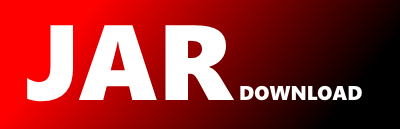
microsoft.dynamics.crm.entity.Email Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import microsoft.dynamics.crm.complex.SendEmailResponse;
import microsoft.dynamics.crm.entity.collection.request.ActioncardCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitymimeattachmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlakpiinstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.ActivitypointerRequest;
import microsoft.dynamics.crm.entity.request.AsyncoperationRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.EmailRequest;
import microsoft.dynamics.crm.entity.request.KnowledgearticleRequest;
import microsoft.dynamics.crm.entity.request.KnowledgebaserecordRequest;
import microsoft.dynamics.crm.entity.request.MailboxRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ProcessstageRequest;
import microsoft.dynamics.crm.entity.request.QueueRequest;
import microsoft.dynamics.crm.entity.request.SlaRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TemplateRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"reminderactioncardid",
"trackingtoken",
"overriddencreatedon",
"readreceiptrequested",
"messageid",
"compressed",
"emailremindertext",
"emailtrackingid",
"emailreminderexpirytime",
"attachmentopencount",
"isunsafe",
"subcategory",
"_parentactivityid_value",
"postponeemailprocessinguntil",
"category",
"replycount",
"directioncode",
"correlationmethod",
"_sendersaccount_value",
"emailreminderstatus",
"linksclickedcount",
"submittedby",
"deliveryreceiptrequested",
"notifications",
"conversationtrackingid",
"deliveryattempts",
"opencount",
"torecipients",
"emailremindertype",
"delayedemailsendtime",
"mimetype",
"followemailuserpreference",
"_templateid_value",
"_emailsender_value",
"inreplyto",
"attachmentcount",
"lastopenedtime",
"importsequencenumber",
"isemailreminderset",
"isemailfollowed",
"conversationindex",
"baseconversationindexhash",
"sender"})
@JsonInclude(Include.NON_NULL)
public class Email extends Activitypointer implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.email";
}
@JsonProperty("reminderactioncardid")
protected String reminderactioncardid;
@JsonProperty("trackingtoken")
protected String trackingtoken;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("readreceiptrequested")
protected Boolean readreceiptrequested;
@JsonProperty("messageid")
protected String messageid;
@JsonProperty("compressed")
protected Boolean compressed;
@JsonProperty("emailremindertext")
protected String emailremindertext;
@JsonProperty("emailtrackingid")
protected String emailtrackingid;
@JsonProperty("emailreminderexpirytime")
protected OffsetDateTime emailreminderexpirytime;
@JsonProperty("attachmentopencount")
protected Integer attachmentopencount;
@JsonProperty("isunsafe")
protected Integer isunsafe;
@JsonProperty("subcategory")
protected String subcategory;
@JsonProperty("_parentactivityid_value")
protected String _parentactivityid_value;
@JsonProperty("postponeemailprocessinguntil")
protected OffsetDateTime postponeemailprocessinguntil;
@JsonProperty("category")
protected String category;
@JsonProperty("replycount")
protected Integer replycount;
@JsonProperty("directioncode")
protected Boolean directioncode;
@JsonProperty("correlationmethod")
protected Integer correlationmethod;
@JsonProperty("_sendersaccount_value")
protected String _sendersaccount_value;
@JsonProperty("emailreminderstatus")
protected Integer emailreminderstatus;
@JsonProperty("linksclickedcount")
protected Integer linksclickedcount;
@JsonProperty("submittedby")
protected String submittedby;
@JsonProperty("deliveryreceiptrequested")
protected Boolean deliveryreceiptrequested;
@JsonProperty("notifications")
protected Integer notifications;
@JsonProperty("conversationtrackingid")
protected String conversationtrackingid;
@JsonProperty("deliveryattempts")
protected Integer deliveryattempts;
@JsonProperty("opencount")
protected Integer opencount;
@JsonProperty("torecipients")
protected String torecipients;
@JsonProperty("emailremindertype")
protected Integer emailremindertype;
@JsonProperty("delayedemailsendtime")
protected OffsetDateTime delayedemailsendtime;
@JsonProperty("mimetype")
protected String mimetype;
@JsonProperty("followemailuserpreference")
protected Boolean followemailuserpreference;
@JsonProperty("_templateid_value")
protected String _templateid_value;
@JsonProperty("_emailsender_value")
protected String _emailsender_value;
@JsonProperty("inreplyto")
protected String inreplyto;
@JsonProperty("attachmentcount")
protected Integer attachmentcount;
@JsonProperty("lastopenedtime")
protected OffsetDateTime lastopenedtime;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("isemailreminderset")
protected Boolean isemailreminderset;
@JsonProperty("isemailfollowed")
protected Boolean isemailfollowed;
@JsonProperty("conversationindex")
protected String conversationindex;
@JsonProperty("baseconversationindexhash")
protected Integer baseconversationindexhash;
@JsonProperty("sender")
protected String sender;
protected Email() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderEmail() {
return new Builder();
}
public static final class Builder {
private OffsetDateTime lastonholdtime;
private Integer actualdurationminutes;
private String _owningteam_value;
private String exchangeitemid;
private Boolean ismapiprivate;
private OffsetDateTime createdon;
private String seriesid;
private Boolean leftvoicemail;
private OffsetDateTime deliverylastattemptedon;
private Boolean isbilled;
private Boolean isworkflowcreated;
private String _sendermailboxid_value;
private String description;
private String _regardingobjectid_value;
private Integer onholdtime;
private String _modifiedby_value;
private Integer community;
private String activityid;
private OffsetDateTime sortdate;
private Integer instancetypecode;
private Integer timezoneruleversionnumber;
private String _createdonbehalfby_value;
private String _transactioncurrencyid_value;
private Long versionnumber;
private String processid;
private OffsetDateTime scheduledend;
private Integer prioritycode;
private String _slaid_value;
private String stageid;
private OffsetDateTime actualstart;
private String _owningbusinessunit_value;
private String _owninguser_value;
private Integer utcconversiontimezonecode;
private String exchangeweblink;
private Integer scheduleddurationminutes;
private OffsetDateTime senton;
private OffsetDateTime scheduledstart;
private Integer statecode;
private String subject;
private OffsetDateTime postponeactivityprocessinguntil;
private String _modifiedonbehalfby_value;
private BigDecimal exchangerate;
private Boolean isregularactivity;
private Integer deliveryprioritycode;
private String activityadditionalparams;
private String traversedpath;
private String _createdby_value;
private String activitytypecode;
private String _ownerid_value;
private OffsetDateTime modifiedon;
private String _slainvokedid_value;
private Integer statuscode;
private OffsetDateTime actualend;
private String reminderactioncardid;
private String trackingtoken;
private OffsetDateTime overriddencreatedon;
private Boolean readreceiptrequested;
private String messageid;
private Boolean compressed;
private String emailremindertext;
private String emailtrackingid;
private OffsetDateTime emailreminderexpirytime;
private Integer attachmentopencount;
private Integer isunsafe;
private String subcategory;
private String _parentactivityid_value;
private OffsetDateTime postponeemailprocessinguntil;
private String category;
private Integer replycount;
private Boolean directioncode;
private Integer correlationmethod;
private String _sendersaccount_value;
private Integer emailreminderstatus;
private Integer linksclickedcount;
private String submittedby;
private Boolean deliveryreceiptrequested;
private Integer notifications;
private String conversationtrackingid;
private Integer deliveryattempts;
private Integer opencount;
private String torecipients;
private Integer emailremindertype;
private OffsetDateTime delayedemailsendtime;
private String mimetype;
private Boolean followemailuserpreference;
private String _templateid_value;
private String _emailsender_value;
private String inreplyto;
private Integer attachmentcount;
private OffsetDateTime lastopenedtime;
private Integer importsequencenumber;
private Boolean isemailreminderset;
private Boolean isemailfollowed;
private String conversationindex;
private Integer baseconversationindexhash;
private String sender;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder lastonholdtime(OffsetDateTime lastonholdtime) {
this.lastonholdtime = lastonholdtime;
this.changedFields = changedFields.add("lastonholdtime");
return this;
}
public Builder actualdurationminutes(Integer actualdurationminutes) {
this.actualdurationminutes = actualdurationminutes;
this.changedFields = changedFields.add("actualdurationminutes");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder exchangeitemid(String exchangeitemid) {
this.exchangeitemid = exchangeitemid;
this.changedFields = changedFields.add("exchangeitemid");
return this;
}
public Builder ismapiprivate(Boolean ismapiprivate) {
this.ismapiprivate = ismapiprivate;
this.changedFields = changedFields.add("ismapiprivate");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder seriesid(String seriesid) {
this.seriesid = seriesid;
this.changedFields = changedFields.add("seriesid");
return this;
}
public Builder leftvoicemail(Boolean leftvoicemail) {
this.leftvoicemail = leftvoicemail;
this.changedFields = changedFields.add("leftvoicemail");
return this;
}
public Builder deliverylastattemptedon(OffsetDateTime deliverylastattemptedon) {
this.deliverylastattemptedon = deliverylastattemptedon;
this.changedFields = changedFields.add("deliverylastattemptedon");
return this;
}
public Builder isbilled(Boolean isbilled) {
this.isbilled = isbilled;
this.changedFields = changedFields.add("isbilled");
return this;
}
public Builder isworkflowcreated(Boolean isworkflowcreated) {
this.isworkflowcreated = isworkflowcreated;
this.changedFields = changedFields.add("isworkflowcreated");
return this;
}
public Builder _sendermailboxid_value(String _sendermailboxid_value) {
this._sendermailboxid_value = _sendermailboxid_value;
this.changedFields = changedFields.add("_sendermailboxid_value");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder _regardingobjectid_value(String _regardingobjectid_value) {
this._regardingobjectid_value = _regardingobjectid_value;
this.changedFields = changedFields.add("_regardingobjectid_value");
return this;
}
public Builder onholdtime(Integer onholdtime) {
this.onholdtime = onholdtime;
this.changedFields = changedFields.add("onholdtime");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder community(Integer community) {
this.community = community;
this.changedFields = changedFields.add("community");
return this;
}
public Builder activityid(String activityid) {
this.activityid = activityid;
this.changedFields = changedFields.add("activityid");
return this;
}
public Builder sortdate(OffsetDateTime sortdate) {
this.sortdate = sortdate;
this.changedFields = changedFields.add("sortdate");
return this;
}
public Builder instancetypecode(Integer instancetypecode) {
this.instancetypecode = instancetypecode;
this.changedFields = changedFields.add("instancetypecode");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder _transactioncurrencyid_value(String _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder processid(String processid) {
this.processid = processid;
this.changedFields = changedFields.add("processid");
return this;
}
public Builder scheduledend(OffsetDateTime scheduledend) {
this.scheduledend = scheduledend;
this.changedFields = changedFields.add("scheduledend");
return this;
}
public Builder prioritycode(Integer prioritycode) {
this.prioritycode = prioritycode;
this.changedFields = changedFields.add("prioritycode");
return this;
}
public Builder _slaid_value(String _slaid_value) {
this._slaid_value = _slaid_value;
this.changedFields = changedFields.add("_slaid_value");
return this;
}
public Builder stageid(String stageid) {
this.stageid = stageid;
this.changedFields = changedFields.add("stageid");
return this;
}
public Builder actualstart(OffsetDateTime actualstart) {
this.actualstart = actualstart;
this.changedFields = changedFields.add("actualstart");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder exchangeweblink(String exchangeweblink) {
this.exchangeweblink = exchangeweblink;
this.changedFields = changedFields.add("exchangeweblink");
return this;
}
public Builder scheduleddurationminutes(Integer scheduleddurationminutes) {
this.scheduleddurationminutes = scheduleddurationminutes;
this.changedFields = changedFields.add("scheduleddurationminutes");
return this;
}
public Builder senton(OffsetDateTime senton) {
this.senton = senton;
this.changedFields = changedFields.add("senton");
return this;
}
public Builder scheduledstart(OffsetDateTime scheduledstart) {
this.scheduledstart = scheduledstart;
this.changedFields = changedFields.add("scheduledstart");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder subject(String subject) {
this.subject = subject;
this.changedFields = changedFields.add("subject");
return this;
}
public Builder postponeactivityprocessinguntil(OffsetDateTime postponeactivityprocessinguntil) {
this.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
this.changedFields = changedFields.add("postponeactivityprocessinguntil");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder isregularactivity(Boolean isregularactivity) {
this.isregularactivity = isregularactivity;
this.changedFields = changedFields.add("isregularactivity");
return this;
}
public Builder deliveryprioritycode(Integer deliveryprioritycode) {
this.deliveryprioritycode = deliveryprioritycode;
this.changedFields = changedFields.add("deliveryprioritycode");
return this;
}
public Builder activityadditionalparams(String activityadditionalparams) {
this.activityadditionalparams = activityadditionalparams;
this.changedFields = changedFields.add("activityadditionalparams");
return this;
}
public Builder traversedpath(String traversedpath) {
this.traversedpath = traversedpath;
this.changedFields = changedFields.add("traversedpath");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder activitytypecode(String activitytypecode) {
this.activitytypecode = activitytypecode;
this.changedFields = changedFields.add("activitytypecode");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder _slainvokedid_value(String _slainvokedid_value) {
this._slainvokedid_value = _slainvokedid_value;
this.changedFields = changedFields.add("_slainvokedid_value");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder actualend(OffsetDateTime actualend) {
this.actualend = actualend;
this.changedFields = changedFields.add("actualend");
return this;
}
public Builder reminderactioncardid(String reminderactioncardid) {
this.reminderactioncardid = reminderactioncardid;
this.changedFields = changedFields.add("reminderactioncardid");
return this;
}
public Builder trackingtoken(String trackingtoken) {
this.trackingtoken = trackingtoken;
this.changedFields = changedFields.add("trackingtoken");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder readreceiptrequested(Boolean readreceiptrequested) {
this.readreceiptrequested = readreceiptrequested;
this.changedFields = changedFields.add("readreceiptrequested");
return this;
}
public Builder messageid(String messageid) {
this.messageid = messageid;
this.changedFields = changedFields.add("messageid");
return this;
}
public Builder compressed(Boolean compressed) {
this.compressed = compressed;
this.changedFields = changedFields.add("compressed");
return this;
}
public Builder emailremindertext(String emailremindertext) {
this.emailremindertext = emailremindertext;
this.changedFields = changedFields.add("emailremindertext");
return this;
}
public Builder emailtrackingid(String emailtrackingid) {
this.emailtrackingid = emailtrackingid;
this.changedFields = changedFields.add("emailtrackingid");
return this;
}
public Builder emailreminderexpirytime(OffsetDateTime emailreminderexpirytime) {
this.emailreminderexpirytime = emailreminderexpirytime;
this.changedFields = changedFields.add("emailreminderexpirytime");
return this;
}
public Builder attachmentopencount(Integer attachmentopencount) {
this.attachmentopencount = attachmentopencount;
this.changedFields = changedFields.add("attachmentopencount");
return this;
}
public Builder isunsafe(Integer isunsafe) {
this.isunsafe = isunsafe;
this.changedFields = changedFields.add("isunsafe");
return this;
}
public Builder subcategory(String subcategory) {
this.subcategory = subcategory;
this.changedFields = changedFields.add("subcategory");
return this;
}
public Builder _parentactivityid_value(String _parentactivityid_value) {
this._parentactivityid_value = _parentactivityid_value;
this.changedFields = changedFields.add("_parentactivityid_value");
return this;
}
public Builder postponeemailprocessinguntil(OffsetDateTime postponeemailprocessinguntil) {
this.postponeemailprocessinguntil = postponeemailprocessinguntil;
this.changedFields = changedFields.add("postponeemailprocessinguntil");
return this;
}
public Builder category(String category) {
this.category = category;
this.changedFields = changedFields.add("category");
return this;
}
public Builder replycount(Integer replycount) {
this.replycount = replycount;
this.changedFields = changedFields.add("replycount");
return this;
}
public Builder directioncode(Boolean directioncode) {
this.directioncode = directioncode;
this.changedFields = changedFields.add("directioncode");
return this;
}
public Builder correlationmethod(Integer correlationmethod) {
this.correlationmethod = correlationmethod;
this.changedFields = changedFields.add("correlationmethod");
return this;
}
public Builder _sendersaccount_value(String _sendersaccount_value) {
this._sendersaccount_value = _sendersaccount_value;
this.changedFields = changedFields.add("_sendersaccount_value");
return this;
}
public Builder emailreminderstatus(Integer emailreminderstatus) {
this.emailreminderstatus = emailreminderstatus;
this.changedFields = changedFields.add("emailreminderstatus");
return this;
}
public Builder linksclickedcount(Integer linksclickedcount) {
this.linksclickedcount = linksclickedcount;
this.changedFields = changedFields.add("linksclickedcount");
return this;
}
public Builder submittedby(String submittedby) {
this.submittedby = submittedby;
this.changedFields = changedFields.add("submittedby");
return this;
}
public Builder deliveryreceiptrequested(Boolean deliveryreceiptrequested) {
this.deliveryreceiptrequested = deliveryreceiptrequested;
this.changedFields = changedFields.add("deliveryreceiptrequested");
return this;
}
public Builder notifications(Integer notifications) {
this.notifications = notifications;
this.changedFields = changedFields.add("notifications");
return this;
}
public Builder conversationtrackingid(String conversationtrackingid) {
this.conversationtrackingid = conversationtrackingid;
this.changedFields = changedFields.add("conversationtrackingid");
return this;
}
public Builder deliveryattempts(Integer deliveryattempts) {
this.deliveryattempts = deliveryattempts;
this.changedFields = changedFields.add("deliveryattempts");
return this;
}
public Builder opencount(Integer opencount) {
this.opencount = opencount;
this.changedFields = changedFields.add("opencount");
return this;
}
public Builder torecipients(String torecipients) {
this.torecipients = torecipients;
this.changedFields = changedFields.add("torecipients");
return this;
}
public Builder emailremindertype(Integer emailremindertype) {
this.emailremindertype = emailremindertype;
this.changedFields = changedFields.add("emailremindertype");
return this;
}
public Builder delayedemailsendtime(OffsetDateTime delayedemailsendtime) {
this.delayedemailsendtime = delayedemailsendtime;
this.changedFields = changedFields.add("delayedemailsendtime");
return this;
}
public Builder mimetype(String mimetype) {
this.mimetype = mimetype;
this.changedFields = changedFields.add("mimetype");
return this;
}
public Builder followemailuserpreference(Boolean followemailuserpreference) {
this.followemailuserpreference = followemailuserpreference;
this.changedFields = changedFields.add("followemailuserpreference");
return this;
}
public Builder _templateid_value(String _templateid_value) {
this._templateid_value = _templateid_value;
this.changedFields = changedFields.add("_templateid_value");
return this;
}
public Builder _emailsender_value(String _emailsender_value) {
this._emailsender_value = _emailsender_value;
this.changedFields = changedFields.add("_emailsender_value");
return this;
}
public Builder inreplyto(String inreplyto) {
this.inreplyto = inreplyto;
this.changedFields = changedFields.add("inreplyto");
return this;
}
public Builder attachmentcount(Integer attachmentcount) {
this.attachmentcount = attachmentcount;
this.changedFields = changedFields.add("attachmentcount");
return this;
}
public Builder lastopenedtime(OffsetDateTime lastopenedtime) {
this.lastopenedtime = lastopenedtime;
this.changedFields = changedFields.add("lastopenedtime");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder isemailreminderset(Boolean isemailreminderset) {
this.isemailreminderset = isemailreminderset;
this.changedFields = changedFields.add("isemailreminderset");
return this;
}
public Builder isemailfollowed(Boolean isemailfollowed) {
this.isemailfollowed = isemailfollowed;
this.changedFields = changedFields.add("isemailfollowed");
return this;
}
public Builder conversationindex(String conversationindex) {
this.conversationindex = conversationindex;
this.changedFields = changedFields.add("conversationindex");
return this;
}
public Builder baseconversationindexhash(Integer baseconversationindexhash) {
this.baseconversationindexhash = baseconversationindexhash;
this.changedFields = changedFields.add("baseconversationindexhash");
return this;
}
public Builder sender(String sender) {
this.sender = sender;
this.changedFields = changedFields.add("sender");
return this;
}
public Email build() {
Email _x = new Email();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.email";
_x.lastonholdtime = lastonholdtime;
_x.actualdurationminutes = actualdurationminutes;
_x._owningteam_value = _owningteam_value;
_x.exchangeitemid = exchangeitemid;
_x.ismapiprivate = ismapiprivate;
_x.createdon = createdon;
_x.seriesid = seriesid;
_x.leftvoicemail = leftvoicemail;
_x.deliverylastattemptedon = deliverylastattemptedon;
_x.isbilled = isbilled;
_x.isworkflowcreated = isworkflowcreated;
_x._sendermailboxid_value = _sendermailboxid_value;
_x.description = description;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.onholdtime = onholdtime;
_x._modifiedby_value = _modifiedby_value;
_x.community = community;
_x.activityid = activityid;
_x.sortdate = sortdate;
_x.instancetypecode = instancetypecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.versionnumber = versionnumber;
_x.processid = processid;
_x.scheduledend = scheduledend;
_x.prioritycode = prioritycode;
_x._slaid_value = _slaid_value;
_x.stageid = stageid;
_x.actualstart = actualstart;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._owninguser_value = _owninguser_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.exchangeweblink = exchangeweblink;
_x.scheduleddurationminutes = scheduleddurationminutes;
_x.senton = senton;
_x.scheduledstart = scheduledstart;
_x.statecode = statecode;
_x.subject = subject;
_x.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.isregularactivity = isregularactivity;
_x.deliveryprioritycode = deliveryprioritycode;
_x.activityadditionalparams = activityadditionalparams;
_x.traversedpath = traversedpath;
_x._createdby_value = _createdby_value;
_x.activitytypecode = activitytypecode;
_x._ownerid_value = _ownerid_value;
_x.modifiedon = modifiedon;
_x._slainvokedid_value = _slainvokedid_value;
_x.statuscode = statuscode;
_x.actualend = actualend;
_x.reminderactioncardid = reminderactioncardid;
_x.trackingtoken = trackingtoken;
_x.overriddencreatedon = overriddencreatedon;
_x.readreceiptrequested = readreceiptrequested;
_x.messageid = messageid;
_x.compressed = compressed;
_x.emailremindertext = emailremindertext;
_x.emailtrackingid = emailtrackingid;
_x.emailreminderexpirytime = emailreminderexpirytime;
_x.attachmentopencount = attachmentopencount;
_x.isunsafe = isunsafe;
_x.subcategory = subcategory;
_x._parentactivityid_value = _parentactivityid_value;
_x.postponeemailprocessinguntil = postponeemailprocessinguntil;
_x.category = category;
_x.replycount = replycount;
_x.directioncode = directioncode;
_x.correlationmethod = correlationmethod;
_x._sendersaccount_value = _sendersaccount_value;
_x.emailreminderstatus = emailreminderstatus;
_x.linksclickedcount = linksclickedcount;
_x.submittedby = submittedby;
_x.deliveryreceiptrequested = deliveryreceiptrequested;
_x.notifications = notifications;
_x.conversationtrackingid = conversationtrackingid;
_x.deliveryattempts = deliveryattempts;
_x.opencount = opencount;
_x.torecipients = torecipients;
_x.emailremindertype = emailremindertype;
_x.delayedemailsendtime = delayedemailsendtime;
_x.mimetype = mimetype;
_x.followemailuserpreference = followemailuserpreference;
_x._templateid_value = _templateid_value;
_x._emailsender_value = _emailsender_value;
_x.inreplyto = inreplyto;
_x.attachmentcount = attachmentcount;
_x.lastopenedtime = lastopenedtime;
_x.importsequencenumber = importsequencenumber;
_x.isemailreminderset = isemailreminderset;
_x.isemailfollowed = isemailfollowed;
_x.conversationindex = conversationindex;
_x.baseconversationindexhash = baseconversationindexhash;
_x.sender = sender;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && activityid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(activityid.toString()));
}
}
@Property(name="reminderactioncardid")
@JsonIgnore
public Optional getReminderactioncardid() {
return Optional.ofNullable(reminderactioncardid);
}
public Email withReminderactioncardid(String reminderactioncardid) {
Email _x = _copy();
_x.changedFields = changedFields.add("reminderactioncardid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.reminderactioncardid = reminderactioncardid;
return _x;
}
@Property(name="trackingtoken")
@JsonIgnore
public Optional getTrackingtoken() {
return Optional.ofNullable(trackingtoken);
}
public Email withTrackingtoken(String trackingtoken) {
Checks.checkIsAscii(trackingtoken);
Email _x = _copy();
_x.changedFields = changedFields.add("trackingtoken");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.trackingtoken = trackingtoken;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Email withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Email _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="readreceiptrequested")
@JsonIgnore
public Optional getReadreceiptrequested() {
return Optional.ofNullable(readreceiptrequested);
}
public Email withReadreceiptrequested(Boolean readreceiptrequested) {
Email _x = _copy();
_x.changedFields = changedFields.add("readreceiptrequested");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.readreceiptrequested = readreceiptrequested;
return _x;
}
@Property(name="messageid")
@JsonIgnore
public Optional getMessageid() {
return Optional.ofNullable(messageid);
}
public Email withMessageid(String messageid) {
Checks.checkIsAscii(messageid);
Email _x = _copy();
_x.changedFields = changedFields.add("messageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.messageid = messageid;
return _x;
}
@Property(name="compressed")
@JsonIgnore
public Optional getCompressed() {
return Optional.ofNullable(compressed);
}
public Email withCompressed(Boolean compressed) {
Email _x = _copy();
_x.changedFields = changedFields.add("compressed");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.compressed = compressed;
return _x;
}
@Property(name="emailremindertext")
@JsonIgnore
public Optional getEmailremindertext() {
return Optional.ofNullable(emailremindertext);
}
public Email withEmailremindertext(String emailremindertext) {
Checks.checkIsAscii(emailremindertext);
Email _x = _copy();
_x.changedFields = changedFields.add("emailremindertext");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.emailremindertext = emailremindertext;
return _x;
}
@Property(name="emailtrackingid")
@JsonIgnore
public Optional getEmailtrackingid() {
return Optional.ofNullable(emailtrackingid);
}
public Email withEmailtrackingid(String emailtrackingid) {
Email _x = _copy();
_x.changedFields = changedFields.add("emailtrackingid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.emailtrackingid = emailtrackingid;
return _x;
}
@Property(name="emailreminderexpirytime")
@JsonIgnore
public Optional getEmailreminderexpirytime() {
return Optional.ofNullable(emailreminderexpirytime);
}
public Email withEmailreminderexpirytime(OffsetDateTime emailreminderexpirytime) {
Email _x = _copy();
_x.changedFields = changedFields.add("emailreminderexpirytime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.emailreminderexpirytime = emailreminderexpirytime;
return _x;
}
@Property(name="attachmentopencount")
@JsonIgnore
public Optional getAttachmentopencount() {
return Optional.ofNullable(attachmentopencount);
}
public Email withAttachmentopencount(Integer attachmentopencount) {
Email _x = _copy();
_x.changedFields = changedFields.add("attachmentopencount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.attachmentopencount = attachmentopencount;
return _x;
}
@Property(name="isunsafe")
@JsonIgnore
public Optional getIsunsafe() {
return Optional.ofNullable(isunsafe);
}
public Email withIsunsafe(Integer isunsafe) {
Email _x = _copy();
_x.changedFields = changedFields.add("isunsafe");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.isunsafe = isunsafe;
return _x;
}
@Property(name="subcategory")
@JsonIgnore
public Optional getSubcategory() {
return Optional.ofNullable(subcategory);
}
public Email withSubcategory(String subcategory) {
Checks.checkIsAscii(subcategory);
Email _x = _copy();
_x.changedFields = changedFields.add("subcategory");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.subcategory = subcategory;
return _x;
}
@Property(name="_parentactivityid_value")
@JsonIgnore
public Optional get_parentactivityid_value() {
return Optional.ofNullable(_parentactivityid_value);
}
public Email with_parentactivityid_value(String _parentactivityid_value) {
Email _x = _copy();
_x.changedFields = changedFields.add("_parentactivityid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x._parentactivityid_value = _parentactivityid_value;
return _x;
}
@Property(name="postponeemailprocessinguntil")
@JsonIgnore
public Optional getPostponeemailprocessinguntil() {
return Optional.ofNullable(postponeemailprocessinguntil);
}
public Email withPostponeemailprocessinguntil(OffsetDateTime postponeemailprocessinguntil) {
Email _x = _copy();
_x.changedFields = changedFields.add("postponeemailprocessinguntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.postponeemailprocessinguntil = postponeemailprocessinguntil;
return _x;
}
@Property(name="category")
@JsonIgnore
public Optional getCategory() {
return Optional.ofNullable(category);
}
public Email withCategory(String category) {
Checks.checkIsAscii(category);
Email _x = _copy();
_x.changedFields = changedFields.add("category");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.category = category;
return _x;
}
@Property(name="replycount")
@JsonIgnore
public Optional getReplycount() {
return Optional.ofNullable(replycount);
}
public Email withReplycount(Integer replycount) {
Email _x = _copy();
_x.changedFields = changedFields.add("replycount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.replycount = replycount;
return _x;
}
@Property(name="directioncode")
@JsonIgnore
public Optional getDirectioncode() {
return Optional.ofNullable(directioncode);
}
public Email withDirectioncode(Boolean directioncode) {
Email _x = _copy();
_x.changedFields = changedFields.add("directioncode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.directioncode = directioncode;
return _x;
}
@Property(name="correlationmethod")
@JsonIgnore
public Optional getCorrelationmethod() {
return Optional.ofNullable(correlationmethod);
}
public Email withCorrelationmethod(Integer correlationmethod) {
Email _x = _copy();
_x.changedFields = changedFields.add("correlationmethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.correlationmethod = correlationmethod;
return _x;
}
@Property(name="_sendersaccount_value")
@JsonIgnore
public Optional get_sendersaccount_value() {
return Optional.ofNullable(_sendersaccount_value);
}
public Email with_sendersaccount_value(String _sendersaccount_value) {
Email _x = _copy();
_x.changedFields = changedFields.add("_sendersaccount_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x._sendersaccount_value = _sendersaccount_value;
return _x;
}
@Property(name="emailreminderstatus")
@JsonIgnore
public Optional getEmailreminderstatus() {
return Optional.ofNullable(emailreminderstatus);
}
public Email withEmailreminderstatus(Integer emailreminderstatus) {
Email _x = _copy();
_x.changedFields = changedFields.add("emailreminderstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.emailreminderstatus = emailreminderstatus;
return _x;
}
@Property(name="linksclickedcount")
@JsonIgnore
public Optional getLinksclickedcount() {
return Optional.ofNullable(linksclickedcount);
}
public Email withLinksclickedcount(Integer linksclickedcount) {
Email _x = _copy();
_x.changedFields = changedFields.add("linksclickedcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.linksclickedcount = linksclickedcount;
return _x;
}
@Property(name="submittedby")
@JsonIgnore
public Optional getSubmittedby() {
return Optional.ofNullable(submittedby);
}
public Email withSubmittedby(String submittedby) {
Checks.checkIsAscii(submittedby);
Email _x = _copy();
_x.changedFields = changedFields.add("submittedby");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.submittedby = submittedby;
return _x;
}
@Property(name="deliveryreceiptrequested")
@JsonIgnore
public Optional getDeliveryreceiptrequested() {
return Optional.ofNullable(deliveryreceiptrequested);
}
public Email withDeliveryreceiptrequested(Boolean deliveryreceiptrequested) {
Email _x = _copy();
_x.changedFields = changedFields.add("deliveryreceiptrequested");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.deliveryreceiptrequested = deliveryreceiptrequested;
return _x;
}
@Property(name="notifications")
@JsonIgnore
public Optional getNotifications() {
return Optional.ofNullable(notifications);
}
public Email withNotifications(Integer notifications) {
Email _x = _copy();
_x.changedFields = changedFields.add("notifications");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.notifications = notifications;
return _x;
}
@Property(name="conversationtrackingid")
@JsonIgnore
public Optional getConversationtrackingid() {
return Optional.ofNullable(conversationtrackingid);
}
public Email withConversationtrackingid(String conversationtrackingid) {
Email _x = _copy();
_x.changedFields = changedFields.add("conversationtrackingid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.conversationtrackingid = conversationtrackingid;
return _x;
}
@Property(name="deliveryattempts")
@JsonIgnore
public Optional getDeliveryattempts() {
return Optional.ofNullable(deliveryattempts);
}
public Email withDeliveryattempts(Integer deliveryattempts) {
Email _x = _copy();
_x.changedFields = changedFields.add("deliveryattempts");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.deliveryattempts = deliveryattempts;
return _x;
}
@Property(name="opencount")
@JsonIgnore
public Optional getOpencount() {
return Optional.ofNullable(opencount);
}
public Email withOpencount(Integer opencount) {
Email _x = _copy();
_x.changedFields = changedFields.add("opencount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.opencount = opencount;
return _x;
}
@Property(name="torecipients")
@JsonIgnore
public Optional getTorecipients() {
return Optional.ofNullable(torecipients);
}
public Email withTorecipients(String torecipients) {
Checks.checkIsAscii(torecipients);
Email _x = _copy();
_x.changedFields = changedFields.add("torecipients");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.torecipients = torecipients;
return _x;
}
@Property(name="emailremindertype")
@JsonIgnore
public Optional getEmailremindertype() {
return Optional.ofNullable(emailremindertype);
}
public Email withEmailremindertype(Integer emailremindertype) {
Email _x = _copy();
_x.changedFields = changedFields.add("emailremindertype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.emailremindertype = emailremindertype;
return _x;
}
@Property(name="delayedemailsendtime")
@JsonIgnore
public Optional getDelayedemailsendtime() {
return Optional.ofNullable(delayedemailsendtime);
}
public Email withDelayedemailsendtime(OffsetDateTime delayedemailsendtime) {
Email _x = _copy();
_x.changedFields = changedFields.add("delayedemailsendtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.delayedemailsendtime = delayedemailsendtime;
return _x;
}
@Property(name="mimetype")
@JsonIgnore
public Optional getMimetype() {
return Optional.ofNullable(mimetype);
}
public Email withMimetype(String mimetype) {
Checks.checkIsAscii(mimetype);
Email _x = _copy();
_x.changedFields = changedFields.add("mimetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.mimetype = mimetype;
return _x;
}
@Property(name="followemailuserpreference")
@JsonIgnore
public Optional getFollowemailuserpreference() {
return Optional.ofNullable(followemailuserpreference);
}
public Email withFollowemailuserpreference(Boolean followemailuserpreference) {
Email _x = _copy();
_x.changedFields = changedFields.add("followemailuserpreference");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.followemailuserpreference = followemailuserpreference;
return _x;
}
@Property(name="_templateid_value")
@JsonIgnore
public Optional get_templateid_value() {
return Optional.ofNullable(_templateid_value);
}
public Email with_templateid_value(String _templateid_value) {
Email _x = _copy();
_x.changedFields = changedFields.add("_templateid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x._templateid_value = _templateid_value;
return _x;
}
@Property(name="_emailsender_value")
@JsonIgnore
public Optional get_emailsender_value() {
return Optional.ofNullable(_emailsender_value);
}
public Email with_emailsender_value(String _emailsender_value) {
Email _x = _copy();
_x.changedFields = changedFields.add("_emailsender_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x._emailsender_value = _emailsender_value;
return _x;
}
@Property(name="inreplyto")
@JsonIgnore
public Optional getInreplyto() {
return Optional.ofNullable(inreplyto);
}
public Email withInreplyto(String inreplyto) {
Checks.checkIsAscii(inreplyto);
Email _x = _copy();
_x.changedFields = changedFields.add("inreplyto");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.inreplyto = inreplyto;
return _x;
}
@Property(name="attachmentcount")
@JsonIgnore
public Optional getAttachmentcount() {
return Optional.ofNullable(attachmentcount);
}
public Email withAttachmentcount(Integer attachmentcount) {
Email _x = _copy();
_x.changedFields = changedFields.add("attachmentcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.attachmentcount = attachmentcount;
return _x;
}
@Property(name="lastopenedtime")
@JsonIgnore
public Optional getLastopenedtime() {
return Optional.ofNullable(lastopenedtime);
}
public Email withLastopenedtime(OffsetDateTime lastopenedtime) {
Email _x = _copy();
_x.changedFields = changedFields.add("lastopenedtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.lastopenedtime = lastopenedtime;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Email withImportsequencenumber(Integer importsequencenumber) {
Email _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="isemailreminderset")
@JsonIgnore
public Optional getIsemailreminderset() {
return Optional.ofNullable(isemailreminderset);
}
public Email withIsemailreminderset(Boolean isemailreminderset) {
Email _x = _copy();
_x.changedFields = changedFields.add("isemailreminderset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.isemailreminderset = isemailreminderset;
return _x;
}
@Property(name="isemailfollowed")
@JsonIgnore
public Optional getIsemailfollowed() {
return Optional.ofNullable(isemailfollowed);
}
public Email withIsemailfollowed(Boolean isemailfollowed) {
Email _x = _copy();
_x.changedFields = changedFields.add("isemailfollowed");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.isemailfollowed = isemailfollowed;
return _x;
}
@Property(name="conversationindex")
@JsonIgnore
public Optional getConversationindex() {
return Optional.ofNullable(conversationindex);
}
public Email withConversationindex(String conversationindex) {
Checks.checkIsAscii(conversationindex);
Email _x = _copy();
_x.changedFields = changedFields.add("conversationindex");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.conversationindex = conversationindex;
return _x;
}
@Property(name="baseconversationindexhash")
@JsonIgnore
public Optional getBaseconversationindexhash() {
return Optional.ofNullable(baseconversationindexhash);
}
public Email withBaseconversationindexhash(Integer baseconversationindexhash) {
Email _x = _copy();
_x.changedFields = changedFields.add("baseconversationindexhash");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.baseconversationindexhash = baseconversationindexhash;
return _x;
}
@Property(name="sender")
@JsonIgnore
public Optional getSender() {
return Optional.ofNullable(sender);
}
public Email withSender(String sender) {
Checks.checkIsAscii(sender);
Email _x = _copy();
_x.changedFields = changedFields.add("sender");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.email");
_x.sender = sender;
return _x;
}
@NavigationProperty(name="regardingobjectid_knowledgebaserecord_email")
@JsonIgnore
public KnowledgebaserecordRequest getRegardingobjectid_knowledgebaserecord_email() {
return new KnowledgebaserecordRequest(contextPath.addSegment("regardingobjectid_knowledgebaserecord_email"));
}
@NavigationProperty(name="Email_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getEmail_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Email_SyncErrors"));
}
@NavigationProperty(name="transactioncurrencyid_email")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid_email() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid_email"));
}
@NavigationProperty(name="regardingobjectid_asyncoperation")
@JsonIgnore
public AsyncoperationRequest getRegardingobjectid_asyncoperation() {
return new AsyncoperationRequest(contextPath.addSegment("regardingobjectid_asyncoperation"));
}
@NavigationProperty(name="sendersaccount")
@JsonIgnore
public AccountRequest getSendersaccount() {
return new AccountRequest(contextPath.addSegment("sendersaccount"));
}
@NavigationProperty(name="emailsender_account")
@JsonIgnore
public AccountRequest getEmailsender_account() {
return new AccountRequest(contextPath.addSegment("emailsender_account"));
}
@NavigationProperty(name="activityid_activitypointer")
@JsonIgnore
public ActivitypointerRequest getActivityid_activitypointer() {
return new ActivitypointerRequest(contextPath.addSegment("activityid_activitypointer"));
}
@NavigationProperty(name="sla_email_sla")
@JsonIgnore
public SlaRequest getSla_email_sla() {
return new SlaRequest(contextPath.addSegment("sla_email_sla"));
}
@NavigationProperty(name="Email_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getEmail_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Email_AsyncOperations"));
}
@NavigationProperty(name="Email_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getEmail_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Email_DuplicateBaseRecord"));
}
@NavigationProperty(name="email_connections1")
@JsonIgnore
public ConnectionCollectionRequest getEmail_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("email_connections1"));
}
@NavigationProperty(name="sendermailboxid_email")
@JsonIgnore
public MailboxRequest getSendermailboxid_email() {
return new MailboxRequest(contextPath.addSegment("sendermailboxid_email"));
}
@NavigationProperty(name="email_activity_mime_attachment")
@JsonIgnore
public ActivitymimeattachmentCollectionRequest getEmail_activity_mime_attachment() {
return new ActivitymimeattachmentCollectionRequest(
contextPath.addSegment("email_activity_mime_attachment"));
}
@NavigationProperty(name="slakpiinstance_email")
@JsonIgnore
public SlakpiinstanceCollectionRequest getSlakpiinstance_email() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("slakpiinstance_email"));
}
@NavigationProperty(name="email_connections2")
@JsonIgnore
public ConnectionCollectionRequest getEmail_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("email_connections2"));
}
@NavigationProperty(name="owningbusinessunit_email")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit_email() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit_email"));
}
@NavigationProperty(name="emailsender_contact")
@JsonIgnore
public ContactRequest getEmailsender_contact() {
return new ContactRequest(contextPath.addSegment("emailsender_contact"));
}
@NavigationProperty(name="owninguser_email")
@JsonIgnore
public SystemuserRequest getOwninguser_email() {
return new SystemuserRequest(contextPath.addSegment("owninguser_email"));
}
@NavigationProperty(name="modifiedby_email")
@JsonIgnore
public SystemuserRequest getModifiedby_email() {
return new SystemuserRequest(contextPath.addSegment("modifiedby_email"));
}
@NavigationProperty(name="owningteam_email")
@JsonIgnore
public TeamRequest getOwningteam_email() {
return new TeamRequest(contextPath.addSegment("owningteam_email"));
}
@NavigationProperty(name="emailsender_queue")
@JsonIgnore
public QueueRequest getEmailsender_queue() {
return new QueueRequest(contextPath.addSegment("emailsender_queue"));
}
@NavigationProperty(name="slainvokedid_email_sla")
@JsonIgnore
public SlaRequest getSlainvokedid_email_sla() {
return new SlaRequest(contextPath.addSegment("slainvokedid_email_sla"));
}
@NavigationProperty(name="Email_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getEmail_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Email_Annotation"));
}
@NavigationProperty(name="Email_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getEmail_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("Email_BulkDeleteFailures"));
}
@NavigationProperty(name="Email_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getEmail_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Email_ProcessSessions"));
}
@NavigationProperty(name="createdonbehalfby_email")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby_email() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby_email"));
}
@NavigationProperty(name="email_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getEmail_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("email_activity_parties"));
}
@NavigationProperty(name="regardingobjectid_knowledgearticle_email")
@JsonIgnore
public KnowledgearticleRequest getRegardingobjectid_knowledgearticle_email() {
return new KnowledgearticleRequest(contextPath.addSegment("regardingobjectid_knowledgearticle_email"));
}
@NavigationProperty(name="email_actioncard")
@JsonIgnore
public ActioncardCollectionRequest getEmail_actioncard() {
return new ActioncardCollectionRequest(
contextPath.addSegment("email_actioncard"));
}
@NavigationProperty(name="Email_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getEmail_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Email_DuplicateMatchingRecord"));
}
@NavigationProperty(name="email_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getEmail_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("email_principalobjectattributeaccess"));
}
@NavigationProperty(name="modifiedonbehalfby_email")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby_email() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby_email"));
}
@NavigationProperty(name="regardingobjectid_account_email")
@JsonIgnore
public AccountRequest getRegardingobjectid_account_email() {
return new AccountRequest(contextPath.addSegment("regardingobjectid_account_email"));
}
@NavigationProperty(name="createdby_email")
@JsonIgnore
public SystemuserRequest getCreatedby_email() {
return new SystemuserRequest(contextPath.addSegment("createdby_email"));
}
@NavigationProperty(name="emailsender_systemuser")
@JsonIgnore
public SystemuserRequest getEmailsender_systemuser() {
return new SystemuserRequest(contextPath.addSegment("emailsender_systemuser"));
}
@NavigationProperty(name="templateid")
@JsonIgnore
public TemplateRequest getTemplateid() {
return new TemplateRequest(contextPath.addSegment("templateid"));
}
@NavigationProperty(name="stageid_processstage")
@JsonIgnore
public ProcessstageRequest getStageid_processstage() {
return new ProcessstageRequest(contextPath.addSegment("stageid_processstage"));
}
@NavigationProperty(name="regardingobjectid_contact_email")
@JsonIgnore
public ContactRequest getRegardingobjectid_contact_email() {
return new ContactRequest(contextPath.addSegment("regardingobjectid_contact_email"));
}
@NavigationProperty(name="Email_QueueItem")
@JsonIgnore
public QueueitemCollectionRequest getEmail_QueueItem() {
return new QueueitemCollectionRequest(
contextPath.addSegment("Email_QueueItem"));
}
@NavigationProperty(name="parentactivityid")
@JsonIgnore
public EmailRequest getParentactivityid() {
return new EmailRequest(contextPath.addSegment("parentactivityid"));
}
@NavigationProperty(name="email_email_parentactivityid")
@JsonIgnore
public EmailCollectionRequest getEmail_email_parentactivityid() {
return new EmailCollectionRequest(
contextPath.addSegment("email_email_parentactivityid"));
}
@NavigationProperty(name="ownerid_email")
@JsonIgnore
public PrincipalRequest getOwnerid_email() {
return new PrincipalRequest(contextPath.addSegment("ownerid_email"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Email patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Email _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Email put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Email _x = _copy();
_x.changedFields = null;
return _x;
}
private Email _copy() {
Email _x = new Email();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.lastonholdtime = lastonholdtime;
_x.actualdurationminutes = actualdurationminutes;
_x._owningteam_value = _owningteam_value;
_x.exchangeitemid = exchangeitemid;
_x.ismapiprivate = ismapiprivate;
_x.createdon = createdon;
_x.seriesid = seriesid;
_x.leftvoicemail = leftvoicemail;
_x.deliverylastattemptedon = deliverylastattemptedon;
_x.isbilled = isbilled;
_x.isworkflowcreated = isworkflowcreated;
_x._sendermailboxid_value = _sendermailboxid_value;
_x.description = description;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.onholdtime = onholdtime;
_x._modifiedby_value = _modifiedby_value;
_x.community = community;
_x.activityid = activityid;
_x.sortdate = sortdate;
_x.instancetypecode = instancetypecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.versionnumber = versionnumber;
_x.processid = processid;
_x.scheduledend = scheduledend;
_x.prioritycode = prioritycode;
_x._slaid_value = _slaid_value;
_x.stageid = stageid;
_x.actualstart = actualstart;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._owninguser_value = _owninguser_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.exchangeweblink = exchangeweblink;
_x.scheduleddurationminutes = scheduleddurationminutes;
_x.senton = senton;
_x.scheduledstart = scheduledstart;
_x.statecode = statecode;
_x.subject = subject;
_x.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.isregularactivity = isregularactivity;
_x.deliveryprioritycode = deliveryprioritycode;
_x.activityadditionalparams = activityadditionalparams;
_x.traversedpath = traversedpath;
_x._createdby_value = _createdby_value;
_x.activitytypecode = activitytypecode;
_x._ownerid_value = _ownerid_value;
_x.modifiedon = modifiedon;
_x._slainvokedid_value = _slainvokedid_value;
_x.statuscode = statuscode;
_x.actualend = actualend;
_x.reminderactioncardid = reminderactioncardid;
_x.trackingtoken = trackingtoken;
_x.overriddencreatedon = overriddencreatedon;
_x.readreceiptrequested = readreceiptrequested;
_x.messageid = messageid;
_x.compressed = compressed;
_x.emailremindertext = emailremindertext;
_x.emailtrackingid = emailtrackingid;
_x.emailreminderexpirytime = emailreminderexpirytime;
_x.attachmentopencount = attachmentopencount;
_x.isunsafe = isunsafe;
_x.subcategory = subcategory;
_x._parentactivityid_value = _parentactivityid_value;
_x.postponeemailprocessinguntil = postponeemailprocessinguntil;
_x.category = category;
_x.replycount = replycount;
_x.directioncode = directioncode;
_x.correlationmethod = correlationmethod;
_x._sendersaccount_value = _sendersaccount_value;
_x.emailreminderstatus = emailreminderstatus;
_x.linksclickedcount = linksclickedcount;
_x.submittedby = submittedby;
_x.deliveryreceiptrequested = deliveryreceiptrequested;
_x.notifications = notifications;
_x.conversationtrackingid = conversationtrackingid;
_x.deliveryattempts = deliveryattempts;
_x.opencount = opencount;
_x.torecipients = torecipients;
_x.emailremindertype = emailremindertype;
_x.delayedemailsendtime = delayedemailsendtime;
_x.mimetype = mimetype;
_x.followemailuserpreference = followemailuserpreference;
_x._templateid_value = _templateid_value;
_x._emailsender_value = _emailsender_value;
_x.inreplyto = inreplyto;
_x.attachmentcount = attachmentcount;
_x.lastopenedtime = lastopenedtime;
_x.importsequencenumber = importsequencenumber;
_x.isemailreminderset = isemailreminderset;
_x.isemailfollowed = isemailfollowed;
_x.conversationindex = conversationindex;
_x.baseconversationindexhash = baseconversationindexhash;
_x.sender = sender;
return _x;
}
@Action(name = "DeliverImmediatePromoteEmail")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped deliverImmediatePromoteEmail(String messageId, String subject, String from, String to, String cc, String bcc, OffsetDateTime receivedOn, String submittedBy, String importance, String body, List attachmentIds, String eWSUrl, String attachmentToken, Crmbaseentity extraProperties) {
Preconditions.checkNotNull(messageId, "messageId cannot be null");
Preconditions.checkNotNull(from, "from cannot be null");
Preconditions.checkNotNull(to, "to cannot be null");
Preconditions.checkNotNull(cc, "cc cannot be null");
Preconditions.checkNotNull(bcc, "bcc cannot be null");
Preconditions.checkNotNull(receivedOn, "receivedOn cannot be null");
Preconditions.checkNotNull(submittedBy, "submittedBy cannot be null");
Preconditions.checkNotNull(importance, "importance cannot be null");
Preconditions.checkNotNull(body, "body cannot be null");
Map _parameters = ParameterMap
.put("MessageId", "Edm.String", Checks.checkIsAscii(messageId))
.put("Subject", "Edm.String", Checks.checkIsAscii(subject))
.put("From", "Edm.String", Checks.checkIsAscii(from))
.put("To", "Edm.String", Checks.checkIsAscii(to))
.put("Cc", "Edm.String", Checks.checkIsAscii(cc))
.put("Bcc", "Edm.String", Checks.checkIsAscii(bcc))
.put("ReceivedOn", "Edm.DateTimeOffset", receivedOn)
.put("SubmittedBy", "Edm.String", Checks.checkIsAscii(submittedBy))
.put("Importance", "Edm.String", Checks.checkIsAscii(importance))
.put("Body", "Edm.String", Checks.checkIsAscii(body))
.put("AttachmentIds", "Collection(Edm.String)", Checks.checkIsAscii(attachmentIds))
.put("EWSUrl", "Edm.String", Checks.checkIsAscii(eWSUrl))
.put("AttachmentToken", "Edm.String", Checks.checkIsAscii(attachmentToken))
.put("ExtraProperties", "Microsoft.Dynamics.CRM.crmbaseentity", extraProperties)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.DeliverImmediatePromoteEmail"), Email.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Action(name = "DeliverPromoteEmail")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped deliverPromoteEmail(String messageId, String subject, String from, String to, String cc, String bcc, OffsetDateTime receivedOn, String submittedBy, String importance, String body, List attachments, Crmbaseentity extraProperties) {
Preconditions.checkNotNull(messageId, "messageId cannot be null");
Preconditions.checkNotNull(from, "from cannot be null");
Preconditions.checkNotNull(to, "to cannot be null");
Preconditions.checkNotNull(cc, "cc cannot be null");
Preconditions.checkNotNull(bcc, "bcc cannot be null");
Preconditions.checkNotNull(receivedOn, "receivedOn cannot be null");
Preconditions.checkNotNull(submittedBy, "submittedBy cannot be null");
Preconditions.checkNotNull(importance, "importance cannot be null");
Preconditions.checkNotNull(body, "body cannot be null");
Preconditions.checkNotNull(attachments, "attachments cannot be null");
Map _parameters = ParameterMap
.put("MessageId", "Edm.String", Checks.checkIsAscii(messageId))
.put("Subject", "Edm.String", Checks.checkIsAscii(subject))
.put("From", "Edm.String", Checks.checkIsAscii(from))
.put("To", "Edm.String", Checks.checkIsAscii(to))
.put("Cc", "Edm.String", Checks.checkIsAscii(cc))
.put("Bcc", "Edm.String", Checks.checkIsAscii(bcc))
.put("ReceivedOn", "Edm.DateTimeOffset", receivedOn)
.put("SubmittedBy", "Edm.String", Checks.checkIsAscii(submittedBy))
.put("Importance", "Edm.String", Checks.checkIsAscii(importance))
.put("Body", "Edm.String", Checks.checkIsAscii(body))
.put("Attachments", "Collection(Microsoft.Dynamics.CRM.attachment)", attachments)
.put("ExtraProperties", "Microsoft.Dynamics.CRM.crmbaseentity", extraProperties)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.DeliverPromoteEmail"), Email.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Action(name = "SendEmail")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped sendEmail(Boolean issueSend, String trackingToken) {
Preconditions.checkNotNull(issueSend, "issueSend cannot be null");
Map _parameters = ParameterMap
.put("IssueSend", "Edm.Boolean", issueSend)
.put("TrackingToken", "Edm.String", Checks.checkIsAscii(trackingToken))
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.SendEmail"), SendEmailResponse.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Email[");
b.append("lastonholdtime=");
b.append(this.lastonholdtime);
b.append(", ");
b.append("actualdurationminutes=");
b.append(this.actualdurationminutes);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("exchangeitemid=");
b.append(this.exchangeitemid);
b.append(", ");
b.append("ismapiprivate=");
b.append(this.ismapiprivate);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("seriesid=");
b.append(this.seriesid);
b.append(", ");
b.append("leftvoicemail=");
b.append(this.leftvoicemail);
b.append(", ");
b.append("deliverylastattemptedon=");
b.append(this.deliverylastattemptedon);
b.append(", ");
b.append("isbilled=");
b.append(this.isbilled);
b.append(", ");
b.append("isworkflowcreated=");
b.append(this.isworkflowcreated);
b.append(", ");
b.append("_sendermailboxid_value=");
b.append(this._sendermailboxid_value);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("_regardingobjectid_value=");
b.append(this._regardingobjectid_value);
b.append(", ");
b.append("onholdtime=");
b.append(this.onholdtime);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("community=");
b.append(this.community);
b.append(", ");
b.append("activityid=");
b.append(this.activityid);
b.append(", ");
b.append("sortdate=");
b.append(this.sortdate);
b.append(", ");
b.append("instancetypecode=");
b.append(this.instancetypecode);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("scheduledend=");
b.append(this.scheduledend);
b.append(", ");
b.append("prioritycode=");
b.append(this.prioritycode);
b.append(", ");
b.append("_slaid_value=");
b.append(this._slaid_value);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("actualstart=");
b.append(this.actualstart);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("exchangeweblink=");
b.append(this.exchangeweblink);
b.append(", ");
b.append("scheduleddurationminutes=");
b.append(this.scheduleddurationminutes);
b.append(", ");
b.append("senton=");
b.append(this.senton);
b.append(", ");
b.append("scheduledstart=");
b.append(this.scheduledstart);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("subject=");
b.append(this.subject);
b.append(", ");
b.append("postponeactivityprocessinguntil=");
b.append(this.postponeactivityprocessinguntil);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("isregularactivity=");
b.append(this.isregularactivity);
b.append(", ");
b.append("deliveryprioritycode=");
b.append(this.deliveryprioritycode);
b.append(", ");
b.append("activityadditionalparams=");
b.append(this.activityadditionalparams);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("activitytypecode=");
b.append(this.activitytypecode);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("_slainvokedid_value=");
b.append(this._slainvokedid_value);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("actualend=");
b.append(this.actualend);
b.append(", ");
b.append("reminderactioncardid=");
b.append(this.reminderactioncardid);
b.append(", ");
b.append("trackingtoken=");
b.append(this.trackingtoken);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("readreceiptrequested=");
b.append(this.readreceiptrequested);
b.append(", ");
b.append("messageid=");
b.append(this.messageid);
b.append(", ");
b.append("compressed=");
b.append(this.compressed);
b.append(", ");
b.append("emailremindertext=");
b.append(this.emailremindertext);
b.append(", ");
b.append("emailtrackingid=");
b.append(this.emailtrackingid);
b.append(", ");
b.append("emailreminderexpirytime=");
b.append(this.emailreminderexpirytime);
b.append(", ");
b.append("attachmentopencount=");
b.append(this.attachmentopencount);
b.append(", ");
b.append("isunsafe=");
b.append(this.isunsafe);
b.append(", ");
b.append("subcategory=");
b.append(this.subcategory);
b.append(", ");
b.append("_parentactivityid_value=");
b.append(this._parentactivityid_value);
b.append(", ");
b.append("postponeemailprocessinguntil=");
b.append(this.postponeemailprocessinguntil);
b.append(", ");
b.append("category=");
b.append(this.category);
b.append(", ");
b.append("replycount=");
b.append(this.replycount);
b.append(", ");
b.append("directioncode=");
b.append(this.directioncode);
b.append(", ");
b.append("correlationmethod=");
b.append(this.correlationmethod);
b.append(", ");
b.append("_sendersaccount_value=");
b.append(this._sendersaccount_value);
b.append(", ");
b.append("emailreminderstatus=");
b.append(this.emailreminderstatus);
b.append(", ");
b.append("linksclickedcount=");
b.append(this.linksclickedcount);
b.append(", ");
b.append("submittedby=");
b.append(this.submittedby);
b.append(", ");
b.append("deliveryreceiptrequested=");
b.append(this.deliveryreceiptrequested);
b.append(", ");
b.append("notifications=");
b.append(this.notifications);
b.append(", ");
b.append("conversationtrackingid=");
b.append(this.conversationtrackingid);
b.append(", ");
b.append("deliveryattempts=");
b.append(this.deliveryattempts);
b.append(", ");
b.append("opencount=");
b.append(this.opencount);
b.append(", ");
b.append("torecipients=");
b.append(this.torecipients);
b.append(", ");
b.append("emailremindertype=");
b.append(this.emailremindertype);
b.append(", ");
b.append("delayedemailsendtime=");
b.append(this.delayedemailsendtime);
b.append(", ");
b.append("mimetype=");
b.append(this.mimetype);
b.append(", ");
b.append("followemailuserpreference=");
b.append(this.followemailuserpreference);
b.append(", ");
b.append("_templateid_value=");
b.append(this._templateid_value);
b.append(", ");
b.append("_emailsender_value=");
b.append(this._emailsender_value);
b.append(", ");
b.append("inreplyto=");
b.append(this.inreplyto);
b.append(", ");
b.append("attachmentcount=");
b.append(this.attachmentcount);
b.append(", ");
b.append("lastopenedtime=");
b.append(this.lastopenedtime);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("isemailreminderset=");
b.append(this.isemailreminderset);
b.append(", ");
b.append("isemailfollowed=");
b.append(this.isemailfollowed);
b.append(", ");
b.append("conversationindex=");
b.append(this.conversationindex);
b.append(", ");
b.append("baseconversationindexhash=");
b.append(this.baseconversationindexhash);
b.append(", ");
b.append("sender=");
b.append(this.sender);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy