",
"_owningbusinessunit_value",
"stateorprovince",
"_createdby_value",
"_transactioncurrencyid_value",
"primarycontactname",
"telephone1",
"city",
"_owninguser_value",
"fax",
"_modifiedonbehalfby_value",
"objecttypecode",
"telephone2",
"upszone",
"composite",
"longitude",
"timezoneruleversionnumber",
"telephone3",
"modifiedon",
"postofficebox",
"overriddencreatedon",
"latitude",
"name"})
@JsonInclude(Include.NON_NULL)
public class Customeraddress extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.customeraddress";
}
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("addresstypecode")
protected Integer addresstypecode;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("customeraddressid")
protected String customeraddressid;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("line2")
protected String line2;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("addressnumber")
protected Integer addressnumber;
@JsonProperty("utcoffset")
protected Integer utcoffset;
@JsonProperty("line3")
protected String line3;
@JsonProperty("shippingmethodcode")
protected Integer shippingmethodcode;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("line1")
protected String line1;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("_parentid_value")
protected String _parentid_value;
@JsonProperty("postalcode")
protected String postalcode;
@JsonProperty("freighttermscode")
protected Integer freighttermscode;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("country")
protected String country;
@JsonProperty("county")
protected String county;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("stateorprovince")
protected String stateorprovince;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("_transactioncurrencyid_value")
protected String _transactioncurrencyid_value;
@JsonProperty("primarycontactname")
protected String primarycontactname;
@JsonProperty("telephone1")
protected String telephone1;
@JsonProperty("city")
protected String city;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("fax")
protected String fax;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("objecttypecode")
protected String objecttypecode;
@JsonProperty("telephone2")
protected String telephone2;
@JsonProperty("upszone")
protected String upszone;
@JsonProperty("composite")
protected String composite;
@JsonProperty("longitude")
protected Double longitude;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("telephone3")
protected String telephone3;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("postofficebox")
protected String postofficebox;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("latitude")
protected Double latitude;
@JsonProperty("name")
protected String name;
protected Customeraddress() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderCustomeraddress() {
return new Builder();
}
public static final class Builder {
private String _modifiedby_value;
private Integer addresstypecode;
private String _ownerid_value;
private String customeraddressid;
private String _createdonbehalfby_value;
private String line2;
private Integer utcconversiontimezonecode;
private Integer addressnumber;
private Integer utcoffset;
private String line3;
private Integer shippingmethodcode;
private Integer importsequencenumber;
private String line1;
private Long versionnumber;
private String _parentid_value;
private String postalcode;
private Integer freighttermscode;
private BigDecimal exchangerate;
private String country;
private String county;
private OffsetDateTime createdon;
private String _owningbusinessunit_value;
private String stateorprovince;
private String _createdby_value;
private String _transactioncurrencyid_value;
private String primarycontactname;
private String telephone1;
private String city;
private String _owninguser_value;
private String fax;
private String _modifiedonbehalfby_value;
private String objecttypecode;
private String telephone2;
private String upszone;
private String composite;
private Double longitude;
private Integer timezoneruleversionnumber;
private String telephone3;
private OffsetDateTime modifiedon;
private String postofficebox;
private OffsetDateTime overriddencreatedon;
private Double latitude;
private String name;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder addresstypecode(Integer addresstypecode) {
this.addresstypecode = addresstypecode;
this.changedFields = changedFields.add("addresstypecode");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder customeraddressid(String customeraddressid) {
this.customeraddressid = customeraddressid;
this.changedFields = changedFields.add("customeraddressid");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder line2(String line2) {
this.line2 = line2;
this.changedFields = changedFields.add("line2");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder addressnumber(Integer addressnumber) {
this.addressnumber = addressnumber;
this.changedFields = changedFields.add("addressnumber");
return this;
}
public Builder utcoffset(Integer utcoffset) {
this.utcoffset = utcoffset;
this.changedFields = changedFields.add("utcoffset");
return this;
}
public Builder line3(String line3) {
this.line3 = line3;
this.changedFields = changedFields.add("line3");
return this;
}
public Builder shippingmethodcode(Integer shippingmethodcode) {
this.shippingmethodcode = shippingmethodcode;
this.changedFields = changedFields.add("shippingmethodcode");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder line1(String line1) {
this.line1 = line1;
this.changedFields = changedFields.add("line1");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder _parentid_value(String _parentid_value) {
this._parentid_value = _parentid_value;
this.changedFields = changedFields.add("_parentid_value");
return this;
}
public Builder postalcode(String postalcode) {
this.postalcode = postalcode;
this.changedFields = changedFields.add("postalcode");
return this;
}
public Builder freighttermscode(Integer freighttermscode) {
this.freighttermscode = freighttermscode;
this.changedFields = changedFields.add("freighttermscode");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder country(String country) {
this.country = country;
this.changedFields = changedFields.add("country");
return this;
}
public Builder county(String county) {
this.county = county;
this.changedFields = changedFields.add("county");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder stateorprovince(String stateorprovince) {
this.stateorprovince = stateorprovince;
this.changedFields = changedFields.add("stateorprovince");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder _transactioncurrencyid_value(String _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder primarycontactname(String primarycontactname) {
this.primarycontactname = primarycontactname;
this.changedFields = changedFields.add("primarycontactname");
return this;
}
public Builder telephone1(String telephone1) {
this.telephone1 = telephone1;
this.changedFields = changedFields.add("telephone1");
return this;
}
public Builder city(String city) {
this.city = city;
this.changedFields = changedFields.add("city");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder fax(String fax) {
this.fax = fax;
this.changedFields = changedFields.add("fax");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder objecttypecode(String objecttypecode) {
this.objecttypecode = objecttypecode;
this.changedFields = changedFields.add("objecttypecode");
return this;
}
public Builder telephone2(String telephone2) {
this.telephone2 = telephone2;
this.changedFields = changedFields.add("telephone2");
return this;
}
public Builder upszone(String upszone) {
this.upszone = upszone;
this.changedFields = changedFields.add("upszone");
return this;
}
public Builder composite(String composite) {
this.composite = composite;
this.changedFields = changedFields.add("composite");
return this;
}
public Builder longitude(Double longitude) {
this.longitude = longitude;
this.changedFields = changedFields.add("longitude");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder telephone3(String telephone3) {
this.telephone3 = telephone3;
this.changedFields = changedFields.add("telephone3");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder postofficebox(String postofficebox) {
this.postofficebox = postofficebox;
this.changedFields = changedFields.add("postofficebox");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder latitude(Double latitude) {
this.latitude = latitude;
this.changedFields = changedFields.add("latitude");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Customeraddress build() {
Customeraddress _x = new Customeraddress();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.customeraddress";
_x._modifiedby_value = _modifiedby_value;
_x.addresstypecode = addresstypecode;
_x._ownerid_value = _ownerid_value;
_x.customeraddressid = customeraddressid;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.line2 = line2;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.addressnumber = addressnumber;
_x.utcoffset = utcoffset;
_x.line3 = line3;
_x.shippingmethodcode = shippingmethodcode;
_x.importsequencenumber = importsequencenumber;
_x.line1 = line1;
_x.versionnumber = versionnumber;
_x._parentid_value = _parentid_value;
_x.postalcode = postalcode;
_x.freighttermscode = freighttermscode;
_x.exchangerate = exchangerate;
_x.country = country;
_x.county = county;
_x.createdon = createdon;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.stateorprovince = stateorprovince;
_x._createdby_value = _createdby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.primarycontactname = primarycontactname;
_x.telephone1 = telephone1;
_x.city = city;
_x._owninguser_value = _owninguser_value;
_x.fax = fax;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.objecttypecode = objecttypecode;
_x.telephone2 = telephone2;
_x.upszone = upszone;
_x.composite = composite;
_x.longitude = longitude;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.telephone3 = telephone3;
_x.modifiedon = modifiedon;
_x.postofficebox = postofficebox;
_x.overriddencreatedon = overriddencreatedon;
_x.latitude = latitude;
_x.name = name;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && customeraddressid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(customeraddressid.toString()));
}
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Customeraddress with_modifiedby_value(String _modifiedby_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="addresstypecode")
@JsonIgnore
public Optional getAddresstypecode() {
return Optional.ofNullable(addresstypecode);
}
public Customeraddress withAddresstypecode(Integer addresstypecode) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.addresstypecode = addresstypecode;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Customeraddress with_ownerid_value(String _ownerid_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="customeraddressid")
@JsonIgnore
public Optional getCustomeraddressid() {
return Optional.ofNullable(customeraddressid);
}
public Customeraddress withCustomeraddressid(String customeraddressid) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("customeraddressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.customeraddressid = customeraddressid;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Customeraddress with_createdonbehalfby_value(String _createdonbehalfby_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="line2")
@JsonIgnore
public Optional getLine2() {
return Optional.ofNullable(line2);
}
public Customeraddress withLine2(String line2) {
Checks.checkIsAscii(line2);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.line2 = line2;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Customeraddress withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="addressnumber")
@JsonIgnore
public Optional getAddressnumber() {
return Optional.ofNullable(addressnumber);
}
public Customeraddress withAddressnumber(Integer addressnumber) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("addressnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.addressnumber = addressnumber;
return _x;
}
@Property(name="utcoffset")
@JsonIgnore
public Optional getUtcoffset() {
return Optional.ofNullable(utcoffset);
}
public Customeraddress withUtcoffset(Integer utcoffset) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.utcoffset = utcoffset;
return _x;
}
@Property(name="line3")
@JsonIgnore
public Optional getLine3() {
return Optional.ofNullable(line3);
}
public Customeraddress withLine3(String line3) {
Checks.checkIsAscii(line3);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.line3 = line3;
return _x;
}
@Property(name="shippingmethodcode")
@JsonIgnore
public Optional getShippingmethodcode() {
return Optional.ofNullable(shippingmethodcode);
}
public Customeraddress withShippingmethodcode(Integer shippingmethodcode) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.shippingmethodcode = shippingmethodcode;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Customeraddress withImportsequencenumber(Integer importsequencenumber) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="line1")
@JsonIgnore
public Optional getLine1() {
return Optional.ofNullable(line1);
}
public Customeraddress withLine1(String line1) {
Checks.checkIsAscii(line1);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.line1 = line1;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Customeraddress withVersionnumber(Long versionnumber) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="_parentid_value")
@JsonIgnore
public Optional get_parentid_value() {
return Optional.ofNullable(_parentid_value);
}
public Customeraddress with_parentid_value(String _parentid_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_parentid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._parentid_value = _parentid_value;
return _x;
}
@Property(name="postalcode")
@JsonIgnore
public Optional getPostalcode() {
return Optional.ofNullable(postalcode);
}
public Customeraddress withPostalcode(String postalcode) {
Checks.checkIsAscii(postalcode);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.postalcode = postalcode;
return _x;
}
@Property(name="freighttermscode")
@JsonIgnore
public Optional getFreighttermscode() {
return Optional.ofNullable(freighttermscode);
}
public Customeraddress withFreighttermscode(Integer freighttermscode) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.freighttermscode = freighttermscode;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Customeraddress withExchangerate(BigDecimal exchangerate) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="country")
@JsonIgnore
public Optional getCountry() {
return Optional.ofNullable(country);
}
public Customeraddress withCountry(String country) {
Checks.checkIsAscii(country);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.country = country;
return _x;
}
@Property(name="county")
@JsonIgnore
public Optional getCounty() {
return Optional.ofNullable(county);
}
public Customeraddress withCounty(String county) {
Checks.checkIsAscii(county);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.county = county;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Customeraddress withCreatedon(OffsetDateTime createdon) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.createdon = createdon;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Customeraddress with_owningbusinessunit_value(String _owningbusinessunit_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="stateorprovince")
@JsonIgnore
public Optional getStateorprovince() {
return Optional.ofNullable(stateorprovince);
}
public Customeraddress withStateorprovince(String stateorprovince) {
Checks.checkIsAscii(stateorprovince);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.stateorprovince = stateorprovince;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Customeraddress with_createdby_value(String _createdby_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Customeraddress with_transactioncurrencyid_value(String _transactioncurrencyid_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="primarycontactname")
@JsonIgnore
public Optional getPrimarycontactname() {
return Optional.ofNullable(primarycontactname);
}
public Customeraddress withPrimarycontactname(String primarycontactname) {
Checks.checkIsAscii(primarycontactname);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.primarycontactname = primarycontactname;
return _x;
}
@Property(name="telephone1")
@JsonIgnore
public Optional getTelephone1() {
return Optional.ofNullable(telephone1);
}
public Customeraddress withTelephone1(String telephone1) {
Checks.checkIsAscii(telephone1);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.telephone1 = telephone1;
return _x;
}
@Property(name="city")
@JsonIgnore
public Optional getCity() {
return Optional.ofNullable(city);
}
public Customeraddress withCity(String city) {
Checks.checkIsAscii(city);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.city = city;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Customeraddress with_owninguser_value(String _owninguser_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="fax")
@JsonIgnore
public Optional getFax() {
return Optional.ofNullable(fax);
}
public Customeraddress withFax(String fax) {
Checks.checkIsAscii(fax);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.fax = fax;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Customeraddress with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="objecttypecode")
@JsonIgnore
public Optional getObjecttypecode() {
return Optional.ofNullable(objecttypecode);
}
public Customeraddress withObjecttypecode(String objecttypecode) {
Checks.checkIsAscii(objecttypecode);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("objecttypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.objecttypecode = objecttypecode;
return _x;
}
@Property(name="telephone2")
@JsonIgnore
public Optional getTelephone2() {
return Optional.ofNullable(telephone2);
}
public Customeraddress withTelephone2(String telephone2) {
Checks.checkIsAscii(telephone2);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.telephone2 = telephone2;
return _x;
}
@Property(name="upszone")
@JsonIgnore
public Optional getUpszone() {
return Optional.ofNullable(upszone);
}
public Customeraddress withUpszone(String upszone) {
Checks.checkIsAscii(upszone);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.upszone = upszone;
return _x;
}
@Property(name="composite")
@JsonIgnore
public Optional getComposite() {
return Optional.ofNullable(composite);
}
public Customeraddress withComposite(String composite) {
Checks.checkIsAscii(composite);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.composite = composite;
return _x;
}
@Property(name="longitude")
@JsonIgnore
public Optional getLongitude() {
return Optional.ofNullable(longitude);
}
public Customeraddress withLongitude(Double longitude) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.longitude = longitude;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Customeraddress withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="telephone3")
@JsonIgnore
public Optional getTelephone3() {
return Optional.ofNullable(telephone3);
}
public Customeraddress withTelephone3(String telephone3) {
Checks.checkIsAscii(telephone3);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.telephone3 = telephone3;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Customeraddress withModifiedon(OffsetDateTime modifiedon) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="postofficebox")
@JsonIgnore
public Optional getPostofficebox() {
return Optional.ofNullable(postofficebox);
}
public Customeraddress withPostofficebox(String postofficebox) {
Checks.checkIsAscii(postofficebox);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.postofficebox = postofficebox;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Customeraddress withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="latitude")
@JsonIgnore
public Optional getLatitude() {
return Optional.ofNullable(latitude);
}
public Customeraddress withLatitude(Double latitude) {
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.latitude = latitude;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Customeraddress withName(String name) {
Checks.checkIsAscii(name);
Customeraddress _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.customeraddress");
_x.name = name;
return _x;
}
@NavigationProperty(name="CustomerAddress_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getCustomerAddress_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("CustomerAddress_BulkDeleteFailures"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"));
}
@NavigationProperty(name="CustomerAddress_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getCustomerAddress_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("CustomerAddress_ProcessSessions"));
}
@NavigationProperty(name="parentid_account")
@JsonIgnore
public AccountRequest getParentid_account() {
return new AccountRequest(contextPath.addSegment("parentid_account"));
}
@NavigationProperty(name="parentid_contact")
@JsonIgnore
public ContactRequest getParentid_contact() {
return new ContactRequest(contextPath.addSegment("parentid_contact"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="CustomerAddress_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getCustomerAddress_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("CustomerAddress_SyncErrors"));
}
@NavigationProperty(name="customeraddress_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getCustomeraddress_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("customeraddress_principalobjectattributeaccess"));
}
@NavigationProperty(name="CustomerAddress_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getCustomerAddress_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("CustomerAddress_AsyncOperations"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Customeraddress patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Customeraddress _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Customeraddress put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Customeraddress _x = _copy();
_x.changedFields = null;
return _x;
}
private Customeraddress _copy() {
Customeraddress _x = new Customeraddress();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x._modifiedby_value = _modifiedby_value;
_x.addresstypecode = addresstypecode;
_x._ownerid_value = _ownerid_value;
_x.customeraddressid = customeraddressid;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.line2 = line2;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.addressnumber = addressnumber;
_x.utcoffset = utcoffset;
_x.line3 = line3;
_x.shippingmethodcode = shippingmethodcode;
_x.importsequencenumber = importsequencenumber;
_x.line1 = line1;
_x.versionnumber = versionnumber;
_x._parentid_value = _parentid_value;
_x.postalcode = postalcode;
_x.freighttermscode = freighttermscode;
_x.exchangerate = exchangerate;
_x.country = country;
_x.county = county;
_x.createdon = createdon;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.stateorprovince = stateorprovince;
_x._createdby_value = _createdby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.primarycontactname = primarycontactname;
_x.telephone1 = telephone1;
_x.city = city;
_x._owninguser_value = _owninguser_value;
_x.fax = fax;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.objecttypecode = objecttypecode;
_x.telephone2 = telephone2;
_x.upszone = upszone;
_x.composite = composite;
_x.longitude = longitude;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.telephone3 = telephone3;
_x.modifiedon = modifiedon;
_x.postofficebox = postofficebox;
_x.overriddencreatedon = overriddencreatedon;
_x.latitude = latitude;
_x.name = name;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Customeraddress[");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("addresstypecode=");
b.append(this.addresstypecode);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("customeraddressid=");
b.append(this.customeraddressid);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("line2=");
b.append(this.line2);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("addressnumber=");
b.append(this.addressnumber);
b.append(", ");
b.append("utcoffset=");
b.append(this.utcoffset);
b.append(", ");
b.append("line3=");
b.append(this.line3);
b.append(", ");
b.append("shippingmethodcode=");
b.append(this.shippingmethodcode);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("line1=");
b.append(this.line1);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("_parentid_value=");
b.append(this._parentid_value);
b.append(", ");
b.append("postalcode=");
b.append(this.postalcode);
b.append(", ");
b.append("freighttermscode=");
b.append(this.freighttermscode);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("country=");
b.append(this.country);
b.append(", ");
b.append("county=");
b.append(this.county);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("stateorprovince=");
b.append(this.stateorprovince);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("primarycontactname=");
b.append(this.primarycontactname);
b.append(", ");
b.append("telephone1=");
b.append(this.telephone1);
b.append(", ");
b.append("city=");
b.append(this.city);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("fax=");
b.append(this.fax);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("objecttypecode=");
b.append(this.objecttypecode);
b.append(", ");
b.append("telephone2=");
b.append(this.telephone2);
b.append(", ");
b.append("upszone=");
b.append(this.upszone);
b.append(", ");
b.append("composite=");
b.append(this.composite);
b.append(", ");
b.append("longitude=");
b.append(this.longitude);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("telephone3=");
b.append(this.telephone3);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("postofficebox=");
b.append(this.postofficebox);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("latitude=");
b.append(this.latitude);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy